

Techniques for Solving Array Problems
Array challenges and problems.
In the world of programming, arrays are versatile and essential data structures, but they often come with their own set of challenges. From searching for elements to optimizing performance, solving array problems efficiently is a valuable skill for any developer. In this guide, we will explore common array challenges and provide techniques to overcome them.
Duplicate Elements in an Array
One common challenge is identifying and handling duplicate elements within an array. Whether you're working with user data or need to remove redundant values, you can use techniques like hash sets or sorting to detect and eliminate duplicates.
Example: Removing Duplicates in Python
Finding an element in an unsorted array.
Searching for an element in an unsorted array can be inefficient. Utilize techniques like linear search to locate an element by iterating through the array one element at a time.
Example: Linear Search in C++
Optimizing Array Traversal
Efficiently traversing arrays is crucial for improving program performance. Utilize techniques like caching or parallelization to speed up array processing, especially when dealing with large datasets.
Handling Array Edge Cases
Array challenges often involve managing edge cases, such as empty arrays or arrays with a single element. Always consider these scenarios when designing your array-related algorithms.
Sorting Arrays
Sorting is a fundamental operation when working with arrays. Techniques like quicksort, mergesort, or the built-in sorting functions in your programming language can help you organize array elements efficiently.
Example: Quicksort in Python
Handling Multi-dimensional Arrays
When working with multi-dimensional arrays, techniques like nested loops and matrix operations can simplify complex tasks like matrix multiplication or image processing.
Solving array challenges and problems is a critical aspect of programming. This guide has explored various techniques, provided code examples, and offered strategies for tackling common array-related issues. By mastering these techniques, you'll become a more proficient and resourceful developer, equipped to handle a wide range of programming tasks.
As you continue your programming journey, remember that array challenges are a stepping stone to more complex data structures and problem-solving. Practice and experience will make you even more adept at handling arrays and other programming challenges.

Hi, I'm Ada, your personal AI tutor. I can help you with any coding tutorial. Go ahead and ask me anything.
I have a question about this topic
Give more examples
Array cheatsheet for coding interviews
Introduction .
Arrays hold values of the same type at contiguous memory locations. In an array, we're usually concerned about two things - the position/index of an element and the element itself. Different programming languages implement arrays under the hood differently and can affect the time complexity of operations you make to the array. In some languages like Python, JavaScript, Ruby, PHP, the array (or list in Python) size is dynamic and you do not need to have a size defined beforehand when creating the array. As a result, people usually have an easier time using these languages for interviews.
Arrays are among the most common data structures encountered during interviews. Questions which ask about other topics would likely involve arrays/sequences as well. Mastery of array is essential for interviews!
- Store multiple elements of the same type with one single variable name
- Accessing elements is fast as long as you have the index, as opposed to linked lists where you have to traverse from the head.
Disadvantages
- Addition and removal of elements into/from the middle of an array is slow because the remaining elements need to be shifted to accommodate the new/missing element. An exception to this is if the position to be inserted/removed is at the end of the array.
- For certain languages where the array size is fixed, it cannot alter its size after initialization. If an insertion causes the total number of elements to exceed the size, a new array has to be allocated and the existing elements have to be copied over. The act of creating a new array and transferring elements over takes O(n) time.
Learning resources
- Array in Data Structure: What is, Arrays Operations , Guru99
- Arrays , University of California San Diego
Common terms
Common terms you see when doing problems involving arrays:
- Example: given an array [2, 3, 6, 1, 5, 4] , [3, 6, 1] is a subarray while [3, 1, 5] is not a subarray.
- Example: given an array [2, 3, 6, 1, 5, 4] , [3, 1, 5] is a subsequence but [3, 5, 1] is not a subsequence.
Time complexity
Things to look out for during interviews .
- Clarify if there are duplicate values in the array. Would the presence of duplicate values affect the answer? Does it make the question simpler or harder?
- When using an index to iterate through array elements, be careful not to go out of bounds.
- Be mindful about slicing or concatenating arrays in your code. Typically, slicing and concatenating arrays would take O(n) time. Use start and end indices to demarcate a subarray/range where possible.
Corner cases
- Empty sequence
- Sequence with 1 or 2 elements
- Sequence with repeated elements
- Duplicated values in the sequence
Techniques
Note that because both arrays and strings are sequences (a string is an array of characters), most of the techniques here will apply to string problems.
Sliding window
Master the sliding window technique that applies to many subarray/substring problems. In a sliding window, the two pointers usually move in the same direction will never overtake each other. This ensures that each value is only visited at most twice and the time complexity is still O(n). Examples: Longest Substring Without Repeating Characters , Minimum Size Subarray Sum , Minimum Window Substring
Two pointers
Two pointers is a more general version of sliding window where the pointers can cross each other and can be on different arrays. Examples: Sort Colors , Palindromic Substrings
When you are given two arrays to process, it is common to have one index per array (pointer) to traverse/compare the both of them, incrementing one of the pointers when relevant. For example, we use this approach to merge two sorted arrays. Examples: Merge Sorted Array
Traversing from the right
Sometimes you can traverse the array starting from the right instead of the conventional approach of from the left. Examples: Daily Temperatures , Number of Visible People in a Queue
Sorting the array
Is the array sorted or partially sorted? If it is, some form of binary search should be possible. This also usually means that the interviewer is looking for a solution that is faster than O(n).
Can you sort the array? Sometimes sorting the array first may significantly simplify the problem. Obviously this would not work if the order of array elements need to be preserved. Examples: Merge Intervals , Non-overlapping Intervals
Precomputation
For questions where summation or multiplication of a subarray is involved, pre-computation using hashing or a prefix/suffix sum/product might be useful. Examples: Product of Array Except Self , Minimum Size Subarray Sum , LeetCode questions tagged "prefix-sum"
Index as a hash key
If you are given a sequence and the interviewer asks for O(1) space, it might be possible to use the array itself as a hash table. For example, if the array only has values from 1 to N, where N is the length of the array, negate the value at that index (minus one) to indicate presence of that number. Examples: First Missing Positive , Daily Temperatures
Traversing the array more than once
This might be obvious, but traversing the array twice/thrice (as long as fewer than n times) is still O(n). Sometimes traversing the array more than once can help you solve the problem while keeping the time complexity to O(n).
Essential questions
These are essential questions to practice if you're studying for this topic.
- Best Time to Buy and Sell Stock
- Product of Array Except Self
- Maximum Subarray
Recommended practice questions
These are recommended questions to practice after you have studied for the topic and have practiced the essential questions.
- Contains Duplicate
- Maximum Product Subarray
- Search in Rotated Sorted Array
- Container With Most Water
- Sliding Window Maximum
Recommended courses
Algomonster .
AlgoMonster aims to help you ace the technical interview in the shortest time possible . By Google engineers, AlgoMonster uses a data-driven approach to teach you the most useful key question patterns and has contents to help you quickly revise basic data structures and algorithms. Best of all, AlgoMonster is not subscription-based - pay a one-time fee and get lifetime access . Join today for a 70% discount →
Grokking the Coding Interview: Patterns for Coding Questions
This course on by Design Gurus expands upon the questions on the recommended practice questions but approaches the practicing from a questions pattern perspective, which is an approach I also agree with for learning and have personally used to get better at coding interviews. The course allows you to practice selected questions in Java, Python, C++, JavaScript and also provides sample solutions in those languages along with step-by-step visualizations. Learn and understand patterns, not memorize answers! Get lifetime access now →
Master the Coding Interview: Data Structures + Algorithms
This Udemy bestseller is one of the highest-rated interview preparation course (4.6 stars, 21.5k ratings, 135k students) and packs 19 hours worth of contents into it. Like Tech Interview Handbook, it goes beyond coding interviews and covers resume, non-technical interviews, negotiations. It's an all-in-one package! Note that JavaScript is being used for the coding demos. Check it out →
Table of Contents
- Introduction
- Learning resources
- Common terms
- Time complexity
- Things to look out for during interviews
- Corner cases
- Essential questions
- Recommended practice questions
- Recommended courses
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
Java Arrays
Java Multidimensional Arrays
Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
Java Math max()
- Java Math min()
- Java ArrayList trimToSize()
An array is a collection of similar types of data.
For example, if we want to store the names of 100 people then we can create an array of the string type that can store 100 names.
Here, the above array cannot store more than 100 names. The number of values in a Java array is always fixed.
- How to declare an array in Java?
In Java, here is how we can declare an array.
- dataType - it can be primitive data types like int , char , double , byte , etc. or Java objects
- arrayName - it is an identifier
For example,
Here, data is an array that can hold values of type double .
But, how many elements can array this hold?
Good question! To define the number of elements that an array can hold, we have to allocate memory for the array in Java. For example,
Here, the array can store 10 elements. We can also say that the size or length of the array is 10.
In Java, we can declare and allocate the memory of an array in one single statement. For example,
- How to Initialize Arrays in Java?
In Java, we can initialize arrays during declaration. For example,
Here, we have created an array named age and initialized it with the values inside the curly brackets.
Note that we have not provided the size of the array. In this case, the Java compiler automatically specifies the size by counting the number of elements in the array (i.e. 5).
In the Java array, each memory location is associated with a number. The number is known as an array index. We can also initialize arrays in Java, using the index number. For example,
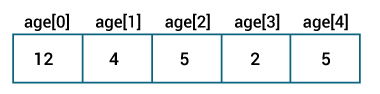
- Array indices always start from 0. That is, the first element of an array is at index 0.
- If the size of an array is n , then the last element of the array will be at index n-1 .
- How to Access Elements of an Array in Java?
We can access the element of an array using the index number. Here is the syntax for accessing elements of an array,
Let's see an example of accessing array elements using index numbers.
Example: Access Array Elements
In the above example, notice that we are using the index number to access each element of the array.
We can use loops to access all the elements of the array at once.
- Looping Through Array Elements
In Java, we can also loop through each element of the array. For example,
Example: Using For Loop
In the above example, we are using the for Loop in Java to iterate through each element of the array. Notice the expression inside the loop,
Here, we are using the length property of the array to get the size of the array.
We can also use the for-each loop to iterate through the elements of an array. For example,
Example: Using the for-each Loop
- Example: Compute Sum and Average of Array Elements
In the above example, we have created an array of named numbers . We have used the for...each loop to access each element of the array.
Inside the loop, we are calculating the sum of each element. Notice the line,
Here, we are using the length attribute of the array to calculate the size of the array. We then calculate the average using:
As you can see, we are converting the int value into double . This is called type casting in Java. To learn more about typecasting, visit Java Type Casting .
- Multidimensional Arrays
Arrays we have mentioned till now are called one-dimensional arrays. However, we can declare multidimensional arrays in Java.
A multidimensional array is an array of arrays. That is, each element of a multidimensional array is an array itself. For example,
Here, we have created a multidimensional array named matrix. It is a 2-dimensional array. To learn more, visit the Java multidimensional array .
- Java Copy Array
- Java Program to Print an Array
- Java Program to Concatenate two Arrays
- Java ArrayList to Array and Array to ArrayList
- Java Dynamic Array
Table of Contents
- Introduction
Sorry about that.

Related Tutorials
Java Tutorial
Java Library
Java Tutorial
Java methods, java classes, java file handling, java how to's, java reference, java examples, java arrays.
Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value.
To declare an array, define the variable type with square brackets :
We have now declared a variable that holds an array of strings. To insert values to it, you can place the values in a comma-separated list, inside curly braces:
To create an array of integers, you could write:
Access the Elements of an Array
You can access an array element by referring to the index number.
This statement accesses the value of the first element in cars:
Try it Yourself »
Note: Array indexes start with 0: [0] is the first element. [1] is the second element, etc.
Change an Array Element
To change the value of a specific element, refer to the index number:
Array Length
To find out how many elements an array has, use the length property:
Test Yourself With Exercises
Create an array of type String called cars .
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
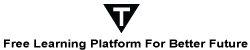
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Two Pointers
- Two Sum Problem
- Three Sum Problem
- Smallest Difference Pair
- Remove duplicates from sorted array
- Remove duplicates from sorted array II
- Squares of a Sorted array
- Find all Triplets with zero sum
- Triplet sum closest to target
Two Number Sum Problem solution in Java
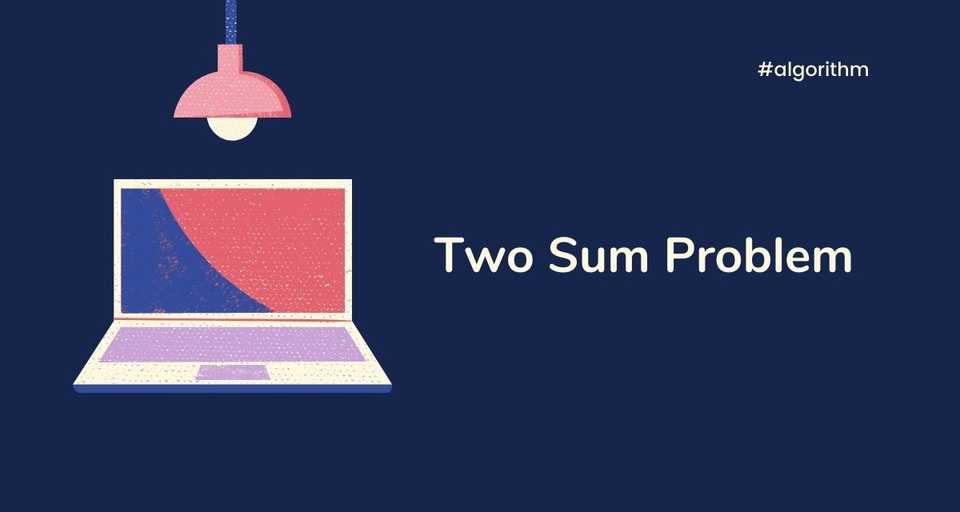
Two Number Sum Problem Statement
Given an array of integers, return the indices of the two numbers whose sum is equal to a given target.
You may assume that each input would have exactly one solution, and you may not use the same element twice.
METHOD 1. Naive approach: Use two for loops
The naive approach is to just use two nested for loops and check if the sum of any two elements in the array is equal to the given target.
Time complexity: O(n^2)
METHOD 2. Use a HashMap (Most efficient)
You can use a HashMap to solve the problem in O(n) time complexity. Here are the steps:
- Initialize an empty HashMap.
- Iterate over the elements of the array.
- If the element exists in the Map, then check if it’s complement ( target - element ) also exists in the Map or not. If the complement exists then return the indices of the current element and the complement.
- Otherwise, put the element in the Map, and move to the next iteration.
Time complexity: O(n)
METHOD 3. Use Sorting along with the two-pointer approach
There is another approach which works when you need to return the numbers instead of their indexes . Here is how it works:
- Sort the array.
- Initialize two variables, one pointing to the beginning of the array ( left ) and another pointing to the end of the array ( right ).
- if arr[left] + arr[right] == target , then return the indices.
- if arr[left] + arr[right] < target , increment the left index.
- else, decrement the right index.
This approach is called the two-pointer approach. It is a very common pattern for solving array related problems.
Time complexity: O(n*log(n))
Share on social media
- Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Segment Tree
- Disjoint Set Union
- Fenwick Tree
- Red-Black Tree
- Advanced Data Structures
- Array Data Structure Guide
- What is Array?
- Getting Started with Array Data Structure
- Applications, Advantages and Disadvantages of Array
Subarrays, Subsequences, and Subsets in Array
Basic operations in array.
- Searching in Array
- Array Reverse in C/C++/Java/Python/JavaScript
- Program for array left rotation by d positions.
- Print array after it is right rotated K times
- Search, Insert, and Delete in an Unsorted Array | Array Operations
- Search, Insert, and Delete in an Sorted Array | Array Operations
- Array | Sorting
- Generating subarrays using recursion
Easy problems on Array
- Find the largest three distinct elements in an array
- Find Second largest element in an array
- Move all zeroes to end of array
- Rearrange array such that even positioned are greater than odd
- Rearrange an array in maximum minimum form using Two Pointer Technique
- Segregate even and odd numbers using Lomuto’s Partition Scheme
- Reversal algorithm for Array rotation
- Print left rotation of array in O(n) time and O(1) space
- Sort an array which contain 1 to n values
- Count the number of possible triangles
- Print all Distinct ( Unique ) Elements in given Array
- Find the element that appears once in an array where every other element appears twice
- Leaders in an array
- Find Subarray with given sum | Set 1 (Non-negative Numbers)
Intermediate problems on Array
- Rearrange an array such that arr[i] = i
- Rearrange positive and negative numbers in O(n) time and O(1) extra space
- Reorder an array according to given indexes
- Find the smallest missing number
- Difference Array | Range update query in O(1)
- Maximum profit by buying and selling a share at most twice
- Smallest subarray with sum greater than a given value
- Inversion count in Array using Merge Sort
- Merge two sorted arrays with O(1) extra space
- Majority Element
- Two Pointers Technique
- Triplet Sum in Array (3sum)
- Equilibrium index of an array
Hard problems on Array
- MO's Algorithm (Query Square Root Decomposition) | Set 1 (Introduction)
- Square Root (Sqrt) Decomposition Algorithm
- Sparse Table
- Range sum query using Sparse Table
- Range LCM Queries
- Minimum number of jumps to reach end (Jump Game)
- Space optimization using bit manipulations
- Find maximum value of Sum( i*arr[i]) with only rotations on given array allowed
- Construct an array from its pair-sum array
- Maximum equilibrium sum in an array
- Smallest Difference Triplet from Three arrays
- Top 50 Array Coding Problems for Interviews
- What is a Subarray?
A subarray is a contiguous part of array, i.e., Subarray is an array that is inside another array.
In general, for an array of size n, there are n*(n+1)/2 non-empty subarrays.
For example, Consider the array [1, 2, 3, 4], There are 10 non-empty sub-arrays. The subarrays are:
(1), (2), (3), (4), (1,2), (2,3), (3,4), (1,2,3), (2,3,4), and (1,2,3,4)
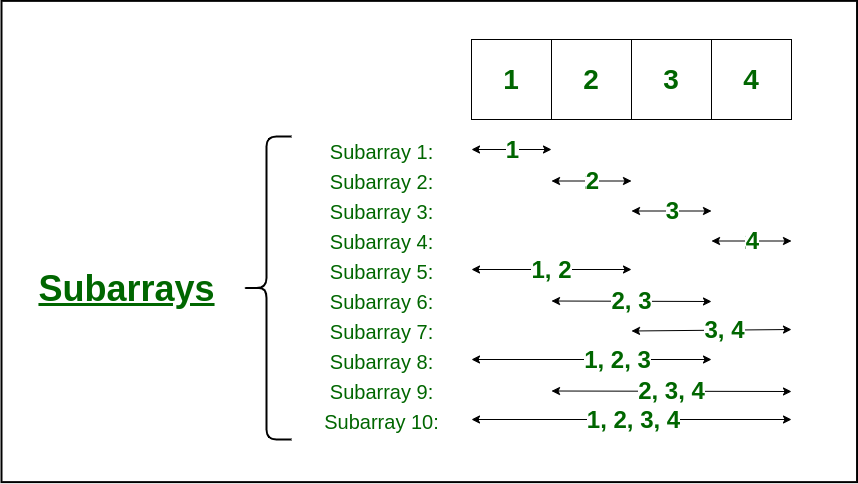
- What is a Subsequence?
A subsequence is a sequence that can be derived from another sequence by removing zero or more elements, without changing the order of the remaining elements.
More generally, we can say that for a sequence of size n, we can have (2 n – 1) non-empty sub-sequences in total.
For the same above example, there are 15 sub-sequences. They are:
(1), (2), (3), (4), (1,2), (1,3),(1,4), (2,3), (2,4), (3,4), (1,2,3), (1,2,4), (1,3,4), (2,3,4), (1,2,3,4).
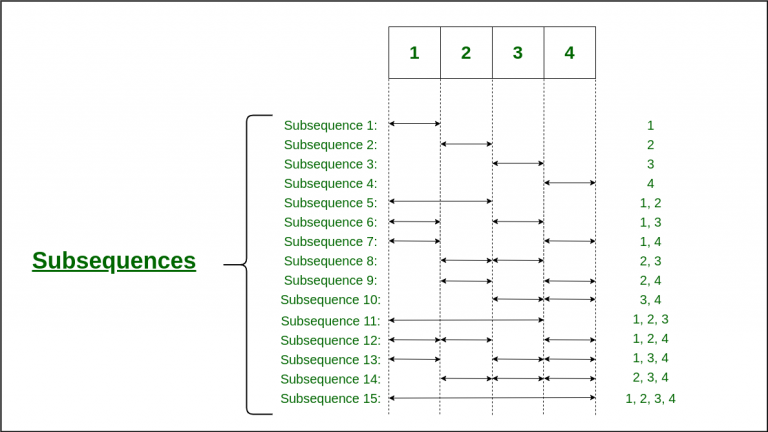
Subsequences
- What is a Subset?
If a Set has all its elements belonging to other sets, this set will be known as a subset of the other set.
A Subset is denoted as “ ⊆ “. If set A is a subset of set B, it is represented as A ⊆ B .
For example, Let Set_A = {m, n, o, p, q}, Set_ B = {k, l, m, n, o, p, q, r}
Then, A ⊆ B.
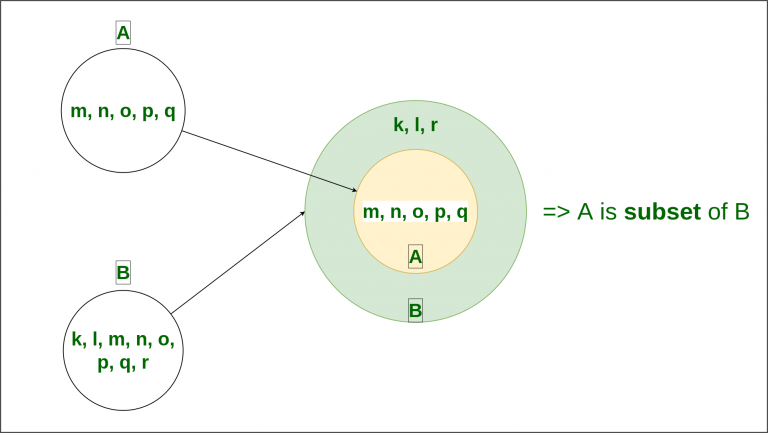
Table of Content
- Easy Problems on Subarray
- Medium Problems on Subarray
- Hard Problems on Subarray
- Easy Problems on Subsequence
- Medium Problems on Subsequence
- Hard Problems on Subsequence
- Easy Problems on Subset
- Medium Problems on Subset
- Hard Problems on Subset
Easy Problems on Subarray:
- Split an array into two equal Sum subarrays
- Check if subarray with given product exists in an array
- Subarray of size k with given sum
- Sort an array where a subarray of a sorted array is in reverse order
- Count subarrays with all elements greater than K
- Maximum length of the sub-array whose first and last elements are same
- Check whether an Array is Subarray of another Array
- Find array such that no subarray has xor zero or Y
- Maximum subsequence sum such that all elements are K distance apart
- Longest sub-array with maximum GCD
- Count of subarrays with sum at least K
- Length of Smallest subarray in range 1 to N with sum greater than a given value
- Sum of all subarrays of size K
- Split array into K disjoint subarrays such that sum of each subarray is odd.
- Find an array of size N having exactly K subarrays with sum S
- Find the subarray of size K with minimum XOR
- Length of the longest alternating even odd subarray
- Count of subarrays which start and end with the same element
- Count of subarrays having exactly K perfect square numbers
- Split array into two subarrays such that difference of their maximum is minimum
Medium Problems on Subarray:
- Print all K digit repeating numbers in a very large number
- Length of longest subarray whose sum is not divisible by integer K
- Min difference between maximum and minimum element in all Y size subarrays
- Longest subarray of non-empty cells after removal of at most a single empty cell
- First subarray with negative sum from the given Array
- Largest subarray with frequency of all elements same
- Bitwise operations on Subarrays of size K
- Count subarrays having sum of elements at even and odd positions equal
- Longest Subarray consisting of unique elements from an Array
- Minimum Decrements on Subarrays required to reduce all Array elements to zero
- Split array into two subarrays such that difference of their sum is minimum
- Maximize count of non-overlapping subarrays with sum K
- Smallest subarray which upon repetition gives the original array
- Split array into maximum subarrays such that every distinct element lies in a single subarray
- Maximize product of subarray sum with its minimum element
- Sum of products of all possible Subarrays
- Check if all subarrays contains at least one unique element
- Length of smallest subarray to be removed such that the remaining array is sorted
- Length of longest subarray having frequency of every element equal to K
- Length of the longest increasing subsequence which does not contain a given sequence as Subarray
Hard Problems on Subarray:
- Length of smallest subarray to be removed to make sum of remaining elements divisible by K
- Maximum length of same indexed subarrays from two given arrays satisfying the given condition
- Count ways to split array into two equal sum subarrays by changing sign of any one array element
- Longest subarray in which all elements are smaller than K
- Maximize product of a strictly increasing or decreasing subarray
- Sum of maximum of all subarrays by adding even frequent maximum twice
- Longest subarray of an array which is a subsequence in another array
- Count of subarrays having product as a perfect cube
- Minimize difference between maximum and minimum array elements by removing a K-length subarray
- Maximum sum submatrix
- Minimum removal of elements from end of an array required to obtain sum K
- Check if any subarray of length M repeats at least K times consecutively or not
- Minimize flips on K-length subarrays required to make all array elements equal to 1
- Split array into K subarrays such that sum of maximum of all subarrays is maximized
- Find minimum subarray sum for each index i in subarray [i, N-1]
- Longest subarray with GCD greater than 1
- Longest subsegment of ‘1’s formed by changing at most k ‘0’s
- Lexicographically smallest Permutation of Array by reversing at most one Subarray
- Find a subsequence which upon reversing gives the maximum sum subarray
- Minimize steps to make Array elements 0 by reducing same A[i] – X from Subarray
Easy Problems on Subsequence:
- Longest subsequence having equal numbers of 0 and 1
- Powers of two and subsequences
- Longest Subsequence where index of next element is arr[arr[i] + i]
- Number of subsequences with zero sum
- Longest sub-sequence with maximum GCD
- Maximum Bitwise AND value of subsequence of length K
- Length of the longest subsequence such that xor of adjacent elements is non-decreasing
- Maximum product of bitonic subsequence of size 3
- Length of Smallest Subsequence such that sum of elements is greater than equal to K
- Longest subsequence of even numbers in an Array
- Maximum length Subsequence with alternating sign and maximum Sum
- Count of possible subarrays and subsequences using given length of Array
- Maximum bitwise OR value of subsequence of length K
- Count of subsequences consisting of the same element
- Smallest occurring element in each subsequence
- Length of the longest subsequence consisting of distinct elements
- Minimize elements to be added to a given array such that it contains another given array as its subsequence
- Maximize subsequences having array elements not exceeding length of the subsequence
- Length of longest subsequence consisting of distinct adjacent elements
- Maximum Sum Subsequence
Medium Problems on Subsequence:
- Minimum removals required to make a given array Bitonic
- Check if a non-contiguous subsequence same as the given subarray exists or not
- Minimize the number of strictly increasing subsequences in an array
- Count of unique subsequences from given number which are power of 2
- Minimum number of insertions required such that first K natural numbers can be obtained as sum of a subsequence of the array
- Length of longest subsequence such that prefix sum at every element remains greater than zero
- Check if given Array can be divided into subsequences of K increasing consecutive integers
- Longest Subsequence such that difference between adjacent elements is either A or B
- Count subsequences of Array having single digit integer sum K
- Shortest Subsequence with sum exactly K
- Printing Longest Bitonic Subsequence
- Sorted subsequence of size 3 in linear time using constant space
- Count of subsequences having maximum distinct elements
- Construct array having X subsequences with maximum difference smaller than d
- Print all subsequences of a string using ArrayList
- Longest Subsequence with at least one common digit in every element
- Maximum Sum Subsequence of length k
- Sum of minimum element of all sub-sequences of a sorted array
- Find all combinations of two equal sum subsequences
- Minimum cost of choosing 3 increasing elements in an array of size N
Hard Problems on Subsequence:
- Number of subsequences with positive product
- Longest subsequence having difference atmost K
- Find all subsequences with sum equals to K
- Maximize product of digit sum of consecutive pairs in a subsequence of length K
- Count of subsequences of length atmost K containing distinct prime elements
- Sum of all subsequences of length K
- Minimize sum of smallest elements from K subsequences of length L
- Unique subsequences of length K with given sum
- Smallest subsequence with sum of absolute difference of consecutive elements maximized
- Maximize product of same-indexed elements of same size subsequences
- Longest Increasing Subsequence having sum value atmost K
- Longest subsequence of a number having same left and right rotation
- Maximize length of Non-Decreasing Subsequence by reversing at most one Subarray
- Maximum subsequence sum possible by multiplying each element by its index
- Generate all distinct subsequences of array using backtracking
- Maximum subsequence sum such that no K elements are consecutive
- Print all possible K-length subsequences of first N natural numbers with sum N
- Longest subsequence having difference between the maximum and minimum element equal to K
- Maximize difference between sum of even and odd-indexed elements of a subsequence
- Convert an array into another by repeatedly removing the last element and placing it at any arbitrary index
Easy Problems on Subset:
- Find if there is any subset of size K with 0 sum in an array of -1 and +1
- Sum of sum of all subsets of a set formed by first N natural numbers
- Count of subsets not containing adjacent elements
- Sum of the sums of all possible subsets
- Find whether an array is subset of another array
- Total number of Subsets of size at most K
- Check if it is possible to split given Array into K odd-sum subsets
- Partition a set into two subsets such that difference between max of one and min of other is minimized
- Sum of all possible expressions of a numeric string possible by inserting addition operators
- Check if it’s possible to split the Array into strictly increasing subsets of size at least K
- Largest subset of Array having sum at least 0
Medium Problems on Subset:
- Number of subsets with sum divisible by m
- Fibonacci sum of a subset with all elements <= k
- Number of possible Equivalence Relations on a finite set
- Largest divisible pairs subset
- Recursive program to print all subsets with given sum
- Subset Sum Queries in a Range using Bitset
- Find all distinct subset (or subsequence) sums of an array | Set-2
- Sum of (maximum element – minimum element) for all the subsets of an array
- Count no. of ordered subsets having a particular XOR value
- Sum of subsets of all the subsets of an array
- Perfect Sum Problem
- Count of subsets having sum of min and max element less than K
- Split array into two equal length subsets such that all repetitions of a number lies in a single subset
- Nth Subset of the Sequence consisting of powers of K in increasing order of their Sum
- Largest possible Subset from an Array such that no element is K times any other element in the Subset
- Check if an array can be split into subsets of K consecutive elements
Hard Problems on Subset:
- Minimize count of divisions by D to obtain at least K equal array elements
- Split array into K-length subsets to minimize sum of second smallest element of each subset
- Median of all non-empty subset sums
- Minimum removals required such that sum of remaining array modulo M is X
- Sum of length of two smallest subsets possible from a given array with sum at least K
- Reduce sum of any subset of an array to 1 by multiplying all its elements by any value
- Sum of all subsets whose sum is a Perfect Number from a given array
- Minimize sum of incompatibilities of K equal-length subsets made up of unique elements
- Maximize sum of subsets from two arrays having no consecutive values
- Product of the maximums of all subsets of an array
- Count ways to place ‘+’ and ‘-‘ in front of array elements to obtain sum K
- Count ways to split array into two subsets having difference between their sum equal to K
- Find the subset of Array with given LCM
- Count of subsets whose product is multiple of unique primes
- Minimum count of elements to be inserted in Array to form all values in [1, K] using subset sum
- Maximum subset sum having difference between its maximum and minimum in range [L, R]
- Find all unique subsets of a given set using C++ STL
- Subset sum problem where Array sum is at most N
Related Articles:
Data Structure and Algorithms Course Recent articles on Subarray Recent articles on Subsequence Recent articles on Subset
Please Login to comment...
Similar reads.
- subsequence
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Data Structures
Java Arraylist
Sometimes it's better to use dynamic size arrays. Java's Arraylist can provide you this feature. Try to solve this problem using Arraylist.
You are given lines. In each line there are zero or more integers. You need to answer a few queries where you need to tell the number located in position of line.
Take your input from System.in.
Input Format The first line has an integer . In each of the next lines there will be an integer denoting number of integers on that line and then there will be space-separated integers. In the next line there will be an integer denoting number of queries. Each query will consist of two integers and .
Constraints
Each number will fit in signed integer. Total number of integers in lines will not cross .
Output Format In each line, output the number located in position of line. If there is no such position, just print "ERROR!"
Sample Input
Sample Output
Explanation
The diagram below explains the queries:

Cookie support is required to access HackerRank
Seems like cookies are disabled on this browser, please enable them to open this website

IMAGES
VIDEO
COMMENTS
Java Array Exercises [79 exercises with solution] [ An editor is available at the bottom of the page to write and execute the scripts. Go to the editor] 1. Write a Java program to sort a numeric array and a string array. Click me to see the solution. 2. Write a Java program to sum values of an array. Click me to see the solution.
String in Java are the objects which can store characters of values in Java, it act the same as an array of characters in Java. Java String is one of the most important topics in Java programming. It is widely used to manipulate and process textual data. In this article, we will learn about Java String with some Java Practice Problems. Take a look
The key to solving array-based questions is having a good knowledge of array data structure as well as basic programming constructors such as loop, recursion, and fundamental operators.
Find the first repeating element in an array of integers. Solve. Find the first non-repeating element in a given array of integers. Solve. Subarrays with equal 1s and 0s. Solve. Rearrange the array in alternating positive and negative items. Solve. Find if there is any subarray with a sum equal to zero.
Java Array Programs. An array is a data structure consisting of a collection of elements (values or variables), of the same memory size, each identified by at least one array index or key. An array is a linear data structure that stores similar elements (i.e. elements of similar data type) that are stored in contiguous memory locations.
Techniques like quicksort, mergesort, or the built-in sorting functions in your programming language can help you organize array elements efficiently. Example: Quicksort in Python. if len(arr) <= 1: return arr. pivot = arr[len(arr) // 2] left = [x for x in arr if x < pivot] middle = [x for x in arr if x == pivot]
Java Arrays Practice Set: In this video, we will see few questions on Java Arrays. We will see some good concepts like how to reverse an array, how to find m...
Common terms you see when doing problems involving arrays: Subarray - A range of contiguous values within an array. Example: given an array [2, 3, 6, 1, 5, 4], [3, 6, 1] is a subarray while [3, 1, 5] is not a subarray. Subsequence - A sequence that can be derived from the given sequence by deleting some or no elements without changing the order ...
To define the number of elements that an array can hold, we have to allocate memory for the array in Java. For example, // declare an array double[] data; // allocate memory. data = new double[10]; Here, the array can store 10 elements. We can also say that the size or length of the array is 10. In Java, we can declare and allocate the memory ...
This makes it a more flexible data structure for handling collections of objects. 1. Write a Java program to create an array list, add some colors (strings) and print out the collection. Click me to see the solution. 2. Write a Java program to iterate through all elements in an array list. Click me to see the solution.
An ArrayList is a re-sizable array, also called a dynamic array. It grows its size to accommodate new elements and shrinks the size when the elements are removed. ArrayList internally uses an array to store the elements. Just like arrays, It allows you to retrieve the elements by their index. Java ArrayList allows duplicate and null values.
Practice Arrays. Solve Arrays coding problems to start learning data structures and algorithms. This curated set of 21 standard Arrays questions will give you the confidence to solve interview questions. Please login to see the progress.
In this article, we will discuss some important concepts related to arrays and problems based on that. Before understanding this, you should have basic idea about Arrays. Type 1. Based on array declaration - These are few key points on array declaration:
Solve over 180 coding problems and challenges to get better at Java. Courses. Learn Python 10 courses. Learn C++ 9 courses. Learn C 9 courses. Learn Java 9 ... Practice problems about creating and modifying arrays using Java. Problem Name: Status: Difficulty: Declare Array: NA: Guess the output: NA: Guess the output: NA: Guess the output: NA ...
Java Arrays. Arrays are used to store multiple values in a single variable, instead of declaring separate variables for each value. To declare an array, define the variable type with square brackets: We have now declared a variable that holds an array of strings. To insert values to it, you can place the values in a comma-separated list, inside ...
Java is a popular programming language that is used to develop a wide variety of applications. One of the best ways to learn Java is to practice writing programs. Many resources are available online and in libraries to help you find Java practice programs. When practising Java programs, it is important to focus on understanding the concepts ...
You can use a HashMap to solve the problem in O(n) time complexity. Here are the steps: Initialize an empty HashMap. Iterate over the elements of the array. For every element in the array - If the element exists in the Map, then check if it's complement (target - element) also exists in the Map or not. If the complement exists then return the ...
1. To solve these kind of problems you can do like, this solution can solve very easily to your problem but here we are just taking a constant space which is 256 length array and complexity will be O(n): int []star = new int[256]; while (df.hasNextLine()) {. Arrays.fill(star,0); String line = df.nextLine();
Java 1D Array. An array is a simple data structure used to store a collection of data in a contiguous block of memory. Each element in the collection is accessed using an index, and the elements are easy to find because they're stored sequentially in memory. Because the collection of elements in an array is stored as a big block of data, we ...
After completing these Java exercises you are a step closer to becoming an advanced Java programmer. We hope these exercises have helped you understand Java better and you can solve beginner to advanced-level questions on Java programming. Solving these Java programming exercise questions will not only help you master theory concepts but also ...
Easy Problems on Subarray: Split an array into two equal Sum subarrays; Check if subarray with given product exists in an array; Subarray of size k with given sum; Sort an array where a subarray of a sorted array is in reverse order; Count subarrays with all elements greater than K; Maximum length of the sub-array whose first and last elements ...
Java's Arraylist can provide you this feature. Try to solve this problem using Arraylist. You are given lines. In each line there are zero or more integers. You need to answer a few queries where you need to tell the number located in position of line. Take your input from System.in. The first line has an integer .