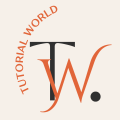

List of All Spring Boot Annotations, Uses with examples
- Post author: Tutorial World
- Post published:
- Post category: Spring Boot
Table of Contents
Commonly used Spring Boot annotations along with their uses and examples
1). @SpringBootApplication: This annotation is used to bootstrap a Spring Boot application. It combines three annotations: @Configuration , @EnableAutoConfiguration , and @ComponentScan .
2). @RestController: This annotation is used to indicate that a class is a RESTful controller. It combines @Controller and @ResponseBody .
3). @RequestMapping: This annotation is used to map web requests to specific handler methods. It can be applied at the class or method level.
4). @Autowired: This annotation is used to automatically wire dependencies in Spring beans. It can be applied to fields, constructors, or methods.
5). @Component: This annotation is used to indicate that a class is a Spring bean. Example:
6). @Service: This annotation is used to indicate that a class is a specialized type of Spring bean, typically used for business logic.
7). @Repository: This annotation is used to indicate that a class is a specialized type of Spring bean, typically used for database access.
8). @Configuration: This annotation is used to declare a class as a configuration class. It is typically used in combination with @Bean methods.
9). @Value: This annotation is used to inject values from properties files or other sources into Spring beans.
10). @EnableAutoConfiguration: This annotation is used to enable Spring Boot’s auto-configuration mechanism. It automatically configures the application based on the classpath dependencies and properties.
11). @GetMapping, @PostMapping, @PutMapping, @DeleteMapping : These annotations are used to map specific HTTP methods to handler methods. They are shortcuts for <strong>@RequestMapping</strong> with the respective HTTP method.
12). @PathVariable: This annotation is used to bind a method parameter to a path variable in a request URL.
13). @RequestParam: This annotation is used to bind a method parameter to a request parameter.
14). @RequestBody: This annotation is used to bind the request body to a method parameter. It is commonly used in RESTful APIs to receive JSON or XML payloads. Example:
15). @Qualifier: This annotation is used to specify which bean to inject when multiple beans of the same type are available.
16). @ConditionalOnProperty: This annotation is used to conditionally enable or disable a bean or configuration based on the value of a property.
17). @Scheduled: This annotation is used to schedule the execution of a method at fixed intervals.
18). @Cacheable, @CachePut, @CacheEvict: These annotations are used for caching method results. They allow you to cache the return value of a method, update the cache, or evict the cache, respectively.
Here’s an extensive list of Spring Boot annotations
1. core annotations:, a). @springbootapplication.
The @SpringBootApplication annotation is used to mark the main class of a Spring Boot application. This is the Spring Boot Application starting point. It combines three annotations: @Configuration , @EnableAutoConfiguration , and @ComponentScan . This annotation enables auto-configuration, component scanning, and configuration capabilities for the application.
b). @ComponentScan
The @ComponentScan annotation is used to specify the base package(s) to scan for Spring components such as controllers, services, repositories, etc.
c). @Configuration
The @Configuration annotation is used to indicate that a class declares one or more bean definitions. It is typically used in combination with @Bean to define Spring configuration classes. In the example, the DatabaseConfig class is marked as a configuration class, and the dataSource() method is annotated with @Bean to define a bean of type DataSource .
d). @EnableAutoConfiguration
The @EnableAutoConfiguration annotation allows Spring Boot to automatically configure the application based on the dependencies present in the classpath. It helps to reduce manual configuration by inferring configuration based on conventions and default settings.
e). @RestController
The @RestController annotation is used to mark a class as a controller in a Spring MVC or Spring WebFlux application. It combines the @Controller and @ResponseBody annotations. In the example, the UserController class is a REST controller that handles HTTP GET requests for the “/users” endpoint.
f). @Controller
The @Controller annotation is used to mark a class as a controller in a Spring MVC or Spring WebFlux application. It handles HTTP requests and returns the view name or a response body. In the example, the HomeController class is a controller that handles requests for the root (“/”) URL and returns the view name “index”.
g). @Service
The @Service annotation is used to mark a class as a service component in the business logic layer. It is used to encapsulate business logic and perform operations such as data retrieval, manipulation, and validation. In the example, the UserService class is a service component that provides a method to retrieve users.
h). @Repository
The @Repository annotation is used to mark a class as a repository component in the data access layer. It is responsible for data access operations such as querying, saving, updating, and deleting data from a database. In the example, the UserRepository class is a repository component that provides a method to retrieve users from the database.
The @Bean annotation is used to declare a method as a bean producer method within a configuration class. It indicates that the method returns an object that should be managed by the Spring container as a bean. In the example, the userService() method is annotated with @Bean to define a bean of type UserService .
j). @Autowired
The @Autowired annotation is used to inject dependencies automatically by type. It can be applied to constructors, fields, and methods. In the example, the UserRepository dependency is autowired into the UserService class constructor.
k). @Qualifier
The @Qualifier annotation is used to resolve ambiguous dependencies when there are multiple beans of the same type. It can be applied along with @Autowired to specify the exact bean to be injected. In the example, the userRepository bean is qualified using its bean name.
The @Value annotation is used to inject values from external sources, such as properties files, into variables. In the example, the appName the variable is injected with the value of the “app.name” property from an external configuration source.
2. Web Annotations :
@RequestMapping
@GetMapping
@PostMapping
@PutMapping
@DeleteMapping
@PatchMapping
@RequestBody
@ResponseBody
@PathVariable
@RequestParam
@RequestHeader
@CookieValue
@ModelAttribute
@ResponseStatus
@ExceptionHandler
3. Data Annotations :
@GeneratedValue
@Repository
@Transactional
@PersistenceContext
@NamedQuery
@JoinColumn
4. Validation Annotations :
5. security annotations :.
@EnableWebSecurity
@Configuration
@EnableGlobalMethodSecurity
@PreAuthorize
@PostAuthorize
@RolesAllowed
@EnableOAuth2Client
@EnableResourceServer
@EnableAuthorizationServer
6. Testing Annotations :
@SpringBootTest
@WebMvcTest
@DataJpaTest
@RestClientTest
@AutoConfigureMockMvc
@BeforeEach
@DisplayName
@ParameterizedTest
@ValueSource
@ExtendWith
7. Caching Annotations :
@EnableCaching
@CacheEvict
8. Scheduling Annotations :
@EnableScheduling
9. Messaging Annotations :
@JmsListener
@MessageMapping
10. Aspect-Oriented Programming (AOP) Annotations :
@AfterReturning
@AfterThrowing
11. Actuator Annotations :
@EnableActuator
@RestControllerEndpoint
@ReadOperation
@WriteOperation
@DeleteOperation
12. Configuration Properties Annotations :
@ConfigurationProperties
@ConstructorBinding
13. Internationalization and Localization :
@EnableMessageSource
@EnableWebMvc
@LocaleResolver
@MessageBundle
@MessageSource
14. Logging and Monitoring :
@ExceptionMetered
15. Data Validation :
@PositiveOrZero
@NegativeOrZero
16. GraphQL Annotations :
@GraphQLApi
@GraphQLQuery
@GraphQLMutation
@GraphQLSubscription
@GraphQLArgument
@GraphQLContext
@GraphQLNonNull
@GraphQLInputType
@GraphQLType
17. Integration Annotations :
@IntegrationComponentScan
@MessagingGateway
@Transformer
@Aggregator
@ServiceActivator
@InboundChannelAdapter
@OutboundChannelAdapter
18. Flyway Database Migrations :
@FlywayTest
@FlywayTestExtension
@FlywayTestExtension.Test
@FlywayTestExtension.BeforeMigration
@FlywayTestExtension.AfterMigration
19. JUnit 5 Annotations :
@TestInstance
@TestTemplate
@DisplayNameGeneration
@DisabledOnOs
@EnabledOnOs
@DisabledIf
20. API Documentation Annotations :
@Api: This annotation is used to provide high-level information about the API.
@ApiOperation: This annotation is used to describe an operation or endpoint in the API.
@ApiParam: This annotation is used to describe a parameter in an API operation.
@ApiModel: This annotation is used to describe a data model used in the API.
@ApiModelProperty: This annotation is used to describe a property of a data model.
21. Exception Handling Annotations :
@ControllerAdvice: This annotation is used to define global exception handling for controllers.
@ExceptionHandler: This annotation is used to define a method to handle specific exceptions.
22. GraphQL Annotations :
@GraphQLSchema: This annotation is used to define the GraphQL schema for a Spring Boot application.
@GraphQLQueryResolver: This annotation is used to define a class as a GraphQL query resolver.
@GraphQLMutationResolver: This annotation is used to define a class as a GraphQL mutation resolver.
@GraphQLSubscriptionResolver: This annotation is used to define a class as a GraphQL subscription resolver.
@GraphQLResolver: This annotation is used to define a class as a generic resolver for GraphQL.
23. Server-Sent Events (SSE) Annotations :
@SseEmitter: This annotation is used to create an SSE endpoint for server-sent events.
@SseEventSink: This annotation is used to inject an SSE event sink into a method parameter.
24. WebFlux Annotations :
@RestController: This annotation is used to create a RESTful controller in a WebFlux application.
@GetMapping, @PostMapping, @PutMapping, @DeleteMapping, @PatchMapping: These annotations are used to map HTTP methods to handler methods in a WebFlux application.
25. Micrometer Metrics Annotations :
@Timed: This annotation is used to measure the execution time of a method.
@Counted: This annotation is used to count the number of times a method is invoked.
@Gauge: This annotation is used to expose a method as a gauge metric.
@ExceptionMetered: This annotation is used to measure the rate of exceptions thrown by a method.
Please note that this is not an exhaustive list, and Spring Boot offers many more annotations across various modules and features. For a comprehensive list of annotations and their usage, I recommend referring to the official Spring Boot documentation and module-specific documentation.
You Might Also Like
5 ways for spring configuration in spring boot application, spring beans and bean scopes explanation, explain spring boot data for working with databases.
Spring Framework Guru
Spring framework annotations.
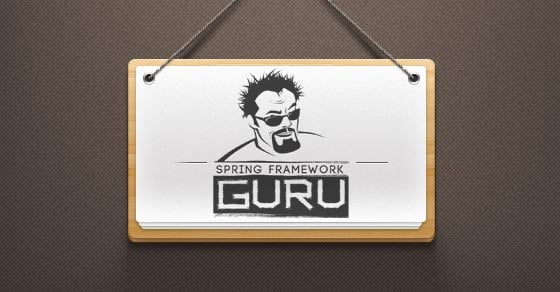
The Java Programming language provided support for Annotations from Java 5.0. Leading Java frameworks were quick to adopt annotations and the Spring Framework started using annotations from the release 2.5. Due to the way they are defined, annotations provide a lot of context in their declaration.
Prior to annotations, the behavior of the Spring Framework was largely controlled through XML configuration. Today, the use of annotations provide us tremendous capabilities in how we configure the behaviors of the Spring Framework.
In this post, we’ll take a look at the annotations available in the Spring Framework.
Core Spring Framework Annotations
This annotation is applied on bean setter methods. Consider a scenario where you need to enforce a required property. The @Required annotation indicates that the affected bean must be populated at configuration time with the required property. Otherwise an exception of type BeanInitializationException is thrown.
This annotation is applied on fields, setter methods, and constructors. The @Autowired annotation injects object dependency implicitly.
When you use @Autowired on fields and pass the values for the fields using the property name, Spring will automatically assign the fields with the passed values.
You can even use @Autowired on private properties, as shown below. (This is a very poor practice though!)
When you use @Autowired on setter methods, Spring tries to perform the by Type autowiring on the method. You are instructing Spring that it should initiate this property using setter method where you can add your custom code, like initializing any other property with this property.
Consider a scenario where you need instance of class A , but you do not store A in the field of the class. You just use A to obtain instance of B , and you are storing B in this field. In this case setter method autowiring will better suite you. You will not have class level unused fields.
When you use @Autowired on a constructor, constructor injection happens at the time of object creation. It indicates the constructor to autowire when used as a bean. One thing to note here is that only one constructor of any bean class can carry the @Autowired annotation.
NOTE: As of Spring 4.3, @Autowired became optional on classes with a single constructor. In the above example, Spring would still inject an instance of the Person class if you omitted the @Autowired annotation.
This annotation is used along with @Autowired annotation. When you need more control of the dependency injection process, @Qualifier can be used. @Qualifier can be specified on individual constructor arguments or method parameters. This annotation is used to avoid confusion which occurs when you create more than one bean of the same type and want to wire only one of them with a property.
Consider an example where an interface BeanInterface is implemented by two beans BeanB1 and BeanB2 .
Now if BeanA autowires this interface, Spring will not know which one of the two implementations to inject. One solution to this problem is the use of the @Qualifier annotation.
With the @Qualifier annotation added, Spring will now know which bean to autowire where beanB2 is the name of BeanB2 .
- @Configuration
This annotation is used on classes which define beans. @Configuration is an analog for XML configuration file – it is configuration using Java class. Java class annotated with @Configuration is a configuration by itself and will have methods to instantiate and configure the dependencies.
Here is an example:
- @ComponentScan
This annotation is used with @Configuration annotation to allow Spring to know the packages to scan for annotated components. @ComponentScan is also used to specify base packages using basePackageClasses or basePackage attributes to scan. If specific packages are not defined, scanning will occur from the package of the class that declares this annotation.
Checkout this post for an in depth look at the Component Scan annotation.
This annotation is used at the method level. @Bean annotation works with @Configuration to create Spring beans. As mentioned earlier, @Configuration will have methods to instantiate and configure dependencies. Such methods will be annotated with @Bean . The method annotated with this annotation works as bean ID and it creates and returns the actual bean.
This annotation is used on component classes. By default all autowired dependencies are created and configured at startup. But if you want to initialize a bean lazily, you can use @Lazy annotation over the class. This means that the bean will be created and initialized only when it is first requested for. You can also use this annotation on @Configuration classes. This indicates that all @Bean methods within that @Configuration should be lazily initialized.
This annotation is used at the field, constructor parameter, and method parameter level. The @Value annotation indicates a default value expression for the field or parameter to initialize the property with. As the @Autowired annotation tells Spring to inject object into another when it loads your application context, you can also use @Value annotation to inject values from a property file into a bean’s attribute. It supports both #{...} and ${...} placeholders.
Spring Framework Stereotype Annotations
This annotation is used on classes to indicate a Spring component. The @Component annotation marks the Java class as a bean or say component so that the component-scanning mechanism of Spring can add into the application context.
@Controller
The @Controller annotation is used to indicate the class is a Spring controller. This annotation can be used to identify controllers for Spring MVC or Spring WebFlux.
This annotation is used on a class. The @Service marks a Java class that performs some service, such as execute business logic, perform calculations and call external APIs. This annotation is a specialized form of the @Component annotation intended to be used in the service layer.
@Repository
This annotation is used on Java classes which directly access the database. The @Repository annotation works as marker for any class that fulfills the role of repository or Data Access Object.
This annotation has a automatic translation feature. For example, when an exception occurs in the @Repository there is a handler for that exception and there is no need to add a try catch block.
Spring Boot Annotations
- @EnableAutoConfiguration
This annotation is usually placed on the main application class. The @EnableAutoConfiguration annotation implicitly defines a base “search package”. This annotation tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.
@SpringBootApplication
This annotation is used on the application class while setting up a Spring Boot project. The class that is annotated with the @SpringBootApplication must be kept in the base package. The one thing that the @SpringBootApplication does is a component scan. But it will scan only its sub-packages. As an example, if you put the class annotated with @SpringBootApplication in com.example then @SpringBootApplication will scan all its sub-packages, such as com.example.a , com.example.b , and com.example.a.x .
The @SpringBootApplication is a convenient annotation that adds all the following:
Spring MVC and REST Annotations
This annotation is used on Java classes that play the role of controller in your application. The @Controller annotation allows autodetection of component classes in the classpath and auto-registering bean definitions for them. To enable autodetection of such annotated controllers, you can add component scanning to your configuration. The Java class annotated with @Controller is capable of handling multiple request mappings.
This annotation can be used with Spring MVC and Spring WebFlux.
@RequestMapping
This annotation is used both at class and method level. The @RequestMapping annotation is used to map web requests onto specific handler classes and handler methods. When @RequestMapping is used on class level it creates a base URI for which the controller will be used. When this annotation is used on methods it will give you the URI on which the handler methods will be executed. From this you can infer that the class level request mapping will remain the same whereas each handler method will have their own request mapping.
Sometimes you may want to perform different operations based on the HTTP method used, even though the request URI may remain the same. In such situations, you can use the method attribute of @RequestMapping with an HTTP method value to narrow down the HTTP methods in order to invoke the methods of your class.
Here is a basic example on how a controller along with request mappings work:
In this example only GET requests to /welcome is handled by the welcomeAll() method.
This annotation also can be used with Spring MVC and Spring WebFlux.
The @RequestMapping annotation is very versatile. Please see my in depth post on Request Mapping bere .
@CookieValue
This annotation is used at method parameter level. @CookieValue is used as argument of request mapping method. The HTTP cookie is bound to the @CookieValue parameter for a given cookie name. This annotation is used in the method annotated with @RequestMapping . Let us consider that the following cookie value is received with a http request:
JSESSIONID=418AB76CD83EF94U85YD34W
To get the value of the cookie, use @CookieValue like this:
@CrossOrigin
This annotation is used both at class and method level to enable cross origin requests. In many cases the host that serves JavaScript will be different from the host that serves the data. In such a case Cross Origin Resource Sharing (CORS) enables cross-domain communication. To enable this communication you just need to add the @CrossOrigin annotation.
By default the @CrossOrigin annotation allows all origin, all headers, the HTTP methods specified in the @RequestMapping annotation and maxAge of 30 min. You can customize the behavior by specifying the corresponding attribute values.
An example to use @CrossOrigin at both controller and handler method levels is this.
In this example, both getExample() and getNote() methods will have a maxAge of 3600 seconds. Also, getExample() will only allow cross-origin requests from http://example.com , while getNote() will allow cross-origin requests from all hosts.
Composed @RequestMapping Variants
Spring framework 4.3 introduced the following method-level variants of @RequestMapping annotation to better express the semantics of the annotated methods. Using these annotations have become the standard ways of defining the endpoints. They act as wrapper to @RequestMapping.
These annotations can be used with Spring MVC and Spring WebFlux.
@GetMapping
This annotation is used for mapping HTTP GET requests onto specific handler methods. @GetMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.GET)
@PostMapping
This annotation is used for mapping HTTP POST requests onto specific handler methods. @PostMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.POST)
@PutMapping
This annotation is used for mapping HTTP PUT requests onto specific handler methods. @PutMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PUT)
@PatchMapping
This annotation is used for mapping HTTP PATCH requests onto specific handler methods. @PatchMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PATCH)
@DeleteMapping
This annotation is used for mapping HTTP DELETE requests onto specific handler methods. @DeleteMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.DELETE)
[divider style=”4″]
@ExceptionHandler
This annotation is used at method levels to handle exception at the controller level. The @ExceptionHandler annotation is used to define the class of exception it will catch. You can use this annotation on methods that should be invoked to handle an exception. The @ExceptionHandler values can be set to an array of Exception types. If an exception is thrown that matches one of the types in the list, then the method annotated with matching @ExceptionHandler will be invoked.
@InitBinder
This annotation is a method level annotation that plays the role of identifying the methods which initialize the WebDataBinder – a DataBinder that binds the request parameter to JavaBean objects. To customise request parameter data binding , you can use @InitBinder annotated methods within our controller. The methods annotated with @InitBinder all argument types that handler methods support. The @InitBinder annotated methods will get called for each HTTP request if you don’t specify the value element of this annotation. The value element can be a single or multiple form names or request parameters that the init binder method is applied to.
@Mappings and @Mapping
This annotation is used on fields. The @Mapping annotation is a meta annotation that indicates a web mapping annotation. When mapping different field names, you need to configure the source field to its target field and to do that you have to add the @Mappings annotation. This annotation accepts an array of @Mapping having the source and the target fields.
@MatrixVariable
This annotation is used to annotate request handler method arguments so that Spring can inject the relevant bits of matrix URI. Matrix variables can appear on any segment each separated by a semicolon. If a URL contains matrix variables, the request mapping pattern must represent them with a URI template. The @MatrixVariable annotation ensures that the request is matched with the correct matrix variables of the URI.
@PathVariable
This annotation is used to annotate request handler method arguments. The @RequestMapping annotation can be used to handle dynamic changes in the URI where certain URI value acts as a parameter. You can specify this parameter using a regular expression. The @PathVariable annotation can be used declare this parameter.
@RequestAttribute
This annotation is used to bind the request attribute to a handler method parameter. Spring retrieves the named attributes value to populate the parameter annotated with @RequestAttribute . While the @RequestParam annotation is used bind the parameter values from query string, the @RequestAttribute is used to access the objects which have been populated on the server side.
@RequestBody
This annotation is used to annotate request handler method arguments. The @RequestBody annotation indicates that a method parameter should be bound to the value of the HTTP request body. The HttpMessageConveter is responsible for converting from the HTTP request message to object.
@RequestHeader
This annotation is used to annotate request handler method arguments. The @RequestHeader annotation is used to map controller parameter to request header value. When Spring maps the request, @RequestHeader checks the header with the name specified within the annotation and binds its value to the handler method parameter. This annotation helps you to get the header details within the controller class.
@RequestParam
This annotation is used to annotate request handler method arguments. Sometimes you get the parameters in the request URL, mostly in GET requests. In that case, along with the @RequestMapping annotation you can use the @RequestParam annotation to retrieve the URL parameter and map it to the method argument. The @RequestParam annotation is used to bind request parameters to a method parameter in your controller.
@RequestPart
This annotation is used to annotate request handler method arguments. The @RequestPart annotation can be used instead of @RequestParam to get the content of a specific multipart and bind to the method argument annotated with @RequestPart . This annotation takes into consideration the “Content-Type” header in the multipart(request part).
@ResponseBody
This annotation is used to annotate request handler methods. The @ResponseBody annotation is similar to the @RequestBody annotation. The @ResponseBody annotation indicates that the result type should be written straight in the response body in whatever format you specify like JSON or XML. Spring converts the returned object into a response body by using the HttpMessageConveter .
@ResponseStatus
This annotation is used on methods and exception classes. @ResponseStatus marks a method or exception class with a status code and a reason that must be returned. When the handler method is invoked the status code is set to the HTTP response which overrides the status information provided by any other means. A controller class can also be annotated with @ResponseStatus which is then inherited by all @RequestMapping methods.
@ControllerAdvice
This annotation is applied at the class level. As explained earlier, for each controller you can use @ExceptionHandler on a method that will be called when a given exception occurs. But this handles only those exception that occur within the controller in which it is defined. To overcome this problem you can now use the @ControllerAdvice annotation. This annotation is used to define @ExceptionHandler , @InitBinder and @ModelAttribute methods that apply to all @RequestMapping methods. Thus if you define the @ExceptionHandler annotation on a method in @ControllerAdvice class, it will be applied to all the controllers.
@RestController
This annotation is used at the class level. The @RestController annotation marks the class as a controller where every method returns a domain object instead of a view. By annotating a class with this annotation you no longer need to add @ResponseBody to all the RequestMapping method. It means that you no more use view-resolvers or send html in response. You just send the domain object as HTTP response in the format that is understood by the consumers like JSON.
@RestController is a convenience annotation which combines @Controller and @ResponseBody .
@RestControllerAdvice
This annotation is applied on Java classes. @RestControllerAdvice is a convenience annotation which combines @ControllerAdvice and @ResponseBody . This annotation is used along with the @ExceptionHandler annotation to handle exceptions that occur within the controller.
@SessionAttribute
This annotation is used at method parameter level. The @SessionAttribute annotation is used to bind the method parameter to a session attribute. This annotation provides a convenient access to the existing or permanent session attributes.
@SessionAttributes
This annotation is applied at type level for a specific handler. The @SessionAtrributes annotation is used when you want to add a JavaBean object into a session. This is used when you want to keep the object in session for short lived. @SessionAttributes is used in conjunction with @ModelAttribute . Consider this example.
The @ModelAttribute name is assigned to the @SessionAttributes as value. The @SessionAttributes has two elements. The value element is the name of the session in the model and the types element is the type of session attributes in the model.
Spring Cloud Annotations
@enableconfigserver.
This annotation is used at the class level. When developing a project with a number of services, you need to have a centralized and straightforward manner to configure and retrieve the configurations about all the services that you are going to develop. One advantage of using a centralized config server is that you don’t need to carry the burden of remembering where each configuration is distributed across multiple and distributed components.
You can use Spring cloud’s @EnableConfigServer annotation to start a config server that the other applications can talk to.
@EnableEurekaServer
This annotation is applied to Java classes. One problem that you may encounter while decomposing your application into microservices is that, it becomes difficult for every service to know the address of every other service it depends on. There comes the discovery service which is responsible for tracking the locations of all other microservices. Netflix’s Eureka is an implementation of a discovery server and integration is provided by Spring Boot. Spring Boot has made it easy to design a Eureka Server by just annotating the entry class with @EnableEurekaServer .
@EnableDiscoveryClient
This annotation is applied to Java classes. In order to tell any application to register itself with Eureka you just need to add the @EnableDiscoveryClient annotation to the application entry point. The application that’s now registered with Eureka uses the Spring Cloud Discovery Client abstraction to interrogate the registry for its own host and port.
@EnableCircuitBreaker
This annotation is applied on Java classes that can act as the circuit breaker. The circuit breaker pattern can allow a micro service continue working when a related service fails, preventing the failure from cascading. This also gives the failed service a time to recover.
The class annotated with @EnableCircuitBreaker will monitor, open, and close the circuit breaker.
@HystrixCommand
This annotation is used at the method level. Netflix’s Hystrix library provides the implementation of Circuit Breaker pattern. When you apply the circuit breaker to a method, Hystrix watches for the failures of the method. Once failures build up to a threshold, Hystrix opens the circuit so that the subsequent calls also fail. Now Hystrix redirects calls to the method and they are passed to the specified fallback methods. Hystrix looks for any method annotated with the @HystrixCommand annotation and wraps it into a proxy connected to a circuit breaker so that Hystrix can monitor it.
Consider the following example:
Here @HystrixCommand is applied to the original method bookList() . The @HystrixCommand annotation has newList as the fallback method. So for some reason if Hystrix opens the circuit on bookList() , you will have a placeholder book list ready for the users.
Spring Framework DataAccess Annotations
@transactional.
This annotation is placed before an interface definition, a method on an interface, a class definition, or a public method on a class. The mere presence of @Transactional is not enough to activate the transactional behaviour. The @Transactional is simply a metadata that can be consumed by some runtime infrastructure. This infrastructure uses the metadata to configure the appropriate beans with transactional behaviour.
The annotation further supports configuration like:
- The Propagation type of the transaction
- The Isolation level of the transaction
- A timeout for the operation wrapped by the transaction
- A read only flag – a hint for the persistence provider that the transaction must be read only The rollback rules for the transaction
Cache-Based Annotations
This annotation is used on methods. The simplest way of enabling the cache behaviour for a method is to annotate it with @Cacheable and parameterize it with the name of the cache where the results would be stored.
In the snippet above , the method getAddress is associated with the cache named addresses. Each time the method is called, the cache is checked to see whether the invocation has been already executed and does not have to be repeated.
This annotation is used on methods. Whenever you need to update the cache without interfering the method execution, you can use the @CachePut annotation. That is, the method will always be executed and the result cached.
Using @CachePut and @Cacheable on the same method is strongly discouraged as the former forces the execution in order to execute a cache update, the latter causes the method execution to be skipped by using the cache.
@CacheEvict
This annotation is used on methods. It is not that you always want to populate the cache with more and more data. Sometimes you may want remove some cache data so that you can populate the cache with some fresh values. In such a case use the @CacheEvict annotation.
Here an additional element allEntries is used along with the cache name to be emptied. It is set to true so that it clears all values and prepares to hold new data.
@CacheConfig
This annotation is a class level annotation. The @CacheConfig annotation helps to streamline some of the cache information at one place. Placing this annotation on a class does not turn on any caching operation. This allows you to store the cache configuration at the class level so that you don’t have declare things multiple times.
Task Execution and Scheduling Annotations
This annotation is a method level annotation. The @Scheduled annotation is used on methods along with the trigger metadata. A method with @Scheduled should have void return type and should not accept any parameters.
There are different ways of using the @Scheduled annotation:
In this case, the duration between the end of last execution and the start of next execution is fixed. The tasks always wait until the previous one is finished.
In this case, the beginning of the task execution does not wait for the completion of the previous execution.
The task gets executed initially with a delay and then continues with the specified fixed rate.
This annotation is used on methods to execute each method in a separate thread. The @Async annotation is provided on a method so that the invocation of that method will occur asynchronously. Unlike methods annotated with @Scheduled , the methods annotated with @Async can take arguments. They will be invoked in the normal way by callers at runtime rather than by a scheduled task.
@Async can be used with both void return type methods and the methods that return a value. However methods with return value must have a Future typed return values.
Spring Framework Testing Annotations
@bootstrapwith.
This annotation is a class level annotation. The @BootstrapWith annotation is used to configure how the Spring TestContext Framework is bootstrapped. This annotation is used as a metadata to create custom composed annotations and reduce the configuration duplication in a test suite.
@ContextConfiguration
This annotation is a class level annotation that defines a metadata used to determine which configuration files to use to the load the ApplicationContext for your test. More specifically @ContextConfiguration declares the annotated classes that will be used to load the context. You can also tell Spring where to locate for the file. @ContextConfiguration(locations={"example/test-context.xml", loader = Custom ContextLoader.class})
@WebAppConfiguration
This annotation is a class level annotation. The @WebAppConfiguration is used to declare that the ApplicationContext loaded for an integration test should be a WebApplicationContext. This annotation is used to create the web version of the application context. It is important to note that this annotation must be used with the @ContextConfiguration annotation.The default path to the root of the web application is src/main/webapp. You can override it by passing a different path to the @WebAppConfiguration .
This annotation is used on methods. The @Timed annotation indicates that the annotated test method must finish its execution at the specified time period(in milliseconds). If the execution exceeds the specified time in the annotation, the test fails.
In this example, the test will fail if it exceeds 10 seconds of execution.
This annotation is used on test methods. If you want to run a test method several times in a row automatically, you can use the @Repeat annotation. The number of times that test method is to be executed is specified in the annotation.
In this example, the test will be executed 10 times.
This annotation can be used as both class-level or method-level annotation. After execution of a test method, the transaction of the transactional test method can be committed using the @Commit annotation. This annotation explicitly conveys the intent of the code. When used at the class level, this annotation defines the commit for all test methods within the class. When declared as a method level annotation @Commit specifies the commit for specific test methods overriding the class level commit.
This annotation can be used as both class-level and method-level annotation. The @RollBack annotation indicates whether the transaction of a transactional test method must be rolled back after the test completes its execution. If this true @Rollback(true) , the transaction is rolled back. Otherwise, the transaction is committed. @Commit is used instead of @RollBack(false) .
When used at the class level, this annotation defines the rollback for all test methods within the class.
When declared as a method level annotation @RollBack specifies the rollback for specific test methods overriding the class level rollback semantics.
@DirtiesContext
This annotation is used as both class-level and method-level annotation. @DirtiesContext indicates that the Spring ApplicationContext has been modified or corrupted in some manner and it should be closed. This will trigger the context reloading before execution of next test. The ApplicationContext is marked as dirty before or after any such annotated method as well as before or after current test class.
The @DirtiesContext annotation supports BEFORE_METHOD , BEFORE_CLASS , and BEFORE_EACH_TEST_METHOD modes for closing the ApplicationContext before a test.
NOTE : Avoid overusing this annotation. It is an expensive operation and if abused, it can really slow down your test suite.
@BeforeTransaction
This annotation is used to annotate void methods in the test class. @BeforeTransaction annotated methods indicate that they should be executed before any transaction starts executing. That means the method annotated with @BeforeTransaction must be executed before any method annotated with @Transactional .
@AfterTransaction
This annotation is used to annotate void methods in the test class. @AfterTransaction annotated methods indicate that they should be executed after a transaction ends for test methods. That means the method annotated with @AfterTransaction must be executed after the method annotated with @Transactional .
This annotation can be declared on a test class or test method to run SQL scripts against a database. The @Sql annotation configures the resource path to SQL scripts that should be executed against a given database either before or after an integration test method. When @Sql is used at the method level it will override any @Sql defined in at class level.
This annotation is used along with the @Sql annotation. The @SqlConfig annotation defines the metadata that is used to determine how to parse and execute SQL scripts configured via the @Sql annotation. When used at the class-level, this annotation serves as global configuration for all SQL scripts within the test class. But when used directly with the config attribute of @Sql , @SqlConfig serves as a local configuration for SQL scripts declared.
This annotation is used on methods. The @SqlGroup annotation is a container annotation that can hold several @Sql annotations. This annotation can declare nested @Sql annotations. In addition, @SqlGroup is used as a meta-annotation to create custom composed annotations. This annotation can also be used along with repeatable annotations, where @Sql can be declared several times on the same method or class.
@SpringBootTest
This annotation is used to start the Spring context for integration tests. This will bring up the full autoconfigruation context.
@DataJpaTest
The @DataJpaTest annotation will only provide the autoconfiguration required to test Spring Data JPA using an in-memory database such as H2.
This annotation is used instead of @SpringBootTest
@DataMongoTest
The @DataMongoTest will provide a minimal autoconfiguration and an embedded MongoDB for running integration tests with Spring Data MongoDB.
@WebMVCTest
The @WebMVCTest will bring up a mock servlet context for testing the MVC layer. Services and components are not loaded into the context. To provide these dependencies for testing, the @MockBean annotation is typically used.
@AutoConfigureMockMVC
The @AutoConfigureMockMVC annotation works very similar to the @WebMVCTest annotation, but the full Spring Boot context is started.
Creates and injects a Mockito Mock for the given dependency.
Will limit the auto configuration of Spring Boot to components relevant to processing JSON.
This annotation will also autoconfigure an instance of JacksonTester or GsonTester .
@TestPropertySource
Class level annotation used to specify property sources for the test class.
- spring framework annotations
You May Also Like
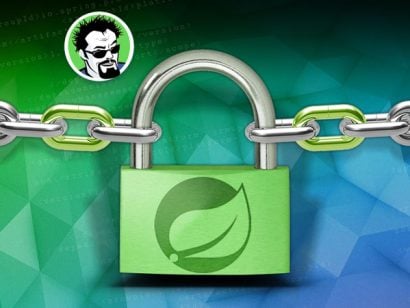
JWT Token Authentication in Spring Boot Microservices
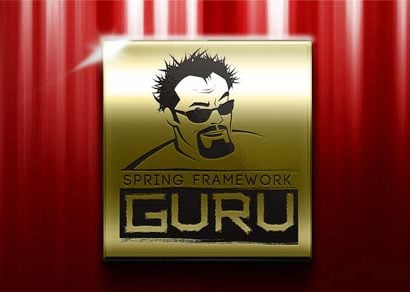
Hikari Configuration for MySQL in Spring Boot 2
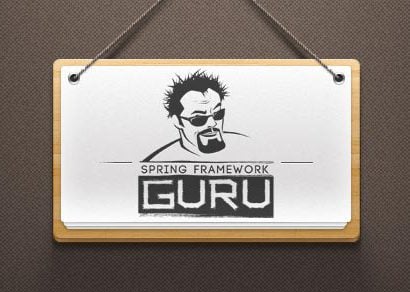
Database Migration with Flyway
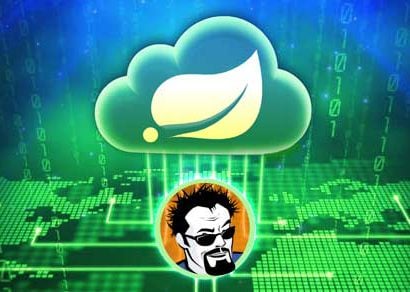
Getting Ready for Spring Framework 6
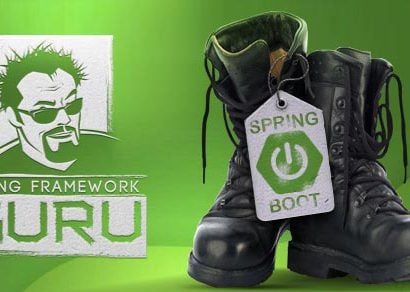
Using Filters in Spring Web Applications
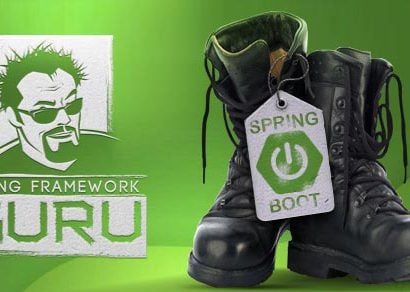
Bootstrapping Data in Spring Boot
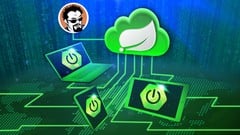
Eureka Service Registry
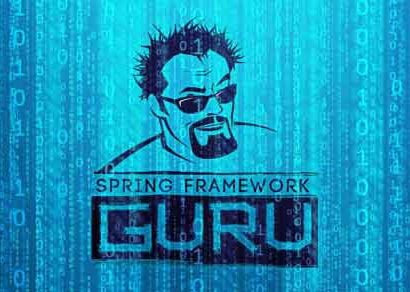
Scheduling in Spring Boot
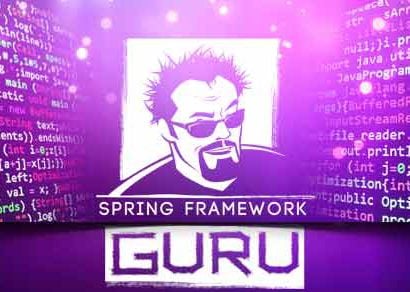
Spring for Apache Kafka
Spring retry.
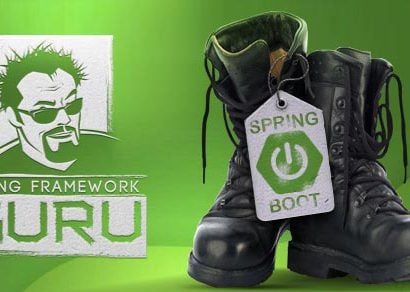
Spring Boot CLI
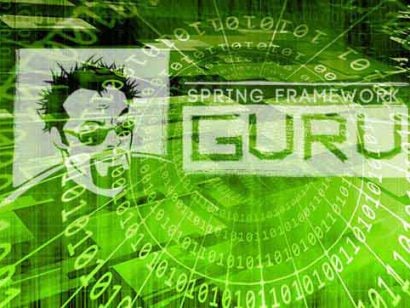
Actuator in Spring Boot
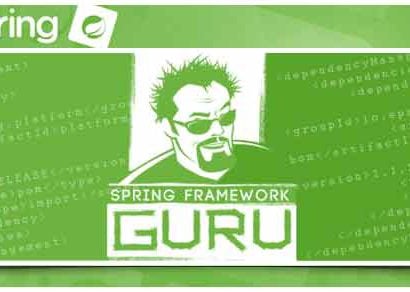
Internationalization with Spring Boot
One-to-one relationship in jpa.
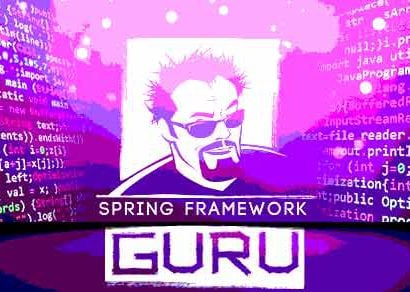
The @RequestBody Annotation
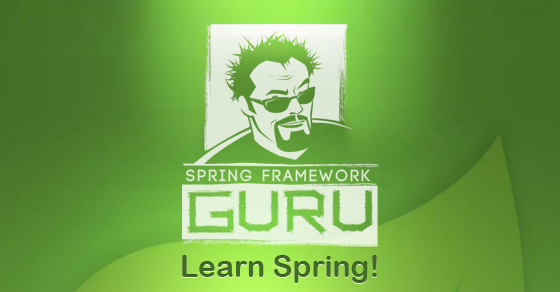
Spring BeanFactory vs ApplicationContext
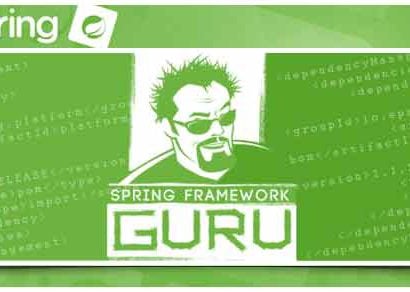
MySQL Stored Procedures with Spring Boot
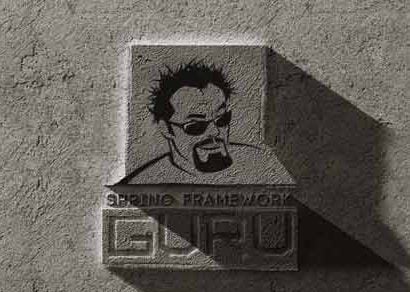
Bean Validation in Spring Boot
Spring state machine.
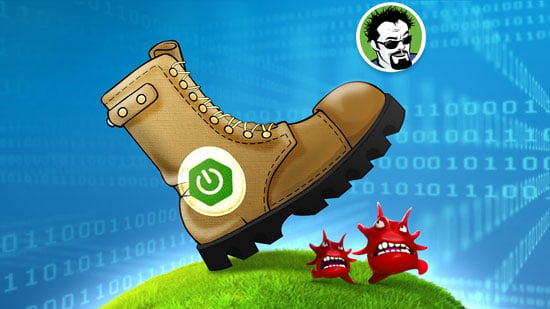
Exception Handling in Spring Boot REST API
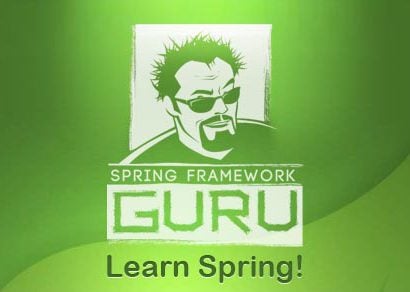
Spring Boot Pagination
Spring rest docs, using mapstruct with project lombok, argumentcaptor in mockito.
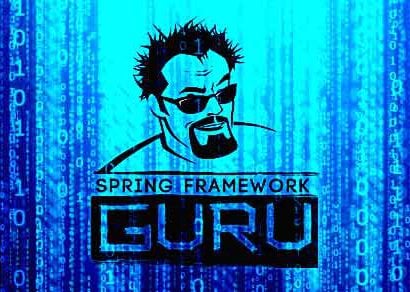
Reading External Configuration Properties in Spring
Api gateway with spring cloud.
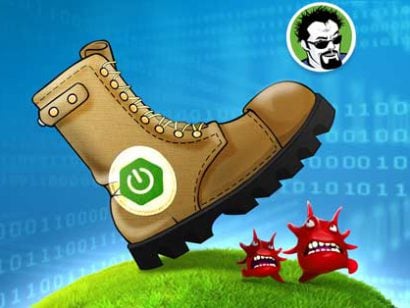
Testing Spring Boot RESTful Services
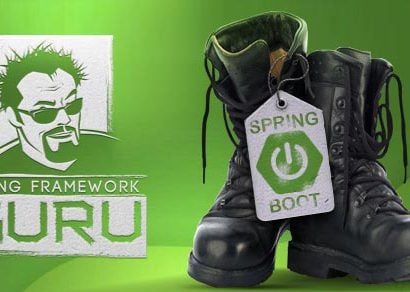
Caching in Spring RESTful Service: Part 2 – Cache Eviction
Spring boot messaging with rabbitmq.
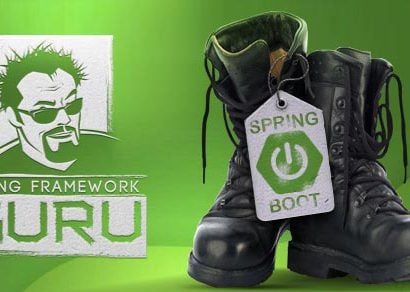
Caching in Spring Boot RESTful Service: Part 1
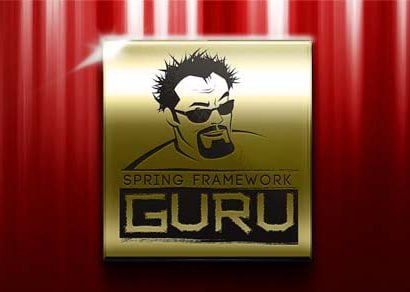
Implementing HTTP Basic Authentication in a Spring Boot REST API
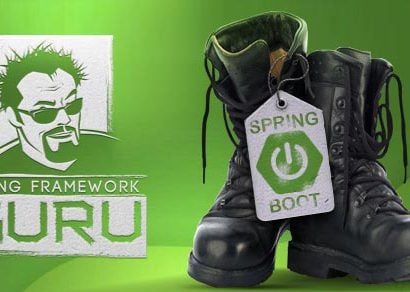
Immutable Property Binding
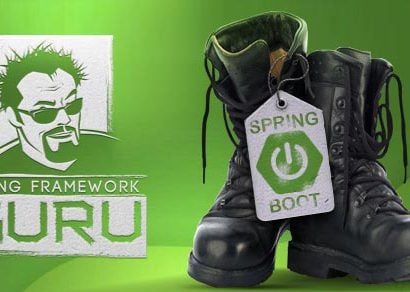
Java Bean Properties Binding
External configuration data in spring.
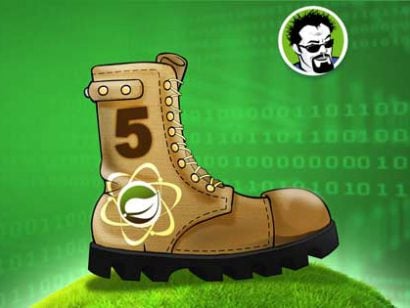
Spring Data JPA @Query
Configuring mysql with circleci.
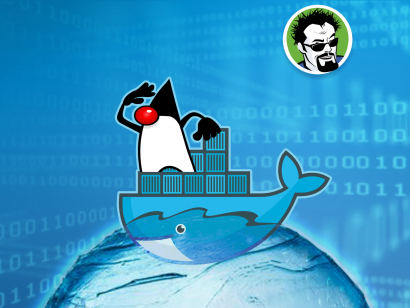
Fabric8 Docker Maven Plugin
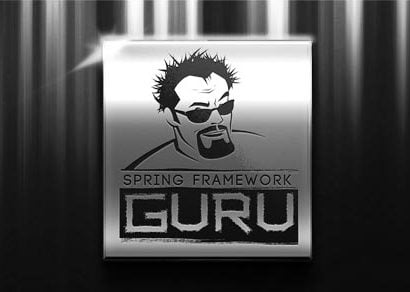
Feign REST Client for Spring Application
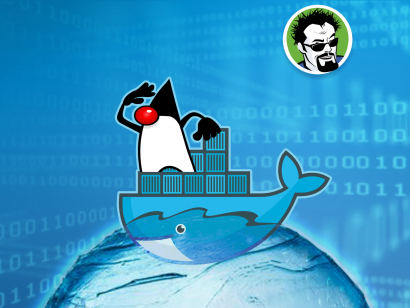
Docker Hub for Spring Boot
Consul miniseries: spring boot application and consul integration part 3, run spring boot on docker, consul miniseries: spring boot application and consul integration part 2.
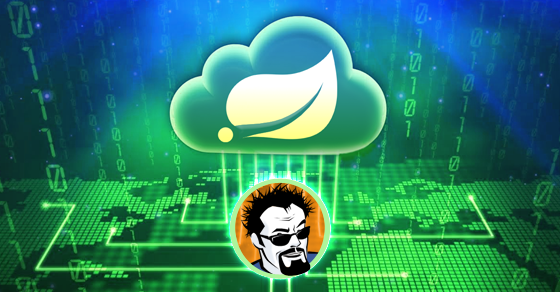
Consul Miniseries: Spring Boot Application and Consul Integration Part 1
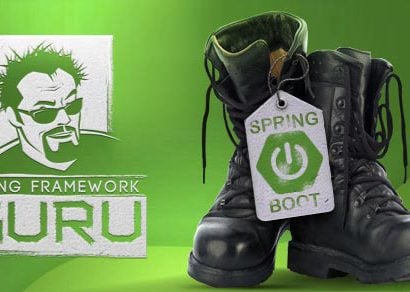
Why You Should be Using Spring Boot Docker Layers
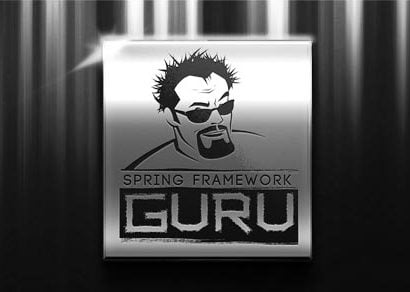
Spring Bean Scopes
Debug your code in intellij idea, stay at home, learn from home with 6 free online courses.
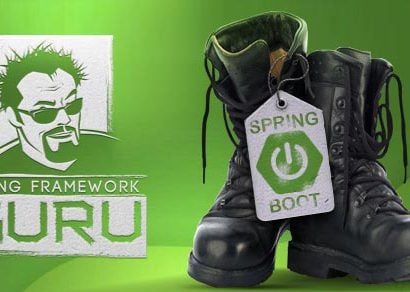
What is the best UI to Use with Spring Boot?
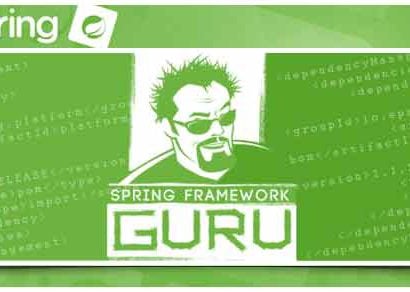
Best Practices for Dependency Injection with Spring
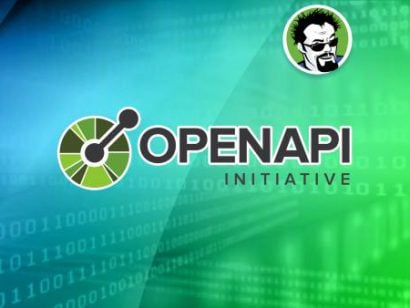
Should I Use Spring REST Docs or OpenAPI?
Spring boot with lombok: part 1.
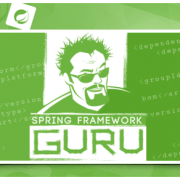
Using Project Lombok with Gradle
Spring bean lifecycle, spring profiles, spring bean definition inheritance, autowiring in spring.
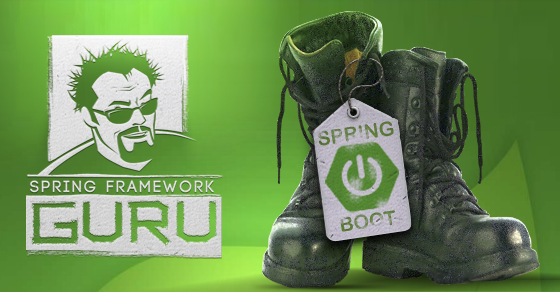
What is New in Spring Boot 2.2?
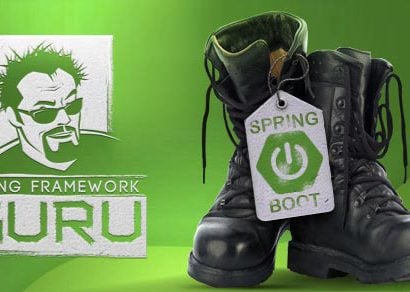
Using Ehcache 3 in Spring Boot
How to configure multiple data sources in a spring boot application.
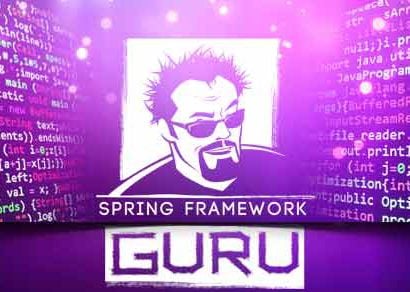
Using RestTemplate with Apaches HttpClient
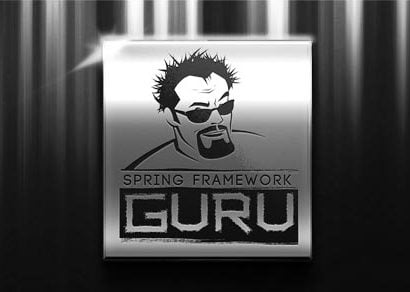
Using RestTemplate in Spring
Working with resources in spring, using spring aware interfaces, service locator pattern in spring, using graphql in a spring boot application, spring jdbctemplate crud operations, contracts for microservices with openapi and spring cloud contract, using swagger request validator to validate spring cloud contracts, spring 5 webclient, defining spring cloud contracts in open api.
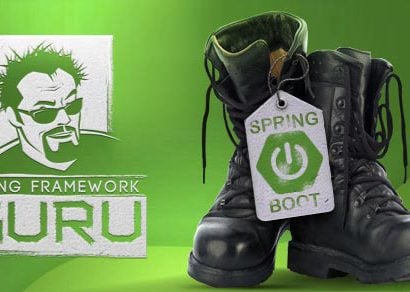
Hibernate Show SQL
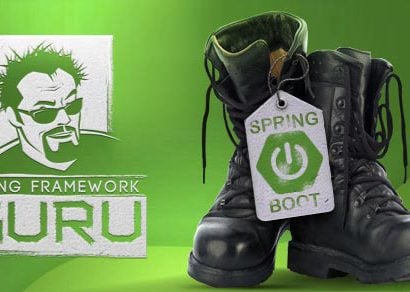
Spring Component Scan
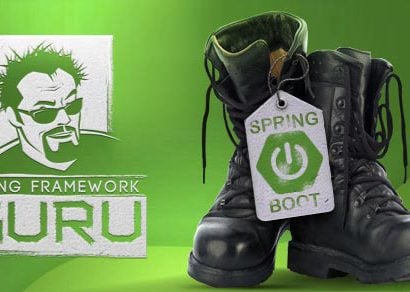
Using CircleCI to Build Spring Boot Microservices
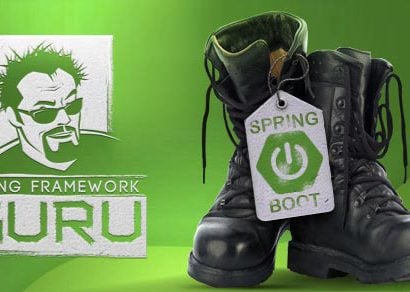
Using JdbcTemplate with Spring Boot and Thymeleaf
Using the spring @requestmapping annotation.
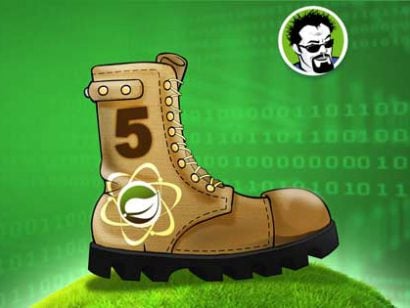
Spring Data MongoDB with Reactive MongoDB
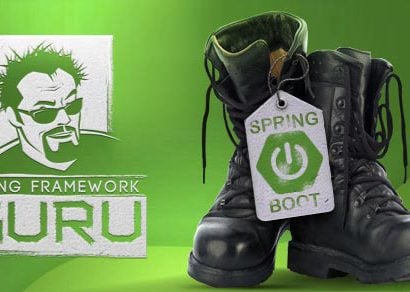
Spring Boot with Embedded MongoDB
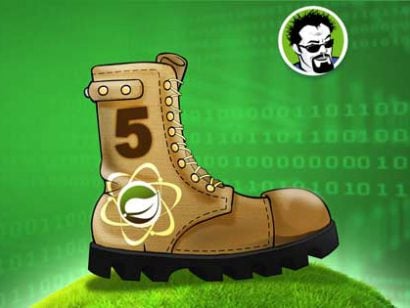
Spring Web Reactive
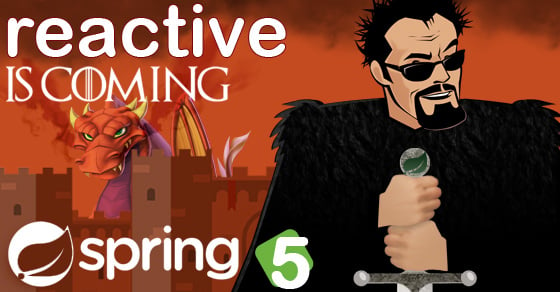
What are Reactive Streams in Java?
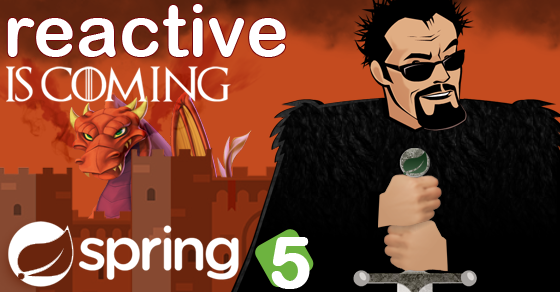
What’s new in Spring Framework 5?
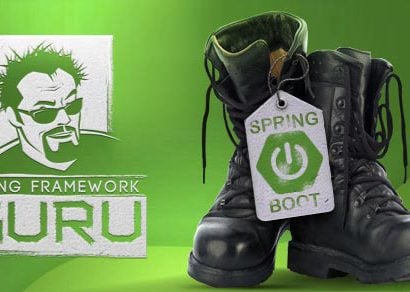
Spring Boot RESTful API Documentation with Swagger 2
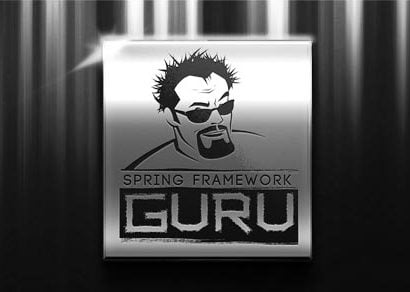
Mockito Mock vs Spy in Spring Boot Tests
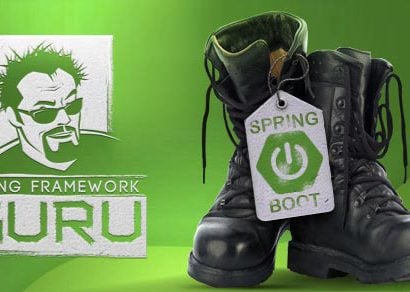
Spring Boot Mongo DB Example Application
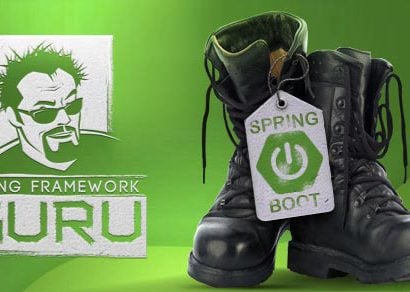
Configuring Spring Boot for MariaDB
Spring boot web application, part 6 – spring security with dao authentication provider.
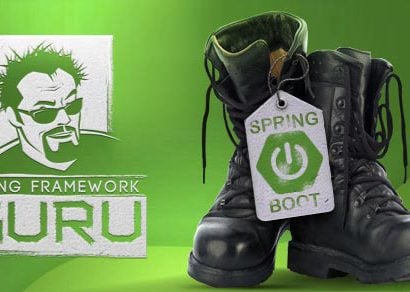
Configuring Spring Boot for MongoDB
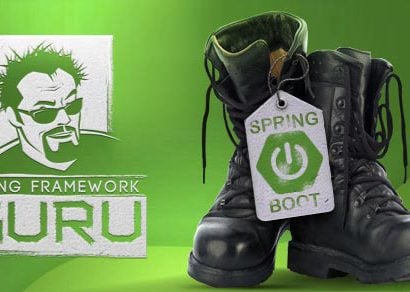
Spring Boot Web Application, Part 5 – Spring Security
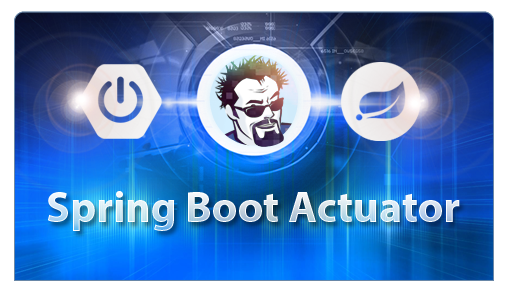
Chuck Norris for Spring Boot Actuator
Testing spring mvc with spring boot 1.4: part 1, running spring boot in a docker container.
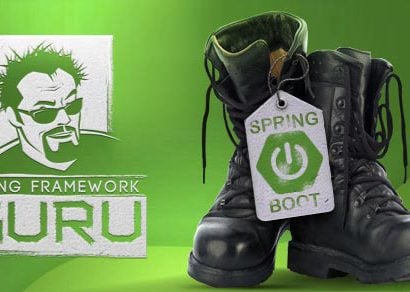
Jackson Dependency Issue in Spring Boot with Maven Build
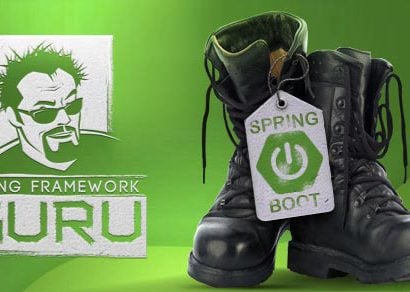
Using YAML in Spring Boot to Configure Logback
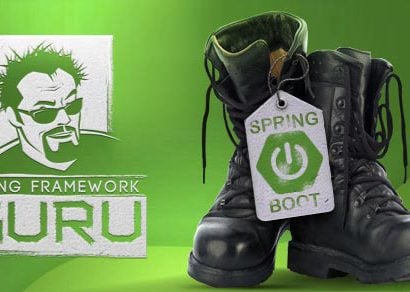
Using Logback with Spring Boot
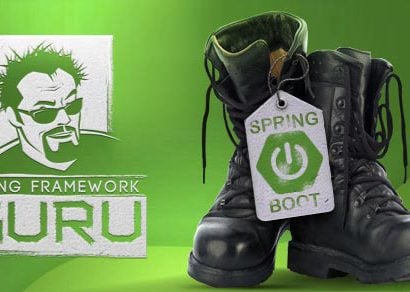
Using Log4J 2 with Spring Boot
Fixing nouniquebeandefinitionexception exceptions, samy is my hero and hacking the magic of spring boot.
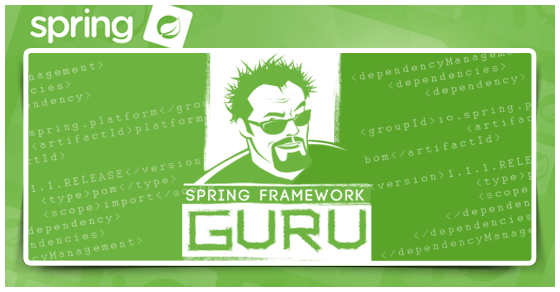
2015 Year in Review
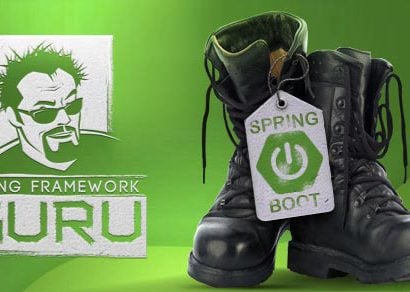
Configuring Spring Boot for PostgreSQL
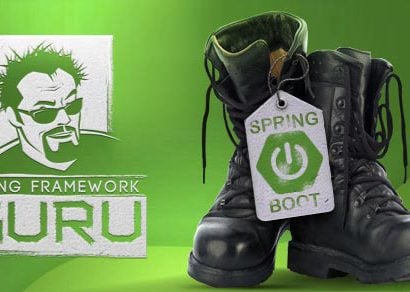
Embedded JPA Entities Under Spring Boot and Hibernate Naming
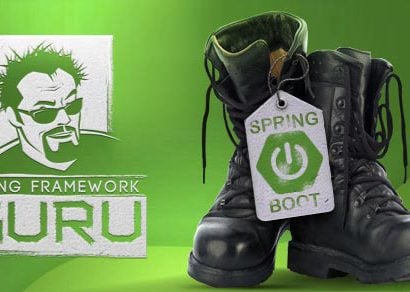
Spring Boot Developer Tools
Spring beanfactoryaware interface.
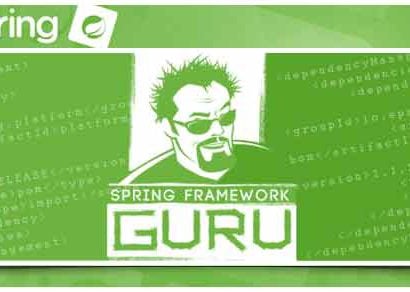
Spring BeanNameAware Interface
Displaying list of objects in table using thymeleaf.
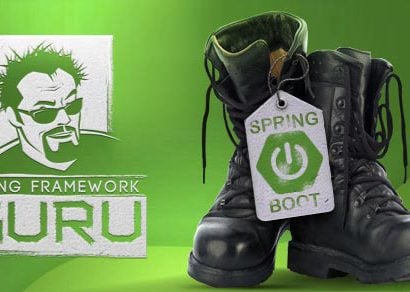
Using H2 and Oracle with Spring Boot
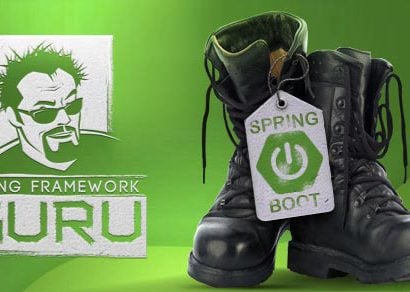
Configuring Spring Boot for Oracle
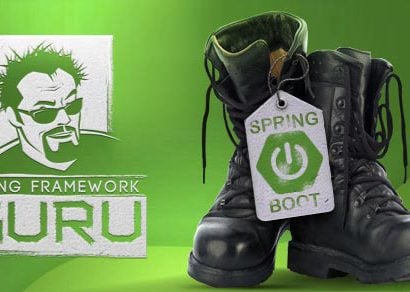
Spring Boot Web Application – Part 4 – Spring MVC
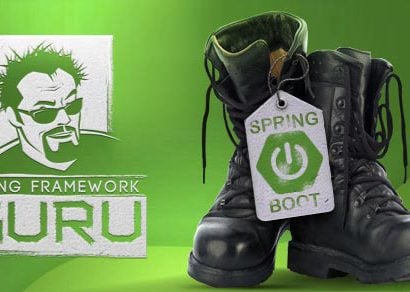
Configuring Spring Boot for MySQL
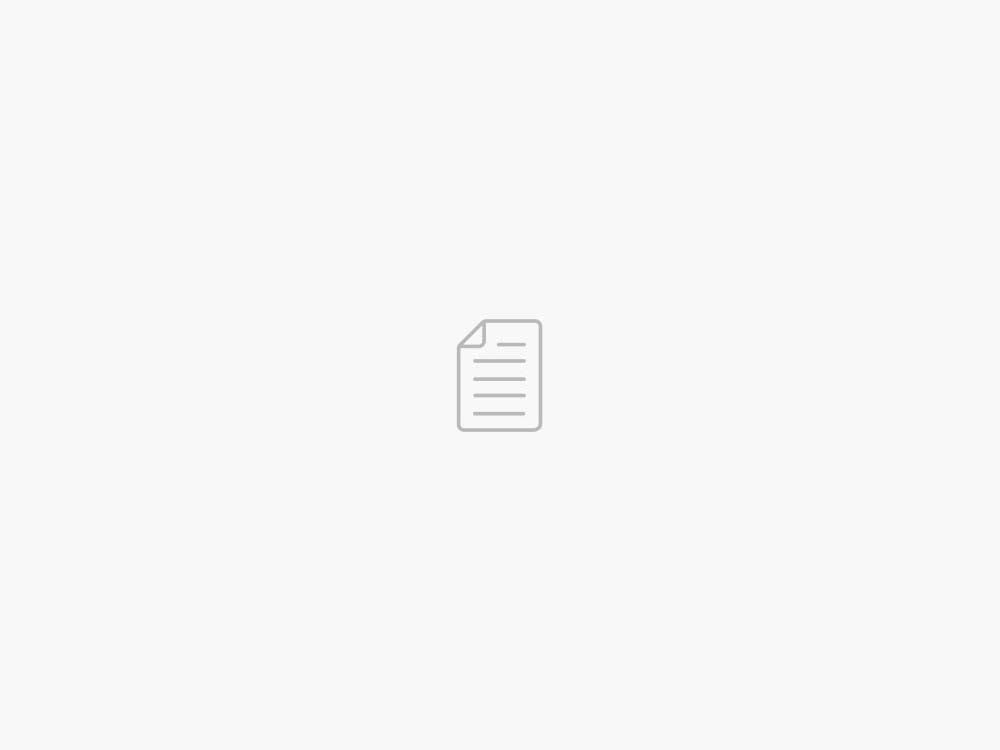
Spring Boot Example of Spring Integration and ActiveMQ
How do i become a java web developer.
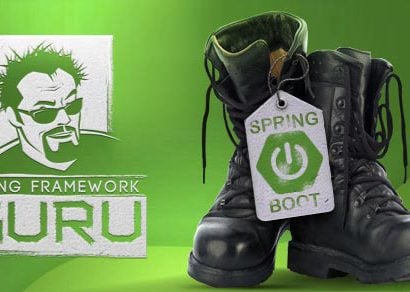
Spring Boot Web Application – Part 3 – Spring Data JPA
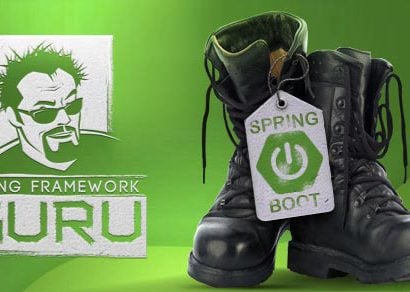
Spring Boot Web Application – Part 2 – Using ThymeLeaf
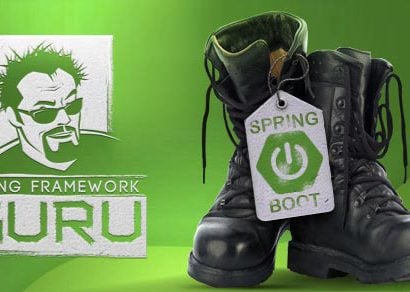
Spring Boot Web Application – Part 1 – Spring Initializr
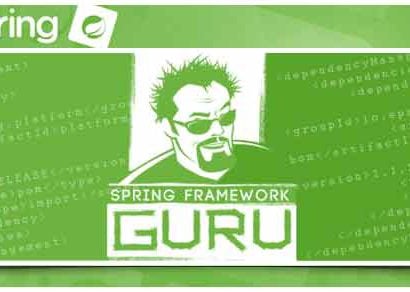
Using the H2 Database Console in Spring Boot with Spring Security
Running code on spring boot startup, integration testing with spring and junit.
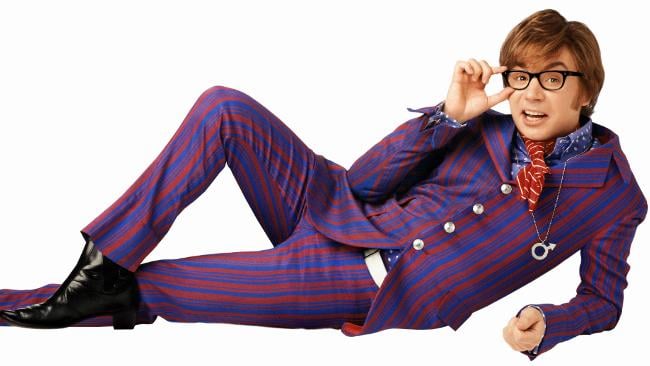
Polyglot Programming in Spring
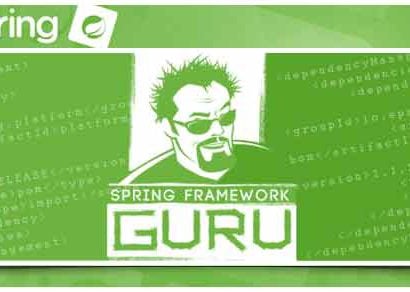
Using Spring Integration Futures
Using the spring framework for enterprise application development.
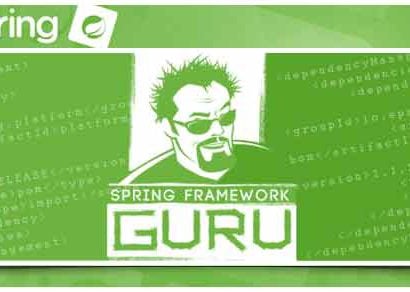
Testing Spring Integration Gateways
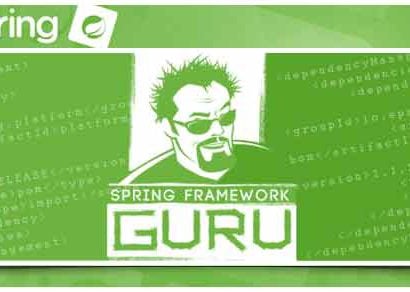
Introduction to Spring Expression Language (SpEL)
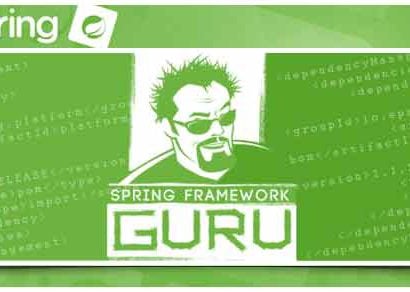
Hello World Using Spring Integration
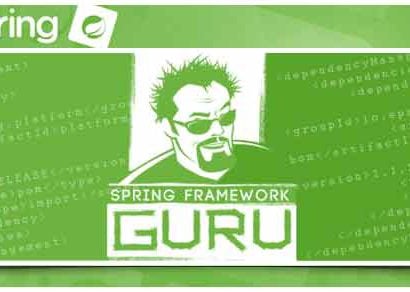
Creating Spring Beans
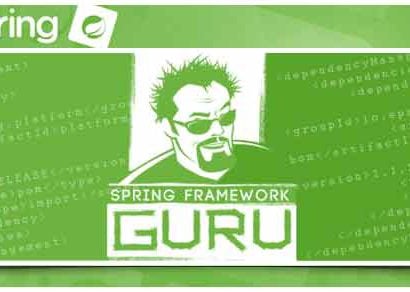
Dependency Injection Example Using Spring
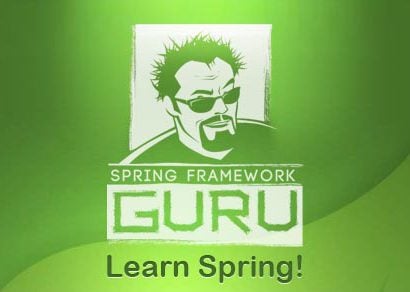
Hello World With Spring 4
Getting started with spring boot.
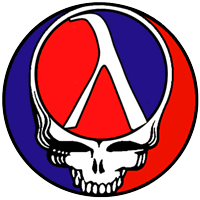
What’s all the fuss about Java Lambdas?
36 comments on “ spring framework annotations ”.
Great summary, thanks. Bookmarked it (on DZone). I found two typos in the examples: @Configu_ar_tion and @Re_u_estMapping(“/cookieValue”)
stephanoapiolaza
Dear, Excelent documentation, thanks for this information
thanks for putting this together..
Thank you all th annotaions described very well!!!
Raviraj Darade
Nice information, Thanks !!!
Mustkeem mansuri
Yeh, its looking good but need to improve more details about annotations.
Simple, Nice and Excellent. Thanks
Ravindra Guvvala
good one ..Very helpful..
Rajeev Kumar Sharma
thanks for putting all together.
Raja Sekhar
Thank you very much. You have put all the information together.
Vikram Aditya
Thank you very much. We need this type of documentation where all the annotations described in one page.
Anamika Pandey
Thanks this was amazingly well put !
Cicciriello
Can I ask why exactly is a poor practice to autowire private fields? I’m new to spring and I’m trying to find my way of doing things
How do you set the property outside of the Spring Context? ie for a unit test? You can’t.
Ashish Kumar Singh
Nice explanation love to read… Can You provide more simple example
Thanks for the great article
It was very handy when we have all the list of annotations at one place.
raghu bhupatiraju
Very well done. Thanks!
A big help!
Good job !! @EnableJpaRepositories and @EntityScan are missing
Very good summary.
Though I came here to look for @EnableWebMvc, which I think needs to be included.
what ever is given spring annotation is correct but you should explain more annotations like @DependsOn.@value,@ComponentScan,@Order and etc .So you have to give more clarity about all anotations
Super explanation on each annotations …
Thanks it helped lots…
Mohammad Zeyaul
Hi This is really a great post but kind of a bit old. Can you please update this.
ravi ranjan
@EnableJpaRepositories It activates the spring JPA repositories defined in your spring-boot application. It carries same namespace as the XML namespace does.
What is the use of @transparent annotation ?
@EventListner? @TransactionEventListner?
Leave a Reply Cancel Reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
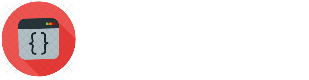
Press ESC to close
![All Spring Annotations ASAP Cheat Sheet [Core+Boot][2023] 1 All Spring Annotations Explained Cheat Sheet](https://www.adevguide.com/wp-content/uploads/2019/09/All-Spring-Annotations-Explained-Cheat-Sheet.png.webp)
All Spring Annotations ASAP Cheat Sheet [Core+Boot][2023]
![All Spring Annotations ASAP Cheat Sheet [Core+Boot][2023] 2 All Spring Annotations Explained Cheat Sheet](https://www.adevguide.com/wp-content/uploads/2019/09/All-Spring-Annotations-Explained-Cheat-Sheet.png.webp)
- 1.1) @Configuration
- 1.2) @ComponentScan
- 1.3) @Autowired
- 1.4) @Component
- 1.6) @Qualifier
- 1.7) @Primary
- 1.8) @Required
- 1.9) @Value
- 1.10) @DependsOn
- 1.11) @Lazy
- 1.12) @Lookup
- 1.13) @Scope
- 1.14) @Profile
- 1.15) @Import
- 1.16) @ImportResource
- 1.17) @PropertySource
- 2.1) @Controller
- 2.2) @Service
- 2.3) @Repository
- 2.4) @RequestMapping
- 2.5) @RequestBody
- 2.6) @GetMapping
- 2.7) @PostMapping
- 2.8) @PutMapping
- 2.9) @DeleteMapping
- 2.10) @PatchMapping
- 2.11) @ControllerAdvice
- 2.12) @ResponseBody
- 2.13) @ExceptionHandler
- 2.14) @ResponseStatus
- 2.15) @PathVariable
- 2.16) @RequestParam
- 2.17) @RestController
- 2.18) @ModelAttribute
- 2.19) @CrossOrigin
- 2.20) @InitBinder
- 3.1) @SpringBootApplication
- 3.2) @EnableAutoConfiguration
- 3.3) @ConditionalOnClass and @ConditionalOnMissingClass
- 3.4) @ConditionalOnBean and @ConditionalOnMissingBean
- 3.5) @ConditionalOnProperty
- 3.6) @ConditionalOnResource
- 3.7) @ConditionalOnWebApplication and @ConditionalOnNotWebApplication
- 3.8) @ConditionalExpression
- 3.9) @Conditional
- 4) Cheat Sheet
- 5) References
All Spring Annotations Explained Cheat Sheet
Nowadays, there are so many spring annotations that it could become overwhelming. Spring Framework started out with XML configuration and over time it has introduced many capabilities to reduce code verbose and get things done quickly. With Spring Boot, we can do almost everything with annotations. Let’s look at all the annotations provided by the Spring Framework.
Spring Core Annotations
@configuration.
@Configuration is used on classes that define beans. @Configuration is an analog for an XML configuration file – it is configured using Java classes. A Java class annotated with @Configuration is a configuration by itself and will have methods to instantiate and configure the dependencies.
@ComponentScan
@ComponentScan is used with the @Configuration annotation to allow Spring to know the packages to scan for annotated components. @ComponentScan is also used to specify base packages using basePackageClasses or basePackage attributes to scan. If specific packages are not defined, scanning will occur from the package of the class that declares this annotation.
@Autowired is used to mark a dependency which Spring is going to resolve and inject automatically. We can use this annotation with a constructor, setter, or field injection.
@Component is used on classes to indicate a Spring component. The @Component annotation marks the Java class as a bean or component so that the component-scanning mechanism of Spring can add it into the application context.
@Bean is a method-level annotation and a direct analog of the XML element. @Bean marks a factory method which instantiates a Spring bean. Spring calls these methods when a new instance of the return type is required.
@Qualifier helps fine-tune annotation-based autowiring. There may be scenarios when we create more than one bean of the same type and want to wire only one of them with a property. This can be controlled using @Qualifier annotation along with the @Autowired annotation. We use @Qualifier along with @Autowired to provide the bean ID or bean name we want to use in ambiguous situations.
We use @Primary to give higher preference to a bean when there are multiple beans of the same type. When a bean is not marked with @Qualifier, a bean marked with @Primary will be served in case on ambiquity.
The @Required annotation is method-level annotation, and shows that the setter method must be configured to be dependency-injected with a value at configuration time. @Required on setter methods to mark dependencies we want to populate through XML; Otherwise, BeanInitializationException will be thrown.
Spring @Value annotation is used to assign default values to variables and method arguments. We can read spring environment variables as well as system variables using @Value annotation. We can use @Value for injecting property values into beans. It’s compatible with the constructor, setter, and field injection.
@DependsOn makes Spring initialize other beans before the annotated one. Usually, this behavior is automatic, based on the explicit dependencies between beans. The @DependsOn annotation may be used on any class directly or indirectly annotated with @Component or on methods annotated with @Bean.
@Lazy makes beans to initialize lazily. By default, the Spring IoC container creates and initializes all singleton beans at the time of application startup. @Lazy annotation may be used on any class directly or indirectly annotated with @Component or on methods annotated with @Bean.
A method annotated with @Lookup tells Spring to return an instance of the method’s return type when we invoke it.
@Scope is used to define the scope of a @Component class or a @Bean definition. It can be either singleton, prototype, request, session, globalSession or some custom scope.
Beans marked with @Profile will be initialized in the container by Spring only when that profile is active. By Default, all beans has “default” value as Profile. We can configure the name of the profile with the value argument of the annotation.
@Import allows to use specific @Configuration classes without component scanning. We can provide those classes with @Import‘s value argument.
@ImportResource
@ImportResource allows to import XML configurations with this annotation. We can specify the XML file locations with the locations argument, or with its alias, the value argument.
@PropertySource
@PropertySource annotation provides a convenient and declarative mechanism for adding a PropertySource to Spring’s Environment. To be used in conjunction with @Configuration classes.
All About Java Regular Expressions Regex 2019
Spring MVC Annotations
@controller.
The @Controller annotation is used to indicate the class is a Spring controller. This annotation is simply a specialization of the @Component class and allows implementation classes to be auto-detected through the class path scanning.
@Service marks a Java class that performs some service, such as executing business logic, performing calculations, and calling external APIs. This annotation is a specialized form of the @Component annotation intended to be used in the service layer.
@Repository
This annotation is used on Java classes that directly access the database. The @Repository annotation works as a marker for any class that fulfills the role of repository or Data Access Object. This annotation has an automatic translation feature. For example, when an exception occurs in the @Repository, there is a handler for that exception and there is no need to add a try-catch block.
@RequestMapping
@RequestMapping marks request handler methods inside @Controller classes. It accepts below options: path/name/value: which URL the method is mapped to. method: compatible HTTP methods. params: filters requests based on presence, absence, or value of HTTP parameters. headers: filters requests based on presence, absence, or value of HTTP headers. consumes: which media types the method can consume in the HTTP request body. produces: which media types the method can produce in the HTTP response body.
@RequestBody
@RequestBody indicates a method parameter should be bound to the body of the web request. It maps the body of the HTTP request to an object. The body of the request is passed through an HttpMessageConverter to resolve the method argument depending on the content type of the request. The deserialization is automatic and depends on the content type of the request.
@GetMapping
@GetMapping is used for mapping HTTP GET requests onto specific handler methods. Specifically, @GetMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.GET).
@PostMapping
@PostMapping is used for mapping HTTP POST requests onto specific handler methods. Specifically, @PostMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.POST).
@PutMapping
@PutMapping is used for mapping HTTP PUT requests onto specific handler methods. Specifically, @PutMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PUT).
@DeleteMapping
@DeleteMapping is used for mapping HTTP DELETE requests onto specific handler methods. Specifically, @DeleteMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.DELETE).
@PatchMapping
@PatchMapping is used for mapping HTTP PATCH requests onto specific handler methods. Specifically, @PatchMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PATCH).
@ControllerAdvice
@ControllerAdvice is applied at the class level. For each controller, you can use @ExceptionHandler on a method that will be called when a given exception occurs. But this handles only those exceptions that occur within the controller in which it is defined. To overcome this problem, you can now use the @ControllerAdvice annotation. This annotation is used to define @ExceptionHandler, @InitBinder, and @ModelAttribute methods that apply to all @RequestMapping methods. Thus, if you define the @ExceptionHandler annotation on a method in a @ControllerAdvice class, it will be applied to all the controllers.
@ResponseBody
@ResponseBody on a request handler method tells Spring to converts the return value and writes it to the HTTP response automatically. It tells Spring to treat the result of the method as the response itself. The @ResponseBody annotation tells a controller that the object returned is automatically serialized into JSON and passed back into the HttpResponse object. If we annotate a @Controller class with this annotation, all request handler methods will use it.
@ExceptionHandler
@ExceptionHandler is used to declare a custom error handler method. Spring calls this method when a request handler method throws any of the specified exceptions. The caught exception can be passed to the method as an argument.
@ResponseStatus
@ResponseStatus is used to specify the desired HTTP status of the response if we annotate a request handler method with this annotation. We can declare the status code with the code argument, or its alias, the value argument.
@PathVariable
@PathVariable is used to indicates that a method argument is bound to a URI template variable. We can specify the URI template with the @RequestMapping annotation and bind a method argument to one of the template parts with @PathVariable.
@RequestParam
@RequestParam indicates that a method parameter should be bound to a web request parameter. We use @RequestParam for accessing HTTP request parameters. With @RequestParam we can specify an injected value when Spring finds no or empty value in the request. To achieve this, we have to set the defaultValue argument.
@RestController
@RestController combines @Controller and @ResponseBody. By annotating the controller class with @RestController annotation, we no longer need to add @ResponseBody to all the request mapping methods.
@ModelAttribute
@ModelAttribute is used to access elements that are already in the model of an MVC @Controller, by providing the model key.
@CrossOrigin
@CrossOrigin enables cross-domain communication for the annotated request handler methods. If we mark a class with it, it applies to all request handler methods in it. We can fine-tune CORS behavior with this annotation’s arguments.
@InitBinder
@InitBinder is a method-level annotation that plays the role of identifying the methods that initialize the WebDataBinder — a DataBinder that binds the request parameter to Java Bean objects. To customize request parameter data binding, you can use @InitBinder annotated methods within our controller. The methods annotated with @InitBinder include all argument types that handler methods support.
The @InitBinder annotated methods will get called for each HTTP request if we don’t specify the value element of this annotation. The value element can be a single or multiple form names or request parameters that the init binder method is applied to.
How is Java Pass by Value and Not by Reference [4 Examples]
Spring Boot Annotations
@springbootapplication.
@SpringBootApplication marks the main class of a Spring Boot application. This is used usually on a configuration class that declares one or more @Bean methods and also triggers auto-configuration and component scanning. The @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan with their default attributes.
@EnableAutoConfiguration
@EnableAutoConfiguration tells Spring Boot to look for auto-configuration beans on its classpath and automatically applies them. It tells Spring Boot to “guess” how you want to configure Spring based on the jar dependencies that you have added. Since spring-boot-starter-web dependency added to classpath leads to configure Tomcat and Spring MVC, the auto-configuration assumes that you are developing a web application and sets up Spring accordingly. This annotation is used with @Configuration.
@ConditionalOnClass and @ConditionalOnMissingClass
The @ConditionalOnClass and @ConditionalOnMissingClass annotations let configuration be included based on the presence or absence of specific classes. With these annotations, Spring will only use the marked auto-configuration bean if the class in the annotation’s argument is present/absent.
@ConditionalOnBean and @ConditionalOnMissingBean
@ConditionalOnBean and @ConditionalOnMissingBean annotations let a bean be included based on the presence or absence of specific beans.
@ConditionalOnProperty
@ConditionalOnProperty annotation lets configuration be included based on a Spring Environment property i.e. make conditions on the values of properties.

@ConditionalOnResource
@ConditionalOnResource annotation lets configuration be included only when a specific resource is present.
@ConditionalOnWebApplication and @ConditionalOnNotWebApplication
@ConditionalOnWebApplication and @ConditionalOnNotWebApplication annotations let configuration be included depending on whether the application is a “web application”. A web application is an application that uses a spring WebApplicationContext, defines a session scope, or has a StandardServletEnvironment.
@ConditionalExpression
@ConditionalExpression is used in more complex situations. Spring will use the marked definition when the SpEL expression is evaluated to true.
@Conditional
@Conditional is used in complex conditions, we can create a class evaluating the custom condition.
Cheat Sheet
![All Spring Annotations ASAP Cheat Sheet [Core+Boot][2023] 3 All Spring Annotations ASAP Cheat Sheet [Core+Boot][2023] 1](https://www.adevguide.com/wp-content/uploads/2019/09/Spring-Annotations-ADevGuide-1024x628.jpg.webp)
View/Download Cheat Sheet in HD
https://dzone.com https://www.baeldung.com https://jrebel.com/
Share Article:
Pratik bhuite.
In 2019, Pratik Bhuite established ADevGuide, a platform where his passion for technology shines. As a seasoned Full Stack Developer, he's dedicated to absorbing, expanding, and disseminating captivating tech insights. Pratik is presently immersed in the worlds of Java, Oracle, JavaScript, HTML5, and Bootstrap4, contributing to his dynamic expertise.
Most Important Spring Boot Interview Questions [2020]
13 most basic docker commands every developer should know.
Thank you very much for this mate, you saved my life!!!
Welcome, Philip.
Leave a Reply Cancel reply
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar
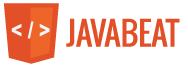
Java Tutorial Blog
- Spring Tutorials
- Spring 4 Tutorials
- Spring Boot
- JSF Tutorials
- Binary Search Tree Traversal
- Spring Batch Tutorial
- AngularJS + Spring MVC
- Spring Data JPA Tutorial
- Packaging and Deploying Node.js
Spring Annotations [Quick Reference]
June 19, 2022 // by ClientAdministrator
Below is the summary of the spring annotations that people use most frequently across spring applications. Spring Framework 2.5 added the annotations to the framework.
Before that, the XML files defined every configuration. Annotations became more convenient to reduce the XML configuration files and annotate the classes and methods with different annotations used at the time of scanning the files in the classpath .
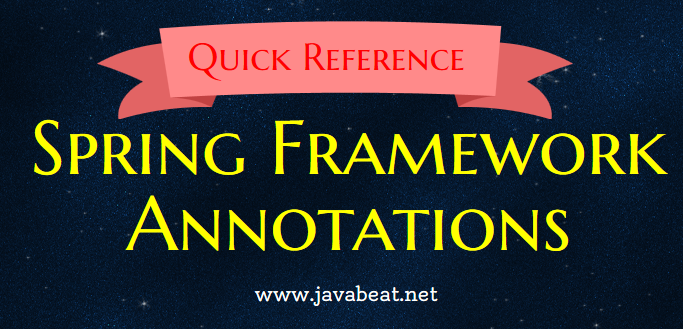
In this tutorial, I will list down the essential spring annotations used in Spring Core, Spring MVC , Spring Boot , Spring Data JPA , etc. modules previously published in this blog . I will continuously update this reference when I am writing the tutorial for new annotations.
You can bookmark this tutorial for quick reference of the most important annotations used in spring applications.
Spring Annotations Reference
I will be adding more tutorials on annotations on this page. The above list will be updated frequently to reflect the latest spring annotations in the framework. If you are interested in receiving the updates on spring framework, please subscribe here .
Thank you for reading my blog!! Happy Learning!! If you have any questions related to spring development, please drop a comment or send me a mail. I will try my best to respond to your queries.

Java Guides
Search this blog, java, java ee, spring and spring boot annotations with examples.
This page contains a one-stop shop for commonly used Java, Java EE, Spring, and Spring Boot annotations with examples. Each annotation is explained with source code examples and its usage.
1. Spring Core Annotations
List of Spring core annotations tutorials
@Component Annotation
The @Component annotation indicates that an annotated class is a “spring bean/component”. The @Component annotation tells the Spring container to automatically create Spring bean.
@Autowired Annotation
The @Autowired annotation is used to inject the bean automatically. The @Autowired annotation is used in constructor injection, setter injection, and field injection
@Qualifier Annotation
@Qualifier annotation is used in conjunction with Autowired to avoid confusion when we have two or more beans configured for the same type.
@Primary Annotation
We use @Primary annotation to give higher preference to a bean when there are multiple beans of the same type.
@Bean Annotation
@Bean annotation indicates that a method produces a bean to be managed by the Spring container. The @Bean annotation is usually declared in the Configuration class to create Spring Bean definitions.
@Lazy Annotation
By default, Spring creates all singleton beans eagerly at the startup/bootstrapping of the application context. You can load the Spring beans lazily (on-demand) using @Lazy annotation.
@Scope Annotation
The @Scope annotation is used to define the scope of the bean. We use @Scope to define the scope of a @Component class or a @Bean definition.
@Value Annotation
Spring @Value annotation is used to assign default values to variables and method arguments. @Value annotation is mostly used to get value for specific property keys from the properties file.
@PropertySource Annotation
Spring @PropertySource annotation is used to provide properties files to Spring Environment.
2. Spring MVC Web Annotations
List of Spring MVC annotations tutorials
@Controller Annotation
Spring provides @Controller annotation to make a Java class as a Spring MVC controller. The @Controller annotation indicates that a particular class serves the role of a controller.
@ResponseBody Annotation
The @ResponseBody annotation tells a controller that the object returned is automatically serialized into JSON and passed back into the HttpResponse object.
@RestController Annotation
Spring 4.0 introduced @RestController, a specialized version of the @Controller which is a convenience annotation that does nothing more than add the @Controller and @ResponseBody annotations.
@RequestMapping Annotation
@RequestMapping is the most common and widely used annotation in Spring MVC. It is used to map web requests onto specific handler classes and/or handler methods.
@GetMapping Annotation
The GET HTTP request is used to get single or multiple resources and @GetMapping annotation for mapping HTTP GET requests onto specific handler methods.
@PostMapping Annotation
The POST HTTP method is used to create a resource and @PostMapping annotation for mapping HTTP POST requests onto specific handler methods.
@PutMapping Annotation
The PUT HTTP method is used to update the resource and @PutMapping annotation for mapping HTTP PUT requests onto specific handler methods.
@DeleteMapping Annotation
The DELETE HTTP method is used to delete the resource and @DeleteMapping annotation for mapping HTTP DELETE requests onto specific handler methods.
@PatchMapping Annotation
The PATCH HTTP method is used to update the resource partially and the @PatchMapping annotation is for mapping HTTP PATCH requests onto specific handler methods.
@PathVariable Annotation
Spring boot @PathVariable annotation is used on a method argument to bind it to the value of a URI template variable.
@ResponseStatus Annotation
The @ResponseStatus annotation is a Spring Framework annotation that is used to customize the HTTP response status code returned by a controller method in a Spring MVC or Spring Boot application.
@Service Annotation
@Service annotation is used to create Spring beans at the Service layer.
@Repository Annotation
@Repository is used to create Spring beans for the repositories at the DAO layer.
@Controller is used to create Spring beans at the controller layer.
3. Spring Boot Annotations
List of Spring Boot Annotations tutorials
@SpringBootApplication annotation
The @SpringBootApplication annotation is a core annotation in the Spring Boot framework. It is used to mark the main class of a Spring Boot application.
@EnableAutoConfiguration annotation
@EnableAutoConfiguration annotation enables Spring Boot's auto-configuration feature, which automatically configures the application based on the classpath dependencies and the environment.
@Async Annotation
The @Async annotation can be provided on a method so that invocation of that method will occur asynchronously.
@Scheduled Annotation
The @Scheduled annotation is added to a method along with some information about when to execute it, and Spring takes care of the rest.
@SpringBootTest annotation
Spring Boot provides @SpringBootTest annotation for Integration testing. This annotation creates an application context and loads the full application context.
@WebMvcTest annotation
The @WebMvcTest annotation is used to perform unit tests on Spring MVC controllers. It allows you to test the behavior of controllers, request mappings, and HTTP responses in a controlled and isolated environment.
4. Spring Data JPA Annotations
List of 4. Spring Data JPA Annotations tutorials
@Query Annotation
In Spring Data JPA, the @Query annotation is used to define custom queries. It allows developers to execute both JPQL (Java Persistence Query Language) and native SQL queries.
@DataJpaTest annotation
Spring Boot provides the @DataJpaTest annotation to test the persistence layer components that will autoconfigure the in-memory embedded database for testing purposes.
5. JPA and Hibernate Annotations
List of JPA and Hibernate Annotations tutorials
JPA @Id and @GeneratedValue
@Id: Marks a field as the primary key of an entity. @GeneratedValue: Specifies the strategy for generating primary key values.
JPA @Entity and @Table
@Entity: Specifies that a class is an entity and is mapped to a database table. @Table: Specifies the table name associated with an entity.
@Column Annotation
@Column: Specifies the mapping for a database column.
JPA @Transient Annotation
@Transient: Excludes a field from being persisted in the database.
JPA @OneToOne Annotation
@OneToOne: Defines a one-to-one relationship between two entities.
JPA @OneToMany Annotation
@OneToMany: Defines a one-to-many relationship between two entities.
JPA @ManyToOne Annotation
@ManyToOne: Defines a many-to-one relationship between two entities.
JPA @ManyToMany Annotation
@ManyToMany: Defines a many-to-many relationship between two entities.
JPA @JoinTable Annotation
@JoinTable: The @JoinTable annotation in JPA is used to customize the association table that holds the relationships between two entities in a many-to-many relationship.
Hibernate @PrimaryKeyJoinColumn
The @PrimaryKeyJoinColumn annotation in JPA (Java Persistence API) and Hibernate is used in the context of inheritance relationships and table mappings, particularly in the Joined Strategy.
Hibernate @Embeddable and @Embedded
@Embeddable: This annotation is used to declare a class as an Embeddable class. @Embedded: This annotation is used in the entity class to specify a field that will be embedded.
6. Java and Servlet Annotations
List of Servlet Annotations tutorials
@WebServlet Annotation
The @WebServlet annotation is used to define a Servlet component in a web application.
@WebInitParam Annotation
The @WebInitParam annotation is used to specify any initialization parameters that must be passed to the Servlet or the Filter.
@WebListener Annotation
The @WebListener annotation is used to annotate a listener to get events for various operations on the particular web application context.
@WebFilter Annotation
The @WebFilter annotation is used to define a Filter in a web application.
@MultipartConfig Annotation
The @MultipartConfig annotation, when specified on a Servlet, indicates that the request it expects is of type multipart/form-data.
Java @FunctionalInterface
The @FunctionalInterface annotation indicates that an interface is a functional interface and contains exactly one abstract method.
Post a Comment
Leave Comment
My Top and Bestseller Udemy Courses
- Spring 6 and Spring Boot 3 for Beginners (Includes Projects)
- Building Real-Time REST APIs with Spring Boot
- Building Microservices with Spring Boot and Spring Cloud
- Full-Stack Java Development with Spring Boot 3 & React
- Testing Spring Boot Application with JUnit and Mockito
- Master Spring Data JPA with Hibernate
- Spring Boot Thymeleaf Real-Time Web Application - Blog App
Check out all my Udemy courses and updates: Udemy Courses - Ramesh Fadatare
Copyright © 2018 - 2025 Java Guides All rights reversed | Privacy Policy | Contact | About Me | YouTube | GitHub


Spring Boot Annotations | Beginners guide
Let’s take a look at a list of important spring boot annotations and when to use them with an example.
What are annotations?
Annotations are a form of hints that a developer can give about their program to the compiler and runtime. Based on these hints, the compilers and runtimes can process these programs differently. That is exactly what happens with annotations in spring boot. Spring Boot uses relies on annotations extensively to define and process bean definitions. Some of the features we discuss here are from Spring Framework. But Spring Boot itself is an opinionated version of Spring.
Spring Boot annotation catagories
Based on the use case, Spring annotations fall in to one the following categories.
- Bean definition annotations (from Core Spring Framework)
- Configuration annotations
Spring Component annotations
- Other modules Specific spring-boot annotations
1. Spring Core Annotations
These annotations are not part of spring boot but the spring framework itself. These annotations deal with defining beans. So, Lets look at each of these in details.
You should use the @Bean on a method that returns a bean object. For example, you could define a bean as shown below.
The @Autowired annotation lets you inject a bean wherever you want(most importantly factory methods like the one we seen above). For example, we needed a MyDto for MyService in our previous example. As we had to hardcode in that example, you can simplify it as shown here.
As you see here, @Autowired annotation makes sure spring boot loads an appropriate MyDto bean. Note that, you would get an error if you haven’t defined a MyDto bean. You could work around this by setting @Autowired(required=false) .
This approach provides you a default approach that you can override later.
You could also use the @Autowired annotation for arbitrary number of beans. This is done by setting the annotation at the method level. For instance, take a look at this snippet.
In the above scenario, all three beans must be defined for the myServiceUsingAutowireMultiple factory method to succeed.
Similar to setting required flag in @Autowired , you should use this annotation to mark a certain field or setter method as required. For instance, take a look at this snippet.
2. Spring Boot Configuration annotations
Spring Boot favors java annotations over xml configurations. Even though both of them have pros and cons, the annotations help create complex configurations that work dynamically. So lets go thorough each of these with some examples.
@SpringBootApplication
The @SpringBootApplication annotation is often used in the main class. This annotation is equivalent to using @Configuration , @EnableAutoConfiguration and @ComponentScan together. This annotation is responsible for setting up which autoconfiguration to enable and where to look for spring bean components and configurations.
@Configuration
This annotation is used together with the bean definition annotations. When the @ComponentScan finds a class that is annotated with @Configuration , it will try to process all @Bean and other spring related methods inside it.
Configuration properties
This spring boot specific annotation helps bind properties file entries to a java bean. For example, take a look at these configs.
Let’s map these into a java bean.
If setup correctly, Spring boot will create a AppConfig bean object with values mapped from properties. Subsequently, We can autowire them wherever we want.
@Value annotation
This annotation is similar to @Autowired . Except this one is for accessing properties entries instead of beans.
You could also use @Value to access complex SpEL expressions.
there are other few spring boot annotations for configurations that we would not discuss here.
While @Bean lets you create bean using factory methods, you could simply mark a class with one of the following component annotations. These annotations create a singleton bean of that specific class.
In a way these spring boot annotations provide context to what those beans represent in the system. They are,
@Component annotation
This is the basic version among those four. This annotation on a class makes a singleton bean of that said class. For example, the following creates a bean of type BookService with the name “bookService”.
Once the component scan finds this class, it will try to use the constructor to create a BookService object. As you see here, the constructor needs a Dto bean. So spring boot will look for one that satisfies that requirement.
@Service Spring boot annotation
This annotation is similar to Component in everyway. The only reason to use a @Service annotation instead of @Component is for convention(domain driven design).
@Controller annotation
The @Controller marks a class as a spring web MVC controller. This annotation is usually used along with web based spring applications. Apart from the MVC related features it brings, the rest of the behavior stands in line with @Component .
Once the spring MVC sees a controller class, it looks for all @RequestMapping and configures them to handle requests. We will take a look at these in detail later.
There is also a restful variant of @Controller called @RestController . There is also @GetMapping, @PostMapping and other mapping annotations available part of Spring MVC. They are more specialized versions of @RequestMapping annotations.
@RequestMapping
The request mapping annotation lets spring MVC know which controller class method to call. This annotation takes two parameters called path and method . This way we could map them based on path as well as HTTP methods like , GET, POST, PUT and DETELE.
@ResponseBody
The response body is an optional annotation for controller methods. When supplied, this annotation will convert the method return value in to JSON response.
For example, the above code would return a JSON array of strings.
@Repository annotation in Spring Boot
The repository annotation is a bit different among all other stereotypes annotations. Even though this annotation is used extensively in JPA, this annotation came into picture as part of Domain Driven Design.
A common use case would be to annotate JPA repository interfaces. This way Spring-JPA module can provide JPQL support. For example, We could write a BookDto as shown here.
Even though we don’t need to annotate interfaces that extend, it is still a good convention. Also, apart from being a repository, all behaviours from @Component is applicable for @Repository annotation.
Spring boot Module-specific annotations
As spring boot comes with various modules like JPA, MVC, RabbitMQ, Scheduling, caching etc., all of these modules also provide their own annotations for us to use. We have seen some of the annotations from JPA and MVC. So let’s go through the remaining quickly.
Scheduling related annotations
The @Scheduled annotation helps make a method execute on a specific interval.
This annotation takes one of fixedDelay , fixedRate or quartz compatible cron expression.
Caching annotations
Caching annotations play important role in application performance. The cache abstraction in spring boot is powerful and you should take a look. Here are the important annotations from this module.
The @EnableCaching annotation enables support for caching by auto-configuring a cache manager. Usually this annotation goes on the spring boot’s main class.
@Cacheable annotation marks a method’s return values to be cacheable. This way, when the first time the method was called with a specific parameter, the value is saved in the cache. If another request calls the same method with the same parameter, spring boot will return the value from cache instead of executing the method.
As you can see, it takes a cache name and a key to store the result. This way when a new request comes with the same key, we could get the result from cache instead of running the method again.
The @CacheEvict annotation lets you clear a specific value from the cache. It could also be used to clear the entire cache if needed. This annotation exists because your cached results may be outdated and might need a refresh. For example, if you update an entity in DB, then you might want to clear the cached value as well.
AMQP/RabbitMQ annotations
The AMQP module provides certain annotations to deal with message queues of RabbitMQ. The important annotations are listed here.
The @EnableRabbit adds autoconfiguration for AMQP. This annotation is responsible for enabling RabbitAutoConfiguration . Without this annotation, the remaining AMQP annotations won’t work.
@RabbitListener takes a queue name and executes the annotated method. For example, you could read messages from queues as shown here.
There are also few annotations like @Exchange , @queue and @QueueBinding which are used along with @RabbitListener .
We saw a hand-picked list of important annotations used in spring boot. These annotations solve the problem of defining spring beans, utilize them dynamically and configure them. You can find most of these annotations in the samples available in our Github repository .
- Spring Cache For Better application performance
- Spring Boot Redis Cache
- Handling Lists in Thymeleaf view
- Constructor dependency injection in Spring Framework
Similar Posts
Ways to add servlet filters in spring boot.
In this post, We will take a look at ways to add servlet filters to your spring boot application. Servlet filters help a web application in many ways. They intercept requests and responses to provide different behaviors based on requests. Some of them are, Authentication and Authorization filters that help deal with security. Logging Filters…
Introduction to WebSocket with Spring Boot
Let’s look at how to add WebSocket support to a spring boot application. We will try to create a simple chat application. Note that this implementation does not use STOMP. What is WebSocket? The WebSocket protocol helps establish a full-duplex two-way communication between client and server over a single TCP connection. This protocol is different…
Connection to a database from Spring Boot
Spring Boot lets you make connections to a database of any type with little to no configuration. In this post, we will see how to connect to a MySQL database with spring boot. Step 1: Add a database starter In spring boot, a starter is a special type of dependency that comes with some auto-configuration….
Understanding Lazy Initialization in Spring Boot
Starting with Spring Boot 2.2, spring beans can be lazy. That is, You could create beans as and when required. Let’s see how to implement lazy initialization with Spring Boot. What is lazy initialization? By default, Spring Framework creates and injects beans and it’s dependencies at the time of context creation or refresh. But with…
Spring Boot Email using Thymeleaf with Example
Let us see how to send HTML emails using thymeleaf templates in a spring boot application. Things got easier with spring boot and thymeleaf template engine. As you know, Thymeleaf is used as a View in Spring MVC applications. However, do you know you can use the same template Engine for generating rich HTML emails?…
Spring Boot RabbitMQ – Complete Guide For Beginners
RabbitMQ is a message broker that receives and forwards messages. In this post, You will learn how to use RabbitMQ with spring boot. Also, you will learn how to publish to and subscribe from queues and exchanges. Setting up RabbitMQ in local machine You can download the RabbitMQ installer from the official download page. However,…
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Notify me via e-mail if anyone answers my comment.
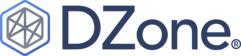
- Manage Email Subscriptions
- How to Post to DZone
- Article Submission Guidelines
- Manage My Drafts
Modern API Management : Dive into APIs’ growing influence across domains, prevalent paradigms, microservices, the role AI plays, and more.
Where and how does low code fit into the engineering experience? Share your opinions and help shape DZone's June report (+ enter a raffle!).
Programming in Python: Dive into the Python ecosystem to learn about popular libraries, tools, modules, and more.
Vector databases: Learn all about the specialized VDBMS — its initial setup, data preparation, collection creation, data querying, and more.
- An Introduction to Microservices With Undertow
- Testcontainers With Kotlin and Spring Data R2DBC
- Distributed Tracing System (Spring Cloud Sleuth + OpenZipkin)
- Java, Spring Boot, and MongoDB: Performance Analysis and Improvements
- Why and How To Integrate Elastic APM in Apache JMeter
- Participate in DZone Research Surveys: You Can Shape Trend Reports! (+ Enter the Raffles)
- Cypress vs. React Testing Library
- Optimizing AWS Costs Through Advanced Machine Learning Solutions
A Guide to Spring Framework Annotations
Here's the lowdown on just about every spring framework annotation, including core, spring cloud, spring mvc, spring rest, and spring boot..
Join the DZone community and get the full member experience.
The Java programming language provided support for annotations from Java 5.0 onward. Leading Java frameworks were quick to adopt annotations, and the Spring Framework started using annotations from the 2.5 release. Due to the way they are defined, annotations provide a lot of context in their declaration.
Prior to annotations, the behavior of the Spring Framework was largely controlled through XML configuration. Today, the use of annotations provide us tremendous capabilities in how we configure the behaviors of the Spring Framework.
In this post, we’ll take a look at the annotations available in the Spring Framework.
Core Spring Framework Annotations
This annotation is applied to bean setter methods. Consider a scenario where you need to enforce a required property. The @Required annotation indicates that the affected bean must be populated at configuration time with the required property. Otherwise, an exception of type BeanInitializationException is thrown.
This annotation is applied to fields, setter methods, and constructors. The @Autowired annotation injects object dependency implicitly.
When you use @Autowired on fields and pass the values for the fields using the property name, Spring will automatically assign the fields with the passed values.
You can even use @Autowired on private properties, as shown below. (This is a very poor practice though!)
When you use @Autowired on setter methods, Spring tries to perform it by Type autowiring on the method. You are instructing Spring that it should initiate this property using a setter method where you can add your custom code, like initializing any other property with this property.
Consider a scenario where you need an instance of class A, but you do not store A in the field of the class. You just use A to obtain an instance of B, and you are storing B in this field. In this case, setter method autowiring will better suit you. You will not have class-level unused fields.
When you use @Autowired on a constructor, then constructor injection happens at the time of object creation. It tells the constructor to autowire when used as a bean. One thing to note here is that only one constructor of any bean class can carry the @Autowired annotation.
NOTE: As of Spring 4.3, @Autowired became optional on classes with a single constructor. In the above example, Spring would still inject an instance of the Person class if you omitted the @Autowired annotation.
This annotation is used along with the @Autowired annotation. When you need more control of the dependency injection process, @Qualifier can be used. @Qualifier can be specified on individual constructor arguments or method parameters. This annotation is used to avoid the confusion that occurs when you create more than one bean of the same type and want to wire only one of them with a property.
Consider an example where an interface BeanInterface is implemented by two beans, BeanB1 and BeanB2.
Now, if BeanA autowires this interface, Spring will not know which one of the two implementations to inject.
One solution to this problem is the use of the @Qualifier annotation.
With the @Qualifier annotation added, Spring will now know which bean to autowire, where beanB2 is the name of BeanB2.
@Configuration
This annotation is used on classes that define beans. @Configuration is an analog for an XML configuration file – it is configured using Java classes. A Java class annotated with @Configuration is a configuration by itself and will have methods to instantiate and configure the dependencies.
Here is an example:
@ComponentScan
This annotation is used with the @Configuration annotation to allow Spring to know the packages to scan for annotated components. @ComponentScan is also used to specify base packages using basePackageClasses or basePackage attributes to scan. If specific packages are not defined, scanning will occur from the package of the class that declares this annotation.
This annotation is used at the method level. The @Bean annotation works with @Configuration to create Spring beans. As mentioned earlier, @Configuration will have methods to instantiate and configure dependencies. Such methods will be annotated with @Bean . The method annotated with this annotation works as the bean ID, and it creates and returns the actual bean.
This annotation is used on component classes. By default, all autowired dependencies are created and configured at startup. But if you want to initialize a bean lazily, you can use the @Lazy annotation over the class. This means that the bean will be created and initialized only when it is first requested for. You can also use this annotation on @Configuration classes. This indicates that all @Bean methods within that @Configuration should be lazily initialized.
This annotation is used at the field, constructor parameter, and method parameter levels. The @Value annotation indicates a default value expression for the field or parameter to initialize the property with. As the @Autowired annotation tells Spring to inject an object into another when it loads your application context, you can also use the @Value annotation to inject values from a property file into a bean’s attribute. It supports both #{...} and ${...} placeholders.
Spring Framework Stereotype Annotations
This annotation is used on classes to indicate a Spring component. The @Component annotation marks the Java class as a bean or component so that the component-scanning mechanism of Spring can add it into the application context.
@Controller
The @Controller annotation is used to indicate the class is a Spring controller. This annotation can be used to identify controllers for Spring MVC or Spring WebFlux.
This annotation is used on a class. @Service marks a Java class that performs some service, such as executing business logic, performing calculations, and calling external APIs. This annotation is a specialized form of the @Component annotation intended to be used in the service layer.
@Repository
This annotation is used on Java classes that directly access the database. The @Repository annotation works as a marker for any class that fulfills the role of repository or Data Access Object.
This annotation has an automatic translation feature. For example, when an exception occurs in the @Repository , there is a handler for that exception and there is no need to add a try-catch block.
Spring Boot Annotations
@enableautoconfiguration.
This annotation is usually placed on the main application class. The @EnableAutoConfiguration annotation implicitly defines a base “search package”. This annotation tells Spring Boot to start adding beans based on classpath settings, other beans, and various property settings.
@SpringBootApplication
This annotation is used on the application class while setting up a Spring Boot project. The class that is annotated with the @SpringBootApplication must be kept in the base package. The one thing that the @SpringBootApplication does is a component scan. But it will scan only its sub-packages. As an example, if you put the class annotated with @SpringBootApplication in the com.example, then @SpringBootApplication will scan all its sub-packages, such as com.example.a, com.example.b, and com.example.a.x.
The @SpringBootApplication is a convenient annotation that adds all the following:
- @Configuration
- @EnableAutoConfiguration
- @ComponentScan
Spring MVC and REST Annotations
This annotation is used on Java classes that play the role of controller in your application. The @Controller annotation allows autodetection of component classes in the classpath and auto-registering bean definitions for them. To enable autodetection of such annotated controllers, you can add component scanning to your configuration. The Java class annotated with @Controller is capable of handling multiple request mappings.
This annotation can be used with Spring MVC and Spring WebFlux.
@RequestMapping
This annotation is used at both the class and method level. The @RequestMapping annotation is used to map web requests onto specific handler classes and handler methods. When @RequestMapping is used on the class level, it creates a base URI for which the controller will be used. When this annotation is used on methods, it will give you the URI on which the handler methods will be executed. From this, you can infer that the class level request mapping will remain the same whereas each handler method will have their own request mapping.
Sometimes you may want to perform different operations based on the HTTP method used, even though the request URI may remain the same. In such situations, you can use the method attribute of @RequestMapping with an HTTP method value to narrow down the HTTP methods in order to invoke the methods of your class.
Here is a basic example of how a controller along with request mappings work:
In this example, only GET requests to /welcome is handled by the welcomeAll() method.
This annotation also can be used with Spring MVC and Spring WebFlux.
The @RequestMapping annotation is very versatile. Please see my in-depth post on Request Mapping here .
@CookieValue
This annotation is used at the method parameter level. @CookieValue is used as an argument of a request mapping method. The HTTP cookie is bound to the @CookieValue parameter for a given cookie name. This annotation is used in the method annotated with @RequestMapping . Let us consider that the following cookie value is received with an HTTP request:
JSESSIONID=418AB76CD83EF94U85YD34W
To get the value of the cookie, use @CookieValue like this:
@CrossOrigin
This annotation is used both at the class and method levels to enable cross-origin requests. In many cases, the host that serves JavaScript will be different from the host that serves the data. In such a case, Cross-Origin Resource Sharing (CORS) enables cross-domain communication. To enable this communication, you just need to add the @CrossOrigin annotation.
By default, the @CrossOrigin annotation allows all origin, all headers, the HTTP methods specified in the @RequestMapping annotation, and a maxAge of 30 min. You can customize the behavior by specifying the corresponding attribute values.
An example of using @CrossOrigin at both the controller and handler method levels is below:
In this example, both the getExample() and getNote() methods will have a maxAge of 3600 seconds. Also, getExample() will only allow cross-origin requests from http://example.com, while getNote() will allow cross-origin requests from all hosts.
Composed @RequestMapping Variants
Spring Framework 4.3 introduced the following method-level variants of @RequestMapping annotation to better express the semantics of the annotated methods. Using these annotations has become the standard ays of defining the endpoints. They act as wrappers to @RequestMapping .
These annotations can be used with Spring MVC and Spring WebFlux.
@GetMapping
This annotation is used for mapping HTTP GET requests onto specific handler methods. @GetMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.GET) .
@PostMapping
This annotation is used for mapping HTTP POST requests onto specific handler methods. @PostMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.POST) .
@PutMapping
This annotation is used for mapping HTTP PUT requests onto specific handler methods. @PutMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PUT) .
@PatchMapping
This annotation is used for mapping HTTP PATCH requests onto specific handler methods. @PatchMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.PATCH) .
@DeleteMapping
This annotation is used for mapping HTTP DELETE requests onto specific handler methods. @DeleteMapping is a composed annotation that acts as a shortcut for @RequestMapping(method = RequestMethod.DELETE) .
@ExceptionHandler
This annotation is used at method levels to handle exceptions at the controller level. The @ExceptionHandler annotation is used to define the class of exception it will catch. You can use this annotation on methods that should be invoked to handle an exception. The @ExceptionHandler values can be set to an array of Exception types. If an exception is thrown that matches one of the types in the list, then the method annotated with the matching @ExceptionHandler will be invoked.
@InitBinder
This annotation is a method-level annotation that plays the role of identifying the methods that initialize the WebDataBinder — a DataBinder that binds the request parameter to JavaBean objects. To customize request parameter data binding, you can use @InitBinder annotated methods within our controller. The methods annotated with @InitBinder includes all argument types that handler methods support.
The @InitBinder annotated methods will get called for each HTTP request if you don’t specify the value element of this annotation. The value element can be a single or multiple form names or request parameters that the init binder method is applied to.
@Mappings and @Mapping
This annotation is used on fields. The @Mapping annotation is a meta-annotation that indicates a web mapping annotation. When mapping different field names, you need to configure the source field to its target field, and to do that, you have to add the @Mappings annotation. This annotation accepts an array of @Mapping having the source and the target fields.
@MatrixVariable
This annotation is used to annotate request handler method arguments so that Spring can inject the relevant bits of a matrix URI. Matrix variables can appear on any segment each separated by a semicolon. If a URL contains matrix variables, the request mapping pattern must represent them with a URI template. The @MatrixVariable annotation ensures that the request is matched with the correct matrix variables of the URI.
@PathVariable
This annotation is used to annotate request handler method arguments. The @RequestMapping annotation can be used to handle dynamic changes in the URI where a certain URI value acts as a parameter. You can specify this parameter using a regular expression. The @PathVariable annotation can be used to declare this parameter.
@RequestAttribute
This annotation is used to bind the request attribute to a handler method parameter. Spring retrieves the named attribute's value to populate the parameter annotated with @RequestAttribute . While the @RequestParamannotation is used to bind the parameter values from a query string, @RequestAttribute is used to access the objects that have been populated on the server-side.
@RequestBody
This annotation is used to annotate request handler method arguments. The @RequestBody annotation indicates that a method parameter should be bound to the value of the HTTP request body. The HttpMessageConveter is responsible for converting from the HTTP request message to object.
@RequestHeader
This annotation is used to annotate request handler method arguments. The @RequestHeader annotation is used to map the controller parameter to request header value. When Spring maps the request, @RequestHeader checks the header with the name specified within the annotation and binds its value to the handler method parameter. This annotation helps you to get the header details within the controller class.
@RequestParam
This annotation is used to annotate request handler method arguments. Sometimes you get the parameters in the request URL, mostly in GET requests. In that case, along with the @RequestMapping annotation, you can use the @RequestParam annotation to retrieve the URL parameter and map it to the method argument. The @RequestParam annotation is used to bind request parameters to a method parameter in your controller.
@RequestPart
This annotation is used to annotate request handler method arguments. The @RequestPart annotation can be used instead of @RequestParam to get the content of a specific multipart and bind it to the method argument annotated with @RequestPart . This annotation takes into consideration the “Content-Type” header in the multipart (request part).
@ResponseBody
This annotation is used to annotate request handler methods. The @ResponseBody annotation is similar to the @RequestBody annotation. The @ResponseBody annotation indicates that the result type should be written straight in the response body in whatever format you specify like JSON or XML. Spring converts the returned object into a response body by using the HttpMessageConveter .
@ResponseStatus
This annotation is used on methods and exception classes. @ResponseStatus marks a method or exception class with a status code and a reason that must be returned. When the handler method is invoked the status code is set to the HTTP response which overrides the status information provided by any other means. A controller class can also be annotated with @ResponseStatus , which is then inherited by all @RequestMapping methods.
@ControllerAdvice
This annotation is applied at the class level. As explained earlier, for each controller, you can use @ExceptionHandler on a method that will be called when a given exception occurs. But this handles only those exceptions that occur within the controller in which it is defined. To overcome this problem, you can now use the @ControllerAdvice annotation. This annotation is used to define @ExceptionHandler , @InitBinder , and @ModelAttribute methods that apply to all @RequestMapping methods. Thus, if you define the @ExceptionHandler annotation on a method in a @ControllerAdvice class, it will be applied to all the controllers.
@RestController
This annotation is used at the class level. The @RestController annotation marks the class as a controller where every method returns a domain object instead of a view. By annotating a class with this annotation, you no longer need to add @ResponseBody to all the RequestMapping methods. It means that you no long use view-resolvers or send HTML in response. You just send the domain object as an HTTP response in the format that is understood by the consumers, like JSON.
@RestController is a convenience annotation that combines @Controller and @ResponseBody .
@RestControllerAdvice
This annotation is applied to Java classes. @RestControllerAdvice is a convenience annotation that combines @ControllerAdvice and @ResponseBody . This annotation is used along with the @ExceptionHandler annotation to handle exceptions that occur within the controller.
@SessionAttribute
This annotation is used at the method parameter level. The @SessionAttribute annotation is used to bind the method parameter to a session attribute. This annotation provides convenient access to the existing or permanent session attributes.
@SessionAttributes
This annotation is applied at the type level for a specific handler. The @SessionAtrributes annotation is used when you want to add a JavaBean object into a session. This is used when you want to keep the object in session for short-lived. @SessionAttributes is used in conjunction with @ModelAttribute .
Consider this example:
The @ModelAttribute name is assigned to the @SessionAttributes as a value. The @SessionAttributes has two elements. The value element is the name of the session in the model and the types element is the type of session attributes in the model.
Spring Cloud Annotations
@enableconfigserver.
This annotation is used at the class level. When developing a project with a number of services, you need to have a centralized and straightforward manner to configure and retrieve the configurations of all the services that you are going to develop. One advantage of using a centralized config server is that you don’t need to carry the burden of remembering where each configuration is distributed across multiple and distributed components.
You can use Spring Cloud’s @EnableConfigServer annotation to start a config server that the other applications can talk to.
@EnableEurekaServer
This annotation is applied to Java classes. One problem that you may encounter while decomposing your application into microservices is that it becomes difficult for every service to know the address of every other service it depends on. There comes the discovery service which is responsible for tracking the locations of all other microservices.
Netflix’s Eureka is an implementation of a discovery server and integration is provided by Spring Boot. Spring Boot has made it easy to design a Eureka Server by just annotating the entry class with @EnableEurekaServer .
@EnableDiscoveryClient
This annotation is applied to Java classes. In order to tell any application to register itself with Eureka, you just need to add the @EnableDiscoveryClient annotation to the application entry point. The application that’s now registered with Eureka uses the Spring Cloud Discovery Client abstraction to interrogate the registry for its own host and port.
@EnableCircuitBreaker
This annotation is applied to Java classes that can act as the circuit breaker. The circuit breaker pattern can allow a microservice to continue working when a related service fails, preventing the failure from cascading. This also gives the failed service a time to recover.
The class annotated with @EnableCircuitBreaker will monitor, open, and close the circuit breaker.
@HystrixCommand
This annotation is used at the method level. Netflix’s Hystrix library provides the implementation of a Circuit Breaker pattern. When you apply the circuit breaker to a method, Hystrix watches for the failures of the method. Once failures build up to a threshold, Hystrix opens the circuit so that the subsequent calls also fail. Now, Hystrix redirects calls to the method, and they are passed to the specified fallback methods.
Hystrix looks for any method annotated with the @HystrixCommand annotation and wraps it into a proxy connected to a circuit breaker so that Hystrix can monitor it.
Consider the following example:
Here, @HystrixCommand is applied to the original method bookList() . The @HystrixCommand annotation has newList as the fallback method. So, for some reason, if Hystrix opens the circuit on bookList() , you will have a placeholder book list ready for the users.
Spring Framework DataAccess Annotations
@transactional.
This annotation is placed before an interface definition, a method on an interface, a class definition, or a public method on a class. The mere presence of @Transactional is not enough to activate the transactional behavior. The @Transactional is simply metadata that can be consumed by some runtime infrastructure. This infrastructure uses the metadata to configure the appropriate beans with transactional behavior.
The annotation further supports configuration like:
- The Propagation type of the transaction
- The Isolation level of the transaction
- A timeout for the operation wrapped by the transaction
- A read-only flag — a hint for the persistence provider that the transaction must be read onlyThe rollback rules for the transaction
Cache-Based Annotations
This annotation is used on methods. The simplest way of enabling the cache behavior for a method is to annotate it with @Cacheable and parameterize it with the name of the cache where the results would be stored.
In the snippet above, the method getAddress is associated with the cache named addresses. Each time the method is called, the cache is checked to see whether the invocation has been already executed and does not have to be repeated.
This annotation is used on methods. Whenever you need to update the cache without interfering the method execution, you can use the @CachePut annotation. That is, the method will always be executed and the result cached.
Using @CachePut and @Cacheable on the same method is strongly discouraged, as the former forces the execution in order to execute a cache update, the latter causes the method execution to be skipped by using the cache.
@CacheEvict
This annotation is used on methods. It is not that you always want to populate the cache with more and more data. Sometimes, you may want to remove some cache data so that you can populate the cache with some fresh values. In such a case, use the @CacheEvict annotation.
Here, an additional element, allEntries , is used along with the cache name to be emptied. It is set to true so that it clears all values and prepares to hold new data.
@CacheConfig
This annotation is a class-level annotation. The @CacheConfig annotation helps to streamline some of the cache information at one place. Placing this annotation on a class does not turn on any caching operation. This allows you to store the cache configuration at the class level so that you don’t have to declare things multiple times.
Task Execution and Scheduling Annotations
This annotation is a method-level annotation. The @Scheduled annotation is used on methods along with the trigger metadata. A method with @Scheduled should have a void return type and should not accept any parameters.
There are different ways of using the @Scheduled annotation:
In this case, the duration between the end of the last execution and the start of the next execution is fixed. The tasks always wait until the previous one is finished.
In this case, the beginning of the task execution does not wait for the completion of the previous execution.
The task gets executed initially with a delay and then continues with the specified fixed rate.
This annotation is used on methods to execute each method in a separate thread. The @Async annotation is provided on a method so that the invocation of that method will occur asynchronously. Unlike methods annotated with @Scheduled , the methods annotated with @Async can take arguments. They will be invoked in the normal way by callers at runtime rather than by a scheduled task.
@Async can be used with both void return type methods and methods that return a value. However, methods with return values must have a Future-typed return value.
Spring Framework Testing Annotations
@bootstrapwith.
This annotation is a class-level annotation. The @BootstrapWith annotation is used to configure how the Spring TestContext Framework is bootstrapped. This annotation is used as metadata to create custom composed annotations and reduce the configuration duplication in a test suite.
@ContextConfiguration
This annotation is a class-level annotation that defines metadata used to determine which configuration files to use to the load the ApplicationContext for your test. More specifically, @ContextConfiguration declares the annotated classes that will be used to load the context. You can also tell Spring where to locate the file.
@WebAppConfiguration
This annotation is a class-level annotation. The @WebAppConfiguration is used to declare that the ApplicationContext loaded for an integration test should be a WebApplicationContext . This annotation is used to create the web version of the application context. It is important to note that this annotation must be used with the @ContextConfiguration annotation. The default path to the root of the web application is src/main/webapp . You can override it by passing a different path to the <span class="theme:classic lang:default decode:true crayon-inline">@WebAppConfiguration.
This annotation is used on methods. The @Timed annotation indicates that the annotated test method must finish its execution at the specified time period (in milliseconds). If the execution exceeds the specified time in the annotation, the test fails.
In this example, the test will fail if it exceeds 10 seconds of execution.
This annotation is used on test methods. If you want to run a test method several times in a row automatically, you can use the @Repeat annotation. The number of times that the test method is to be executed is specified in the annotation.
In this example, the test will be executed 10 times.
This annotation can be used as both class-level or method-level annotation. After the execution of a test method, the transaction of the transactional test method can be committed using the @Commit annotation. This annotation explicitly conveys the intent of the code. When used at the class level, this annotation defines the commit for all test methods within the class. When declared as a method-level annotation, @Commit specifies the commit for specific test methods overriding the class level commit.
This annotation can be used as both class-level and method-level annotation. The @RollBack annotation indicates whether the transaction of a transactional test method must be rolled back after the test completes its execution. If this true, @Rollback(true) , the transaction is rolled back. Otherwise, the transaction is committed. @Commit is used instead of @RollBack(false) .
When used at the class level, this annotation defines the rollback for all test methods within the class.
When declared as a method level annotation, @RollBack specifies the rollback for specific test methods overriding the class level rollback semantics.
@DirtiesContext
This annotation is used as both class-level and method-level annotation. @DirtiesContext indicates that the Spring ApplicationContext has been modified or corrupted in some manner and it should be closed. This will trigger the context reloading before execution of next test. The ApplicationContext is marked as dirty before or after any such annotated method as well as before or after current test class.
The @DirtiesContext annotation supports BEFORE_METHOD , BEFORE_CLASS , and BEFORE_EACH_TEST_METHOD modes for closing the ApplicationContext before a test.
NOTE : Avoid overusing this annotation. It is an expensive operation and if abused, it can really slow down your test suite.
@BeforeTransaction
This annotation is used to annotate void methods in the test class. @BeforeTransaction annotated methods indicate that they should be executed before any transaction starts executing. That means the method annotated with @BeforeTransaction must be executed before any method annotated with @Transactional .
@AfterTransaction
This annotation is used to annotate void methods in the test class. @AfterTransaction annotated methods indicate that they should be executed after a transaction ends for test methods. That means the method annotated with @AfterTransaction must be executed after the method annotated with @Transactional .
This annotation can be declared on a test class or test method to run SQL scripts against a database. The @Sql annotation configures the resource path to SQL scripts that should be executed against a given database either before or after an integration test method. When @Sql is used at the method level, it will override any @Sqldefined in at class level.
This annotation is used along with the @Sql annotation. The @SqlConfig annotation defines the metadata that is used to determine how to parse and execute SQL scripts configured via the @Sql annotation. When used at the class level, this annotation serves as global configuration for all SQL scripts within the test class. But when used directly with the config attribute of @Sql , @SqlConfig serves as a local configuration for SQL scripts declared.
This annotation is used on methods. The @SqlGroup annotation is a container annotation that can hold several @Sql annotations. This annotation can declare nested @Sql annotations. In addition, @SqlGroup is used as a meta-annotation to create custom composed annotations. This annotation can also be used along with repeatable annotations, where @Sql can be declared several times on the same method or class.
@SpringBootTest
This annotation is used to start the Spring context for integration tests. This will bring up the full autoconfigruation context.
@DataJpaTest
The @DataJpaTest annotation will only provide the autoconfiguration required to test Spring Data JPA using an in-memory database such as H2.
This annotation is used instead of @SpringBootTest.
@DataMongoTest
The @DataMongoTest will provide a minimal autoconfiguration and an embedded MongoDB for running integration tests with Spring Data MongoDB.
@WebMVCTest
The @WebMVCTest will bring up a mock servlet context for testing the MVC layer. Services and components are not loaded into the context. To provide these dependencies for testing, the @MockBean annotation is typically used.
@AutoConfigureMockMVC
The @AutoConfigureMockMVC annotation works very similarly to the @WebMVCTest annotation, but the full Spring Boot context is started.
Creates and injects a Mockito Mock for the given dependency.
Will limit the auto-configuration of Spring Boot to components relevant to processing JSON.
This annotation will also autoconfigure an instance of JacksonTester or GsonTester .
@TestPropertySource
Class-level annotation used to specify property sources for the test class.
Published at DZone with permission of John Thompson , DZone MVB . See the original article here.
Opinions expressed by DZone contributors are their own.
Partner Resources
- About DZone
- Send feedback
- Community research
- Advertise with DZone
CONTRIBUTE ON DZONE
- Become a Contributor
- Core Program
- Visit the Writers' Zone
- Terms of Service
- Privacy Policy
- 3343 Perimeter Hill Drive
- Nashville, TN 37211
- [email protected]
Let's be friends:
- Java Tutorial
- Java Spring
- Spring Interview Questions
- Java SpringBoot
- Spring Boot Interview Questions
- Spring MVC Interview Questions
- Java Hibernate
- Hibernate Interview Questions
- Advance Java Projects
- Java Interview Questions
- Spring Boot Tutorial
- Introduction to Spring Boot
- Best Way to Master Spring Boot – A Complete Roadmap
- How to Create a Spring Boot Project?
- Spring Boot - Annotations
- Spring Boot - Architecture
- Spring Boot Actuator
- Spring Boot - Introduction to RESTful Web Services
- How to create a basic application in Java Spring Boot
- How to create a REST API using Java Spring Boot
- Easiest Way to Create REST API using Spring Boot
- Java Spring Boot Microservices Sample Project
- Difference between Spring MVC and Spring Boot
- Spring Boot - Spring JDBC vs Spring Data JDBC
- Best Practices For Structuring Spring Boot Application
- Spring Boot - Start/Stop a Kafka Listener Dynamically
- How To Dockerize A Spring Boot Application With Maven ?
- Dynamic Dropdown From Database using Spring Boot
- Spring - RestTemplate
- Spring Boot - Scheduling
- Spring Boot - Sending Email via SMTP
- Different Ways to Establish Communication Between Spring Microservices
- JSON using Jackson in REST API Implementation with Spring Boot
- How to encrypt passwords in a Spring Boot project using Jasypt
- Containerizing Spring Boot Application
Spring Boot – Annotations
Spring Boot Annotations are a form of metadata that provides data about a spring application. Spring Boot is built on the top of the spring and contains all the features of spring. And is becoming a favorite of developers these days because of its rapid production-ready environment which enables the developers to directly focus on the logic instead of struggling with the configuration and setup. Spring Boot is a microservice-based framework and making a production-ready application in it takes very little time. Following are some of the features of Spring Boot:
- It allows for avoiding heavy configuration of XML which is present in the spring
- It provides easy maintenance and creation of REST endpoints
- It includes embedded Tomcat-server
- Deployment is very easy, war and jar files can be easily deployed in the Tomcat server
Annotations are used to provide supplemental information about a program. It does not have a direct effect on the operation of the code they annotate. It does not change the action of the compiled program. So in this article, we are going to discuss some important Spring Boot Annotations that are available in Spring Boot with examples.
Spring Boot Annotations
Spring annotations are present in the org.springframework.boot.autoconfigure and org.springframework.boot.autoconfigure.condition packages are commonly known as Spring Boot annotations.
Spring Boot Annotations List
Some of the annotations that are available in this category are:
- @SpringBootApplication
- @SpringBootConfiguration
- @EnableAutoConfiguration
- @ComponentScan
- @ConditionalOnClass, and @ConditionalOnMissingClass
- @ConditionalOnBean, and @ConditionalOnMissingBean
- @ConditionalOnProperty
- @ConditionalOnResource
- @ConditionalOnWebApplication and @ConditionalOnNotWebApplication
- @ConditionalExpression
- @Conditional
1. @SpringBootApplication Annotation
This annotation is used to mark the main class of a Spring Boot application. It encapsulates @SpringBootConfiguration , @EnableAutoConfiguration , and @ComponentScan annotations with their default attributes.
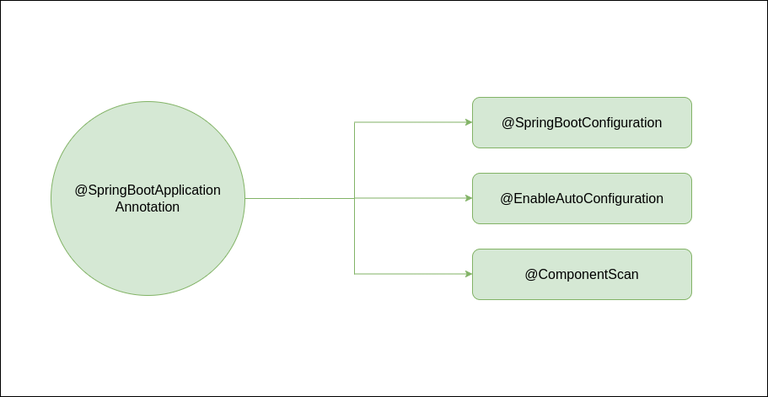
2. @SpringBootConfiguration Annotation
It is a class-level annotation that is part of the Spring Boot framework. It implies that a class provides Spring Boot application configuration. It can be used as an alternative to Spring’s standard @Configuration annotation so that configuration can be found automatically. Most Spring Boot Applications use @SpringBootConfiguration via @SpringBootApplication. If an application uses @SpringBootApplication, it is already using @SpringBootConfiguration.
3. @EnableAutoConfiguration Annotation
This annotation auto-configures the beans that are present in the classpath. It simplifies the developer’s work by assuming the required beans from the classpath and configure it to run the application. This annotation is part of the spring boot framework. For example, when we illustrate the spring-boot-starter-web dependency in the classpath, Spring boot auto-configures Tomcat , and Spring MVC . The package of the class declaring the @EnableAutoConfiguration annotation is considered as the default. Therefore, we need to apply the @EnableAutoConfiguration annotation in the root package so that every sub-packages and class can be examined.
4. @ComponentScan Annotation
@ComponentScan tells Spring in which packages you have annotated classes that should be managed by Spring. So, for example, if you have a class annotated with @Controller which is in a package that is not scanned by Spring, you will not be able to use it as a Spring controller. So we can say @ComponentScan enables Spring to scan for things like configurations, controllers, services, and other components that are defined. Generally, @ComponentScan annotation is used with @Configuration annotation to specify the package for Spring to scan for components.
5. @ConditionalOnClass Annotation and @ConditionalOnMissingClass Annotation
@ConditionalOnClass Annotation used to mark auto-configuration bean if the class in the annotation’s argument is present/absent.
6. @ConditionalOnBean Annotation and @ConditionalOnMissingBean Annotation
These annotations are used to let a bean be included based on the presence or absence of specific beans.
7. @ConditionalOnProperty Annotation
These annotations are used to let configuration be included based on the presence and value of a Spring Environment property.
8. @ConditionalOnResource Annotation
These annotations are used to let configuration be included only when a specific resource is present in the classpath.
9. @ConditionalOnExpression Annotation
These annotations are used to let configuration be included based on the result of a SpEL (Spring Expression Language) expression.
SpEL (Spring Expression Language): It is a powerful expression language that supports querying and manipulating an object graph at runtime.
10. @ConditionalOnCloudPlatform Annotation
These annotations are used to let configuration be included when the specified cloud platform is active.
Request Handling and Controller annotations:
Some important annotations comes under this category are:
- @Controller
- @RestController
- @RequestMapping
- @RequestParam
- @PathVariable
- @RequestBody
- @ResponseBody
- @ModelAttribute
1. @Controller Annotation
This annotation provides Spring MVC features. It is used to create Controller classes and simultaneously it handles the HTTP requests. Generally we use @Controller annotation with @RequestMapping annotation to map HTTP requests with methods inside a controller class.
2. @RestController Annotation
This annotation is used to handle REST APIs such as GET, PUT, POST, DELETE etc. and also used to create RESTful web services using Spring MVC.
It encapsulates @Controller annotation and @ResponseBody annotation with their default attributes.
@RestController = @Controller + @ResponseBody
3. @RequestMapping Annotation
This annotation is used to map the HTTP requests with the handler methods inside the controller class.
For handling specific HTTP requests we can use
- @GetMapping
- @PutMapping
- @PostMapping
- @PatchMapping
- @DeleteMapping
NOTE : We can manually use GET, POST, PUT and DELETE annotations along with the path as well as we can use @RequestMapping annotation along with the method for all the above handler requests
4. @RequestParam Annotation
This annotation is basically used to obtain a parameter from URI. In other words, we can say that @RequestParam annotation is used to read the form data and binds the web request parameter to a specific controller method.
5. @PathVariable Annotation
This annotation is used to extract the data from the URI path. It binds the URL template path variable with method variable.
6. @RequestBody Annotation
This annotation is used to convert HTTP requests from incoming JSON format to domain objects directly from request body. Here method parameter binds with the body of the HTTP request.
7. @ResponseBody Annotation
This annotation is used to convert the domain object into HTTP request in the form of JSON or any other text. Here, the return type of the method binds with the HTTP response body.
8. @ModelAttribute Annotation
This annotation refers to model object in Spring MVC. It can be used on methods or method arguments as well.
Here, we don’t need to add object explicitly to the model, because automatically object will be the part of the attribute.
Please Login to comment...
Similar reads.
- Java-Annotation
- Java-Spring-Boot
- Advance Java
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JavaTechOnline
Making java easy to learn, spring boot annotations with examples.
- Spring Boot
Last Updated on December 24th, 2023

Here in this article on ‘Spring Boot Annotations With Examples’, we will discuss about all annotations that we use in a Spring Boot Application. Annotations which are not part of this article, will be included in other respective articles. Link will be provided here only for easy navigation. In addition, you can also check one more article ‘ Annotations in Java ‘ in order to know annotation basics and how to create custom annotations in Java. Let’s start discussing our topic ‘Spring Boot Annotations With Examples’.
Table of Contents
Annotation Basics
Before discussing about ‘Spring Boot Annotations With Examples’, let’s first talk about some basic terminologies used during the explanation of annotations.
What is The IoC container?
In a nutshell, the IoC is a container that injects dependencies while creating the bean. IoC stands for ‘Inversion Of Control’. Instead of creating objects by the developer, the bean itself controls the instantiation or association of its dependencies by using direct construction of classes with the help of the IoC container. Hence, this process is known as ‘Inversion of control’. Sometimes we also call it Spring Container in short.
The org.springframework.beans and org.springframework.context packages are the basis for Spring Framework’s IoC container . ApplicationContext is a sub-interface of BeanFactory.
What is an Application Context in Spring Framework?
When you create a project in Spring or Spring Boot, a container or wrapper gets created to manage your beans. This is nothing but Application Context. However Spring supports two containers : Bean Factory and Application Context. In short, the BeanFactory provides the configuration framework and basic functionality. On the other hand, ApplicationContext adds more enterprise-specific functionality including easier integration with Spring’s AOP features; message resource handling (for use in internationalization), event publication; and application-layer specific contexts such as the WebApplicationContext for use in web applications. The ApplicationContext is a complete superset of the BeanFactory and is used exclusively in this topic in descriptions of Spring’s IoC container . Spring Framework recommends to use Application Context to get the full features of the framework. Moreover, Dependency injection and auto-wiring of beans is done in Application Context.
The interface org.springframework.context.ApplicationContext represents the Spring IoC container and it manages the process of instantiating, configuring, and assembling the beans. The container gets its instructions on what objects to instantiate, configure, and assemble by reading configuration metadata. The configuration metadata can be represented in three formats: XML, Java annotations, or Java code. Since this is out of our topic ‘Spring Boot Annotations With Examples’, we have discussed about this in detail in a separate article ‘ Spring Core Tutorial ‘.
What is Spring Bean or Components?
During Application startup, Spring instantiates objects and adds them to the Application Context(IoC container). These objects in the Application Context are called ‘Spring Beans’ or ‘Spring Components’. As they are managed by Spring, therefore we also call them Spring-managed Bean or Spring-managed Component.
What is Component Scanning?
The process of discovering classes that can contribute to the Application Context, is called Component Scanning. During Component Scanning, if Spring finds a class annotated with a particular annotation, It will consider this class as a candidate for Spring Bean/Component and adds it to the Application Context. Spring explicitly provides a way to identify Spring bean candidates via the @ComponentScan annotation.
When to use Component Scanning in a Spring Boot Application?
By default, the @ComponentScan annotation will scan for components in the current package and its all sub-packages. If it is a Spring Boot application, then all the packages under the package containing the Main class (a class annotated with @SpringBootApplication ) will be covered by an implicit component scan. So if your package doesn’t come under the hierarchy of the package containing the Main class, then there is a need for explicit component scanning.
Spring Annotations vs Spring Boot Annotations
As we know that Spring Boot Framework is created using libraries of the Spring Framework and removed the XML configuration approach. However, all the Spring Framework’s annotations are still applicable for Spring Boot. Furthermore, Spring Boot has provided some additional annotations which are specific to Spring Boot only. In some cases, Spring Boot created annotations after adding more than one annotations of the Spring Framework. Here, we will learn most commonly used annotations under our topic of discussion ‘Spring Boot Annotations With Examples’, whether it is a part of Spring Framework or Spring Boot.
Annotations that Supports to create a Spring Bean
Let’s start discussing our topic ‘Spring Boot Annotations with Examples’ with the annotations to create a Bean. Needless to say, we can’t work on Spring Boot/Spring framework without creating a Bean. Now you can imagine how important is that!
Next annotations in our topic ‘Spring Boot Annotations With Examples’ are the stereotype annotations.
@Component
@Complonent annotation is also an important & most widely used at the class level. This is a generic stereotype annotation which indicates that the class is a Spring-managed bean/component. @Component is a class level annotation. Other stereotypes are a specialization of @Component . During the component scanning, Spring Framework automatically discovers the classes annotated with @Componen t, It registers them into the Application Context as a Spring Bean. Applying @Component annotation on a class means that we are marking the class to work as Spring-managed bean/component. For example, observe the code below:
On writing a class like above, Spring will create a bean instance with name ‘myBean’. Please keep in mind that, By default, the bean instances of this class have the same name as the class name with a lowercase initial. However, we can explicitly specify a different name using the optional argument of this annotation like below.
@Controller
@Controller tells Spring Framework that the class annotated with @Controller will work as a controller in the Spring MVC project.
@RestControlle r
@RestController tells Spring Framework that the class annotated with @RestController will work as a controller in a Spring REST project.
@Service tells Spring Framework that the class annotated with @Service is a part of service layer and it will include business logics of the application.
@Repository
@Repository tells Spring Framework that the class annotated with @Repos itory is a part of data access layer and it will include logics of accessing data from the database in the application.
Apart from Stereotype annotations, we have two annotations that are generally used together: @Configuration & @Bean.
@Configuration
We apply this annotation on classes. When we apply this to a class, that class will act as a configuration by itself. Generally the class annotated with @Configuration has bean definitions as an alternative to <bean/> tag of an XML configuration. It also represents a configuration using Java class. Moreover the class will have methods to instantiate and configure the dependencies. For example :
♥ The benefit of creating an object via this method is that you will have only one instance of it. You don’t need to create the object multiple times when required. Now you can call it anywhere in your code.
This is the basic annotation and important for our topic ‘Spring Boot Annotations With Examples’.
We use @Bean at method level. If you remember the xml configuration of a Spring, It is a direct analog of the XML <bean/> element. It creates Spring beans and generally used with @Configuration . As aforementioned, a class with @Configuration (we can call it as a Configuration class) will have methods to instantiate objects and configure dependencies. Such methods will have @Bean annotation.
By default, the bean name will be the same as the method name. It instantiates and returns the actual bean. The annotated method produces a bean managed by the Spring IoC container. For example:
For the sake of comparison, the configuration above is exactly equivalent to the following Spring XML:
The annotation supports most of the attributes offered by <bean/>, such as: init-method , destroy-method , autowiring , lazy-init , dependency-check , depends-on and scope .
@Bean vs @Component
@ Component is a class level annotation whereas @ Bean is a method level annotation and name of the method serves as the bean name. @ Bean annotation has to be used within the class and that class should be annotated with @ Configuration . However, @Component needs not to be used with the @Configuration . @Component auto detects and configures the beans using classpath scanning, whereas @Bean explicitly declares a single bean, rather than letting Spring do it automatically.
Configuration Annotations
Next annotations in our article ‘Spring Boot Annotations with Examples’ are for Configurations. Since Spring Framework is healthy in configurations, we can’t avoid learning annotations on configurations. No doubt, they save us from complex coding effort.
@ComponentScan
Spring container detects Spring managed components with the help of @ComponentScan . Once you use this annotation, you tell the Spring container where to look for Spring components. When a Spring application starts, Spring container needs the information to locate and register all the Spring components with the application context. However It can auto scan all classes annotated with the stereotype annotations such as @Component, @Controller, @Service , and @Repository from pre-defined project packages.
The @ComponentScan annotation is used with the @Configuration annotation to ask Spring the packages to scan for annotated components. @ComponentScan is also used to specify base packages and base package classes using basePackages or basePackageClasses attributes of @ComponentScan . For example :
Here the @ComponentScan annotation uses the basePackages attribute to specify three packages including their subpackages that will be scanned by the Spring container. Moreover the annotation also uses the basePackageClasses attribute to declare the Bean1 class, whose package Spring Boot will scan.
Moreover, In a Spring Boot project, we typically apply the @SpringBootApplication annotation on the main application class. Internally, @SpringBootApplication is a combination of the @Configuration, @ComponentScan, and @EnableAutoConfiguration annotations. Further, with this default setting, Spring Boot will auto scan for components in the current package (containing the SpringBoot main class) and its sub packages.
Suppose we have multiple Java configuration classes annotated by @Configuration . @Import imports one or more Java configuration classes. Moreover, it has the capability to group multiple configuration classes. We use this annotation where one @Configuration class logically imports the bean definitions defined by another. For example:
@PropertySource
If you create a Spring Boot Starter project using an IDE such as STS , application.properties comes under resources folder by default. In contrast, You can provide the name & location of the properties file (containing the key/value pair) as per your convenience by using @PropertySource . Moreover, this annotation provides a convenient and declarative mechanism for adding a PropertySource to Spring’s Environment. For example,
@PropertySources (For Multiple Property Locations)
Of course, If we have multiple property locations in our project, we can also use the @PropertySources annotation and specify an array of @PropertySource .
However, we can also write the same code in another way as below. The @PropertySource annotation is repeatable according to Java 8 conventions. Therefore, if we’re using Java 8 or higher, we can use this annotation to define multiple property locations. For example:
♥ Note : If there is any conflict in names such as the same name of the properties, the last source read will always take precedence.
We can use this annotation to inject values into fields of Spring managed beans. We can apply it at field or constructor or method parameter level. For example, let’s first define a property file, then inject values of properties using @Value.
Now inject the value of server.ip using @Value as below:
@Value for default Value
Suppose we have not defined a property in the properties file. In that case we can provide a default value for that property. Here is the example:
Here, the value Admin will be injected for the property emp.department . However, if we have defined the property in the properties file, the value of property file will override it.
♥ Note : If the same property is defined as a system property and also in the properties file, then the system property would take preference.
@Value for multiple values
Sometimes, we need to inject multiple values of a single property. We can conveniently define them as comma-separated values for the single property in the properties file. Further, we can easily inject them into property that is in the form of an array. For example:
Spring Boot Specific Annotations
Now it’s time to extend our topic ‘Spring Boot Annotations with Examples’ with Spring Boot Specific Annotations. They are discovered by Spring Boot Framework itself. However, most of them internally use annotations provided by Spring Framework and extend them further.
@SpringBootApplication (@Configuration + @ComponentScan + @EnableAutoConfiguration)
Everyone who worked on Spring Boot must have used this annotation either intentionally or unintentionally. When we create a Spring Boot Starter project using an IDE like STS, we receive this annotation as a gift. This annotation applies at the main class which has main () method. The Main class serves two purposes in a Spring Boot application: configuration and bootstrapping .
In fact @SpringBootApplication is a combination of three annotations with their default values. They are @Configuration, @ComponentScan, and @EnableAutoConfiguration. Therefore, we can also say that @SpringBootApplication is a 3-in-1 annotation. This is an important annotation in the context of our topic ‘Spring Boot Annotations With Examples’.
@EnableAutoConfiguration : enables the auto-configuration feature of Spring Boot. @ComponentScan : enables @Component scan on the package to discover and register components as beans in Spring’s application Context. @Configuration : allows to register extra beans in the context or imports additional configuration classes.
We can also use above three annotations separately in place of @SpringBootApplication if we want any customized behavior of them.
@EnableAutoConfiguration
@EnableAutoConfiguration enables auto-configuration of beans present in the classpath in Spring Boot applications. In a nutshell, this annotation enables Spring Boot to auto-configure the application context. Therefore, it automatically creates and registers beans that are part of the included jar file in the classpath and also the beans defined by us in the application. For example, while creating a Spring Boot starter project when we select Spring Web and Spring Security dependency in our classpath, Spring Boot auto-configures Tomcat, Spring MVC and Spring Security for us.
Moreover, Spring Boot considers the package of the class declaring the @EnableAutoConfiguration as the default package. Therefore, if we apply this annotation in the root package of the application, every sub-packages & classes will be scanned. As a result, we won’t need to explicitly declare the package names using @ComponentScan .
Furthermore, @EnableAutoConfiguration provides us two attributes to manually exclude classes from auto-configurations. If we don’t want some classes to be auto-configured, we can use exclude attribute to disable them. Another attribute is excludeName to declare a fully qualified list of classes to exclude. For example, below are the codes.
Use of ‘exclude’ in @EnableAutoConfiguration
Use of ‘excludename’ in @enableautoconfiguration , @springbootconfiguration.
This annotation is a part of Spring Boot Framework. However, @SpringBootApplication inherits from it. Therefore, If an application uses @SpringBootApplication , it is already using @SpringBootConfiguration . Moreover, It acts as an alternative to the @Configuration annotation. The primary difference is that @SpringBootConfiguration allows configuration to be automatically discovered. @SpringBootConfiguration indicates that the class provides configuration and also applied at the class level . Particularly, this is useful in case of unit or integration tests. For example, observe the below code:
@ConfigurationProperties
Spring Framework provides various ways to inject values from the properties file. One of them is by using @Value annotation. Another one is by using @ConfigurationProperties on a configuration bean to inject properties values to a bean. But what is the difference among both ways and what are the benefits of using @ConfigurationProperties , you will understand it at the end. Now Let’s see how to use @ConfigurationProperties annotation to inject properties values from the application.properties or any other properties file of your own choice.
First, let’s define some properties in our application.properties file as follows. Let’s assume that we are defining some properties of our development working environment. Therefore, representing properties name with prefix ‘dev’.
Now, create a bean class with getter and setter methods and annotate it with @ConfigurationProperties.
Here, Spring will automatically bind any property defined in our property file that has the prefix ‘ dev’ and the same name as one of the fields in the MyDevAppProperties class.
Next, register the @ConfigurationProperties bean in your @Configuration class using the @EnableConfigurationProperties annotation.
Finally create a Test Runner to test the values of properties as below.
We can also use the @ConfigurationProperties annotation on @Bean -annotated methods.
@EnableConfigurationProperties
In order to use a configuration class in our project, we need to register it as a regular Spring bean. In this situation @EnableConfigurationProperties annotation support us. We use this annotation to register our configuration bean (a @ConfigurationProperties annotated class) in a Spring context . This is a convenient way to quickly register @ConfigurationProperties annotated beans. Moreover, It is strictly coupled with @ConfiguratonProperties . For example, you can refer @ConfigurationProperties from the previous section.
@EnableConfigurationPropertiesScan
@EnableConfigurationPropertiesScan annotation scans the packages based on the parameter value passed into it and discovers all classes annotated with @ConfiguratonProperties under the package . For example, observe the below code:
From the above example, @EnableConfigurationPropertiesScan will scan all the @ConfiguratonProperties annotated classes under the package “com.dev.spring.test.annotation” and register them accordingly.
@EntityScan and @EnableJpaRepositories
Spring Boot annotations like @ComponentScan , @ConfigurationPropertiesScan and even @SpringBootApplication use packages to define scanning locations. Similarly @EnityScan and @EnableJpaRepositories also use packages to define scanning locations. Here, we use @EntityScan for discovering entity classes, whereas @EnableJpaRepositories for JPA repository classes by convention . These annotations are generally used when your discoverable classes are not under the root package or its sub-packages of your main application.
Remember that, @EnableAutoConfiguration( as part of @SpringBootApplication) scans all the classes under the root package or its sub-packages of your main application. Therefore, If the repository classes or other entity classes are not placed under the main application package or its sub package, then the relevant package(s) should be declared in the main application configuration class with @EntityScan and @EnableJpaRepositories annotation accordingly. For example, observe the below code:
Links to Other Annotations
As stated in the introduction section of our article ‘Spring Boot Annotations With Examples’, we will discuss all annotations that we generally use in a Spring Boot web Application. Below are the links to continue with ‘Spring boot Annotations with Examples’:
1) Spring Boot Bean Annotations With Examples
2) spring boot mvc & rest annotations with examples, 3) spring boot security annotations with examples, 4) spring boot scheduling annotations with examples, 5) spring boot transactions annotations with examples, 6) spring boot errors, exceptions and aop annotations with examples, 6) spring cloud annotations with examples, where can we use annotations.
Apart from learning ‘Spring Boot Annotations With Examples’, its also important to know what are places to apply annotations.
We can apply annotations to the declarations of different elements of a program such as to the declarations of classes, fields, methods, and other program elements. By convention, we apply them just before the declaration of the element. Moreover, as of the Java 8 release, we can apply annotations to the use of types. For example, below code snippets demonstrate the usage of annotations:
1) Class instance creation expression
2) type cast, 3) implements clause, 4) thrown exception declaration.
This form of annotation is called a type annotation. For more information, see Type Annotations and Pluggable Type Systems .
However, these annotations are not in tradition at present, but in the future we can see them in the code. Since we are discussing about ‘Spring Boot Annotations With Examples’, we need to at least have the basic idea of them.

- Spring Boot
- Interview Q
Spring Boot Tutorial
Creating project, project components, spring boot aop, spring boot database, spring boot view, spring boot misc, spring boot - restful, spring tutorial, spring cloud, spring microservices.
Interview Questions
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Part 1: Spring Annotations
This tutorial is part 1 of 5-part tutorial on JEE annotations. We recommend that you read Prerequisite section first, review the abstract and Example Application to understand the context. You can also jump to other parts by clicking on the links below.
Spring Annotations: Contents:
@repository.

@Transactional
- Propagation setting is PROPAGATION_REQUIRED.
- Isolation level is ISOLATION_DEFAULT.
- Transaction is read/write.
- Transaction timeout defaults to the default timeout of the underlying transaction system, or to none if timeouts are not supported.
- Any RuntimeException triggers rollback, and any checked Exception does not.
Spring MVC Annotations
@controller, @requestmapping, @pathvariable, @requestparam, @modelattribute, @sessionattributes.
- @SessionAttribute is initialized when you put the corresponding attribute into model (either explicitly or using @ModelAttribute-annotated method).
- @SessionAttribute is updated by the data from HTTP parameters when controller method with the corresponding model attribute in its signature is invoked.
- @SessionAttributes are cleared when you call setComplete() on SessionStatus object passed into controller method as an argument.
Spring Security Annotations
@preauthorize, references:.
- Spring Annotations : http://static.springsource.org/spring/docs/current/spring-framework-reference/html/beans.html#beans-annotation-config
- Spring Framework Reference : http://static.springsource.org/spring/docs/current/spring-framework-reference/pdf/spring-framework-reference.pdf

IMAGES
VIDEO
COMMENTS
The @Bean is a method-level annotation used to declare a spring bean. When the container executes the annotated method, it registers the return value as a bean within a BeanFactory. By default, the bean name will be the same as the method name. To customize the bean name, use its' name ' or ' value ' attribute. 2.2.
Commonly used Spring Boot annotations along with their uses and examples. 1). @SpringBootApplication: This annotation is used to bootstrap a Spring Boot application. It combines three annotations: @Configuration, @EnableAutoConfiguration, and @ComponentScan. Example: 2). @RestController: This annotation is used to indicate that a class is a ...
Spring Annotations. Spring framework implements and promotes the principle of control inversion (IOC) or dependency injection (DI) and is in fact an IOC container. Traditionally, Spring allows a developer to manage bean dependencies by using XML-based configuration. There is an alternative way to define beans and their dependencies.
frameworks quickly adopt the annotation's concept. Spring Boot has provided the support for the annotations since the release of Spring 2.5. Due to their extensive structure and internal concepts, the annotations provide a lot of context in their declaration. Before the annotations, the Spring Boot project's behavior was controlled and
The Java Programming language provided support for Annotations from Java 5.0. Leading Java frameworks were quick to adopt annotations and the Spring Framework started using annotations from the release 2.5. Due to the way they are defined, annotations provide a lot of context in their declaration. Prior to annotations, the behavior of the Spring Framework
Spring @Value annotation is used to assign default values to variables and method arguments. We can read spring environment variables as well as system variables using @Value annotation. We can use @Value for injecting property values into beans. It's compatible with the constructor, setter, and field injection.
Spring Framework Annotations. Spring framework is one of the most popular Java EE frameworks. It is an open-source lightweight framework that allows Java EE 7 developers to build simple, reliable, and scalable enterprise applications. This framework mainly focuses on providing various ways to help you manage your business objects.
Here are the most important annotations any Java developer working with Spring should know: @Configuration - used to mark a class as a source of the bean definitions. Beans are the components of the system that you want to wire together. A method marked with the @Bean annotation is a bean producer. Spring will handle the life cycle of the beans ...
It means that Spring Boot looks for auto-configuration beans on its classpath and automatically applies them. Note, that we have to use this annotation with @Configuration: 4. Auto-Configuration Conditions. Usually, when we write our custom auto-configurations, we want Spring to use them conditionally.
This tutorial explains the JSR-250 annotations that Spring 2.5 introduces, which include @Resource, @PostConstruct, and @PreDestroy annotations. @RestController. @ RestController annotation is inherited from the @ Controller annotation. This is the special version of @ Controller annotation for implementing the RESTful Web Services.
It provides defaults for code and annotation configuration to quick start new Spring projects within no time. Let's list all the annotations from these packages. 1. @SpringBootApplication. @SpringBootApplication annotation indicates a configuration class that declares one or more @Bean methods and also triggers auto-configuration and component ...
This article is part of a series: 1. Overview. We can leverage the capabilities of Spring DI engine using the annotations in the org.springframework.beans.factory.annotation and org.springframework.context.annotation packages. We often call these "Spring core annotations" and we'll review them in this tutorial. 2.
Spring Annotations. This article is part of a series: 1. Introduction. Spring Data provides an abstraction over data storage technologies. Therefore, our business logic code can be much more independent of the underlying persistence implementation. Also, Spring simplifies the handling of implementation-dependent details of data storage.
The @WebMvcTest annotation is used to perform unit tests on Spring MVC controllers. It allows you to test the behavior of controllers, request mappings, and HTTP responses in a controlled and isolated environment. 4. Spring Data JPA Annotations. List of 4.
Spring boot Module-specific annotations. As spring boot comes with various modules like JPA, MVC, RabbitMQ, Scheduling, caching etc., all of these modules also provide their own annotations for us to use. We have seen some of the annotations from JPA and MVC. So let's go through the remaining quickly.
Leading Java frameworks were quick to adopt annotations, and the Spring Framework started using annotations from the 2.5 release. Due to the way they are defined, annotations provide a lot of ...
Spring Boot Annotations are a form of metadata that provides data about a spring application. Spring Boot is built on the top of the spring and contains all the features of spring. And is becoming a favorite of developers these days because of its rapid production-ready environment which enables the developers to directly focus on the logic instead of struggling with the configuration and setup.
They are @Configuration, @ComponentScan, and @EnableAutoConfiguration. Therefore, we can also say that @SpringBootApplication is a 3-in-1 annotation. This is an important annotation in the context of our topic 'Spring Boot Annotations With Examples'. @EnableAutoConfiguration: enables the auto-configuration feature of Spring Boot.
Spring Boot Annotations @EnableAutoConfiguration: It auto-configures the bean that is present in the classpath and configures it to run the methods. The use of this annotation is reduced in Spring Boot 1.2.0 release because developers provided an alternative of the annotation, i.e. @SpringBootApplication. @SpringBootApplication: It is a combination of three annotations @EnableAutoConfiguration ...
This section covers annotations that you can use when you test Spring applications. Section Summary. Standard Annotation Support; Spring Testing Annotations; Spring JUnit 4 Testing Annotations; Spring JUnit Jupiter Testing Annotations; Meta-Annotation Support for Testing; Appendix Standard Annotation Support. Spring Framework. 6.2.0-SNAPSHOT
For spring to process annotations, add the following lines in your application-context.xml file. <context:annotation-config />. <context:component-scan base-package="...specify your package name..." />. Spring supports both Annotation based and XML based configurations. You can even mix them together.
This article is part of a series: 1. Overview. In this tutorial, we'll explore Spring Web annotations from the org.springframework.web.bind.annotation package. 2. @RequestMapping. Simply put, @RequestMapping marks request handler methods inside @Controller classes; it can be configured using: path, or its aliases, name, and value: which URL ...
A single @SpringBootApplication annotation can be used to enable those three features, that is: @EnableAutoConfiguration: enable Spring Boot's auto-configuration mechanism. @ComponentScan: enable @Component scan on the package where the application is located (see the best practices) @SpringBootConfiguration: enable registration of extra ...