Kata Library: Dart Practice
- Collections

Collect: kata
Loading collection data...
You have not created any collections yet.
Collections are a way for you to organize kata so that you can create your own training routines. Every collection you create is public and automatically sharable with other warriors. After you have added a few kata to a collection you and others can train on the kata contained within the collection.
Get started now by creating a new collection .
Set the name for your new collection. Remember, this is going to be visible by everyone so think of something that others will understand.
- Skills Directory
Dart is a modern, open-source programming language developed by Google. It is used for various applications, including web development, mobile app development, and server-side programming. Dart is known for its performance, productivity, and ease of use.
These key competencies in Dart lay the foundation for becoming proficient in the language and its application in various domains, such as web development with frameworks like AngularDart and server-side programming with Dart's server frameworks. As you progress, you'll have the tools to build versatile and powerful applications with Dart.
This competency area includes understanding the fundamentals of Dart programming, including variables, functions, control flow, and object-oriented concepts.
Key Competencies:
- Dart Basics - Understanding the fundamentals of Dart programming language, including variables, data types, functions, and control flow.
- Object-Oriented Programming - Grasping the concepts of classes, objects, inheritance, and encapsulation for building reusable and organized code.
- Functions and Methods - Mastering the use of functions and methods to modularize code and implement behavior.
- Collections - Working with Dart collections like lists, sets, and maps to manage data effectively.
- Control Flow - Utilizing conditional statements and loops for decision-making and iteration tasks.
- Exception Handling - Implementing error handling mechanisms to manage exceptions in Dart programs.
Cookie support is required to access HackerRank
Seems like cookies are disabled on this browser, please enable them to open this website
Dart Academy
Learn about dart and flutter from the experts. dart academy is a growing collection of dart/flutter articles, tutorials, and videos, ranging from beginner to advanced content., building design systems in flutter.
Photo by Balázs Kétyi on UnsplashNielsen Norman Group (a UX research consultancy) defines a design system as follows: A design system is a set of standards to manage design at scale by reducing redundancy while creating a shared language and visual consistency across different
Immutable Data Patterns in Dart and Flutter
Immutable data constructs are those that cannot be mutated (altered) after they've been initialized. The Dart language is full of these. In fact, most basic variable types operate this way. Once created, strings, numbers, and boolean values cannot be mutated. A string variable doesn't
Creational Design Patterns for Dart and Flutter: Factory Method
The Factory Method pattern, sometimes referred to as the Virtual Constructor pattern, provides a way to conceal an object's creation logic from client code, but the object returned is guaranteed to adhere to a known interface. It's one of the most widely used creational
Structural Design Patterns for Dart and Flutter: Façade
In cases where you have a large number of subsystems, each with its own simple or complicated interface, it can sometimes be to your advantage to abstract away those interfaces by unifying them under one common, usually simpler interface. This is the essence of
Structural Design Patterns for Dart and Flutter: Flyweight
The Flyweight pattern is all about using memory efficiently. If your Dart or Flutter app deals with relatively heavy objects, and it needs to instantiate many of them, using this pattern can help save memory and increase performance by avoiding data duplication. Objects that
Structural Design Patterns for Dart and Flutter: Adapter
With the Adapter pattern, you create an intermediary between two incompatible class interfaces, allowing one to work with the other. This works very much like physical adapters in the real world, such as those that attach to a power cord to enable a device
Structural Design Patterns for Dart and Flutter: Composite
The general structure of the Composite pattern will be instantly familiar to Flutter developers, because Flutter's widgets are constructed with an expanded version. Regular Flutter widgets, defined as those that can have only one child, and collection Flutter widgets, defined as those that take
Structural Design Patterns for Dart and Flutter: Decorator
Using inheritance, we can statically extend the functionality of a Dart class. But what if we want more flexibility than that? We may want to add behavior to an object dynamically, perhaps as a user makes attribute selections. The Decorator pattern can help! With
Creational Design Patterns for Dart and Flutter: Singleton
When you need to ensure only a single instance of a class is created, and you want to provide a global point of access to it, the Singleton design pattern can be of great help. Examples of typical singletons include an app-wide debug logger
Creational Design Patterns for Dart and Flutter: Builder
The purpose of the Builder pattern is to separate the construction of a complex object from its representation. This is especially useful for objects with many properties, some of which may have obvious defaults, and some of which may be optional. Early object-oriented languages
Creational Design Patterns for Dart and Flutter: Prototype
Sometimes you need to create a copy, or clone, of an existing object. For mutable objects with properties that can change, you may need a separate copy of an object to avoid corrupting the original. For immutable objects, where properties can be initialized but
Streams and Sinks in Dart and Flutter
Streams and sinks are mainstays in Dart and Flutter asynchronous programming, and now it's time to explore what streams are and how they can be used to solve problems. The code for this article was tested with Dart 2.8.4 and Flutter 1.
Futures and Completers in Dart and Flutter
Even though Dart, with its convenient async/await syntax, is able to take a lot of the complexity out of managing asynchronous calls, sometimes you need to interact with a more traditional callback library or a persistent connection that doesn't operate with futures. In
Asynchronous Programming in Dart and Flutter
The UI widgets available in the Flutter framework use Dart's asynchronous programming features to great effect, helping to keep your code organized and preventing the UI from locking up on the user. In this article, we'll look at how asynchronous code patterns can help
Asynchrony Primer for Dart and Flutter
Dart is a single-threaded language. This trait is often misunderstood. Many programmers believe this means Dart can't run code in parallel, but that's not the case. So how does Dart manage and execute operations? The code for this article was tested with Dart 2.
Using Redis with Dart
Redis is a really fast key value store that has many different applications, from leaderboards to caching, Redis is like the swiss army knife of the web. Let's take a look at how easy it is to use Redis with Dart! First in your
Generics in Dart and Flutter
Flutter code uses Dart generics all over the place to ensure object types are what we expect them to be. Simply put, generics are a way to code a class or function so that it works with a range of data types instead of
Inheritance, Polymorphism, and Composition in Dart and Flutter
There is a trend in software development away from the deep, branching class trees popular with object-oriented languages. Some believe newer functional paradigms should outright replace OOP in software design. This leaves many with the idea that inheritance has no place in software construction
Creating Objects and Classes in Dart and Flutter
Dart has some conventions and special syntax to be aware of when designing classes and instantiating objects of those classes. There is more than one way to do almost anything, but what are the best practices in Dart? Here, we'll explore a few for class design and object instantiation.
Flutter For Web: A Complete Guide to Create & Run a Web Application
Source: https://cdn-images-1.medium.com/max/1600/0*gD64Y8ECWBBuSZrx At Google I/O 2019 developer conference, Google launched version 1.5 of Flutter, it's open source mobile UI framework that helps developers build native interfaces for Android and iOS. But that’s no longer
Web Games with Dart and the HTML5 Canvas: Snake
With many of the advanced organizational structures of heavy hitters like C++ and Java, but featuring simpler semantics and a lightweight syntax for common web idioms, Dart is a great language for building web games. Are you ready to learn how to write games
Web Games with Dart: Hangman
Well, here we are again, sports fans. We're going to whip out a stupid simple game application in order to demonstrate some key programming principles, as well as highlight the power and simplicity of Dart. Hangman is a game as old as time (or
How to Cancel a Dart Future
Once in a while, you need to cancel a Future in Dart code. I've seen a bit of discussion about this online, but it's not that easy to discover a solution, so I thought I'd improve that situation a bit by detailing one possible
Easy JavaScript Interop Without Dart Wrappers
Most of the Dart web developers I know do their best to avoid using JavaScript in their projects when they can, but it's not always possible. Sometimes you've just gotta have that one JS library in your project that does exactly what you need,
Build a Real-Time Chat Web App with AngularDart and Firebase
In this tutorial, you're going to build a real-time chat web app that supports Google authentication and both text and image messages, and you're going to do it without writing a single line of server-side code. "But how?" you may exclaim. Dart,
Subscribe to Dart Academy
Stay up to date! Get all the latest & greatest posts delivered straight to your inbox
- Collections
- Dart Interview Questions
- Kotlin Android
- Android with Java
- Android Studio
Dart Tutorial
- Introduction to Dart Programming Language
- Dart SDK Installation
- Dart - Comments
- Dart - Variables
- Operators in Dart
- Dart - Standard Input Output
- Dart - Data Types
- Basics of Numbers in Dart
- Strings in Dart
- Dart - Sets
- Dart Programming - Map
- Queues in Dart
- Data Enumeration in Dart
Control Flow
- Switch Case in Dart
- Dart - Loops
- Dart - Loop Control Statements (Break and Continue)
- Labels in Dart
Key Functions
- Dart - Anonymous Functions
- Dart - main() Function
- Dart - Common Collection Methods
- How to Exit a Dart Application Unconditionally?
- Dart - Getters and Setters
- Dart - Classes And Objects
Object-Oriented Programming
- Dart - this keyword
- Dart - Static Keyword
- Dart - Super and This keyword
- Dart - Concept of Inheritance
- Instance and class methods in Dart
- Method Overriding in Dart
- Getter and Setter Methods in Dart
- Abstract Classes in Dart
- Dart - Builder Class
- Concept of Callable Classes in Dart
- Interface in Dart
- Dart - extends Vs with Vs implements
- Dart - Date and Time
- Using await async in Dart
Dart Utilities
- How to Combine Lists in Dart?
- Dart - Finding Minimum and Maximum Value in a List
- Dart - Splitting of String
Dart Programs
- Dart - Sort a List
- Dart - String toUpperCase() Function with Examples
- Dart - Convert All Characters of a String in Lowercase
- How to Replace a Substring of a String in Dart?
- How to Check String is Empty or Not in Dart (Null Safety)?
- Exception Handling in Dart
- Assert Statements in Dart
- Fallthrough Condition in Dart
- Concept of Isolates in Dart
Advance Concepts
- Dart - Collections
- Dart - Basics of Packages
- Dart - String codeUnits Property
- HTML Document Object Model and Dart Programming
Dart is an open-source general-purpose programming language developed by Google. It supports application development on both the client and server side. However, it is widely used for the development of Android apps, iOS apps, IoT(Internet of Things), and web applications using the Flutter Framework .
Syntactically, Dart bears a strong resemblance to Java, C, and JavaScript. It is a dynamic object-oriented language with closure and lexical scope. The Dart language was released in 2011 but came into popularity after 2015 with Dart 2.0.
Table of Content
Dart Basics
Data types in dart, control flow in dart, dart key functions.
- OOPS in Dart
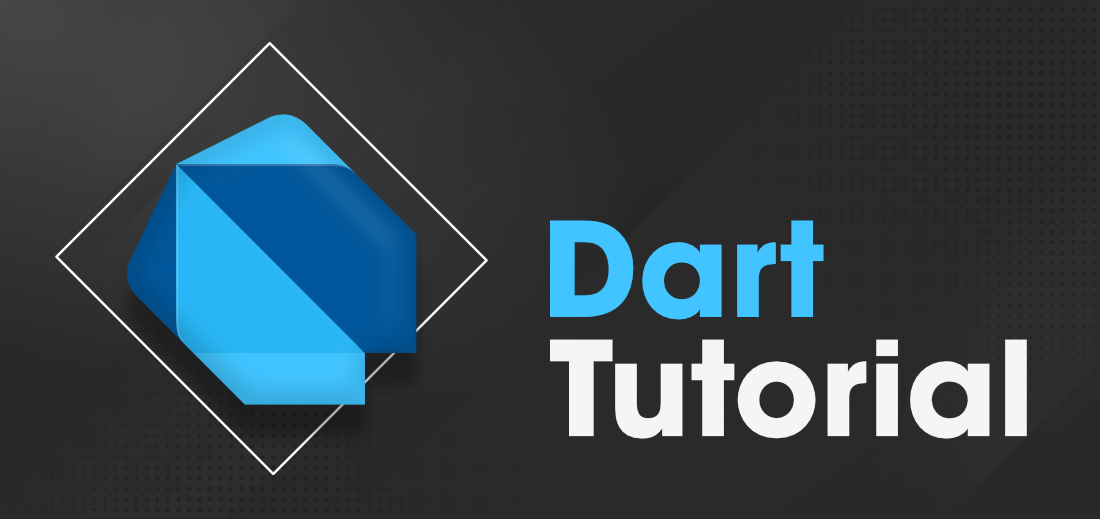
- Dart – SDK Installation
- Dart – Comments
- Dart – Variables
- Dart – Operators
- Dart – Standard Input Output
Data Types are used for defining the type of data that a variable can store. We can store multiple types of data in Dart as mentioned below:
- Dart – Data Types
- Dart – Numbers
- Dart – Strings
- Dart – List
- Dart – Sets
- Dart – Map
- Dart – Enums
Control Flow in Programming is refers to the order in which set of instructions or statements that are executed or evaluated. It provides flexibility in Decision making and makes code more user friendly.
- Dart – If-Else Statements
- Dart – Switch Case Statements
- Dart – Loops
- Dart – Loop Control Statements
Functions are used for making our Dart Programs more organised and efficient. Here, we will check how to build functions and learn about few inbuilt functions in Dart which can lower some of the loads from us.
- Dart – Function
- Dart – Types of Functions
- Dart – Anonymous Function
- Dart – main() Function
- Dart – Common Collection Methods
- Dart – exit() function
Object-Oriented Programming(OOPS) in Dart
OOPS is important part of Dart Programming Language let us learn topics from encapsulation to inheritance, polymorphism, abstract classes, and iterators, we’ll cover the essential concepts that empower you to build modular, reusable, and scalable code.
- Dart – Classes & Objects
- Dart – Constructors
- Dart – Super Constructor
- Dart – this Keyword
- Dart – static Keyword
- Dart – super Keyword
- Dart – Const And Final Keyword
- Dart – Inheritance
- Dart – Methods
- Dart – Method Overloading
- Dart – Getters & Setters
- Dart – Abstract Classes
- Dart – Builder Class
- Dart – Interfaces
- Dart – extends Vs with Vs implements
- Dart – Date and Time
- Dart – Type System
- Generators in Dart
- Dart – Finding Minimum and Maximum Value in a List
- Dart – Splitting of String
- How to Append or Concatenate Strings in Dart?
- How to Find the Length of a String in Dart?
- Dart – Sort a List
- How to convert a lowercase string to an uppercase string?
- How to convert all characters of a string to lowercase?
- How to Check String is Empty in Dart?
- Dart – Typedef
- Dart – URIs
- Dart – Collections
- Dart – Packages
- Dart – Generators
- Dart – Callable Classes
- Dart – Isolates
- Dart – Async
- Dart – String codeUnits Property
- Dart – HTML DOM
Interesting Reads
Rust vs Dart – Which is More Likely to Replace C++? Difference Between Golang and Dart Difference Between DART and C++ Comparison of Dart and JavaScript
Dart Flutter
Dart Language is primarily used for Android Development, iOS Development, and Web Development using the Flutter Framework . If you are interested in exploring more on Flutter, check out the below links:
- Basics of Flutter Framework
- Key Widgets in Flutter
- Pre-Built UI Components in Flutter
- Designs & Animations in Flutter
- Concept of Navigation & Routing in Flutter
- Forms & Gestures in Flutter
- Accessing the Hardware in Flutter
- Sample Flutter Applications
Practice Flutter Learing in Dartpad Flutter to Keep Up Your Good Work
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
The Dart 101
Difficulty : Easy
Community success rate: 53%
0" class="metrics-moderators"> Approved by Davidl.mkh Orleger nicola
A higher resolution is required to access the IDE
- = api.feedbackMetrics.averageRounded}" class="content-details-top-star-item">
- = api.feedbackMetrics.averageRounded}" class="content-details-top-star-item rate-off">

Learning Opportunities
This puzzle can be solved using the following concepts. Practice using these concepts and improve your skills.
Goal
The #1 tech hiring platform
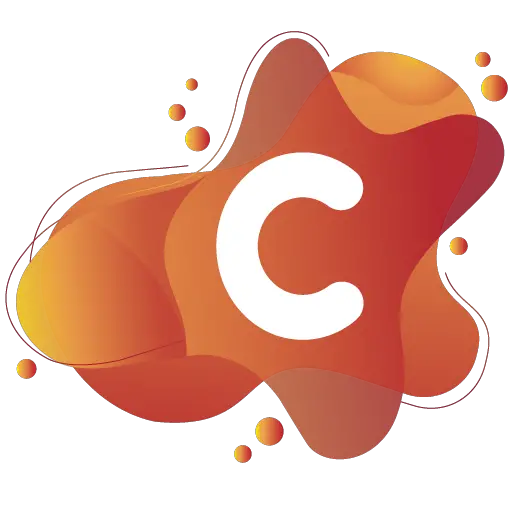
CodeVsColor
Dart tutorials and example programs.
- Dart 2 programming language Writing your first program (Introduction)
- Dart tutorial : How to use Boolean in Dart
- Compare two numbers using compareTo method in Dart
- Dart number datatype : integers and double
- Dart tutorial : string (explanation with examples)
- Convert all characters of a string to uppercase or lowercase in dart
- Dart 2 tutorial : Variables in dart with example
- How to convert a string to DateTime and DateTime to ISO8601 in Dart
- Dart comparable example for comparing objects
- How to split a string in Dart
- Dart example program to iterate through a list
- Dart list : Explanation with example
- Dart program to check if an integer is odd or even
- Dart replace all substring in a string example
- Dart padLeft and padRight examples
- Create a random integer, double or a boolean in dart using Random class
- Dart example program to replace a substring
- Dart program to check if a string ends or starts with a substring
- An introduction to dart string with examples
- Inbuilt methods of dart string
- Convert all characters in a string to uppercase and lowercase in dart
- Trim a string in Dart : Explanation with examples
- How to get the ASCII value of an character in dart
- For loop in dart : explanation with example
- How to install dart sdk on mac
- Different ways to find the sum of all dart list elements
- 7 different ways to find the largest, smallest number in dart list
- Constructors of a Dart list
- Dart set explanation with examples
- Introduction to Dart Map class
- Dart program to check if a character is uppercase
- How to print unicode of character in dart
- Runes in dart explanation with example
- Keywords in dart
- Dart function introduction with examples
- Different examples to create dart functions
- Dart program to find factorial of a number recursively
- Dart scope and lexical scoping
- Using optional parameters as required in dart
- How to use stagehand to create dart projects
- What is cascade notation or method chaining in Dart
- Conditional expression in Dart
- for-in loop in Dart with example
- Dart for loop with example
- if-else condition in Dart explanation with example
- Different ways to iterate through a Dart map
- Dart while loop explanation with example
- Dart optional positional, named and default parameters
- do…while loop in Dart with examples
- Dart switch case with example
- assert statement in Dart explanation with example
- Dart break statement explanation with example
- Dart continue statement explanation with example
- Exceptions and how to handle exceptions in Dart
- How to throw an exception in Dart
- How to print exception StackTrace in Dart
- Dart String replaceAll method
- Dart program to remove the last character of a string
- How to print the dollar sign in Dart
- Different Dart class constructors
- How Dart class constructors initialized in subclass/superclass
- Dart instance variable examples
- Instance method in Dart with examples
- Abstract method and abstract class in Dart
- Dart class inheritance : extends and override
- Implicit interface in Dart with examples
- Enumeration in Dart explanation with example
- Generics in Dart explanation with example
- What is mixins in Dart
- How to do runtime type checking in Dart
- Dart program to capitalize the first character of a string
- Dart string splitMapJoin examples
- Dart: math library Point class
- Dart: math library, its classes, constants and functions
- How to find random numbers in a range in Dart
- Asynchronous programming in Dart with examples
- Generators in dart with examples
- What is a callable class in Dart programming language
- Dart comments and types of comments supported in dart
- Typedef in dart with examples
- Dart metadata and how to create custom metadata
- Dart program to concat string with integer
- How to remove string character using index in Dart
- Dart check for any or every element in a list satisfy a given condition
- Dart program to check if a list contains a specific element or not
- How to shuffle a dart list
- Encoding/decoding JSON in Dart explanation with examples
- Dart program to check if a year is leap year or not
- How to find the ceil and floor values of a number in Dart
- Dart program to find the remainder using remainder method
- Dart program to find substring in a string
- How to use custom exceptions in dart
- How to convert string to integer in dart
- Dart program to convert a string to double
- How to find the length of a string in Dart
- Dart program to convert hexadecimal to integer
- How to reverse a list in dart
- Dart string contains method explanation with example
- Dart program to convert degree to radian and vice versa
- Dart program to change single or multiple list item
- Different ways to insert items to a list in dart
- Different ways to remove items from a dart list
- Dart program to swap two user given numbers
- Constants defined in dart-math library
- How to check if a string contains a number in Dart
- How to get the current date-time in Dart
- Dart program to find the absolute value of a number
- Dart program to round a number to its nearest value
- Dart program to find the hash value of a string
- How to multiply strings in Dart
- What is string interpolation in dart
- try-catch in dart explanation with example
- Dart map() function explanation with example
- Dart remove items from a list that doesn’t satisfy a condition
- Get the only single element that satisfy a condition in dart list
- Dart program to get the first n elements from a list
- How to create a string from ASCII values in Dart
- Dart StringBuffer class explanation with examples
- 5 ways in Dart to print the multiplication table
- Introduction to Queue in Dart and its methods
- How to add and remove items from a Queue in Dart
- How to remove and retain items from Queue in Dart with condition
- Dart Queue reduce function example
- Dart HashMap explanation with examples
- How to add and remove items of a HashMap in Dart
- How to iterate a HashMap in Dart in different ways
- HashSet in Dart and its methods and properties
- How to add and remove items from a HashSet in Dart
- Union and intersection of two HashSet in Dart
- Different ways to create a HashSet in Dart
- 3 ways to find if a HashSet is empty or not in Dart
- Dart HashSet.take and HashSet.takeWhile methods
- Dart HashSet where and whereType explanation with examples
- Dart HashSet fold and reduce methods explanation with examples
- Dart HashSet skip and skipWhile methods explanation with example
- Introduction to Dart LinkedHashMap class
- 7 ways to create a LinkedHashMap in Dart
- 3 ways to add items to a LinkedHashMap in Dart
- 3 ways to remove items to a LinkedHashMap in Dart
- Dart program to update single or multiple items of a LinkedHashMap
- 5 ways to check if Dart LinkedHashMap contains a key or value

Google uses cookies to deliver its services, to personalize ads, and to analyze traffic. You can adjust your privacy controls anytime in your Google settings . Learn more .
Dart 3.4 is live! Check out the blog post .
- List of Dart codelabs
- Language cheatsheet
- Iterable collections
- Asynchronous programming
- Introduction
- Libraries & imports
- Built-in types
- Collections
- Type system
- Overview & usage
- Pattern types
- Error handling
- Constructors
- Extend a class
- Extension methods
- Extension types
- Callable objects
- Class modifiers for API maintainers
- Asynchronous support
- Sound null safety
- Migrating to null safety
- Understanding null safety
- Unsound null safety
- dart:convert
- Using streams
- Creating streams
- Futures and error handling
- Documentation
- How to use packages
- Commonly used packages
- Creating packages
- Publishing packages
- Writing package pages
- Dependencies
- Package layout conventions
- Pub environment variables
- Pubspec file
- Troubleshooting pub
- Verified publishers
- Security advisories
- Futures, async, await
- Number representation
- Google APIs
- Multi-platform apps
- Get started
- Write command-line apps
- Fetch data from the internet
- Write HTTP servers
- Libraries & packages
- Google Cloud
- Wasm compilation
- Environment declarations
- Objective-C & Swift interop
- Java & Kotlin interop
- Past JS interop
- Web interop
- IntelliJ & Android Studio
- Dart DevTools
- Troubleshooting DartPad
- dart analyze
- dart compile
- dart create
- dart format
- dartaotruntime
- Experiment flags
- build_runner
- Customizing static analysis
- Fixing common type problems
- Fixing type promotion failures
- Linter rules
- Diagnostic messages
- Debugging web apps
- What not to commit
- Breaking changes
- Language evolution
- Language specification
- Dart 3 migration guide
- JavaScript to Dart
- Swift to Dart
- API reference
- DartPad (online editor)
- Package site
Apply fixes
Vs code support.
The dart fix command finds and fixes two types of issues:
Analysis issues identified by dart analyze that have associated automated fixes (sometimes called quick-fixes or code actions ).
Outdated API usages when updating to newer releases of the Dart and Flutter SDKs.
To preview proposed changes, use the --dry-run flag:
To apply the proposed changes, use the --apply flag:
Customize behavior
The dart fix command only applies fixes when there is a "problem" identified by a diagnostic. Some diagnostics, such as compilation errors, are implicitly enabled, while others, such as lints, must be explicitly enabled in the analysis options file , as individual preferences for these vary.
You can sometimes increase the number of fixes that can be applied by enabling additional lints. Note that not all diagnostics have associated fixes.
Imagine you have code like this:
Dart 2.17 introduced a new language feature called super initializers, which allows you to write the constructor of Vector3d with a more compact style:
To enable dart fix to upgrade existing code to use this feature, and to ensure that the analyzer warns you when you later forget to use it, configure your analysis_options.yaml file as follows:
We also need to make sure the code enables the required language version . Super initializers were introduced in Dart 2.17, so update pubspec.yaml to have at least that in the lower SDK constraint:
You should then see the following when viewing the proposed changes:
To learn more about customizing analysis results and behavior, see Customizing static analysis .
When you open a project in VS Code, the Dart plugin scans the project for issues that dart fix can repair. If it finds issues for repair, VS Code displays a prompt to remind you.
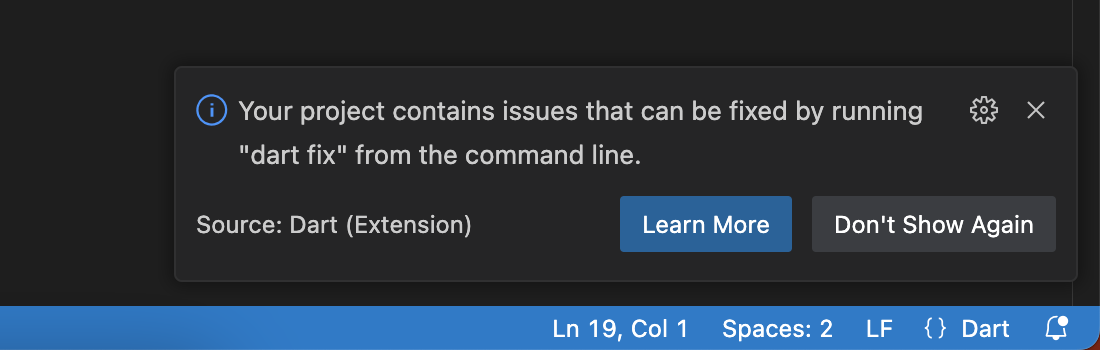
After running dart pub get or dart pub upgrade , VS Code might also display this prompt if package changes add issues that dart fix can repair.
Save all of your files before running dart fix . This ensures that Dart uses the latest versions of your files.

22 Dart Interview Questions (ANSWERED) Flutter Dev Must Know
Dart is an open source, purely object-oriented, optionally typed, and a class-based language which has excellent support for functional as well as reactive programming. Dart was the fastest-growing language between 2018 and 2019, with usage up a massive 532%. Follow along and check 22 common Dart Interview Questions Flutter and Mobile developers should be prepared for in 2020.
Q1 : What is Dart and why does Flutter use it?
Dart is an object-oriented , garbage-collected programming language that you use to develop Flutter apps. It was also created by Google, but is open-source, and has community inside and outside Google. Dart was chosen as the language of Flutter for the following reason:
- Dart is AOT (Ahead Of Time) compiled to fast, predictable, native code, which allows almost all of Flutter to be written in Dart. This not only makes Flutter fast, virtually everything (including all the widgets) can be customized.
- Dart can also be JIT (Just In Time) compiled for exceptionally fast development cycles and game-changing workflow (including Flutter’s popular sub-second stateful hot reload).
- Dart allows Flutter to avoid the need for a separate declarative layout language like JSX or XML , or separate visual interface builders, because Dart’s declarative, programmatic layout is easy to read and visualize. And with all the layout in one language and in one place, it is easy for Flutter to provide advanced tooling that makes layout a snap.
Q2 : What is Fat Arrow Notation in Dart and when do you use it?
The fat arrow syntax is simply a short hand for returning an expression and is similar to (){ return expression; } .
The fat arrow is for returning a single line, braces are for returning a code block.
Only an expression—not a statement—can appear between the arrow ( => ) and the semicolon ( ; ). For example, you can’t put an if statement there, but you can use a conditional expression
Q3 : Differentiate between named parameters and positional parameters in Dart?
Both named and positional parameters are part of optional parameter:
Optional Positional Parameters:
- A parameter wrapped by [ ] is a positional optional parameter. ```dart getHttpUrl(String server, String path, [int port=80]) { // ... } ```
- You can specify multiple positional parameters for a function: ```dart getHttpUrl(String server, String path, [int port=80, int numRetries=3]) { // ... } ``` In the above code, `port` and `numRetries` are optional and have default values of 80 and 3 respectively. You can call `getHttpUrl` with or without the third parameter. The optional parameters are _positional_ in that you can't omit `port` if you want to specify `numRetries`.
Optional Named Parameters:
- A parameter wrapped by { } is a named optional parameter. ```dart getHttpUrl(String server, String path, {int port = 80}) { // ... } ```
- You can specify multiple named parameters for a function: ```dart getHttpUrl(String server, String path, {int port = 80, int numRetries = 3}) { // ... } ``` You can call `getHttpUrl` with or without the third parameter. You **must** use the parameter name when calling the function.
- Because named parameters are referenced by name, they can be used in an order different from their declaration. ```dart getHttpUrl('example.com', '/index.html', numRetries: 5, port: 8080); getHttpUrl('example.com', '/index.html', numRetries: 5); ```
Q4 : Differentiate between required and optional parameters in Dart
Required Parameters
Dart required parameters are the arguments that are passed to a function and the function or method required all those parameters to complete its code block.
Optional Parameters
- Optional parameters are defined at the end of the parameter list, after any required parameters.
- In Flutter/Dart, there are 3 types of optional parameters: - Named - Parameters wrapped by { } - eg. getUrl(int color, [int favNum]) - Positional - Parameters wrapped by [ ] ) - eg. getUrl(int color, {int favNum}) - Default - Assigning a default value to a parameter. - eg. getUrl(int color, [int favNum = 6])
Q5 : Explain the different types of Streams ?
There are two kinds of streams. 1. Single subscription streams - The most common kind of stream. - It contains a sequence of events that are parts of a larger whole. Events need to be delivered in the correct order and without missing any of them. - This is the kind of stream you get when you read a file or receive a web request. - Such a stream can only be listened to once. Listening again later could mean missing out on initial events, and then the rest of the stream makes no sense. - When you start listening, the data will be fetched and provided in chunks.
- It intended for individual messages that can be handled one at a time. This kind of stream can be used for mouse events in a browser, for example.
- You can start listening to such a stream at any time, and you get the events that are fired while you listen.
- More than one listener can listen at the same time, and you can listen again later after canceling a previous subscription.
Q6 : How do you check if an async void method is completed in Dart?
Changing the return type to Future<void> .
Then you can do await save(...); or save().then(...);
Q7 : How is whenCompleted() different from then() in Future?
- .whenComplete will fire a function either when the Future completes with an error or not, while .then returns a new Future which is completed with the result of the call to onValue (if this future completes with a value) or to onError (if this future completes with an error)
- .whenCompleted is the asynchronous equivalent of a " finally " block.
Q8 : How to duplicate repeating items inside a Dart list?
Consider the code:
What can to be done in order to not repeat Ball() multiple times.
Using collection-for if we need different instances of Ball()
If we need the same instance of Ball() , List.filled should be used
Q9 : How to declare async function as a variable in Dart?
Async functions are normal functions with some sugar on top. The function variable type just needs to specify that it returns a Future:
Q10 : How to get difference of lists in Flutter/Dart?
Consider you have two lists [1,2,3,4,5,6,7] and [3,5,6,7,9,10] . How would you get the difference as output? eg. [1, 2, 4]
You can do something like this:
alternative answer:
note that for both cases, you need to loop over the larger list .
Q11 : What are Streams in Flutter/Dart?
- Asynchronous programming in Dart is characterized by the Future and Stream classes.
- A stream is a sequence of asynchronous events. It is like an asynchronous Iterable —where, instead of getting the next event when you ask for it, the stream tells you that there is an event when it is ready.
- Streams can be created in many ways but they all are used in the same way; the asynchronous for loop ( await for ). E.g
- Streams provide an asynchronous sequence of data.
- Data sequences include user-generated events and data read from files.
- You can process a stream using either await for or listen() from the Stream API .
- Streams provide a way to respond to errors.
- There are two kinds of streams: single subscription or broadcast .
Q12 : What are Null-aware operators?
- Dart offers some handy operators for dealing with values that might be null.
- One is the ??= assignment operator, which assigns a value to a variable only if that variable is currently null:
- Another null-aware operator is ??, which returns the expression on its left unless that expression’s value is null, in which case it evaluates and returns the expression on its right:
Q13 : Explain async , await in Flutter/Dart?
Asynchronous operations let your program complete work while waiting for another operation to finish. Here are some common asynchronous operations:
- Fetching data over a network.
- Writing to a database.
- Reading data from a file.
To perform asynchronous operations in Dart, you can use the Future class and the async and await keywords.
The async and await keywords provide a declarative way to define asynchronous functions and use their results. Remember these two basic guidelines when using async and await :
- To define an async function, add async before the function body
- The await keyword works only in async functions.
An async function runs synchronously until the first await keyword. This means that within an async function body, all synchronous code before the first await keyword executes immediately.
Consider an example:
Q14 : How do you convert a List into a Map in Dart?
You can use Map.fromIterable :
or collection-for (starting from Dart 2.3):
Q15 : How does Dart AOT work?
- Dart source code will be translated into assembly files, then assembly files will be compiled into binary code for different architectures by the assembler.
- For mobile applications the source code is compiled for multiple processors ARM, ARM64, x64 and for both platforms - Android and iOS. This means there are multiple resulting binary files for each supported processor and platform combination.
Q16 : How to compare two dates that are constructed differently in Dart?
You can do that by converting the other date into utc and then comparing them with isAtSameMomentAs method.
Q17 : What are the similarities and differences of Future and Stream ?
Similarity:
- Future and Stream both work asynchronously.
- Both have some potential value.
Differences:
- A Stream is a combination of Futures .
- Future has only one response but Stream could have any number of Response .
Q18 : What does non-nullable by default mean in Dart?
- Non-nullable by default means that any variable that is declared normally cannot be null .
- Any operation accessing the variable before it has been assigned is illegal .
- Additionally, assigning null to a non-nullable variable is also not allowed.
Q19 : What does a class with a method named ._() mean in Dart/Flutter?
- In Dart, if the leading character is an underscore, then the function/constructor is private to the library.
- Class._(); is a named constructor (another example might be the copy constructor on some object in Flutter : AnotherClass.copy(...); ).
- The Class._(); isn't necessary unless you don't want Class to ever be accidentally instantiated using the implicit default constructor.
Q20 : What is Future in Flutter/Dart?
- A Future is used to represent a potential value, or error, that will be available at some time in the future. Receivers of a Future can register callbacks that handle the value or error once it is available. For example:
- If a future doesn’t produce a usable value, then the future’s type is Future<void> .
A future represents the result of an asynchronous operation, and can have two states:
Uncompleted When you call an asynchronous function, it returns an uncompleted future. That future is waiting for the function’s asynchronous operation to finish or to throw an error.
Completed If the asynchronous operation succeeds, the future completes with a value. Otherwise it completes with an error.
Q21 : What is a difference between these operators ?? and ?.
- It is a null-aware operator which returns the expression on its left unless that expression’s value is null, in which case it evaluates and returns the expression on its right:
- It is a conditional property access which is used to guard access to a property or method of an object that might be null, put a question mark (?) before the dot (.):
- You can chain multiple uses of ?. together in a single expression:
The preceding code returns null (and never calls someMethod() ) if either myObject or myObject.someProperty is null.
Q22 : What's the difference between async and async* in Dart?
- async gives you a Future while async* gives you a Stream
- You add the async keyword to a function that does some work that might take a long time. It returns the result wrapped in a Future .
- You add the async* keyword to make a function that returns a bunch of future values one at a time. The results are wrapped in a Stream.
- async* will always returns a Stream and offer some syntax sugar to emit a value through yield keyword.
Rust has been Stack Overflow’s most loved language for four years in a row and emerged as a compelling language choice for both backend and system developers, offering a unique combination of memory safety, performance, concurrency without Data races...
Clean Architecture provides a clear and modular structure for building software systems, separating business rules from implementation details. It promotes maintainability by allowing for easier updates and changes to specific components without affe...
Azure Service Bus is a crucial component for Azure cloud developers as it provides reliable and scalable messaging capabilities. It enables decoupled communication between different components of a distributed system, promoting flexibility and resili...
FullStack.Cafe is a biggest hand-picked collection of top Full-Stack, Coding, Data Structures & System Design Interview Questions to land 6-figure job offer in no time.
Coded with 🧡 using React in Australia 🇦🇺
by @aershov24 , Full Stack Cafe Pty Ltd 🤙, 2018-2023
Privacy • Terms of Service • Guest Posts • Contacts • MLStack.Cafe

OOP in Dart
Oop in dart.
Object-oriented programming (OOP) is a programming method that uses objects and their interactions to design and program applications. It is one of the most popular programming paradigms and is used in many programming languages, such as Dart, Java, C++, Python, etc.

In OOP , an object can be anything, such as a person, a bank account, a car, or a house. Each object has its attributes (or properties) and behavior (or methods). For example, a person object may have the attributes name , age and height , and the behavior walk and talk .
- It is easy to understand and use.
- It increases reusability and decreases complexity.
- The productivity of programmers increases.
- It makes the code easier to maintain, modify and debug.
- It promotes teamwork and collaboration.
- It reduces the repetition of code.
Features Of OOP
- Encapsulation
- Inheritance
- Polymorphism
- Abstraction
Note: The main purpose of OOP is to break complex problems into smaller objects. You will learn all these OOPs features later in this dart tutorial.
- Object Oriented Programming (OOP) is a programming paradigm that uses objects and their interactions to design and program applications.
- OOP is based on objects, which are data structures containing data and methods.
- OOP is a way of thinking about programming that differs from traditional procedural programming.
- OOP can make code more modular, flexible, and extensible.
- OOP can help you to understand better and solve problems.
Home » Dart Tutorial » Dart if
Summary : in this tutorial, you’ll learn how to use the Dart if statement to execute a code block based on a condition.
Introduction to the Dart if statement
An if statement allows you to do something only if a condition is true . Here’s the syntax of the if statement:
In this syntax, if the condition is true , the if statement executes the statement . But if the condition is false , the if statement won’t execute the statement .
If you want to execute multiple statements, you need to place them in curly braces like this:
It’s a good practice to use a block with the if statement even though it has a single statement.
The following flowchart illustrates how the Dart if statement works:
Dart if statement examples
The following examples demonstrate how the if statement works.
1) A simple Dart if statement example
The following example uses the if statement to show a message when the isWeekend is true:
How it works.
- First, declare a boolean variable isWeekend with the value true .
- Second, display the message "Let's play!" if the isWeekend is true. Since the isWeekend is true, the program shows the message.
2) Dart if statement with a condition evaluates to false
The following program doesn’t display anything because the isWeekend is false. Therefore, the if statement doesn’t execute the print statement between the curly braces:
3) Dart if statement example with a complex condition
In practice, the condition of the if statement this more complex, which consists of multiple expressions like this:
Nested Dart if statement
Dart allows you to nest if statements inside an if statement. Here is an example:
- First, declare the isWeekend and weather variables and initialize their values to true and "rainy" .
- Second, check if the isWeekend is true in the if statement. Because the isWeeknd is true , the if statement executes the statement inside its block.
- Third, check if the weather is "sunny" in the first nested if statement. Since the weather is "rainy" , the first nested if statement does do anything.
- Finally, check if the weather is "rainy" in the second nested if statment. Because the weather is "rainy" , the if statement executes its body that displays the message "Let's play computer game at home!" .
Even though you can nest if statements, you should always avoid doing this. Because nested if statements make the code difficult to read and maintain. It’s a good practice to use flat if statements. For example, you can flatten the example above using two if statements like this:
- Use the if statement to execute one or more statements when a condition is true .
- Avoid using nested if statements to make the code easier to read.
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
A set of dart problem solving exercises.
a-hamouda/dart_problem_solving
Folders and files, repository files navigation, exercises set 1:.
Refer all_tests.dart to run all tests for the exercises
1- Create a program that asks the user to enter their name and their age. Print out a message that tells how many years they have to be 100 years old.
2- Ask the user for a number. Depending on whether the number is even or odd, print out an
appropriate message to the user.
3- Take a list, say for example this one:
a = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89]
and write a program that prints out all the elements of the list that are less than 5.
4- Create a program that asks the user for a number and then prints out a list of all the divisors of that number.
If you don’t know what a divisor is, it is a number that divides evenly into another number. For example, 13 is a divisor of 26 because 26 / 13 has no remainder.
5- Take two lists, for example:
a = [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89] b = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11,
and write a program that returns a list that contains only the elements that are common between them (without duplicates). Make sure your program works on two lists of different sizes.
6- Ask the user for a string and print out whether this string is a palindrome or not.
A palindrome is a string that reads the same forwards and backwards.
Like “Mom , Wow , Dad , smms ,mokom” 7- et’s say you are given a list saved in a variable:
a = [1, 4, 9, 16, 25, 36, 49, 64, 81, 100].
Write a Dart code that takes this list and makes a new list that has only the even elements of this list in it.
8- Make a two-player Rock-Paper-Scissors game against computer.
Ask for player’s input, compare them, print out a message to the winner. 9- Generate a random number between 1 and 100. Ask the user to guess the number, then tell them whether they guessed too low, too high, or exactly right.
Keep track of how many guesses the user has taken, and when the game ends, print this out.
10- Ask the user for a number and determine whether the number is prime or not.
Do it using a function
11- Write a program that takes a list of numbers for example
a = [5, 10, 15, 20, 25]
and makes a new list of only the first and last elements of the given list. For practice, write this code inside a function.
12- Write a program (function) that takes a list and returns a new list that contains all the elements of the first list minus all the duplicates.
13- Write a program (using functions!) that asks the user for a long string containing multiple words. Print back to the user the same string, except with the words in backwards order.
For example, say I type the string:
My name is Michele
Then I would see the string:
Michele is name My
14- Write a password generator in Dart. Be creative with how you generate passwords - strong passwords have a mix of lowercase letters, uppercase letters, numbers, and symbols. The passwords should be random, generating a new password every time the user asks for a new password. Include your run-time code in a main method.
15- Create a program that will play the “cows and bulls” game with the user. The game works like this:
• Randomly generate a 4-digit number. Ask the user to guess a 4digit number. For every digit the user guessed correctly in the correct place, they have a “cow”. For every digit the user guessed correctly in the wrong place is a “bull.”
• Every time the user makes a guess, tell them how many “cows” and “bulls” they have. Once the user guesses the correct number, the game is over. Keep track of the number of guesses the user makes throughout the game and tell the user at the end. 16- Little John wants to calculate and show the amount of spent fuel liters on a trip, using a car that does 12 Km/L. For this, he would like you to help him through a simple program. To perform the calculation, you have to read spent time (in hours) and the same average speed (km/h). In this way, you can get distance and then, calculate how many liters would be needed. Show the value with three decimal places after the point.

18- Make a program that reads five integer values. Count how many of these values are even and print this information
19- Make a program that Read the start time and end time of a game in hours , and tell us how many hours the game continued but the game will start in a day and end in the next day
20- Read an integer number between 1 and 12, including. Corresponding to this number, you must print the month of the year, in english, with the first letter in uppercase.
21- When we think of a mobile phone we have three types Samsung , iphone , nokia All of this have some shared attributes like 1- model , name , screen resolution, operating system And another attributes which is not common Ex : FaceTime on iphone Please think and build this scenario depends on your knowledge of oop.
- Dart 100.0%
Armstrong Numbers
The home of mathematics education in New Zealand.
- Forgot password ?
- Resource Finder
The Ministry is migrating nzmaths content to Tāhurangi. Relevant and up-to-date teaching resources are being moved to Tāhūrangi (tahurangi.education.govt.nz). When all identified resources have been successfully moved, this website will close. We expect this to be in June 2024. e-ako maths, e-ako Pāngarau, and e-ako PLD 360 will continue to be available.
For more information visit https://tahurangi.education.govt.nz/updates-to-nzmaths
This problem solving activity has a number focus.
- What scores can Maddie get?
- Maddie decides to change the rules. She can now add and subtract the 3s and 7s. What possible positive scores can she get?
- What whole positive numbers can Maddie get if she uses any of the four operations of addition, subtraction, multiplication and division?
- Apply the number operations to single digit numbers.
- Use a problem solving strategy to identify all the possible outcomes.
This problem gives students the opportunity to be systematic in the way they combine numbers, and to be alert to number patterns.
Note that this is not a probability problem. The challenge is to see what scores can be made.
- Copymaster of the problem (English)
- Copymaster of the problem (Māori)
- A dartboard or a picture of a dart board to introduce the problem
The Problem
Maddie has a dart board on which the outer ring scores 3 points and the inner ring scores 7 points. Maddie has three darts.
Teaching Sequence
- Introduce the problem with a discussion about the game of darts. Using the picture ask the students to share their understanding of the game.
- Display or draw the game board that is used in the problem.
- Ask the students to identify the highest score that can be obtained from throwing three darts.
- In pairs, ask the students to find all the possible scores obtained from throwing three darts. Encourage them to be systematic in recording what they find out.
- Share the possible scores.
- Pose parts (b) and then (c) of the problem for the students to work on. Check that they understand what is required.
- As they work, ask questions that focus students on their manipulation of numbers using the number operations. Encourage them to justify their solutions. How did you begin part (b)? Why did you start there? How did you work out the scores?(Elaboration of mental strategies) Do you think that you have found all the possible scores? How do you know?
- As the students work, encourage them to record their solutions so that they can be shared with others in the class.
- Share solutions.
Students could be given more darts; there might be different points for the two rings of the board; more rings could be used.
They might also be asked how many ways there are of getting each number, or of getting negative whole numbers.
Maddie can get three 3s; two 3s and a 7; one 3 and two 7s; or three 7s.
- Maddie can only get 9, 13, 17 or 21.
- Maddie can now get 1 = 7 – 3 – 3; 3 = 3 + 3 – 3; 7 = 7 + 3 – 3; 11 = 7 + 7 – 3; in addition to the scores in (a). (It’s interesting to note here that it is not possible to get an even number using three odd numbers. Is it clear why?)
- And finally, Maddie can, as well as the addition and subtraction combination, get:
Note the systematic approach to finding these solutions.
Log in or register to create plans from your planning space that include this resource.
- Printer-friendly version
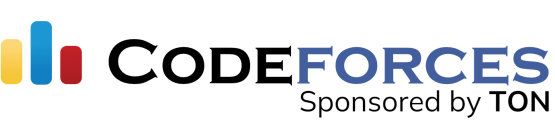
Round 948 Div 2 Solution Discussion (with Jan)

- Wael.Al.Jamal
- Submissions
Wael.Al.Jamal's blog
DART Language in codeforces
Hi codeforces,
I am asking about adding Dart programming language,
what is your opinion, I am interested.
have a good day


IMAGES
VIDEO
COMMENTS
The best part, it's 100% free for everyone. Develop fluency in 70 programming languages with our unique blend of learning, practice and mentoring. Exercism is fun, effective and 100% free, forever. Get fluent in Dart by solving 63 exercises. And then level up with mentoring from our world-class team.
Algorithms. 8 kyu. Invert values. 148,827 user7657844 1 Issue Reported. Lists. Fundamentals. Arrays. Practice Dart coding with code challenges designed to engage your programming skills. Solve coding problems and pick up new techniques from your fellow peers.
Basic Dart Practice Questions. Write a program to print your name in Dart. Write a program to print Hello I am "John Doe" and Hello I'am "John Doe" with single and double quotes. Declare constant type of int set value 7. Write a program in Dart that finds simple interest. Formula= (p * t * r) / 100.
Dart is a modern, open-source programming language developed by Google. It is used for various applications, including web development, mobile app development, and server-side programming. Dart is known for its performance, productivity, and ease of use. These key competencies in Dart lay the foundation for becoming proficient in the language ...
Dart is an open-source, object-oriented programming language developed by Google. Dart is designed to be fast, flexible, and easy to learn, with a syntax tha...
Learn and practice Dart by completing 63 exercises that explore different concepts and ideas. Join The Dart Track Explore the Dart exercises on Exercism. Unlock more exercises as you progress. They're great practice and fun to do! Code practice and mentorship for everyone.
Practice Dart - exercises for beginners. Hello everyone. When I was learning Python, I found Practice Python to be very useful. There, author gives a set of 36 exercises, from very simple to more complex, that teaches the basics of the language. Even to this day, I go through the exercises occasionally, to brush up the fundamentals or to come ...
Web Games with Dart and the HTML5 Canvas: Snake. With many of the advanced organizational structures of heavy hitters like C++ and Java, but featuring simpler semantics and a lightweight syntax for common web idioms, Dart is a great language for building web games. Are you ready to learn how to write games.
The basics. The following tours assume a basic familiarity with the Dart language, which you can get from skimming the language tour. Next, learn about futures by following the asynchronous programming codelab. Once you're familiar with the language and futures, learn about streams and packages, which are fundamental to most Dart programs.
Dart is an open-source general-purpose programming language developed by Google. It supports application development on both the client and server side. However, it is widely used for the development of Android apps, iOS apps, IoT (Internet of Things), and web applications using the Flutter Framework. Syntactically, Dart bears a strong ...
Welcome to another captivating episode of vanAmsen's coding vlog! Today, we dive headfirst into solving the renowned 'Two Sum' problem using Dart. If you're ...
The player who has reached 101 in the fewest rounds wins the game. You have to print the winner's name. Example of calculation : 10 5 3*18 20 X 2*14 17 4. In the round 1, the player scores 10 + 5 + 3*18 = 10 + 5 + 54 = 69 points. In the round 2, the player scores 20 - 20 + 28 = 28 points. The total is 69 + 28 = 97 points.
Dart example program to iterate through a list. Dart list : Explanation with example. Dart program to check if an integer is odd or even. Dart replace all substring in a string example. Dart padLeft and padRight examples. Create a random integer, double or a boolean in dart using Random class.
The dart fix command only applies fixes when there is a "problem" identified by a diagnostic. Some diagnostics, such as compilation errors, are implicitly enabled, while others, such as lints, must be explicitly enabled in the analysis options file, as individual preferences for these vary.. You can sometimes increase the number of fixes that can be applied by enabling additional lints.
Dart is an open source, purely object-oriented, optionally typed, and a class-based language which has excellent support for functional as well as reactive programming. Dart was the fastest-growing language between 2018 and 2019, with usage up a massive 532%. Follow along and check 22 common Dart Interview Questions Flutter and Mobile developers should be prepared for in 2020.
OOP in Dart Create Free Backend With Appwrite OOP In Dart. Object-oriented programming (OOP) is a programming method that uses objects and their interactions to design and program applications. It is one of the most popular programming paradigms and is used in many programming languages, such as Dart, Java, C++, Python, etc.
); } } Code language: Dart (dart) Output: Let 's play! Code language: Dart (dart) How it works. First, declare a boolean variable isWeekend with the value true. Second, display the message "Let's play!" if the isWeekend is true. Since the isWeekend is true, the program shows the message. 2) Dart if statement with a condition evaluates to false
Exercises Set 1: Refer all_tests.dart to run all tests for the exercises. 1- Create a program that asks the user to enter their name and their age. Print out a message that tells how many years they have to be 100 years old. 2- Ask the user for a number. Depending on whether the number is even or odd, print out an.
Explore other people's solutions to Armstrong Numbers in Dart, and learn how others have solved the exercise. Learn. Language Tracks. Upskill in 65+ languages #48in24 Challenge. A different challenge each week in 2024. Your Journey. Explore your Exercism journey. Discover. Exercism Perks.
Need a problem solving website for practising dart. Dart Hi, everyone. I've started learning flutter for past 6 months in parallel with my computer science course. I'm still lacking a lot in Dart programming language. That's why I wanted to practice dart independent of flutter. I've looked for some popular sites like leetcode, hackerrank, etc.
This problem solving activity has a number focus. Achievement Objectives. NA3-1: Use a range of additive and simple multiplicative strategies with whole numbers, fractions, decimals, and percentages. ... The Problem. Maddie has a dart board on which the outer ring scores 3 points and the inner ring scores 7 points. Maddie has three darts.
Dart, though being a general-purpose programming language, is largely geared towards building apps and therefore has a relatively small standard library for doing math, vectors, sorting, and so on. Standard input/output is also slower; it does have streams to more efficiently read I/O, but there's no good built-in way to achieve this and ...