Get full access to The Complete Coding Interview Guide in Java and 60K+ other titles, with a free 10-day trial of O'Reilly.
There are also live events, courses curated by job role, and more.
- The Complete Coding Interview Guide in Java
Read it now on the O’Reilly learning platform with a 10-day free trial.
O’Reilly members get unlimited access to books, live events, courses curated by job role, and more from O’Reilly and nearly 200 top publishers.

Book description
Explore a wide variety of popular interview questions and learn various techniques for breaking down tricky bits of code and algorithms into manageable chunks
Key Features
- Discover over 200 coding interview problems and their solutions to help you secure a job as a Java developer
- Work on overcoming coding challenges faced in a wide array of topics such as time complexity, OOP, and recursion
- Get to grips with the nuances of writing good code with the help of step-by-step coding solutions
Book Description
Java is one of the most sought-after programming languages in the job market, but cracking the coding interview in this challenging economy might not be easy. This comprehensive guide will help you to tackle various challenges faced in a coding job interview and avoid common interview mistakes, and will ultimately guide you toward landing your job as a Java developer.
This book contains two crucial elements of coding interviews - a brief section that will take you through non-technical interview questions, while the more comprehensive part covers over 200 coding interview problems along with their hands-on solutions. This book will help you to develop skills in data structures and algorithms, which technical interviewers look for in a candidate, by solving various problems based on these topics covering a wide range of concepts such as arrays, strings, maps, linked lists, sorting, and searching. You'll find out how to approach a coding interview problem in a structured way that produces faster results. Toward the final chapters, you'll learn to solve tricky questions about concurrency, functional programming, and system scalability.
By the end of this book, you'll have learned how to solve Java coding problems commonly used in interviews, and will have developed the confidence to secure your Java-centric dream job.
What you will learn
- Solve the most popular Java coding problems efficiently
- Tackle challenging algorithms that will help you develop robust and fast logic
- Practice answering commonly asked non-technical interview questions that can make the difference between a pass and a fail
- Get an overall picture of prospective employers' expectations from a Java developer
- Solve various concurrent programming, functional programming, and unit testing problems
- Who this book is for
This book is for students, programmers, and employees who want to be invited to and pass interviews given by top companies. The book assumes high school mathematics and basic programming knowledge.
Table of contents
- Why subscribe?
- Contributors
- About the author
- About the reviewer
- Packt is searching for authors like you
- What this book covers
- To get the most out of this book
- Download the example code files
- Download the color images
- Conventions used
- Get in touch
- Section 1: The Non-Technical Part of an Interview
- The novice interview roadmap
- Know yourself
- Know the market
- Start something
- It’s time to shine online
- What resume screeners are looking for
- How long the resume should be
- How to list your employment history
- List the most relevant projects (top five)
- Nominate your technical skills
- LinkedIn resume
- Finding companies that are hiring
- Submitting the resume
- The phone screening stage
- Going to in-person interviews
- Avoiding common mistakes
- Interviews at Google
- Interviews at Amazon
- Interviews at Microsoft
- Interviews at Facebook
- Interviews at Crossover
- What is the purpose of non-technical questions?
- What is your experience?
- What is your favorite programming language?
- What do you want to do?
- What are your career goals?
- What's your working style?
- Why are you looking to change jobs?
- What is your salary history?
- Why should we hire you?
- How much money do you want to make?
- Do you have a question for me?
- Accepting or rejecting an offer
- Failure is an option
- Getting feedback after the interview
- Objectively identifying and eliminating the mismatches
- Don’t form an obsession for a company
- Don’t lose confidence in yourself – sometimes, they don’t deserve you!
- Technical quiz
- The problems specific to coding challenges are meant to be difficult
- Tackling a coding challenge problem
- Section 2: Concepts
- Technical requirements
- What is an object?
- What is a class?
- What is abstraction?
- What is encapsulation?
- What is inheritance?
- What is polymorphism?
- What is association?
- What is aggregation?
- What is composition?
- What is method overriding in OOP (Java)?
- What is method overloading in OOP (Java)?
- What is covariant method overriding in Java?
- What are the main restrictions in terms of working with exceptions in overriding and overloading methods?
- How can the superclass overridden method be called from the subclass overriding method?
- Can we override or overload the main() method?
- Can we override a non-static method as static in Java?
- What are the main differences between interfaces with default methods and abstract classes?
- What is the main difference between abstract classes and interfaces?
- Can we have an abstract class without an abstract method?
- Can we have a class that is both abstract and final at the same time?
- What is the difference between polymorphism, overriding, and overloading?
- What are the main differences between static and dynamic binding?
- What is method hiding in Java?
- Can we write virtual methods in Java?
- What is the difference between polymorphism and abstraction?
- Do you consider overloading an approach for implementing polymorphism?
- Which OOP concept serves the Decorator design pattern?
- When should the Singleton design pattern be used?
- What is the difference between the Strategy and State design patterns?
- What is the difference between the Proxy and Decorator patterns?
- What is the difference between the Facade and Decorator patterns?
- What is the key difference between the Builder and Factory patterns?
- What is the key difference between the Adapter and Bridge patterns?
- Example 1: Jukebox
- Example 2: Vending machine
- Example 3: Deck of cards
- Example 4: Parking lot
- Example 5: Online reader system
- Example 6: Hash table
- Example 7: File system
- Example 8: Tuple
- Example 9: Cinema with a movie ticket booking system
- Big O complexity time
- The best case, worst case, and expected case
- Example 1 – O(1)
- Example 2 – O(n), linear time algorithms
- Example 3 – O(n), dropping the constants
- Example 6 – different steps are summed or multiplied
- Example 7 – log n runtimes
- Example 9 – in-order traversal of a binary tree
- Example 10 – n may vary
- Example 11 – memoization
- Example 13 – identifying O(1) loops
- Example 14 – looping half of the array
- Example 15 – reducing Big O expressions
- Example 16 – looping with O(log n)
- Example 17 – string comparison
- Example 18 – factorial Big O
- Example 19 – using n notation with caution
- Example 21 – the number of iteration counts in Big O
- Example 22 – digits
- Example 23 – sorting
- Key hints to look for in an interview
- Recognizing a recursive problem
- Memoization (or Top-Down Dynamic Programming)
- Tabulation (or Bottom-Up Dynamic Programming)
- Coding challenge 1 – Robot grid (I)
- Coding challenge 3 – Josephus
- Coding challenge 6 – Five towers
- Coding challenge 8 – The falling ball
- Coding challenge 9 – The highest colored tower
- Coding challenge 10 – String permutations
- Coding challenge 11 – Knight tour
- Coding challenge 12 – Curly braces
- Coding challenge 13 – Staircase
- Coding challenge 14 – Subset sum
- Coding challenge 15 – Word break (this is a famous Google problem)
- Obtaining the binary representation of a Java integer
- Bitwise operators
- Bit shift operators
- Tips and tricks
- Coding challenge 1 – Getting the bit value
- Coding challenge 2 – Setting the bit value
- Coding challenge 3 – Clearing bits
- Coding challenge 4 – Summing binaries on paper
- Coding challenge 5 – Summing binaries in code
- Coding challenge 6 – Multiplying binaries on paper
- Coding challenge 7 – Multiplying binaries in code
- Coding challenge 8 – Subtracting binaries on paper
- Coding challenge 9 – Subtracting binaries in code
- Coding challenge 10 – Dividing binaries on paper
- Coding challenge 11 – Dividing binaries in code
- Coding challenge 12 – Replacing bits
- Coding challenge 13 – Longest sequence of 1
- Coding challenge 14 – Next and previous numbers
- Coding challenge 15 – Conversion
- Coding challenge 16 – Maximizing expressions
- Coding challenge 17 – Swapping odd and even bits
- Coding challenge 18 – Rotating bits
- Coding challenge 19 – Calculating numbers
- Coding challenge 20 – Unique elements
- Coding challenge 21 – Finding duplicates
- Coding challenge 22 – Two non-repeating elements
- Coding challenge 23 – Power set of a set
- Coding challenge 24 – Finding the position of the only set bit
- Coding challenge 25 – Converting a float into binary and vice versa
- Section 3: Algorithms and Data Structures
- Arrays and strings in a nutshell
- Coding challenge 1 – Unique characters (1)
- Coding challenge 2 – Unique characters (2)
- Coding challenge 3 – Encoding strings
- Coding challenge 4 – One edit away
- Coding challenge 5 – Shrinking a string
- Coding challenge 6 – Extracting integers
- Coding challenge 7 – Extracting the code points of surrogate pairs
- Coding challenge 8 – Is rotation
- Coding challenge 9 – Rotating a matrix by 90 degrees
- Coding challenge 10 – Matrix containing zeros
- Coding challenge 11 – Implementing three stacks with one array
- Coding challenge 12 – Pairs
- Coding challenge 13 – Merging sorted arrays
- Coding challenge 14 – Median
- Coding challenge 15 – Sub-matrix of one
- Coding challenge 16 – Container with the most water
- Coding challenge 17 – Searching in a circularly sorted array
- Coding challenge 18 – Merging intervals
- Coding challenge 19 – Petrol bunks circular tour
- Coding challenge 20 – Trapping rainwater
- Coding challenge 21 – Buying and selling stock
- Coding challenge 22 – Longest sequence
- Coding challenge 23 – Counting game score
- Coding challenge 24 – Checking for duplicates
- Coding challenge 25 – Longest distinct substring
- Coding challenge 26 – Replacing elements with ranks
- Coding challenge 27 – Distinct elements in every sub-array
- Coding challenge 28 – Rotating the array k times
- Coding challenge 29 – Distinct absolute values in sorted arrays
- Linked lists in a nutshell
- Maps in a nutshell
- Coding challenge 1 – Map put, get, and remove
- Coding challenge 2 – Map the key set and values
- Coding challenge 3 – Nuts and bolts
- Coding challenge 4 – Remove duplicates
- Coding challenge 5 – Rearranging linked lists
- Coding challenge 6 – The nth to last node
- Coding challenge 7 – Loop start detection
- Coding challenge 8 – Palindromes
- Coding challenge 9 – Sum two linked lists
- Coding challenge 10 – Linked lists intersection
- Coding challenge 11 – Swap adjacent nodes
- Coding challenge 12 – Merge two sorted linked lists
- Coding challenge 13 – Remove the redundant path
- Coding challenge 14 – Move the last node to the front
- Coding challenge 15 – Reverse a singly linked list in groups of k
- Coding challenge 16 – Reverse a doubly linked list
- Coding challenge 17 – LRU cache
- Stacks in a nutshell
- Queues in a nutshell
- Coding challenge 1 – Reverse string
- Coding challenge 2 – Stack of curly braces
- Coding challenge 3 – Stack of plates
- Coding challenge 4 – Stock span
- Coding challenge 5 – Stack min
- Coding challenge 6 – Queue via stacks
- Coding challenge 7 – Stack via queues
- Coding challenge 8 – Max histogram area
- Coding challenge 9 – Smallest number
- Coding challenge 10 – Islands
- Coding challenge 11 – Shortest path
- Infix, postfix, and prefix expressions
- General tree
- Binary Search Tree
- Balanced and unbalanced binary trees
- Complete binary tree
- Full binary tree
- Perfect binary tree
- Binary Heaps
- Adjacency matrix
- Adjacency list
- Graph traversal
- Coding challenge 1 – Paths between two nodes
- Coding challenge 2 – Sorted array to minimal BST
- Coding challenge 3 – List per level
- Coding challenge 4 – sub-tree
- Coding challenge 5 – Landing reservation system
- Coding challenge 6 – Balanced binary tree
- Coding challenge 7 – Binary tree is a BST
- Coding challenge 8 – Successor node
- Coding challenge 9 – Topological sort
- Coding challenge 10 – Common ancestor
- Coding challenge 11 – Chess knight
- Coding challenge 12 – Printing binary tree corners
- Coding challenge 13 – Max path sum
- Coding challenge 14 – Diagonal traversal
- Coding challenge 15 – Handling duplicates in BSTs
- Coding challenge 16 – Isomorphism of binary trees
- Coding challenge 17 – Binary tree right view
- Coding challenge 18 – kth largest element
- Coding challenge 19 – Mirror binary tree
- Coding challenge 20 – Spiral-level order traversal of a binary tree
- Coding challenge 21 – Nodes at a distance k from leafs
- Coding challenge 22 – Pair for a given sum
- Coding challenge 23 – Vertical sums in a binary tree
- Coding challenge 23 – Converting a max heap into a min heap
- Coding challenge 24 – Finding out whether a binary tree is symmetric
- Coding challenge 25 – Connecting n ropes at the minimum cost
- Advanced topics
- Bucket Sort
- Searching algorithms
- Coding challenge 1 – Merging two sorted arrays
- Coding challenge 2 – Grouping anagrams together
- Coding challenge 3 – List of unknown size
- Coding challenge 4 – Merge sorting a linked list
- Coding challenge 5 – Strings interspersed with empty strings
- Coding challenge 6 – Sorting a queue with the help of another queue
- Coding challenge 7 – Sorting a queue without extra space
- Coding challenge 8 – Sorting a stack with the help of another stack
- Coding challenge 9 – Sorting a stack in place
- Coding challenge 10 – Searching in a full sorted matrix
- Coding challenge 11 – Searching in a sorted matrix
- Coding challenge 12 – First position of first one
- Coding challenge 13 – Maximum difference between two elements
- Coding challenge 14 – Stream ranking
- Coding challenge 15 – Peaks and valleys
- Coding challenge 16 – Nearest left smaller number
- Coding challenge 17 – Word search
- Coding challenge 18 – Sorting an array based on another array
- Tips and suggestions
- Coding challenge 1 – FizzBuzz
- Coding challenge 2 – Roman numerals
- Coding challenge 3 – Visiting and toggling 100 doors
- Coding challenge 4 – 8 teams
- Coding challenge 5 – Finding the kth number with the prime factors 3, 5, and 7
- Coding challenge 6 – Count decoding a digit's sequence
- Coding challenge 7 – ABCD
- Coding challenge 8 – Rectangles overlapping
- Coding challenge 9 – Multiplying large numbers
- Coding challenge 10 – Next greatest number with the same digits
- Coding challenge 11 – A number divisible by its digits
- Coding challenge 12 – Breaking chocolate
- Coding challenge 13 – Clock angle
- Coding challenge 14 – Pythagorean triplets
- Coding challenge 15 – Scheduling one elevator
- Section 4: Bonus – Concurrency and Functional Programming
- Technical Requirements
- Java concurrency (multithreading) in a nutshell
- Coding challenge 1 – Thread life cycle states
- Coding challenge 2 – Deadlocks
- Coding challenge 3 – Race conditions
- Coding challenge 5 – Executor and ExecutorService
- Coding challenge 7 – Starvation
- Coding challenge 10 – Thread versus Runnable
- Coding challenge 12 – wait() versus sleep()
- Coding challenge 14 – ThreadLocal
- Coding challenge 15 – submit() versus execute()
- Coding challenge 16 – interrupted() and isInterrupted()
- Coding challenge 18 – sharing data between threads
- Coding challenge 20 – Producer-Consumer
- Producer-Consumer via wait() and notify()
- Key concepts of functional-style programming
- Coding challenge 1 – Lambda parts
- Coding challenge 2 – Functional interface
- Coding challenge 3 – Collections versus streams
- Coding challenge 4 – The map() function
- Coding challenge 5 – The flatMap() function
- Coding challenge 6 – map() versus flatMap()
- Coding challenge 7 – The filter() function
- Coding challenge 8 – Intermediate versus terminal operations
- Coding challenge 9 – The peek() function
- Coding challenge 10 – Lazy streams
- Coding challenge 11 – Functional interfaces versus regular interfaces
- Coding challenge 12 – Supplier versus Consumer
- Coding challenge 13 – Predicates
- Coding challenge 14 – findFirst() versus findAny()
- Coding challenge 15 – Converting arrays to streams
- Coding challenge 16 – Parallel streams
- Coding challenge 17 – The method reference
- Coding challenge 18 – The default method
- Coding challenge 19 – Iterator versus Spliterator
- Coding challenge 20 – Optional
- Coding challenge 21 – String::valueOf
- Unit testing in a nutshell
- Coding challenge 1 – AAA
- Coding challenge 2 – FIRST
- Coding challenge 3 – Test fixtures
- Coding challenge 4 – Exception testing
- Coding challenge 5 – Developer or tester
- Coding challenge 6 – JUnit extensions
- Coding challenge 7 – @Before* and @After* annotations
- Coding challenge 8 – Mocking and stubbing
- Coding challenge 9 – Test suite
- Coding challenge 10 – Ignoring test methods
- Coding challenge 11 – Assumptions
- Coding challenge 12 – @Rule
- Coding challenge 13 – Method test return type
- Coding challenge 14 – Dynamic tests
- Coding challenge 15 – Nested tests
- Scalability in a nutshell
- Coding challenge 1 – Scaling types
- Coding challenge 2 – High availability
- Coding challenge 3 – Low latency
- Coding challenge 4 – Clustering
- Coding challenge 5 – Latency, bandwidth, and throughput
- Coding challenge 6 – Load balancing
- Coding challenge 7 – Sticky session
- Coding challenge 8 – Sharding
- Coding challenge 9 – Shared-nothing architecture
- Coding challenge 10 – Failover
- Coding challenge 11 – Session replication
- Coding challenge 12 – The CAP theorem
- Coding challenge 13 – Social networks
- Designing bitly, TinyURL, and goo.gl (a service for shorting URLs)
- Designing Netflix, Twitch, and YouTube (a global video streaming service)
- Designing WhatsApp and Facebook Messenger (a global chat service)
- Designing Reddit, HackerNews, Quora, and Voat (a message board service and social network)
- Designing Google Drive, Google Photos, and Dropbox (a global file storage and sharing service)
- Designing Twitter, Facebook, and Instagram (an extremely large social media service)
- Designing Lyft, Uber, and RideAustin (a ride-sharing service)
- Designing a type-ahead and web crawler (a search engine related service)
- Designing an API rate limiter (for example, GitHub or Firebase)
- Designing nearby places/friends and Yelp (a proximity server)
- Leave a review - let other readers know what you think
Product information
- Title: The Complete Coding Interview Guide in Java
- Author(s): Anghel Leonard
- Release date: August 2020
- Publisher(s): Packt Publishing
- ISBN: 9781839212062
You might also like
The complete java developer course: from beginner to master.
by Codestars By Rob Percival, John P. Baugh
Be it websites, mobile apps, or desktop software, Java remains one of the most popular programming …
Java SE 17 Developer (1Z0-829)
by Simon Roberts
21+ Hours of Video Instruction An intensive and guided video course to learn and practice while …
Java Coding Problems
by Anghel Leonard
Develop your coding skills by exploring Java concepts and techniques such as Strings, Objects and Types, …
Design Patterns and SOLID Principles with Java
by Róbert Kohányi
Write maintainable, extensible, and cloud-ready code in Java, using design patterns and SOLID principles About This …
Don’t leave empty-handed
Get Mark Richards’s Software Architecture Patterns ebook to better understand how to design components—and how they should interact.
It’s yours, free.
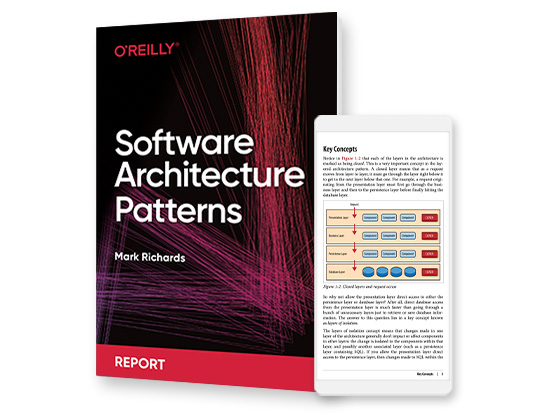
Check it out now on O’Reilly
Dive in for free with a 10-day trial of the O’Reilly learning platform—then explore all the other resources our members count on to build skills and solve problems every day.
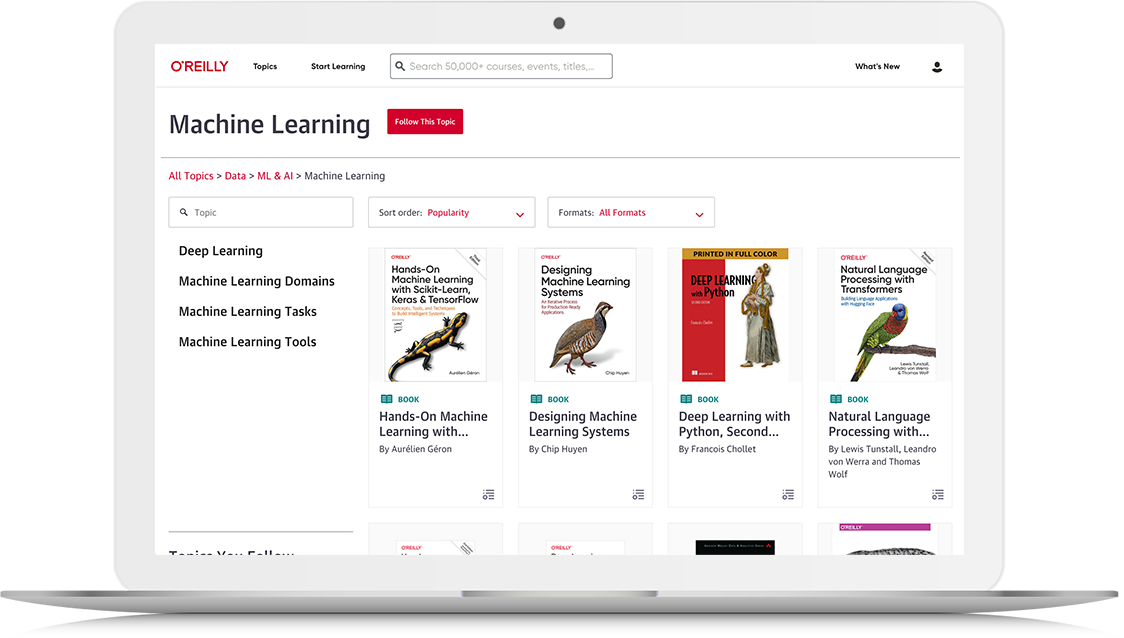
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
The Complete Coding Interview Guide in Java, published by Packt
PacktPublishing/The-Complete-Coding-Interview-Guide-in-Java
Folders and files, repository files navigation, the complete coding interview guide in java.
This is the code repository for The Complete Coding Interview Guide in Java , published by Packt.
An effective guide for aspiring Java developers to ace their programming interviews
What is this book about?
Java is one of the most sought-after programming languages in the job market, but cracking the coding interview in this challenging economy might not be easy. This comprehensive guide will help you to tackle various challenges faced in a coding job interview and avoid common interview mistakes, and will ultimately guide you toward landing your job as a Java developer.
This book covers the following exciting features: Solve the most popular Java coding problems efficiently Tackle challenging algorithms that will help you develop robust and fast logic Practice answering commonly asked non-technical interview questions that can make the difference between a pass and a fail Get an overall picture of prospective employers' expectations from a Java developer Solve various concurrent programming, functional programming, and unit testing problems
If you feel this book is for you, get your copy today!
Instructions and Navigations
All of the code is organized into folders. For example, Chapter02.
The code will look like the following:
Following is what you need for this book: This book is for students, programmers, and employees who want to be invited to and pass interviews given by top companies. The book assumes high school mathematics and basic programming knowledge.
With the following software and hardware list you can run all code files present in the book (Chapter 1-19).
Software and Hardware List
We also provide a PDF file that has color images of the screenshots/diagrams used in this book. Click here to download it .
Related products
Java Coding Problems [Packt] [Amazon]
Learn Java 12 Programming [Packt] [Amazon]
Get to Know the Author
Anghel Leonard is a chief technology strategist with more than 20 years’ experience in the Java ecosystem. In his daily work, he is focused on architecting and developing Java-distributed applications that empower robust architectures, clean code, and high performance. He is also passionate about coaching, mentoring, and technical leadership.
Suggestions and Feedback
Click here if you have any feedback or suggestions.
Download a free PDF
If you have already purchased a print or Kindle version of this book, you can get a DRM-free PDF version at no cost. Simply click on the link to claim your free PDF.
https://packt.link/free-ebook/9781839212062
Contributors 6
- Java 100.0%
LTCWM > Blog > Working in tech > Job hunting > The 33 Best Technical Interview Prep Courses, Books, & Resources
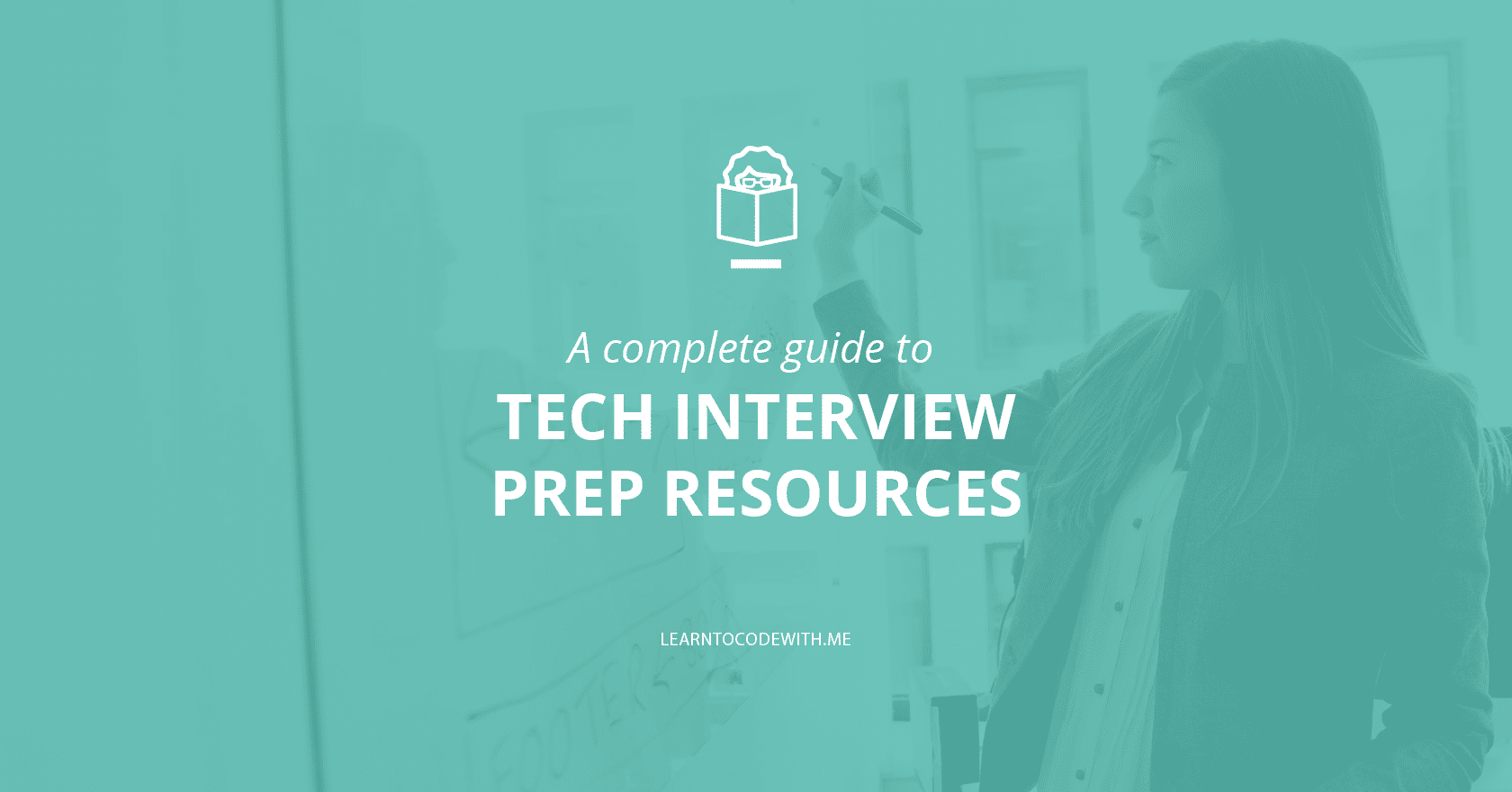
The 33 Best Technical Interview Prep Courses, Books, & Resources
Updated on March 6th, 2023 | Sign up for learn to code tips
Tech interviews can be intimidating. They often have several phases , from the first technical phone screen, to a remote coding interview or assignment, to the final on-site interview and whiteboard challenge.
If you’ve never been through one before, you may have no idea how to prepare for a coding interview.
It’s not enough to just have the raw tech skills . You also need to be able to perform under pressure, talk through your code to give the interviewer a window into your thoughts, and be clear and precise with your tech vocabulary and communication. 😅
As with most things in life, the way to build up your confidence and experience is through practice. If you know what you’ll be facing in technical interviews and have done a ton of code interview prep, you’ll breeze through the real thing. 👌
32 amazing resources to help you ace your technical interview Click To Tweet
Fortunately, there are lots of resources out there to help you do this! Whether you want to take a tech interview prep course, read some of the best coding interview books, or practice with live mock interviews, there are great options out there!
Below, I’ve rounded up some of the best coding interview books and tech interview prep courses to help you practice programming interview questions and wow the hiring managers at your code interview.
Disclosure: I’m a proud affiliate for some of the resources mentioned in this article. If you buy a product through my links on this page, I may get a small commission for referring you. Thanks!
TL;DR Best Coding Interview Resources
If you’re just looking for some quick links to find a good tech interview prep course, I won’t make you read the whole article! Here’s a quick list of the best coding interview prep sites/resources.
- 🖥️ Best technical interview prep course: Interview Cake
- 📝 Best text-based code interview course: Grokking the Coding Interview
- 👩🏫 Best program for mock interviews/ career support: Interview Kickstart
- 🗣️ Best free coding interview course: Full-Stack Interview Prep on Udacity
- 📚 Best coding interview book, via Amazon: Cracking the Coding Interview: 189 Programming Questions and Solutions
- 📕 Best technical interview book for new coders: Programming Interviews Exposed
How Long to Prepare for a Coding Interview: How Much Time Do You Have?
⏱️ How many hours of preparation for coding interviews are recommended? It depends on what code interview prep methods you’re using. Start by checking out our article on the best ways to prepare for a tech interview .
Then, tailor the resources you use based on how much time you have before your interview.
For example, if you only have one week, you probably don’t want to start an interview bootcamp that lasts 3+ weeks. However, if you’re looking into how to prepare for a coding interview in one month or more, a structured course or detailed book could be an option for you.
⌛ There are plenty of resources to help you prepare for a coding interview in one week, including mock interviews, skimmable books with practice problems, short courses that only require a few hours of study, etc. Since your time is limited in this scenario, it’s wise to focus on breadth over depth.
⏳ But if you’re preparing well in advance, you can consider something more involved, such as a software engineer interview prep course, bootcamp, or book with lots of practice problems you can study/work on over the course of a few weeks or months. Theoretical algorithms books can be good if you have the time to dive deeper into important interview subjects and make sure your knowledge is solid.
It can be smart to take a technical interview prep course (or start reading coding interview books) before you even have one scheduled. That way, you won’t find yourself panicking when you get that first interview request!
Best Coding Interview Courses
With a coding interview preparation course, you can practice coding interview questions in a hands-on way, and their video content is useful if you’re a visual-audio learner.
Here are some of the best coding interview courses you can find:
Please note that pricing listed below may change in the future!
1. Interview Cake
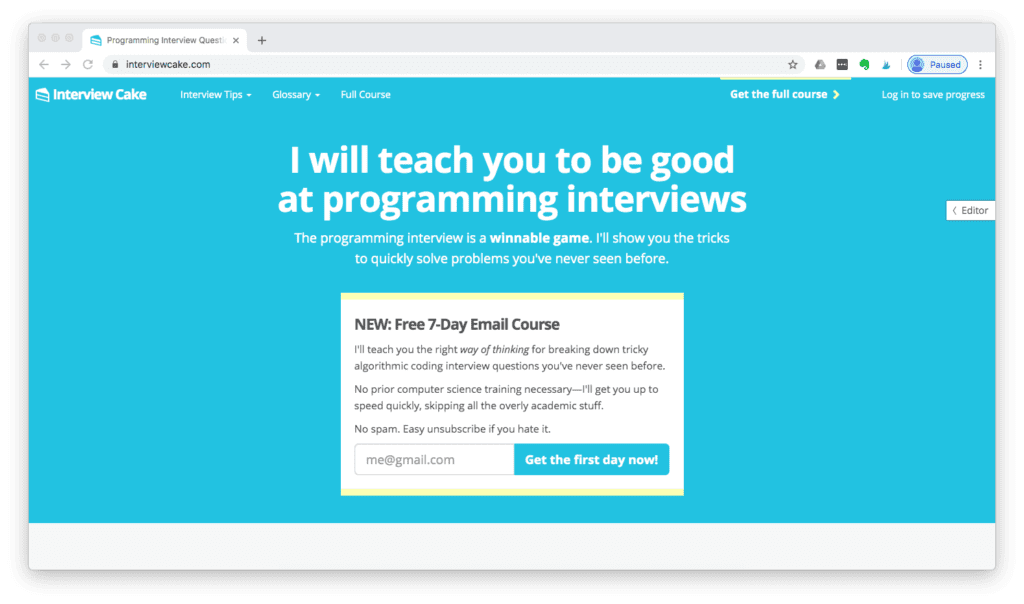
Hands down one of the best coding interview preparation courses out there!
Interview Cake comes with 50+ hours of coding interview practice questions and readings that don’t just give you the answer, but teach you the right way of thinking about the question. Build the skills to derive the right answers yourself and explain your reasoning.
- 🔗 Link: Get it from Interview Cake
- Common coding interview questions
- All the core data structures (linked lists, queues, stacks, etc.)
- All the core problem types (system design, recursion, etc.)
- How to answer technical interview questions you don’t know
- 💰 Price details: $149 for 3 weeks access or $249 for 3 months (plus 20% off for LTCWM readers and a full refund if you’re unsatisfied)
“Every interview I’ve gone through since I started studying your problem set has included a variation of the questions on Interview Cake! There was one problem that wasn’t on here, but I was able to solve it because of the themes you taught. Today I am accepting an offer at a startup in SF!” – Ulises
“I used Interview Cake to get my job at Netflix” – @kittycatbites on Twitter
Read my full review of Interview Cake here.
2. AlgoExpert
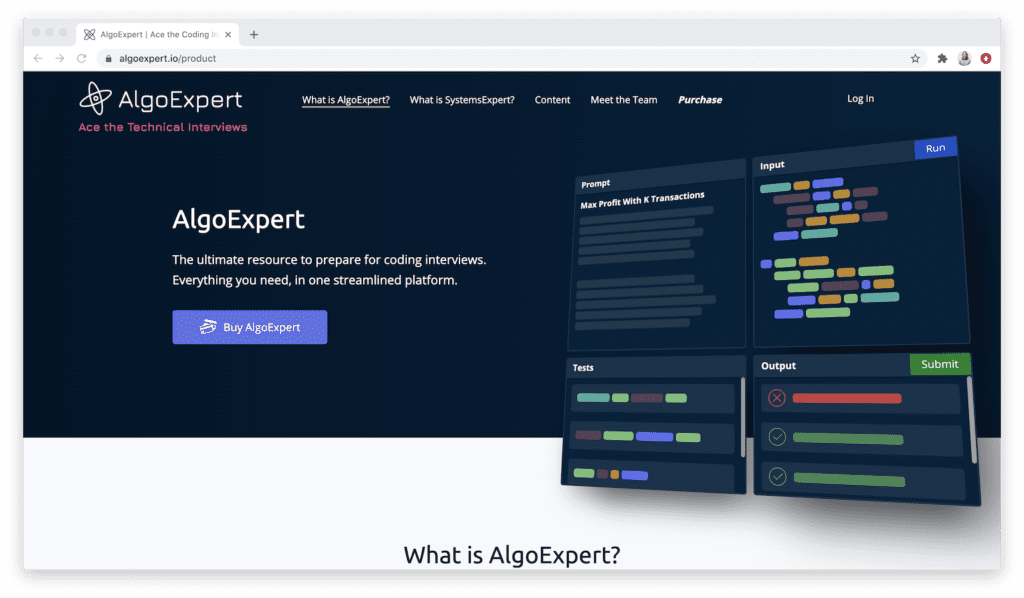
A comprehensive overview of 115 of the best programmer interview questions in nine different languages: JavaScript, Python, Swift, C++, Java , C#, Kotlin, TypeScript and Go. You type out your answers in a code-execution environment and get 70+ hours of video explanations to help you understand the core principles behind each question.
- 🔗 Link: Get it from AlgoExpert
- Binary search trees, linked lists, strings, etc.
- How much memory an algorithm uses and how fast it runs
- Tips for standing out in your interview
- 💰 Price details: $99 for 1 year of access (plus 10% off for LTCWM readers with the coupon code ltcwm )
“After failing technical interviews over and over again during my internship hunt, I decided to start using AlgoExpert alongside LeetCode. The user friendly UI and excellent explanation from Clement makes the learning experience very enjoyable, and I was able to make incredible progress. This year I crushed the Amazon interviews and received a new grad offer from them to work as a Software Development Engineer. Thank you AlgoExpert!” – James
“Studying up for technical interviews and can’t recommend AlgoExpert enough for knocking out programming questions and staying sharp. Good way to learn too” – @BB_kidcazer on Twitter
3. Skilled.dev
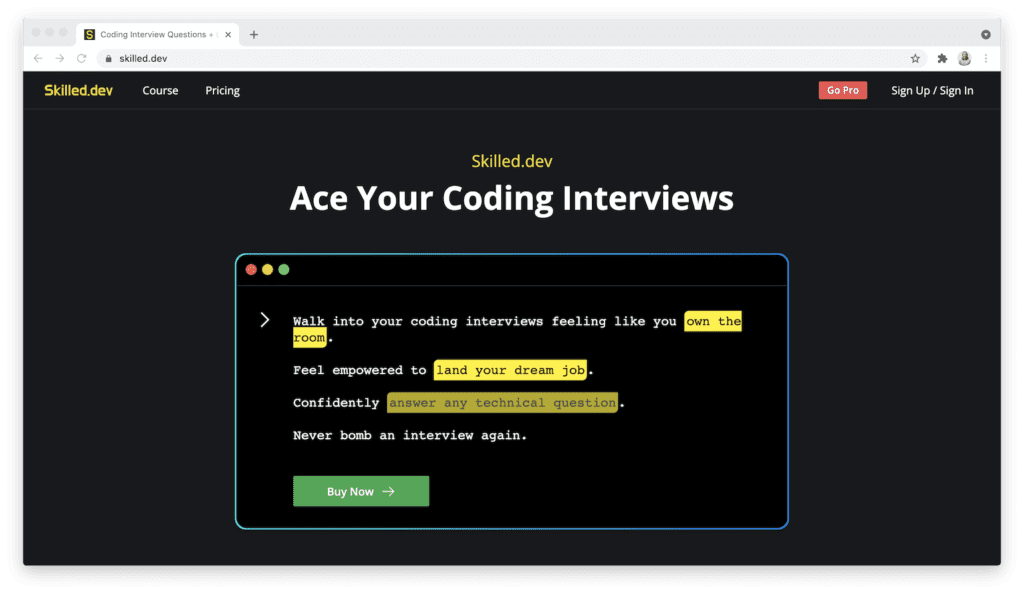
The tech interview prep course on Skilled.dev distills years of interview expertise into one convenient package!
Their programming interview prep questions are carefully selected to teach you exactly what you need to know to crush your coding interviews. This coding interview course comes with detailed video walkthroughs for every solution, a coding environment, and articles that break down how to think through each question.
- 🔗 Link: Get it from Skilled.dev
- Common coding interview questionsCore data structures and algorithms (linked lists, queues, stacks, etc.)
- Detailed Big O complexity analysis
- JavaScript interview questions
- 💰 Price details: $99 for 1 year full course access
“Thanks to @Skilled_dev, I also passed my Microsoft interview. First question I received was to output a matrix in spiral form” – @CodeWithDean on Twitter
4. Land Your First Tech Job on Break Into Tech
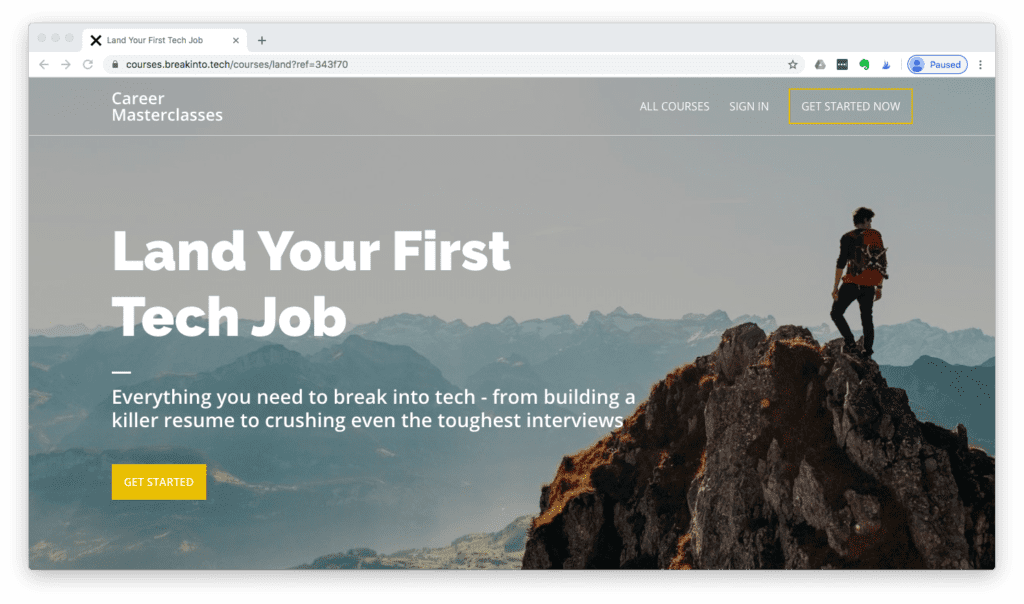
This technical interview prep course goes beyond the tech interview to include the rest of the job application process .
In 20 hours of videos and exercises, you’ll learn about optimizing your resume and LinkedIn profile , how to get referred to a company , how to answer the dreaded “tell me about yourself” questions, and templates to help you answer any tech interview question.
- 🔗 Link: Get it from Break Into Tech
- ⬇️ What you’ll learn: Keywords to include on your resume, communication tips, strategies to navigate tech interviews, how to think like an interviewer, etc.
- 💰 Price: $99 for the course or $199 for a resume review too (plus a refund if you’re not happy)
“My completion percentage of Jeremy’s course had an almost direct correlation with me getting a job. After following the advice in the course, I received a verbal offer and a final interview with an EVP before even watching all the video content. (Although I’d read all the text summaries…) Strongly recommend for structuring your tech job search process.” – Adil
5. Interview Camp
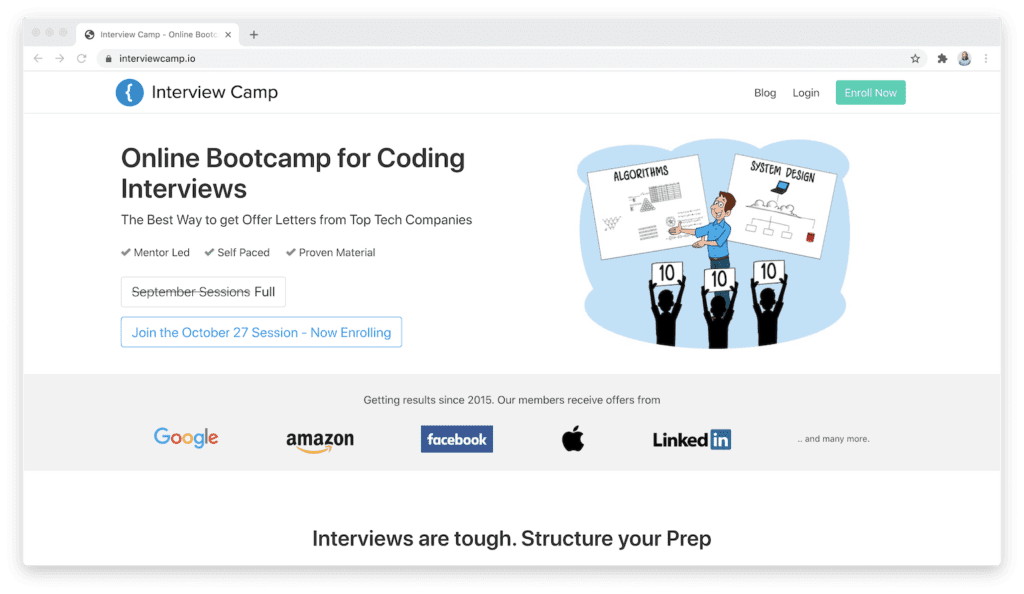
Interview Camp specializes in weekly live sessions to help you learn tried and tested, real-life techniques for conquering coding interviews. There’s an additional 60+ hours of self-paced online content, so you can get as much coding interview preparation as you want. Plus, join the private Slack community for an extra level of support and accountability.
- 🔗 Link: Get it from Interview Camp
- Algorithms – practical techniques with real practice problems and homework assignments
- System design – learn essential frameworks and study core system design components in depth
- 💰 Price details: $250/year for all of the above
6. Mastering the Software Engineering Interview on Coursera
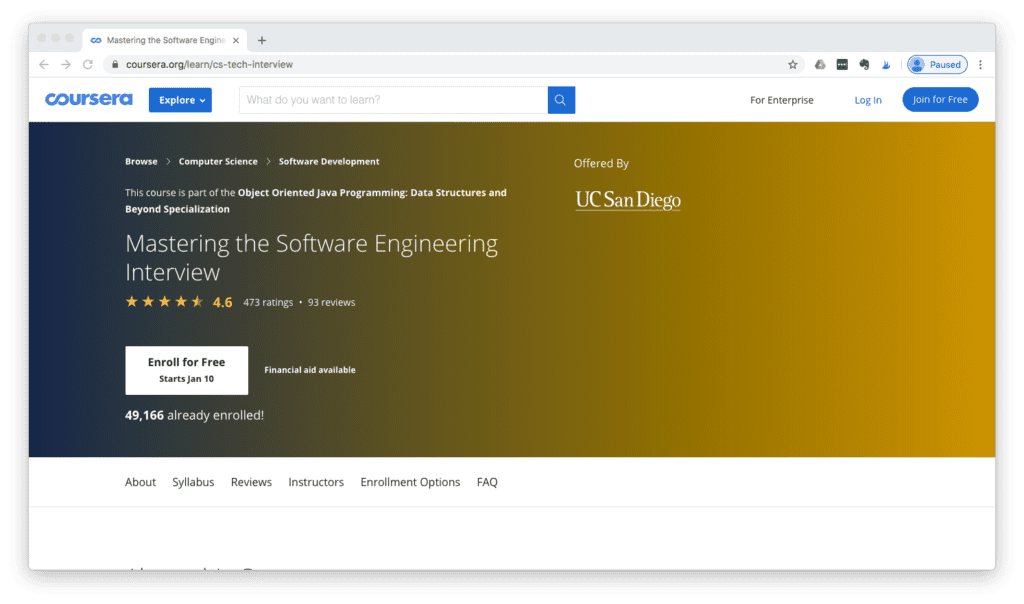
This code interview course of the University of California San Diego was put together with the support of Google’s recruiting and engineering teams, so you know you’re getting relevant advice. It takes about 21 hours to complete the videos, reading, and exercises, so it’s doable to prepare for a coding interview in one week with this technical interview preparation course.
- 🔗 Link: Get it from Coursera
- Algorithmic thinking on the fly
- Practice and tips for getting through the technical phone interview
- How to speak about your experience
- 💰 Price details: 7-day free trial, then $49/month You can also audit the course for free
“I found this course very useful. It covers all of the important points of facing a technology job interview, even including how to deal with psychological emotions and build a strong growth mindset.” – MH
7. Master the Coding Interview: Algorithms + Data Structures on Udemy and Skillshare
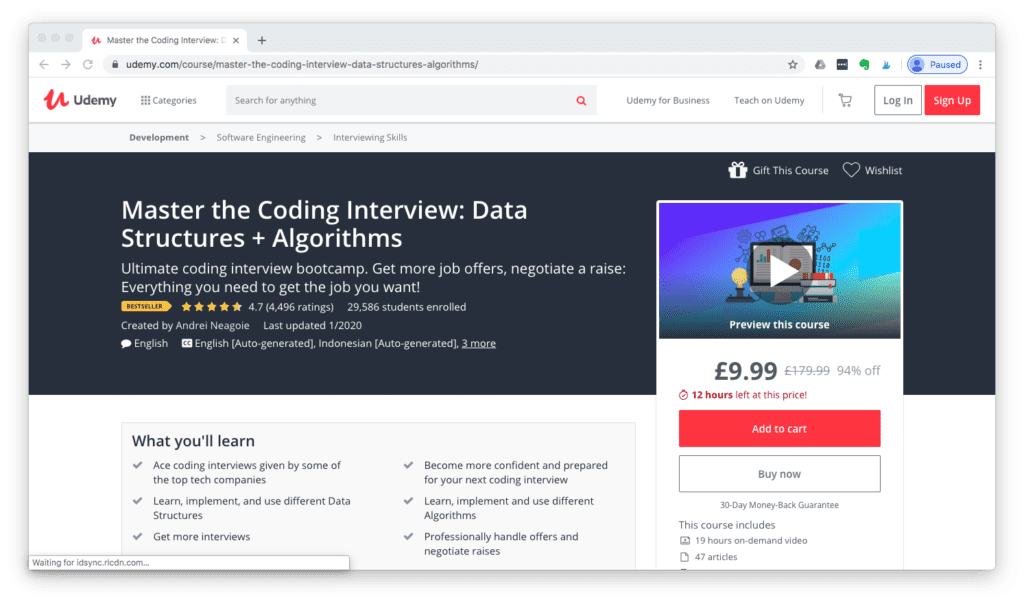
Algorithms and data structures usually constitute the bulk of coding interview questions. This tech interview prep course focuses on the fundamental building blocks of these computer science topics. It includes 19 hours of video, 51 articles, and other downloadable resources.
- 🔗 Link: Get it on Udemy or on Skillshare
- Big O notation
- Data structures: arrays, hash tables, queues, etc.
- Algorithms: recursion, sorting, searching, etc.
- How to get more interviews and handle offers
- 💰 Price: $84.99 on Udemy (but there are often sales), or do a one month free trial of Skillshare Premium (reg. $32/mo)
“Andrei’s course is really great! For non-CS students, this is a great introduction with easy-to-understand graphics, animation and explanation. For CS-students it’s a good review guide to comb through knowledge you might have forgotten […] Highly recommend it to anyone who is seeking a developer job and needs to go through code/technical interview.” – Annie
8. How to Pass a Coding Interview on Udemy and Skillshare
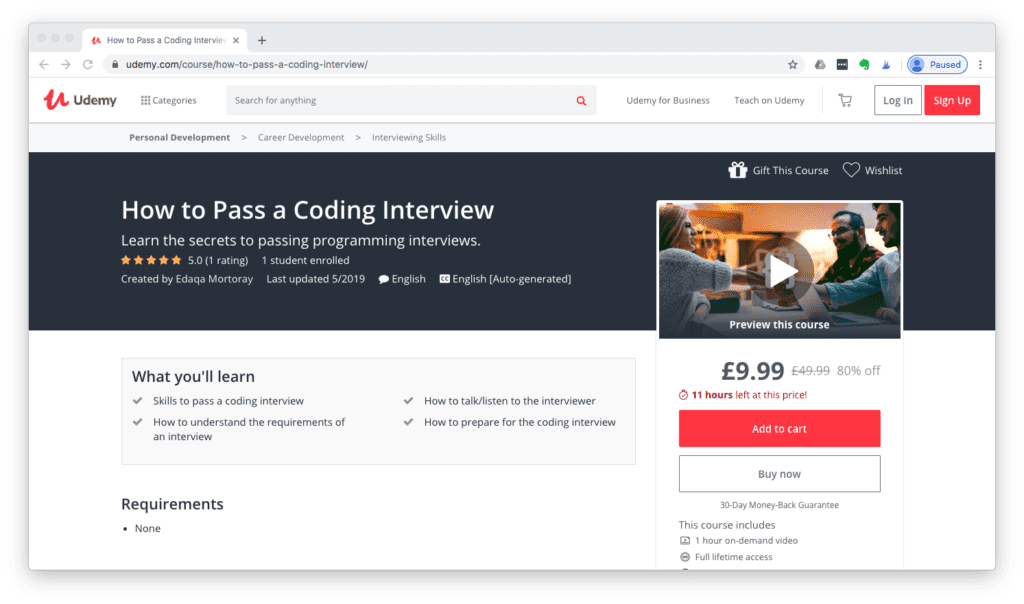
This short and sweet one-hour video course goes over stress-free coding interview prep, from the soft skills of interacting with your interviewer to the more technical elements like writing code and dealing with algorithms.
If you want to know the best way to prepare for coding interviews quickly , ultra-efficient courses like this certainly help!
- 🔗 Links: Get it on Udemy or on Skillshare
- How to talk to the interviewer
- The requirements of an interview
- How to prepare for the coding interview
- 💰 Price: $49.99 on Udemy (but there are often sales) or do a 14-day free trial of Skillshare Premium (reg. $32/mo)
9. Master the JavaScript Interview on Udemy and Skillshare
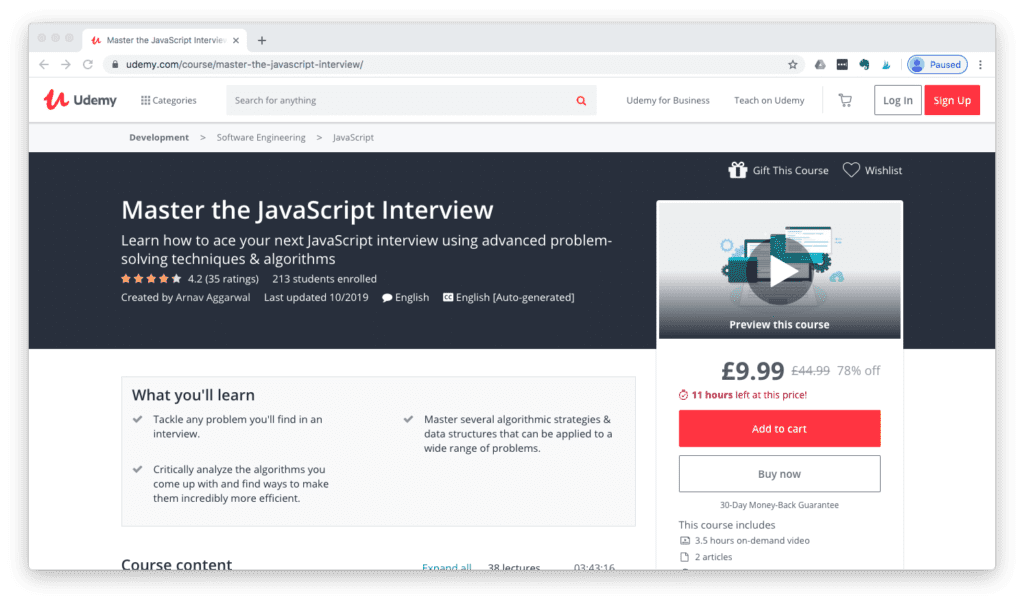
This is another relatively short technical interview prep course that specifically focuses on JavaScript interviews, diving into advanced problem-solving techniques and algorithms. You’ll see how some questions can have multiple solutions, which will all be thoroughly explained. The code interview course contains 3.5 hours of on-demand videos and two articles.
- Strings and arrays, algorithms and data structures, JavaScript
- Algorithm strategies and critical analysis
- Common interview problems
- 💰 Price: $44.99 on Udemy (but there are often sales) or do a 14-day free trial of Skillshare Premium (reg. $32/mo)
“Concepts are properly explained by the instructor. This is really a good course for Javascript interviews.” – Sheshadri
10. Get Ready for Your Coding Interview on LinkedIn Learning
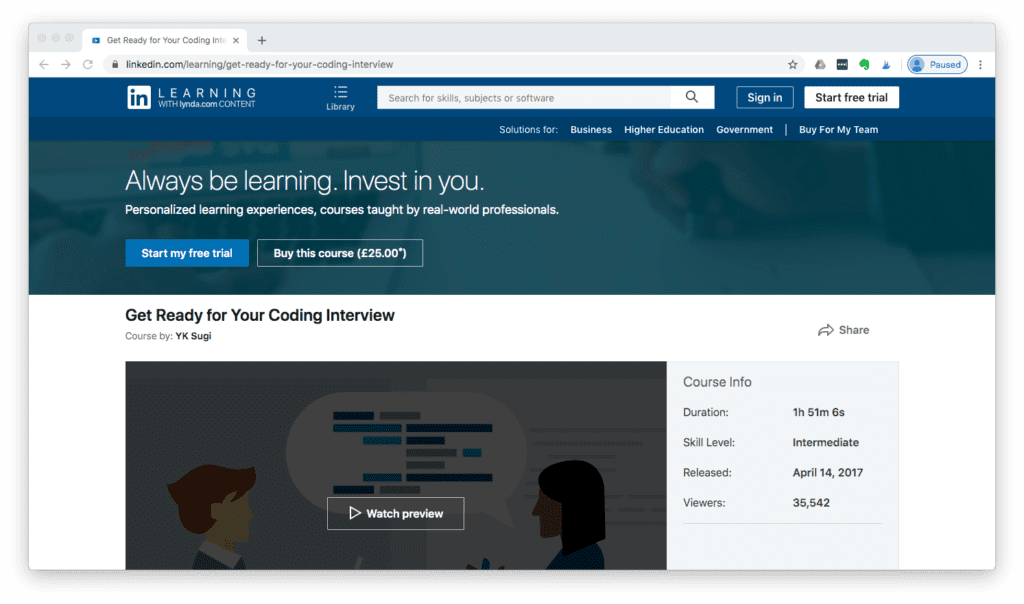
A two-hour video course covering useful concepts about what you’ll face in a coding interview. It also includes coding interview practice questions to test you.
- 🔗 Link: Get it from LinkedIn Learning
- What coding interviews are like
- Two-dimensional arrays, time complexity, Big-O notation, hash tables
- Coming up with an optimal solution and testing code
- 💰 Price: $19.99/month billed annually or $39.99/month billed monthly (or free with one-month trial)
11. The Coding Interview Bootcamp: Algorithms + Data Structures on Udemy
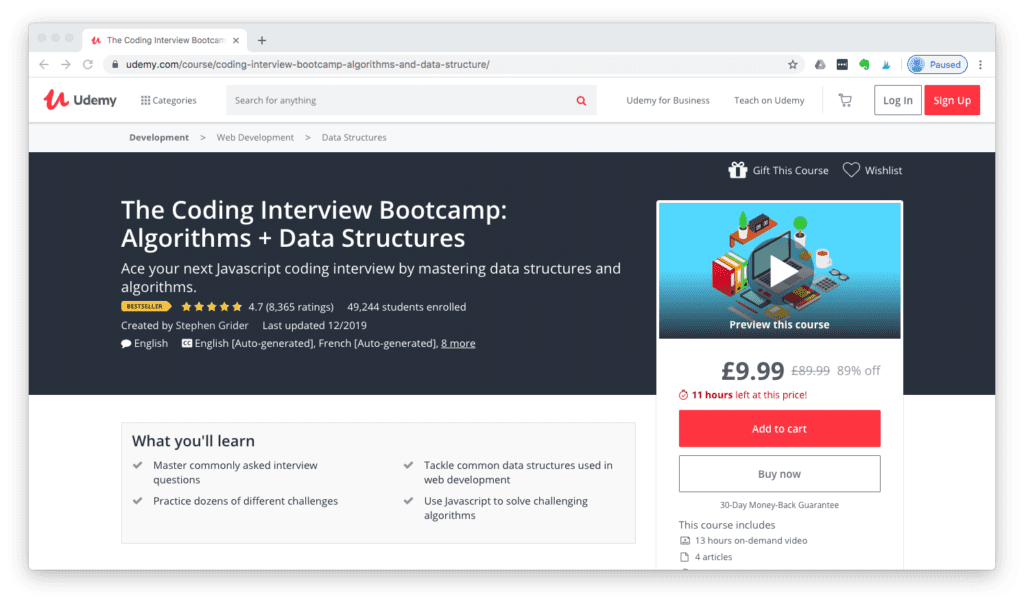
This 13-hour coding interview course goes over dozens of the most common interview questions you may face—and the tricks to solve them. It features real questions asked at companies like Google, Facebook, and Amazon, and lays out clear explanations for each solution.
You’ll need a basic understanding of JavaScript as a prerequisite.
- 🔗 Link: Get it from Udemy
- Interview questions asked at top companies
- Common data structures used in web development
- How to use JavaScript to solve challenging algorithms
- String reversal, anagrams, etc.
- 💰 Price: Full price $89.99 (less during Udemy sales)
“Stephen does an excellent job of thoroughly explaining each method and data structure. He uses plenty of detailed examples, making it very easy to understand. He doesn’t go straight to the solution, he explains things in a way that makes it easy to understand with charts and very descriptive diagrams. It makes for very easy learning, Bravo Stephen you sir are very good at what you do!!! I give my highest recommendation for this course. You won’t be disappointed.” – Essence
12. 11 Essential Coding Interview Questions + Coding Exercises! on Udemy
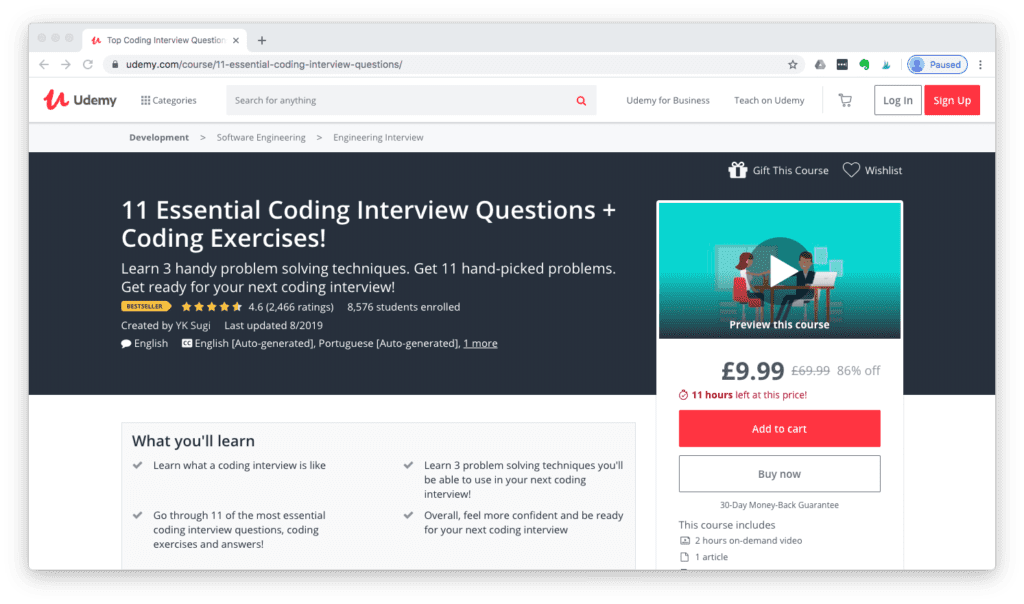
In this two-hour video course with plenty of interactive exercises, you’ll learn three handy problem-solving techniques to figure them out.
Before taking this coding interview preparation course, you should be familiar with basic data structures , Big O notation, and ideally Python and/or Java , which you’ll be using to solve problems.
- 3 problem-solving techniques
- 11 of the most essential coding interview questions
- Arrays, strings, linked lists, etc.
- 💰 Price: Full price $69.99 (less during Udemy sales)
“His way of explaining the problem is excellent! It really speaks to me and how I think. It must have been so time consuming for him to go through such detail per problem. But it’s so helpful and appreciated!! Thank you for sharing your gift of technical understanding. So few can do this so well.” – J
13. Java Interview Guide: 200+ Interview Questions and Answers on Udemy
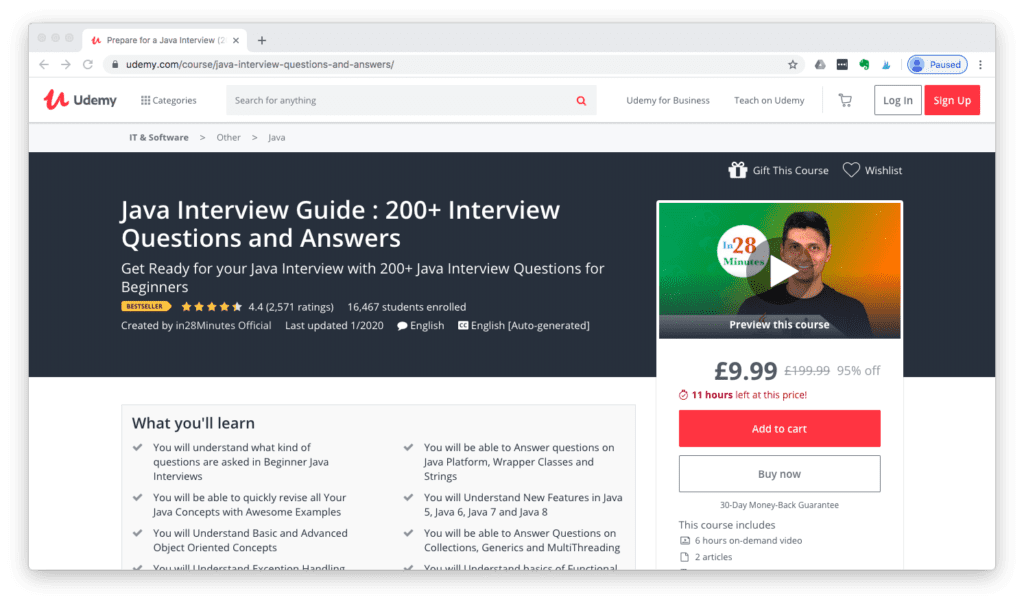
This Java-specific programming interview prep course will give you a useful refresher on key concepts before your whiteboarding interview. It contains 6 hours of on-demand video with hands-on examples, so give yourself a couple of days to work through it all before the big day!
- Basic and advanced object-oriented concepts
- Exception handling best practices
- Basics of functional programming
- 💰 Price: $$99.99 (less during Udemy sales)
“This course is excellent. Gives easy memorable answers to java interview questions. They’re simple to remember and easy to retain and explain to the interviewer. 10 out of 5 stars!!” – Jacqueline
14. Technical Interview Practice with Python on Codecademy
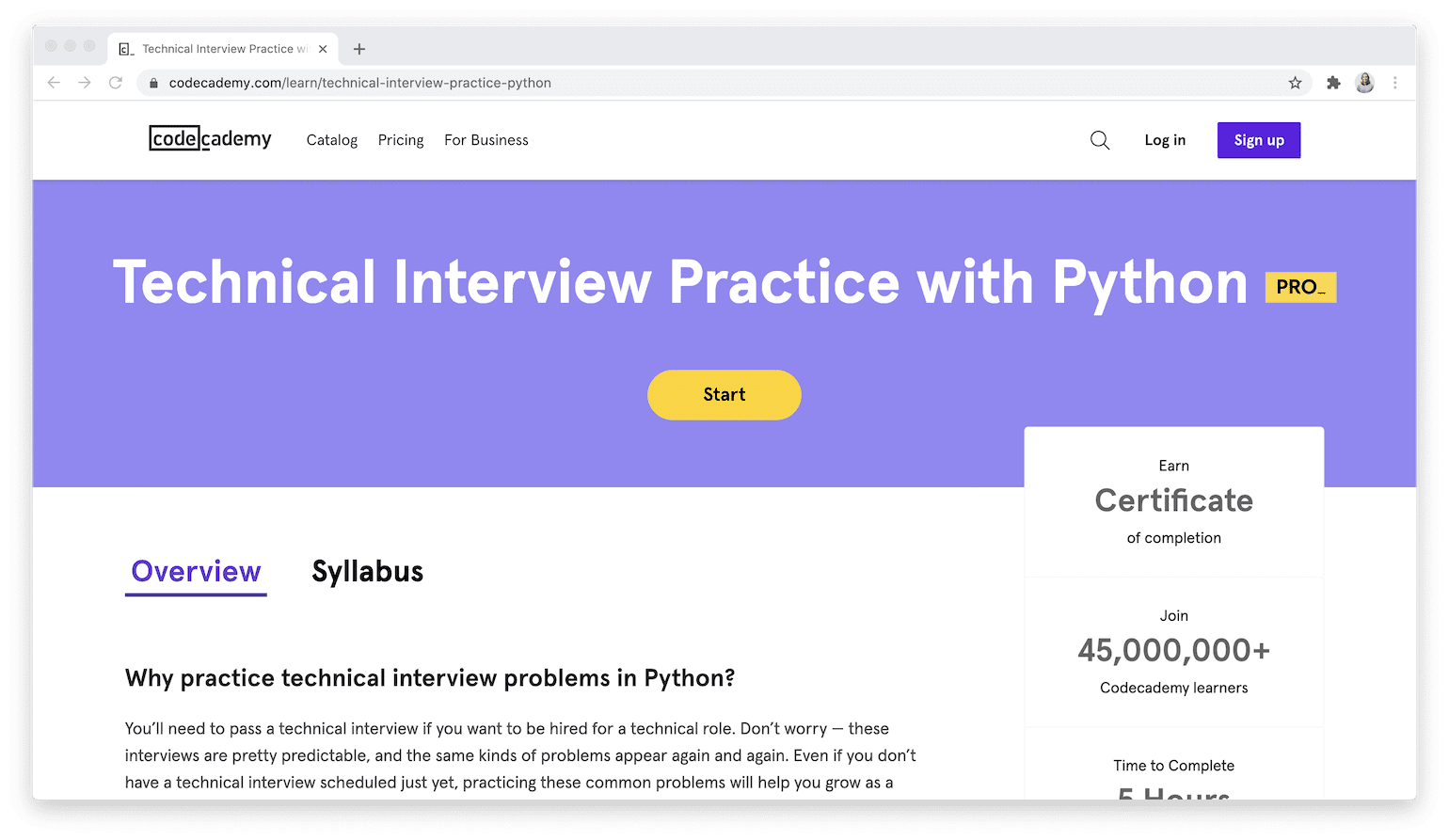
Even if you don’t have a technical interview scheduled just yet, practicing these common problems will help you grow as a programmer and problem solver, and will help you write cleaner and better code. After completing this tech interview prep course, you’ll be ready to ace coding interviews anywhere and you’ll write more efficient code!
- 🔗 Link: Get it from Codecademy
- Whiteboarding skills
- Common technical interview problems – lists and linked lists
- Dynamic programming
- 💰 Price: Free for 7 days with a trial of Codecademy Pro, then $17.49/month billed annually or $34.99 billed monthly
15. Full-Stack Interview Prep on Udacity
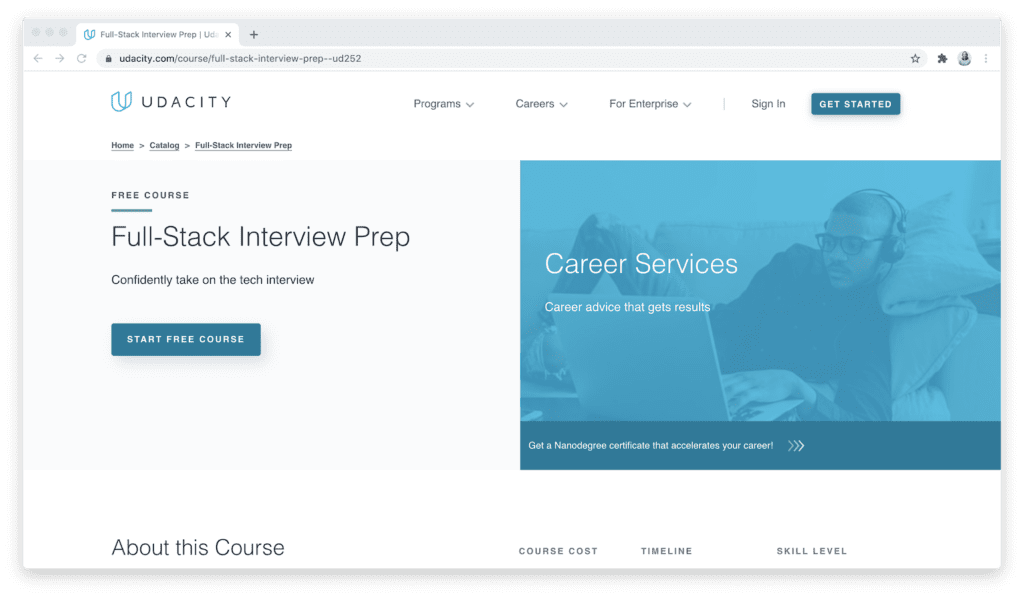
This course is an excellent way to prepare for technical interviews. You’ll experience a tech mock interview, and review detailed analysis on how to field key industry questions. Upon completing this course, you’ll be ready to showcase your skills during your full stack web developer interview!
- 🔗 Link: Get it from Udacity
- How to field key industry questions
- Common full-stack web developer interview topics like palindrome functions and data structures
- Best practices for behavioral questions and whiteboard problems
- 💰 Price: Free
16. Java Interview Questions: Data Structures and Algorithms on Mammoth Interactive
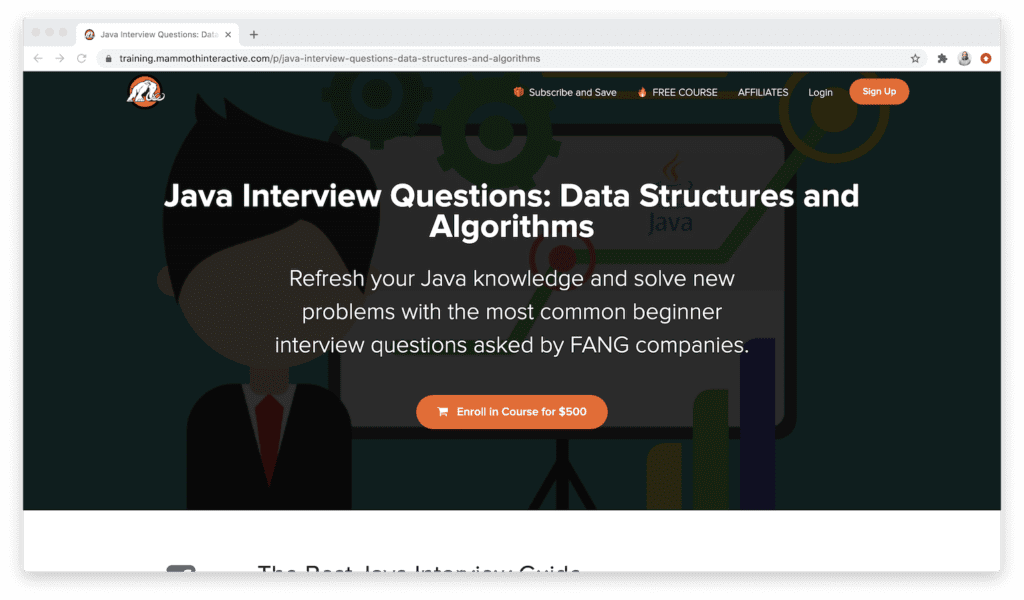
This course will help you to refresh your Java knowledge and solve new problems with the most common beginner interview questions asked by FANG companies such as Facebook, Google, Amazon, and Spotify.
- 🔗 Link: Get it from Mammoth Interactive
- Commonly asked interview questions
- The best way to answer an interview question
- How to analyze the time complexity of various algorithms
- 💰 Price: $19/month or $199/year for access to Mammoth Interactive’s huge library of courses
17. Big Machine Coding Interview Bootcamp
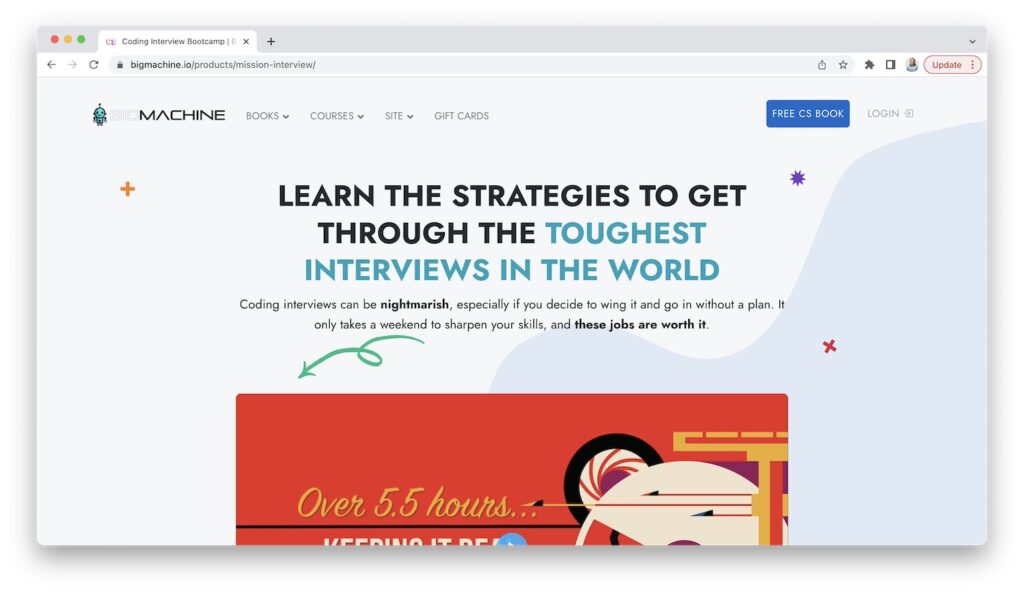
Trying to get in at one of the top tech companies in the world? This technical interview prep course from Big Machine includes 5 hours of video for you spread over 18 lessons that will teach you the strategies and techniques your peers use to pass tough tech interviews.
- 🔗 Link: Get it from Big Machine
- The art of coding every day
- The core programming concepts you need to pass a coding interview
- Algorithms and strategies
- Understanding the mechanics of the interview question
- Practice questions from real companies
- 💰 Price: $69
18. Interview Kickstart
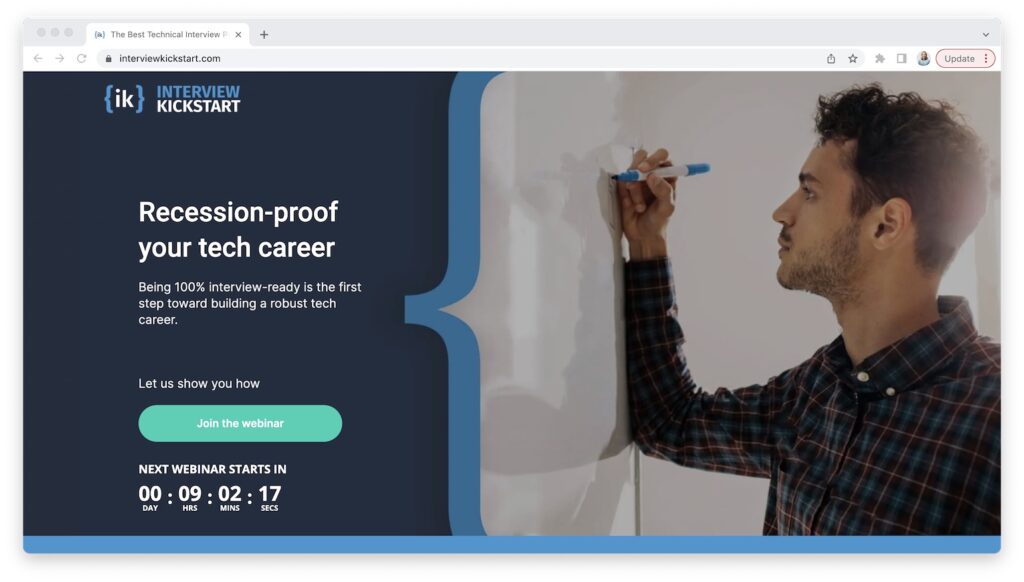
Interview Kickstart has 18 tech interview prep courses for key engineering roles, including back-end engineering, data science, embedded systems, full stack engineering, and more. Each coding interview course is designed and taught by FAANG+ experts in the domain.
Interview Kickstart also includes rigorous mock tech interviews with actual hiring managers, 1:1 technical coaching, personalized feedback, and more.
- 🔗 Link : Get it from Interview Kickstart
- How to recognize patterns in interview problems
- Overcome interview anxiety
- Data structures/algorithms
- Distributed systems design
- Domain topics
- Soft skills training
💰 Price: From $4,800 to $9,600 depending on the track you choose
19. AlgoMonster
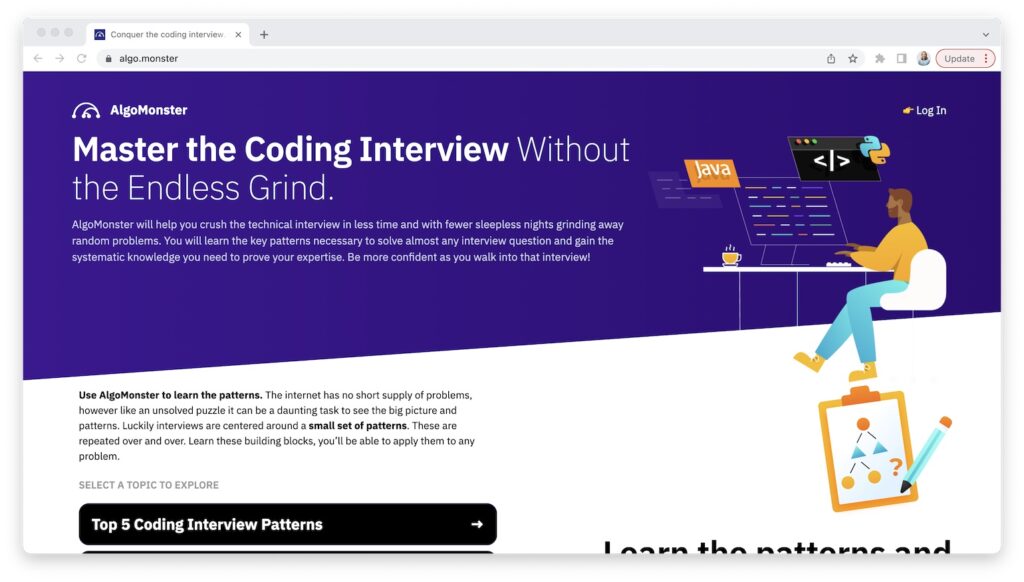
This code interview course is text-based, not video-based. Instead of an endless library of problems, AlgoMonster gives you a structured look at a much smaller, focused selection of coding interview patterns. AlgoMonster was designed by a group of Google engineers. It supports Python, Java, JavaScript, C++, Racket, Go, and Haskell.
They guarantee that you will receive an offer from at least one tech company—and 83% of people that complete the program do get a job offer!
- 🔗 Link : Get it from AlgoMonster
- The key patterns necessary to solve almost any interview question
- Binary search fundamentals
- Graph basics
- Heaps and priority queues
- 💰 Price: $24.99 for a monthly subscription / $169.99 for lifetime access
20. Grokking the Coding Interview: Patterns for Coding Questions
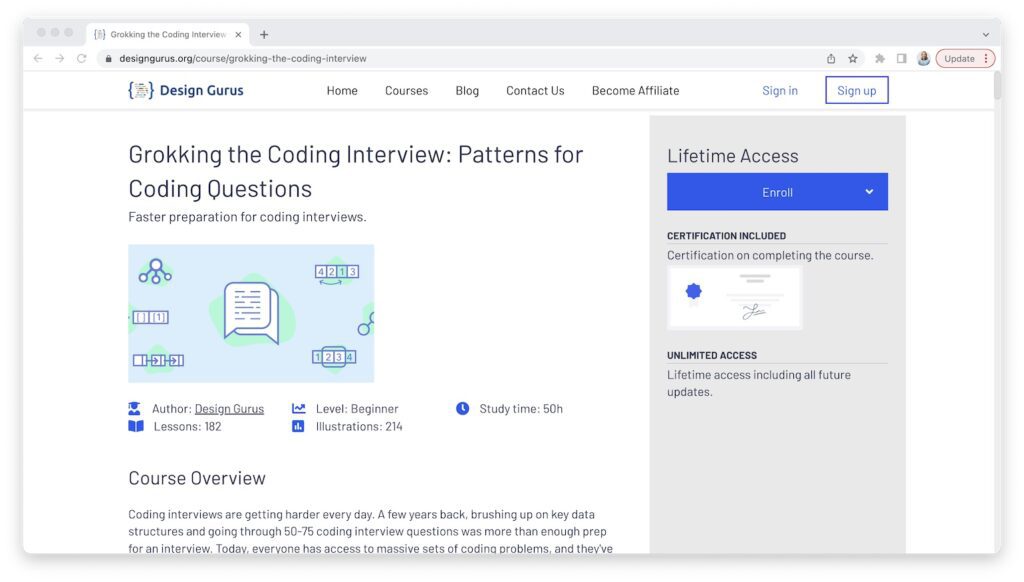
The techniques taught in this programming interview prep course have helped developers land jobs in top companies including Google, Facebook, Amazon, and Microsoft. It essentially condenses 3 years of CS into short bite-sized courses and lectures.
- 🔗 Link : Get it from DesignGurus
- ➡️ What you’ll learn: A list of 16 patterns for coding questions
- 💰 Price: $79
“I’ve tried every possible resource (Blind 75, Neetcode, YouTube, Cracking the Coding Interview, Udemy) and idk if it was just the right time or everything finally clicked but everything’s been so easy to grasp recently with Grokking the Coding Interview!” – @pokecoder on Twitter
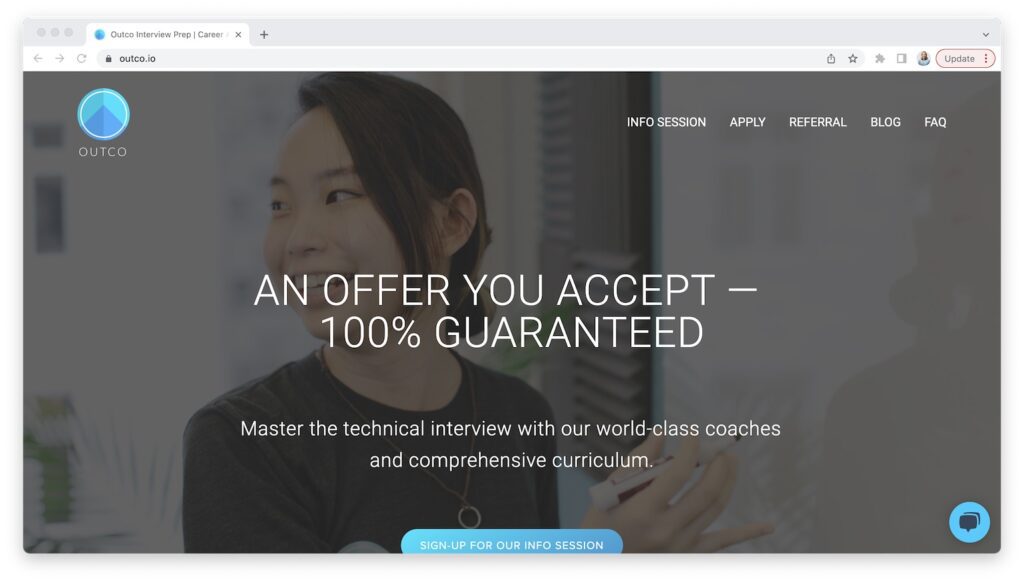
A 4-week or 8-week accelerator course for tech interview prep. Do unlimited mock tech interviews and work one-on-one with Outco career coaches until you get an offer. With their small classroom sizes, you’ll get personalized feedback to help you achieve your highest potential.
- 🔗 Link : Get it from Outco
- Data structures & algorithms
- System design
- Behavioral & leadership sessions
💰 Price: $5,500
Download the LinkedIn profile checklist
Created with aspiring techies in mind.
Success! Now check your email to confirm your subscription.
There was an error submitting your subscription. Please try again.
Best Coding Interview Books (General)
If you like a more traditional form of study, reading coding interview books might be your style! Find some of the best coding interview books below. In the next section, we’ll look at the best programming interview books for specific coding languages.
Disclosure : As an Amazon Associate, I earn from qualifying purchases and may earn a commission if you decide to buy through my links.
22. Cracking the Coding Interview: 189 Programming Questions and Solutions
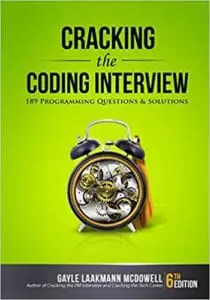
Learn strategies for reading between the lines in a tech interview question, how to answer technical interview questions you don’t know and get un-stuck, and core computer science concepts to refresh your memory. Practice with 189 Java-based questions and solutions.
The author worked as a software engineer at Google, Microsoft, and Apple, and conducted interviews with hundreds of other software engineers.
- 🔗 Link: Get it from Amazon
- Strategies to deal with algorithm questions
- An inside look at how big tech companies hire developers
- How to handle behavioral questions
“Special shoutout to Cracking the Coding Interview for helping me get a new job.” – @DallasRLillie on Twitter
23 . Elements of Programming Interviews in Python: The Insiders’ Guide
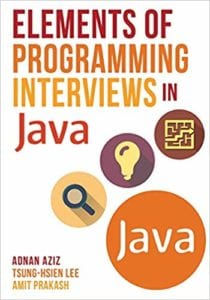
If you’re seeking a Python role, this book will prepare you with over 250 Python coding interview practice problems and their detailed solutions.
Versions are also available in C++ and Java .
- Data structures , algorithms, and problem-solving patterns
- Common coding interview mistakes
- Tips on negotiating the best job offer
“Book tip 4: ‘Elements of Programming interviews (in Python)’. This is by far the BEST book on preparing for coding/algorithm interviews. It was a key ingredient for me passing 20+ of these interviews, and still relevant now when I’m on the other side, interviewing👍 #Books #Mustread” – @Grananqvist on Twitter
24 . Daily Coding Problem: Get exceptionally good at coding interviews by solving one problem every day
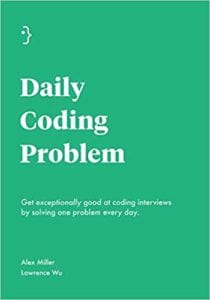
What better way to prepare for a coding interview than to practice code interview prep questions every single day? This book contains a wide variety of real-world-inspired coding questions and solutions. It’ll get you in the habit, so the day of your real whiteboard interview feels just like any other.
- ➡️ What you’ll learn: linked lists, arrays, heaps, trees, graphs, randomized algorithms, backtracking, dynamic programming, stacks and queues, bit manipulation, system design
25 . The Complete Software Developer’s Career Guide
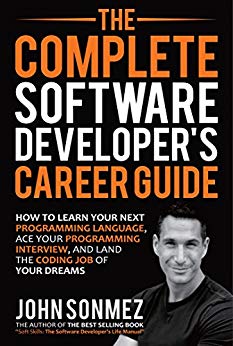
This book has a much broader scope beyond technical interviews. The author explains the things he’s learned about soft skills like communication, dedication to learning, negotiation tactics , and teamwork. He also covers questions like how to decide between different forms of coding education , the technical skills every developer needs, and what to wear to your interview .
- What skills will benefit you in your tech job hunt
- What kind of work to look for
- How to make yourself more marketable while learning to code
“The complete software developer’s career guide by @jsonmez is amazing 💯” – @fredcode_ on Twitter
26. Programming Interviews Exposed: Coding Your Way Through the Interview
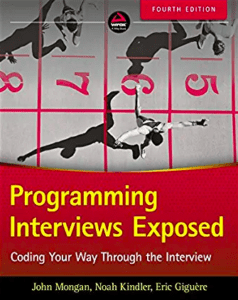
Written by three authors with tech experience, this book gives an inside look at unique programmer interviews, how online coding contests factor in, why contributing to GitHub can help you, and ultimately how to make yourself into a candidate that companies will want to hire.
- How to talk to interviewers
- Common interview problems and explanations
- How to come up with solutions to new problems
“I passionately recommend Programming Interviews Exposed: Secrets to Landing Your Next Job, 3rd Ed John Mongan, Noah Kindler, Eric Giguere :D” – @iam3ddie on Twitter
27. Surviving the Whiteboard Interview: A Developer’s Guide to Using Soft Skills to Get Hired
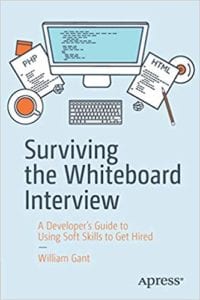
- Skills required to succeed at a whiteboard interview, covering coding tests as well as psychological preparation
- Master solving common whiteboard problems
You can do well at your code interview but still not get the job for one simple reason: the interviewers simply got along with someone better. Soft skills matter! This book goes over how to showcase positive elements of your personality like work ethic, positive attitude, and willingness to learn.
William Gant also gives tips for making it through the psychological roadblocks you might encounter during the process and taming your fears.
32 of the best coding interview courses and books to help you land your dream job in tech Click To Tweet
Best Coding Interview Books By Programming Language
28. java – the complete coding interview guide in java: an effective guide for aspiring java developers to ace their programming interviews.
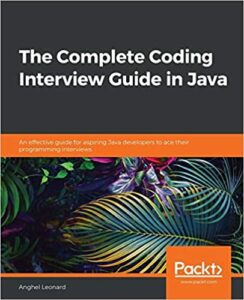
Covers over 200 Java coding interview problems and their solutions. This book will help you to develop skills in data structures and algorithms by solving various problems based on topics like arrays, strings, maps, linked lists, sorting, and searching.
- 200 coding interview problems
- How to solve tricky questions about concurrency, functional programming, and system scalability
- How to approach a coding interview problem in a structured way
29. Python – The Big Book of Coding Interviews in Python, 3rd Edition: answers to the best programming interview questions on data structures and algorithms
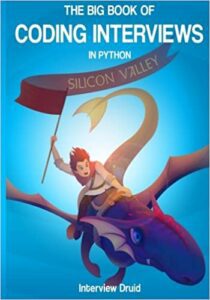
The questions in the book have been carefully selected so that they represent the most frequently asked questions in Python coding interviews. Solutions are clearly explained with plenty of diagrams and comments in the code so that you can easily understand.
- 300 coding interview questions
- Data structures
- Sorting searching
30. C++ – Advanced C++ Interview Questions You’ll Most Likely Be Asked (Job Interview Questions Series)
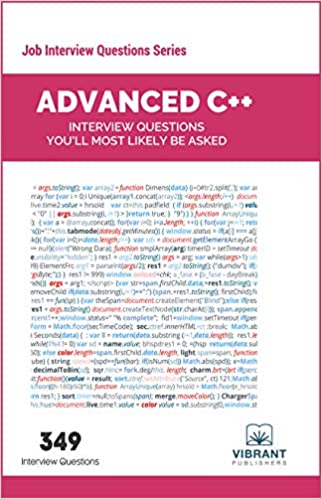
With real-life-scenario-based questions, strategies to respond to interview questions, and over 300 interview questions (coding and HR-based), this book preps you for a coding interview in C++.
Rather than going through comprehensive, textbook-sized reference guides, this book includes only the most important info you need to know to pass an interview.
- 274 coding interview questions
- 75 HR interview questions
- Free online aptitude tests
31. JavaScript – Slay the JavaScript Interview: 100 answers that every developer needs to know
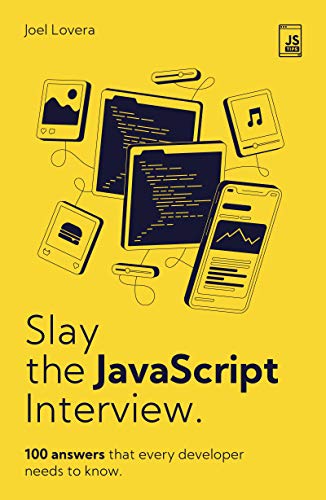
This book covers answers to the 100 most common and tricky interview questions in the JavaScript language. The solutions give you the absolute clearest explanation of the concepts possible. The purpose of this book is to share lessons and learnings the author has learned from 30+ interviews with top tech companies.
- The fundamental concepts in JavaScript
- Topics: events, variables, objects, arrays, functions, loops, conditional statements, garbage collection, RegExp, Functional programming and much more
32. C – Cracking C Programming Interview: 500+ interview questions and explanations to sharpen your C concepts for a lucrative programming career
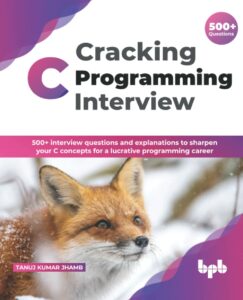
This coding interview prep book includes 500+ interview questions on C data structures/arrays with detailed solutions. Helps you strengthen your programming principles and understand how to answer the most popular interview questions asked at companies like Amazon, Facebook, Google, VMware, and Microsoft.
- Extensive coverage of C principles
- Scenarios and examples to explore programming strategies
- Procedural programming and the memory layout of a running C program
What are the best coding interview prep sites/resources?
There is no one-size-fits-all answer to this one! It depends on your learning style, which languages you’re training for, etc.
That said, my personal favorite resources to practice programming interview questions are Interview Cake and AlgoExpert.
You’ll get 20% off Interview Cake by using this link , and they have a refund guarantee if you don’t find it helpful—so it’s a no-risk way to get valuable programming interview prep. You can also get a 15% discount on AlgoExpert using the coupon code ltcwm .
Trying to prepare for a virtual interview, rather than an in-person one? Check out our guide on the remote interview process and get some tips for success.
Whatever resources you choose, I’m rooting for you! Best of luck crushing the technical interview and getting a job you love.
Note: This article contains Amazon affiliate links. As an Amazon Associate, I earn from qualifying purchases.

- Computers & Technology
- Programming

Enjoy fast, free delivery, exclusive deals, and award-winning movies & TV shows with Prime Try Prime and start saving today with fast, free delivery
Amazon Prime includes:
Fast, FREE Delivery is available to Prime members. To join, select "Try Amazon Prime and start saving today with Fast, FREE Delivery" below the Add to Cart button.
- Cardmembers earn 5% Back at Amazon.com with a Prime Credit Card.
- Unlimited Free Two-Day Delivery
- Streaming of thousands of movies and TV shows with limited ads on Prime Video.
- A Kindle book to borrow for free each month - with no due dates
- Listen to over 2 million songs and hundreds of playlists
- Unlimited photo storage with anywhere access
Important: Your credit card will NOT be charged when you start your free trial or if you cancel during the trial period. If you're happy with Amazon Prime, do nothing. At the end of the free trial, your membership will automatically upgrade to a monthly membership.
Buy new: .savingPriceOverride { color:#CC0C39!important; font-weight: 300!important; } .reinventMobileHeaderPrice { font-weight: 400; } #apex_offerDisplay_mobile_feature_div .reinventPriceSavingsPercentageMargin, #apex_offerDisplay_mobile_feature_div .reinventPricePriceToPayMargin { margin-right: 4px; } -21% $31.77 $ 31 . 77 FREE delivery Saturday, May 18 on orders shipped by Amazon over $35 Ships from: Amazon.com Sold by: Amazon.com
Return this item for free.
Free returns are available for the shipping address you chose. You can return the item for any reason in new and unused condition: no shipping charges
- Go to your orders and start the return
- Select the return method
Save with Used - Good .savingPriceOverride { color:#CC0C39!important; font-weight: 300!important; } .reinventMobileHeaderPrice { font-weight: 400; } #apex_offerDisplay_mobile_feature_div .reinventPriceSavingsPercentageMargin, #apex_offerDisplay_mobile_feature_div .reinventPricePriceToPayMargin { margin-right: 4px; } $12.56 $ 12 . 56 FREE delivery Saturday, May 18 on orders shipped by Amazon over $35 Ships from: Amazon Sold by: Jenson Books Inc

Download the free Kindle app and start reading Kindle books instantly on your smartphone, tablet, or computer - no Kindle device required .
Read instantly on your browser with Kindle for Web.
Using your mobile phone camera - scan the code below and download the Kindle app.

Follow the author

Image Unavailable

- To view this video download Flash Player

Java Programming Interviews Exposed 1st Edition
Purchase options and add-ons.
Java is a popular and powerful language that is a virtual requirement for businesses making use of IT in their daily operations. For Java programmers, this reality offers job security and a wealth of employment opportunities. But that perfect Java coding job won't be available if you can't ace the interview. If you are a Java programmer concerned about interviewing, Java Programming Interviews Exposed is a great resource to prepare for your next opportunity. Author Noel Markham is both an experienced Java developer and interviewer, and has loaded his book with real examples from interviews he has conducted.
- Review over 150 real-world Java interview questions you are likely to encounter
- Prepare for personality-based interviews as well as highly technical interviews
- Explore related topics, such as middleware frameworks and server technologies
- Make use of chapters individually for topic-specific help
- Use the appendix for tips on Scala and Groovy, two other languages that run on JVMs
Veterans of the IT employment space know that interviewing for a Java programming position isn't as simple as sitting down and answering questions. The technical coding portion of the interview can be akin to a difficult puzzle or an interrogation. With Java Programming Interviews Exposed , skilled Java coders can prepare themselves for this daunting process and better arm themselves with the knowledge and interviewing skills necessary to succeed.
- ISBN-10 1118722868
- ISBN-13 978-1118722862
- Edition 1st
- Publisher Wrox
- Publication date February 7, 2014
- Language English
- Dimensions 7.38 x 0.87 x 9.25 inches
- Print length 384 pages
- See all details

Editorial Reviews
From the inside flap.
Ace the interview and get the Java programming job you deserve
Java Programming Interviews Exposed is written to specifically prepare you for the questions you’ll face when interviewing for highly sought-after jobs in Java. Expert interviewer and Java developer Noel Markham has teamed with Interview Zen to provide challenging Java-specific questions and answers compiled from over 20,000 interviews conducted by real-world IT employers. This invaluable resource will prepare you to effectively demonstrate your knowledge of the entire Java ecosystem and overcome common interview missteps. Walk into your next interview with confidence—and walk out with the Java programming job of your dreams.
Java Programming Interviews Exposed:
- Provides over 200 interview questions and solutions taken directly from Java coding position interviews
- Develops three key skill sets for Java job seekers: The Interview Process, Core Java, and Java Components and Frameworks
- Offers an expanded appendix on the Java Virtual Machine (JVM) language, Scala, to impress potential employers
- Grants you access to a companion website that will provide all of the sample code in the book, plus a link to Interview Zen for additional support material and testing
Wrox guides are crafted to make learning programming languages and technologies easier than you think. Written by programmers for programmers, they provide a structured, tutorial format that will guide you through all the techniques involved.
www.wrox.com
Programmer Forums
Join our Programmer to Programmer forums to ask and answer programming questions about this book, join discussions on the hottest topics in the industry, and connect with fellow programmers from around the world.
Code Downloads
Take advantage of free code samples from this book, as well as code samples from hundreds of other books, all ready to use.
Find articles, e-books, sample chapters, and tables of contents for hundreds of books, and more reference resources on programming topics that matter to you.
From the Back Cover
Java Programming Interviews Exposed is written to specifically prepare you for the questions you'll face when interviewing for highly sought-after jobs in Java. Expert interviewer and Java developer Noel Markham has teamed with Interview Zen to provide challenging Java-specific questions and answers compiled from over 20,000 interviews conducted by real-world IT employers. This invaluable resource will prepare you to effectively demonstrate your knowledge of the entire Java ecosystem and overcome common interview missteps. Walk into your next interview with confidence--and walk out with the Java programming job of your dreams.
About the Author
Noel Markham is an experienced interviewer and Java developer with experience across technology, financial, and gaming industries. Most recently he has been working in startups for social gaming and digital entertainment. He has hosted interviews for developers of all experience levels, from recent graduates to technical leaders.
Product details
- Publisher : Wrox; 1st edition (February 7, 2014)
- Language : English
- Paperback : 384 pages
- ISBN-10 : 1118722868
- ISBN-13 : 978-1118722862
- Item Weight : 1.35 pounds
- Dimensions : 7.38 x 0.87 x 9.25 inches
- #613 in Software Design & Engineering
- #731 in Java Programming
- #1,826 in Computer Programming Languages
About the author
Noel markham.
Discover more of the author’s books, see similar authors, read author blogs and more
Customer reviews
Customer Reviews, including Product Star Ratings help customers to learn more about the product and decide whether it is the right product for them.
To calculate the overall star rating and percentage breakdown by star, we don’t use a simple average. Instead, our system considers things like how recent a review is and if the reviewer bought the item on Amazon. It also analyzed reviews to verify trustworthiness.
- Sort reviews by Top reviews Most recent Top reviews
Top reviews from the United States
There was a problem filtering reviews right now. please try again later..

Top reviews from other countries

- Amazon Newsletter
- About Amazon
- Accessibility
- Sustainability
- Press Center
- Investor Relations
- Amazon Devices
- Amazon Science
- Sell on Amazon
- Sell apps on Amazon
- Supply to Amazon
- Protect & Build Your Brand
- Become an Affiliate
- Become a Delivery Driver
- Start a Package Delivery Business
- Advertise Your Products
- Self-Publish with Us
- Become an Amazon Hub Partner
- › See More Ways to Make Money
- Amazon Visa
- Amazon Store Card
- Amazon Secured Card
- Amazon Business Card
- Shop with Points
- Credit Card Marketplace
- Reload Your Balance
- Amazon Currency Converter
- Your Account
- Your Orders
- Shipping Rates & Policies
- Amazon Prime
- Returns & Replacements
- Manage Your Content and Devices
- Recalls and Product Safety Alerts
- Conditions of Use
- Privacy Notice
- Consumer Health Data Privacy Disclosure
- Your Ads Privacy Choices
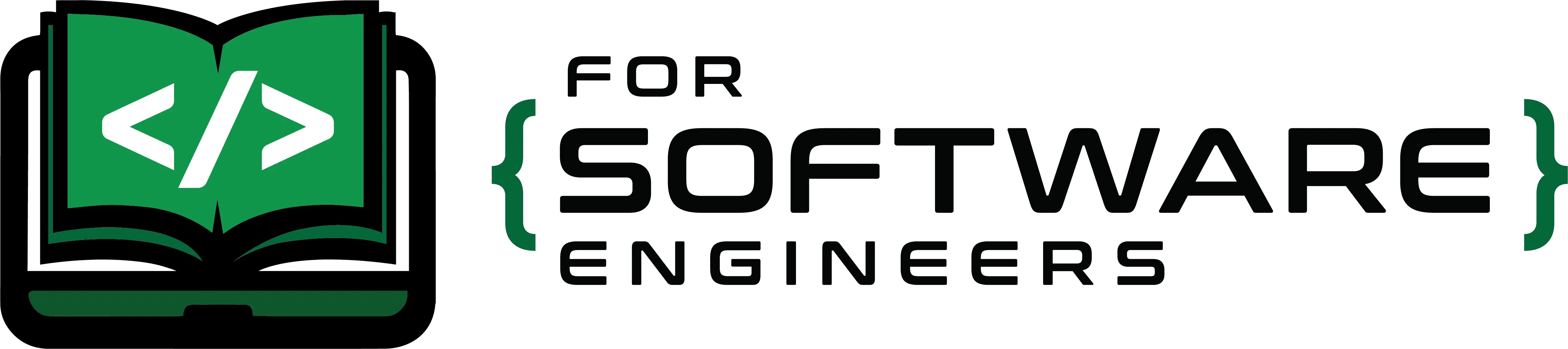
17 Best Coding Interview Books (For Beginners) [2023]
Over the past 5 years, I’ve interviewed for various levels of software engineering positions from intern, new-grad, entry-level, and then mid-level software engineer. Through reading coding interview books, I’ve learned what’s necessary to pass interviews and get job offers at companies like Capital One, Google, and my current company Indeed.
In this article, I’ll be going over my recommended books to get better at coding interviews. These books cover not only the coding interview itself, but also everything the coding interview entails, such as coding fundamentals, mindset, psychology, and preparation habits.
You definitely don’t need to read all of these books to pass your coding interviews. You only need to read what’s relevant to your own personal circumstances, so I hope the notes I left on each book are immensely helpful in allowing you to make a decision on what books to read.
What Are The Best Coding Interview Books?
- Cracking The Coding Interview
- Elements of The Programming Interview
- A Common-Sense Guide to Data Structures and Algorithms, Second Edition
- Introduction to Algorithms, 4th Edition
- Grokking Algorithms
- Programming Interviews Exposed
- Design Patterns: Elements of Reusable Object-Oriented Software
- Clean Code: A Handbook of Agile Software Craftsmanship
- Think Like a Programmer: An Introduction to Creative Problem Solving
- Python Crash Course: A Hands-On, Project-Based Introduction To Programming
- Learn Java The Easy Way: A Hands-On Introduction To Programming
- C++ Crash Course: A Fast-Paced Introduction
- Master Your Emotions: A Practical Guide to Overcome Negativity and Better Manage Your Feelings
- Atomic Habits: An Easy & Proven Way to Build Good Habits & Break Bad Ones
- How To Win Friends and Influence People
- Relentless: From Good to Great to Unstoppable
1. Cracking The Coding Interview
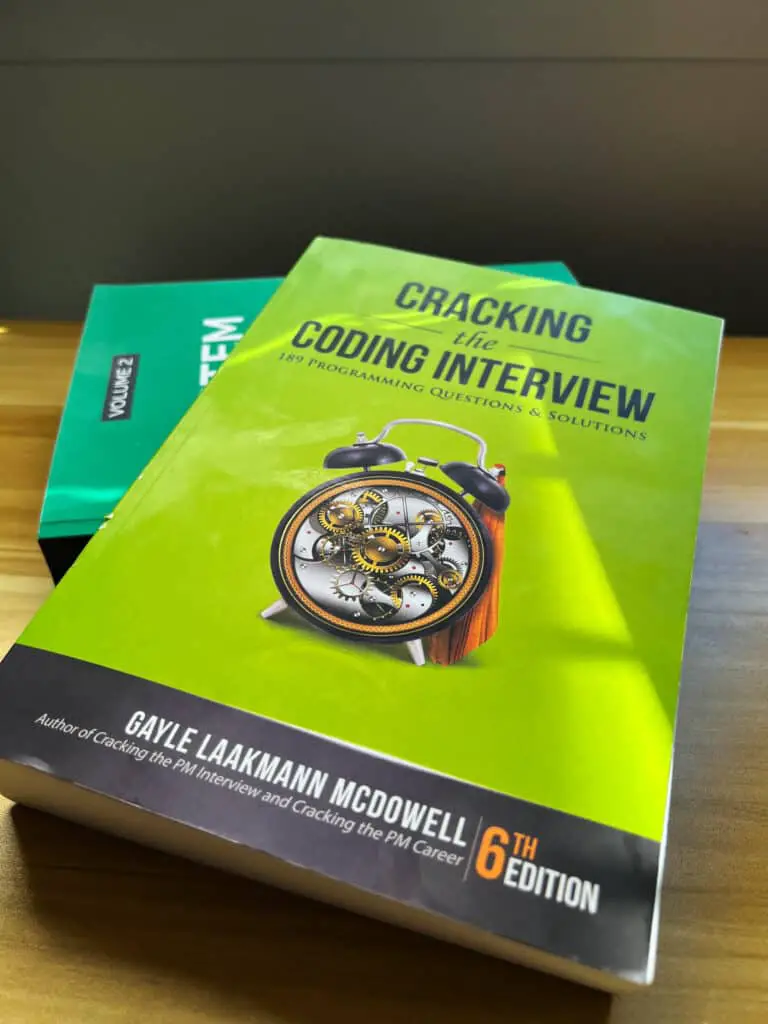
Cracking the Coding Interview is considered the technical interview bible by many software engineers. It covers a lot of topics from what to expect before, during, and after a technical interview.
It also has some good, long, and descriptive sections on preparing for the most popular types of interview problems, which is great for beginners.
Top Features
When I was starting out with interview preparation as a college student and had no idea about what to expect during interview loops at tech companies, Cracking the Coding interview was my compass to navigate the tech interview scene.
If you’re brand new to coding interviews and the technical interview process, this book is perfect for you. Specifically, I’d recommend this book to aspiring software engineering interns, new-graduates, and entry-level software engineers.
2. Elements of The Programming Interview
Elements of the Programming Interview is a book that primarily covers a broad range of types of coding interview questions that would be asked during a real technical interview.
If you’re looking for a comprehensive book that covers coding interview problems specifically, then this is the perfect boot for you.
It covers more problems than Cracking the Coding Interview and has many figures and variations given which are useful for knowing what to expect during coding interviews.
3. A Common-Sense Guide to Data Structures and Algorithms, Second Edition
This is a book that gives an overview of common data structures and algorithms that can be applied to coding interview problems.
If you’re shaky on the fundamentals of data structures and algorithms to the point where you are struggling to complete the easiest coding interview practice problems, then this book might be for you.
It’s specially tailored toward beginners and is very verbose in its explanations.
4. Introduction to Algorithms, 4th Edition
This book, Introduction to Algorithms (also known as CLRS), provides you with a very deep understanding of algorithms and time complexity. Many people argue that this book goes into algorithms too deeply, but for certain job roles in software engineering, it’s necessary to understand algorithms on a deeper level.
If you’re pressed for time and are applying for Fortune 500, startups, and other big tech companies, you might be able to skip reading this book.
Only certain companies like Google may expect you to understand algorithms on a deeper level for their interviews, and that’s why I read through many of this book’s sections before I applied to Google (and eventually received an offer).
If you’re applying for roles in finance that deal with trading and or quantitative finance, then this book may actually be necessary to read.
5. Grokking Algorithms
If you’re a beginner to algorithms and want a basic book to introduce you to all the most important concepts of algorithmic problem solving, then Grokking Algorithms could be the book for you.
Personally, I’ve never used this book, but I read that it was useful for some other people online who couldn’t dive into books like Introduction To Algorithms (aka CLRS).
I would imagine that this book is especially good for people who come from a non-traditional tech background like bootcamp grads who need a little bit more guidance before diving into the heavier data structure and algorithm interview prep books.
6. Programming Interviews Exposed
This book is similar to Cracking The Coding Interview because it teaches you everything you need to know from start to finish for the entire technical interview process.
While this book and Cracking The Coding Interview both try to help you pass your technical interviews, they both provide different angles and perspectives to doing so. That’s why this book might be worth a read instead of or in addition to Cracking The Coding Interview .
If the book Cracking The Coding Interview is difficult to understand for you or you want more insights into the interview process to best prepare yourself, this book is worth a read as well even if you do skip some of the problems.
This book is another primer for the entire coding interview process that may click more than other books.
7. Design Patterns: Elements of Reusable Object-Oriented Software
This book teaches you about the common patterns that are used within Object-Oriented software design (which is typically preferred within the current industry and in interviews).
Coding interviews will sometimes have low-level system design interviews disguised as regular coding interviews. During these low-level design system design interviews, you’ll be expected to know elements of the design patterns discussed within this book.
If you’re trying to be a competitive candidate across big tech, then this book might be worth checking out. However, it’s probably more important to study coding interview-specific topics like data structures and algorithms if you’re pressed for time.
8. Clean Code: A Handbook of Agile Software Craftsmanship
Clean Code is a book about the principles, patterns, and practices of writing clean code. While this is primarily helpful in developing complete systems, lessons from the book can also be applied to the coding interview.
I think that this book is definitely worth it for aspiring and new software engineers to read through. Many of the principles of clean coding aren’t taught in a bootcamp or in college, and clean code has practical applications beyond just the coding interview.
While this book will help you learn to code cleanly for coding interviews (which is a skill worth some points during the interview), it’s not an absolute must-have for the purposes of coding interviews but a great supplemental book.

9. Think Like a Programmer: An Introduction to Creative Problem Solving
This book provides you with a light introduction to general programming problem-solving. This book might be nice to read before jumping into a coding interview-specific book where you’re expected to already understand creative problem-solving in programming.
- Covers many programming puzzles and problems to improve your problem-solving
- Many practical topics are covered like arrays, pointers, classes, and recursion
- Written for beginners to understand
If you’re already comfortable with programming, you might be able to skip this book. Otherwise, if you need an easy-to-read book on problem-solving before attempting to read and solve harder interview book problems, then this book could be for you.
10. Python Crash Course: A Hands-On, Project-Based Introduction To Programming
This book teaches you to use Python to create projects and applications.
The reason some people struggle with coding interviews is that they don’t have strong control over a programming language, and this book could help you get enough strength in Python to pass interviews.
- Popular Python libraries and tools are covered (useful for an actual role that uses Python)
- Introductory Part 1 teaches you everything you need to know about Python before jumping into projects to get you comfortable
- Exercise / practice-driven learning helps you retain the information in the book better
If you are an absolute beginner who is trying to learn enough to pass coding interviews, you have to start with learning a language. I personally would recommend Python as an interview language because of how productive it is (you can do more with less code).
This book could help you learn Python to the point you’ll be comfortable with the programming language to start doing interview problems later.
11. Learn Java The Easy Way: A Hands-On Introduction To Programming
This book teaches you to use Java through creating projects and applications.
The reason some people struggle with coding interviews is that they don’t have strong control over a programming language, and this book could help you get enough strength in Java to pass interviews.
- Heavily project-driven, meaning you get a lot of practice out of the book
- Teaches you to create GUI and Android applications
- Contains additional programming challenges to further challenge yourself to become a better Java programmer
If you are an absolute beginner who is trying to learn enough to pass coding interviews, you have to start with learning a language. While I would initially recommend Python because of how productive the programming language is (less code for more work done), if you already have a basic understanding of Java, this book could help you fill in your knowledge gaps.
This book could help you learn Java to the point you’ll be comfortable with the programming language to start doing interview problems later.
12. C++ Crash Course: A Fast-Paced Introduction
This book teaches you to use C++ to create projects and applications.
The reason some people struggle with coding interviews is that they don’t have strong control over a programming language, and this book could help you get enough strength in C++ to pass interviews.
- Teaches you everything from the fundamentals of the C++ programming language, to popular libraries, and popular frameworks
- The information within the book can be supplemented with additional exercises provided on the book’s website (source code and more included)
- While “pitched” as fast-paced, it’s really geared toward beginners and slow-paced enough for beginners to fully understand everything being discussed
If you are an absolute beginner who is trying to learn enough to pass coding interviews, you have to start with learning a language.
While I would initially recommend Python because of how productive the programming language is (less code for more work done), you might need to learn C++ if you’re applying to trade firms, quant jobs, or roles that require lower-level programming languages.
This book could help you learn C++ to the point you’ll be comfortable with the programming language to start doing interview problems later.
13. Master Your Emotions: A Practical Guide to Overcome Negativity and Better Manage Your Feelings
This book provides you with a lot of insight and wisdom into controlling your emotions.
I think this is very practical for coding interviews where you’ll find yourself in high-pressure situations and possibly face anxiety in waiting for decisions.
- 31 ways to cope with negativity (in a positive way)
- New perspectives on how to view and manage your emotions throughout your day-to-day life
- A downloadable workbook for accountability
Everyone focuses on LeetCode, data structures, and algorithms for coding interview preparation, but sometimes you need to focus on your own psychology (in my personal opinion).
If you’re a person who struggles in high-pressure situations, with anxiety, and/or negative emotions, this book could be good for you to read to learn to manage your emotions.
It only takes one bad interview within an onsite coding interview loop to jeopardize your potential offer at the end (not to give you more anxiety), but even if you have a bad performance on one interview you don’t want that to carry on into the rest of your interviews for that company and future company interviews.
14. Atomic Habits: An Easy & Proven Way to Build Good Habits & Break Bad Ones
This book shows you how to create good habits and destroy bad habits. As simple of a skill as this seems, it’s especially important when preparing for coding interviews.
It doesn’t matter how good your preparation materials are if you’re unable to make habits to utilize them to their full potential.
- Teaches you how to overcome the lack of motivation and willpower
- Gives you practical steps to creating atomic habits that’ll stick with you for as long as you need them to
- Describes how to create an environment for your success
- Shows you how to get back on track when you fall off
I’ve personally read this book (well, technically listened through Audible), and I highly recommend it to people who have issues with creating habits and sticking to them for long periods of time.
This is one of the soft skills that I think is necessary to prepare for coding interviews, but it’s a skill that’ll help you beyond the coding interview as well. The skill of creating good habits and breaking bad habits doesn’t only apply to your career, it also applies to your life as a whole.
15. How To Win Friends and Influence People
This book is about learning to influence people around you to do things you want them to do. It’s a lot less manipulative than how that sounds.
This book is regarded as a timeless piece of non-fiction that I still reread every now and then.
- Includes practical ways to make people like you
- Includes practical ways to change people’s ideas of you without resentment
- Includes practical ways to shift people’s ways of thinking to yours
During a coding interview, you might think that you’re being evaluated solely on your code and thought process.
A bigger part of the interview is making sure you’re leaving a good mark on the interviewer to the point they’ll want to write a great evaluation of you after your interview is over. To do this, you’ll need to be able to persuade them to want to write you the best evaluation they can regardless of your interview performance.
Persuasion and influencing is a soft skill that transcends beyond the coding interview but can definitely be useful for the actual coding interview itself. For that reason, I strongly recommend this book.
16. Deep Work
This book highlights the importance of doing cognitively demanding work in a completely undistracted way. It also highlights ways of achieving time to do deep work within a busy schedule.
- You will be taught to learn to work deeply, along with its benefits
- You will learn how to focus in an increasingly distracting world
When you’re preparing for coding interviews, from my experience, it requires your undivided attention to study and practice through coding interview problems.
This book can aid you in setting up a proper environment to study. At the very least, this book will change your perspective on doing cognitively demanding work with your complete focus or undivided attention.
17. Relentless: From Good to Great to Unstoppable
This book is about the mindset for becoming from good to great in any aspect of your life.
It’s a book by a trainer who coached various top-performing athletes like Michael Jordan, Kobe Bryant, and Dwayne Wade who highlights lessons in what’s necessary to achieve great feats.
- Teaches you the elite athlete mindset which is necessary to crack the most competitive interviews
Sometimes people think they need motivation or willpower to study for coding interviews. This book is essentially about the mindset that’s necessary to become an elite athlete, but its lessons span to anything else competitive.
If you’re serious about preparing for coding interviews and giving it your all, this book could be perfect for you. This could especially be the case if you’ve never participated in competitive activities because this book could offer you a unique perspective.
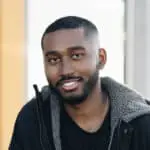
Joe Onifade is an ex-Google software engineer, and over the past 3 years, he's worked as a full-stack software engineer but becoming a backend-focused engineer. His programming interests span developing scalability systems and web3.
Similar Posts
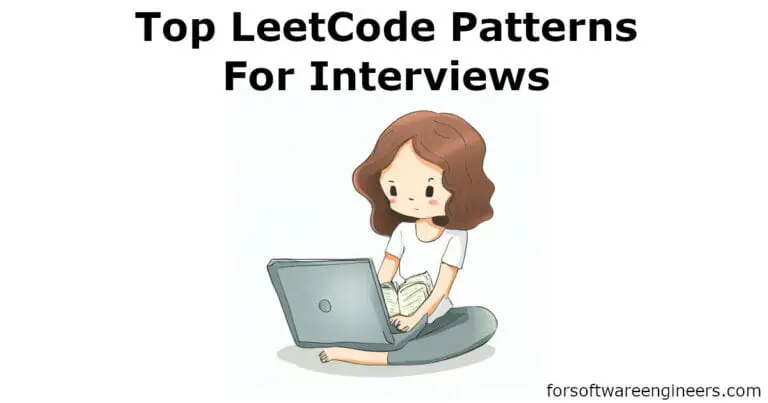
8 Common LeetCode Patterns To Pass Coding Interviews
LeetCode patterns are common ways of approaching an optimal solution to a coding question on the LeetCode platform. The patterns vary in complexity to understand and apply and are important to know in order to solve a greater breadth of LeetCode questions efficiently and quickly. These patterns also apply to the coding interview questions that…
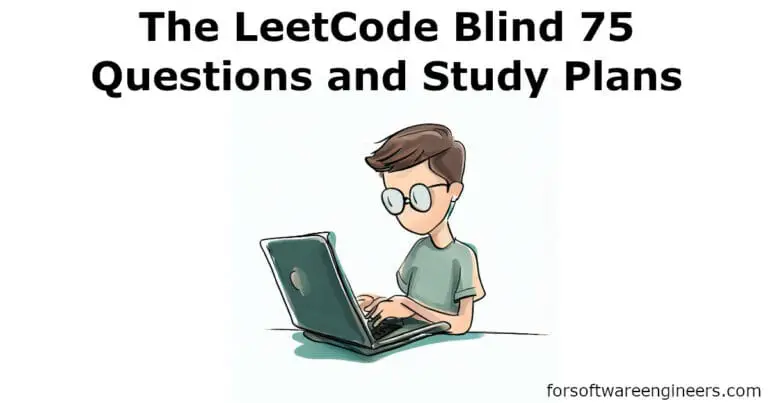
LeetCode Blind 75 (Grind 75): What It Is, Questions, and Study Plan
LeetCode (abbreviated LC) is a platform that software engineers use to practice coding problems (called LeetCode questions or LeetCode problems) for coding interviews. The Blind 75 (also called the LeetCode Blind 75) is a focused list of curated LeetCode problems that cover the most common types of problems and patterns that are asked within real…
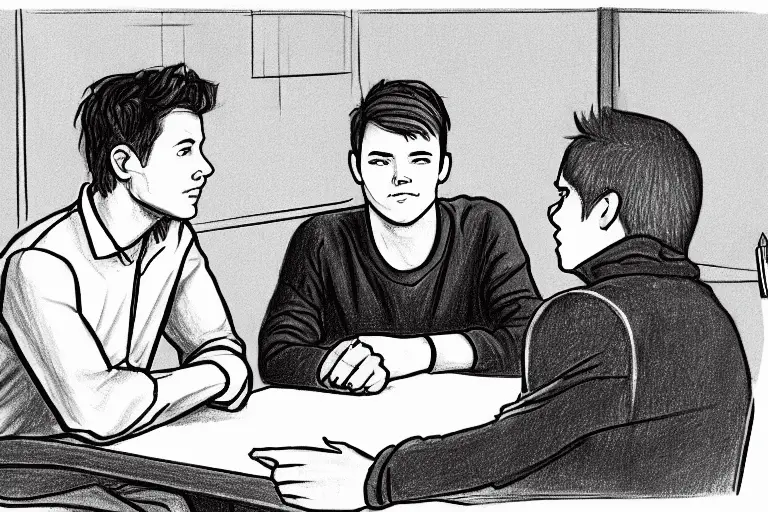
6 Things To Do On The Day of a Coding Interview?
So you’ve finally made it to the day of your technical interviews. You’ve prepped for this day for a long time, putting in a massive amount of effort (maybe in addition to a full-time job). So, what should you do on the day of your coding interview? Should you grind through some more LeetCode questions…
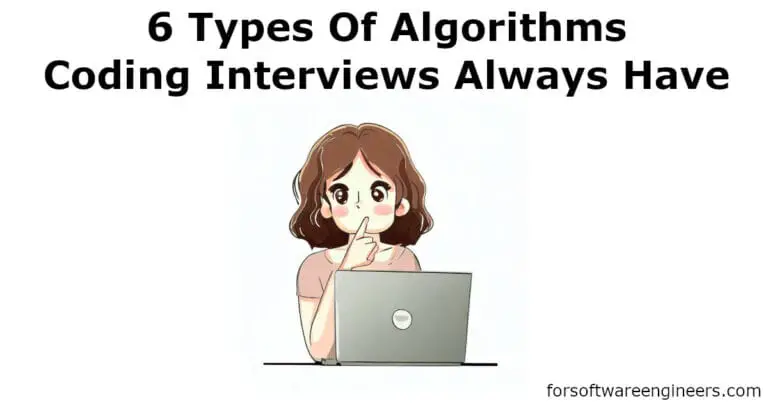
6 Essential Types Of Algorithms For Coding Interviews
Algorithms are a set of steps or instructions that are designed to perform a specific task or action, primarily to solve a problem. Your ability to create correct and efficient algorithms is evaluated within software engineer coding interviews because companies like Google, Amazon, and Microsoft want to hire software engineers who deeply understand algorithms. If…
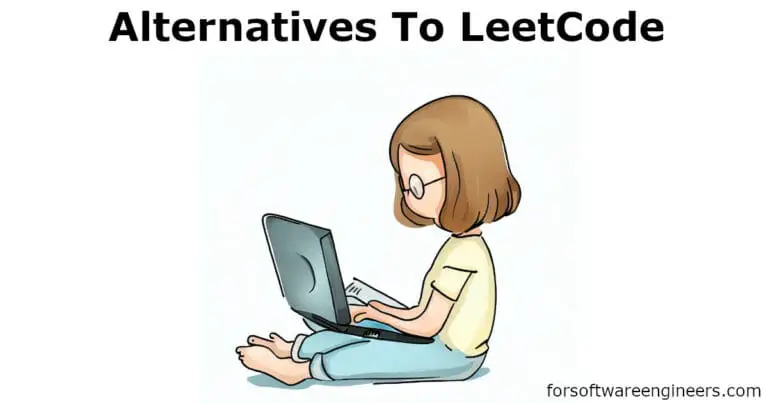
7 Best LeetCode Alternatives For Interview Preparation
A LeetCode alternative for interview preparation refers to an online platform or resource designed to help software engineering candidates prepare for technical interviews, particularly focusing on coding challenges and algorithmic problems. These alternatives often provide a curated collection of coding questions that cover essential topics like data structures, algorithms, and problem-solving techniques commonly encountered in…
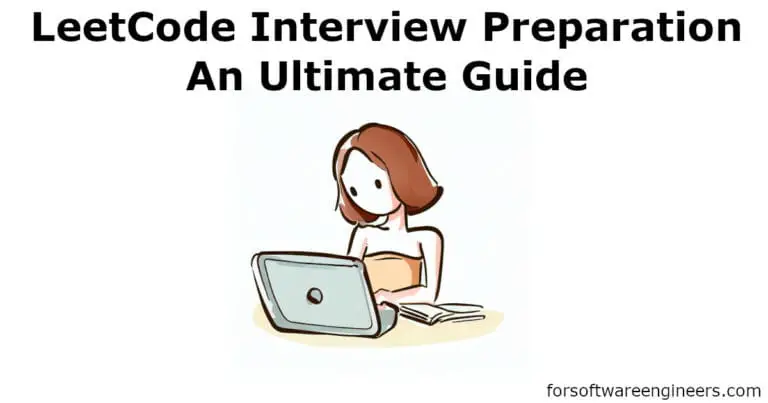
LeetCode For Interview Preparation: Everything To Know
LeetCode (LC) is a popular online platform that software engineers use to practice coding interview problems and prepare for software engineer technical interviews. LeetCode provides thousands of questions, each tagged with relevant information such as their difficulty and the types of companies that ask questions like it. Additionally, there is a strong community on LeetCode…

The Best Book for Technical Coding Interview Prep

Technical coding interviews are notoriously difficult — almost borderline quiz-like for those unprepared. It can sometimes be a daunting task to navigate all the technical coding preparation resources available online, and one might ask: is there a single book/resource that best prepares for technical coding interviews?
Best , is relative of course in this case. If you’re looking for a book that will teach you how to solve any coding question, guides you on how to design something like Instagram that scales to a billion users, and makes your sandwich while you’re at it, then this book definitely isn’t it.
But for polishing your technical chops and making sure you’re well prepared to face the programming challenges in an interview, this book comes darn close to it.
In today’s book review, I go over in detail the good and the bad of one of the top-selling books on Amazon for technical coding interviews: Elements of Programming Interviews (a.k.a EPI , and it’ll be referred to as such in the rest of this article).

What is this book about?
EPI is a book that focuses on preparing for technical software engineering interview questions that are commonly asked at tech companies. If you’re looking for a position as a software engineer, this book will have something for you.
EPI was written by 3 folks who’ve worked across some of the best-known tech companies in the world, the likes of Facebook, Google, Uber, and Microsoft. The authors have very strong technical backgrounds, and this is evidenced by the technical focus in the contents of the book.
The book itself is highly technical in nature and deep dives into fundamental computer science. The types of technical coding questions vary widely from string manipulation to graph traversals. The book also offers concrete tips and tricks for solving specific types of coding problems, and includes a cheatsheet to help ace technical questions.
In short: EPI is a highly technical book that is well-suited for anyone looking to prepare for technical coding questions that revolve around data structures and algorithms.
The things that I particularly enjoyed:
- has many questions (300+) with detailed answers, compared to another popular book (Cracking The Coding Interview) that only has 170+
- comes in 3 different versions: Java, Python and C++. Suitable for people who want to use the language of their choice
- has a great cheatsheet (Chapter 4) that summarizes all the techniques and data structures you’d need
- covers in detail every single data structure you need, algorithms you should know, and techniques you should know in a simple, understandable format
- covers relevant technical topics you might get asked in a coding interview, like what is TCP/IP, how the Internet works, and how to capture relationships between different entities in schema design
Those are some of the top-level points I enjoyed about the book. What I really enjoy is that the answers are very, very well constructed.
The answers often take a brute force/simplistic approach to the problems. The authors then provide concrete examples of how you can improve on the brute force approach. With each iteration, the authors point out where the limitations are and how you can solve them with incremental optimizations.
For example, EPI explains how to detect a cycle in a linked list. The first approach: use a hash map to store every node you traverse, and then check against the hash map to see if you’ve seen it. This does the job, albeit it takes additional space (so-called O(N) space complexity and O(N) time complexity).
The book then points out that the additional space is not required, and suggests that detecting cycles in a linked list can be done without additional space by manipulating the pointers instead.
It takes that same approach in explaining almost all the questions, so everyone can understand how to get from a rough solution to an optimized one.
For someone who’s rusty and looking to start preparing for interviews, EPI does a great job — every chapter starts off with a summary of the data structure or algorithm that you will learn, why the data structure is special, what are the strengths/weaknesses of said data structure and what you need to take note of.
In short, EPI is the notebook I wished I had when I was preparing for technical interviews. This is the book I’d give a friend who’s looking to prepare for technical interviews — aside from my best-selling interviewing course (Acing The Tech Interview), which prepares any candidate for the entire interview experience from resume preparation, behavioral questions to how to solve coding problems creatively. Classes fill up fast, so book your slot here today.
EPI has its downsides as well. Being a highly technical book, this means the book has its focus set on coding questions.
This, inadvertently, means that the book falls short in terms of other aspects of the technical interview process which are, arguably, just as important — offer negotiation, how to write a persuasive resume, what to do when you get an offer, how to handle behavioral questions etc.
In addition, the book goes into deep detail with data structures and algorithms, but only provides a high-level overview, to the point of being confusing due to lack of context, of various other important concepts like SQL, NoSQL, how to design a proper schema for a simple application and more.
The book sort of covers SQL design by explaining how to structure tables to capture entity relationships, but it does not explain more about JOINs or how to query the tables properly. I think understanding the what (SQL tables) is just as important as the how (queries).
EPI also has a chapter on systems designs, but the chapter does not cover distributed designs. I would’ve liked it better if the book had described in more details what distributed systems are or where to find more information about these.
Is this a good book for me?
If you have ~$40 to spare and a big appetite to learn, I’d say so.
The book sells for $36 on average (there are 3 versions). This book is highly rated on Amazon.com, with an average of 4.53 stars across all 3 versions. The stats are as follows:
On Amazon.com :
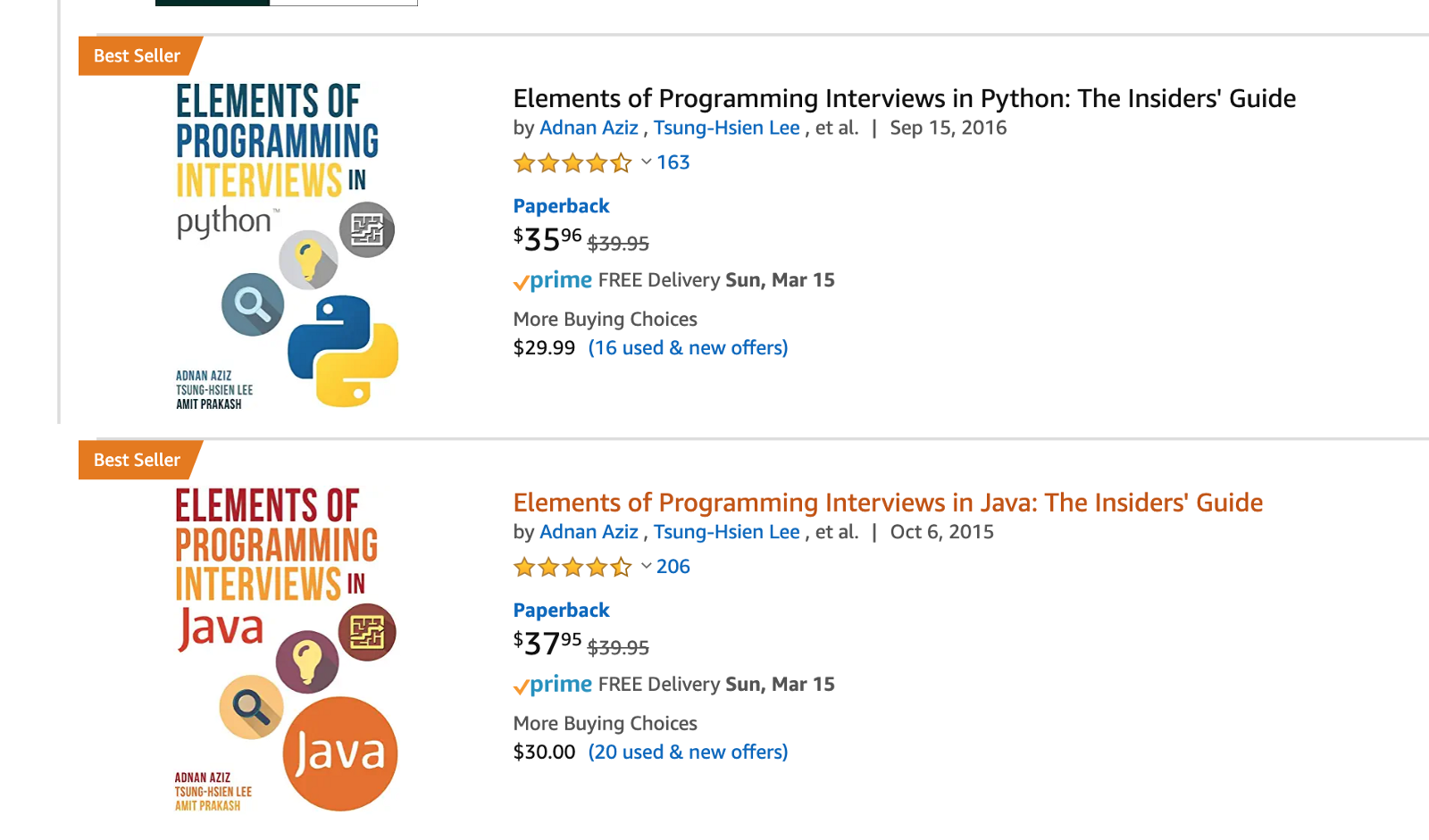
3 versions (as of March 12):
- Python: 169 ratings 4.3 ave
- Java: 203 ratings 4.6 ave
- C++: 436 ratings 4.7 ave
In aggregate, there were a total of 808 reviews.
Some of the reviews:
“.. the best algorithm/data structure I ever took”
“the code is of high quality, using meaningful variable names”
“this is a must-have book”
Now, should you buy it?
I think that EPI is one of the best resources to prepare for technical coding interviews, bar none. Regardless of your experience level, there’s something in there for you. I particularly enjoy reading the book for its clear and concise explanations, and I use this book as a reference in my programming course.
If you want all the data structures, algorithms you need to know in a single, easy-to-read book, then EPI is the book for you.
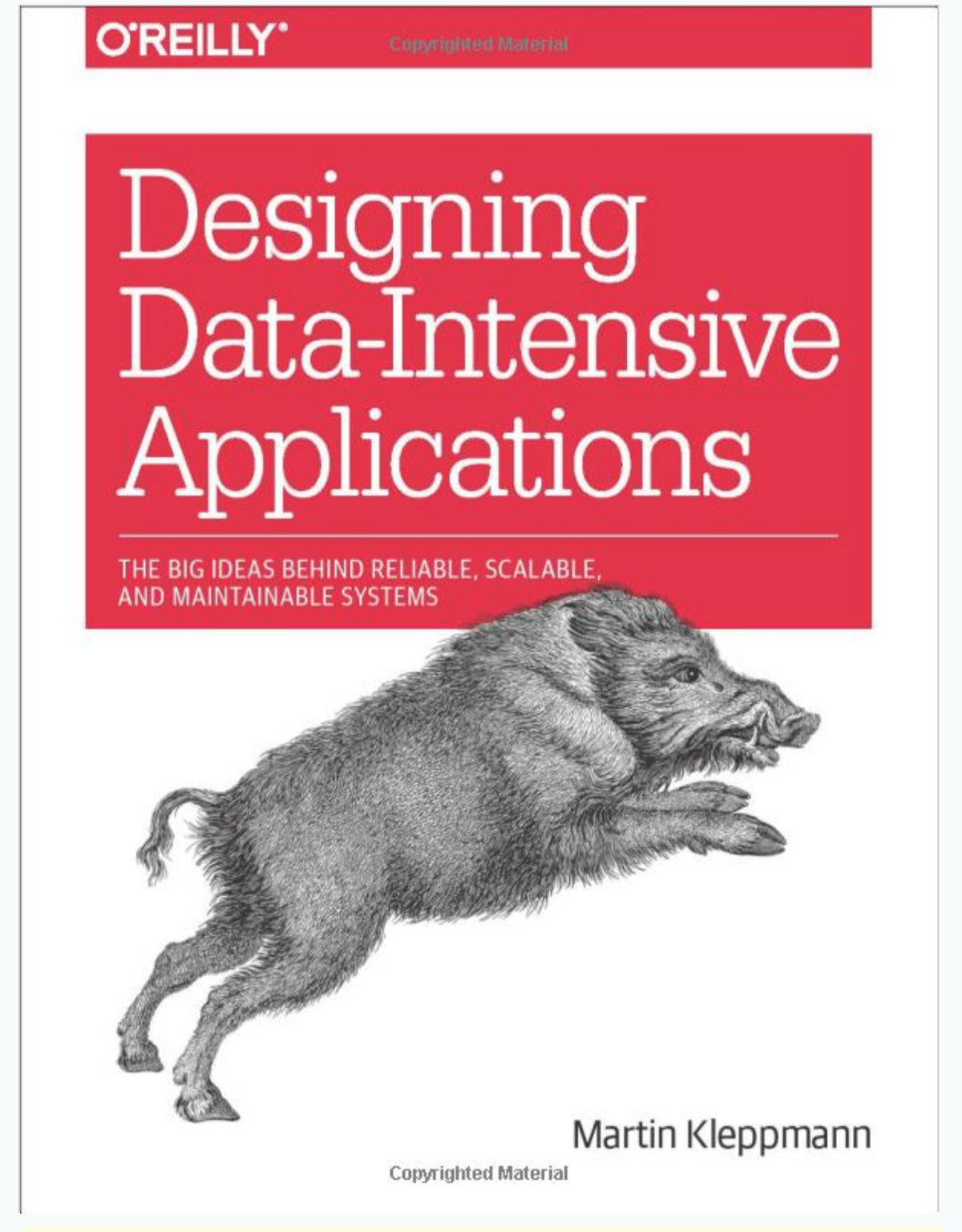
For distributed systems designs, I recommend looking elsewhere. A good start is Designing Data Intensive Applications .
I love it for the depth and insights, and I recommend it to many students who are taking my courses as well.
Resources I Recommend
Designing Data Intensive Applications — great resource for learning about distributed systems and how large-scale systems work.
Acing The Technical Interview — my best-selling personal coaching course on how to ace technical interviews. We cover resume review, technical coding interview, behavioral questions and more.
Acing The Distributed Systems Design Interview — my personal coaching course on designing large scale distributed systems. Learn about how to design features like Instagram Stories, Groupon, movie streaming sites like Netflix at scale.
Software engineer @Facebook. Ex-Twitter. Helping others get into tech.
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- SAT Exam Preparation 2024: Best Preparation Tips along with Resources
- Top 5 SAT Preparation Books To Score 1400+
- 60 Day Preparation Plan to Score Above 90% Marks in CBSE Class 10 Exam 2024
- Top 20 Design Patterns Books of All Time from Beginner to Experts
- Top 7 Study Tips For Class 12 Science Students To Prepare For CBSE Board Exam 2023-24
- Best Books for UPSC Prelims & Mains 2024
- UPTET Exam 2024: Notification, Registration, Exam Pattern, Result
- JEE Main Chemistry Syllabus 2024 Released: Download Free PDF, Check Topic Wise Syllabus, Preparation Books
- SAT Exam Maths Syllabus 2024: Important Topics & Preparation Tips
- List of Books for Government Exam 2024 (Famous book for all competitive Exams)
- How to Score Above 90% in CBSE Class 10 Exams 2024
- 10 Best UPSC Preparation Strategy (IAS Exam)
- CAT 2019 Preparation Strategy : How to Ace the CAT Exam in 4 Months
- Best Books to Prepare QA, VARC and DILR For CAT 2021
- Tips to Clear GATE CS Exam [2024]: Road to Success
- Best JEE Main Physics Books 2024: Tips to Prepare for Physics Section (Mains and Advanced)
- Which Book Is Best For IIT JEE Preparation?
- SBI PO English Syllabus 2024: Exam Pattern, Preparation Tips, PDF Download
- Which book is best for preparation of chemistry for JEE main?
List of Top TOEFL Books For Preparation | Best Books & Resources 2024
Best Books for TOEFL Exam Preparation : Among all the resources accessible, books play an important role in giving thorough preparation for this difficult exam. Books provide an organized approach to TOEFL study, covering all aspects of the exam in detail.
In this article, we’ll look at the importance of quality study materials in TOEFL preparation and the multiple advantages of including books in your study routine.
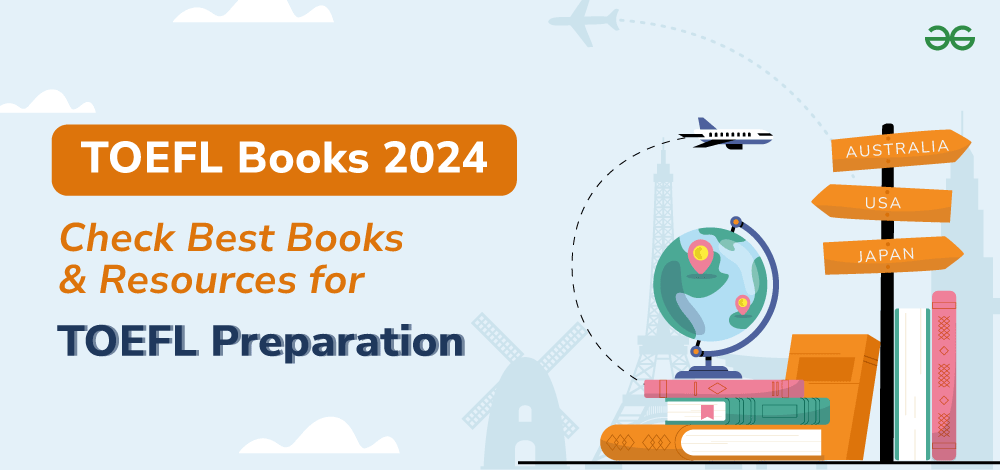
Best Books For TOEFL Exam Preparation
Table of Content
Best TOEFL Books 2024
Top 8 toefl prep books for 2024, kaplan toefl ibt premier, the official toefl ibt tests collection, practice test books for toefl 2024, vocabulary building resources for toefl 2024, toefl preparation tips 2024, toefl sample paper, toefl exam day tips.
To select the most appropriate books and guides, it’s crucial to identify your proficiency level (beginner, expert), specific focus areas (vocabulary, grammar), and study preferences. As the TOEFL exam involves simulated tasks such as listening to audio recordings and speaking with emphasis on accent and pronunciation, relying solely on books may not suffice. It’s essential to utilize software and applications to practice all exam sections comprehensively. Many print materials come with accompanying CDs, eliminating the need for separate software downloads.
1. The Official Guide to the TOEFL Test
Key Features : Developed by ETS, this guide offers authentic practice tests, detailed explanations of test sections, and effective strategies for tackling each question type. It provides comprehensive content coverage and insider tips from the makers of the TOEFL exam.
2. “TOEFL iBT Prep Plus 2024” by Kaplan Test Prep
Key Features : This study guide includes four full-length practice tests with answer explanations, an extensive review of all TOEFL sections, and online resources such as video lessons and practice quizzes. It offers effective strategies for time management and test-taking techniques.
3. “Barron’s TOEFL iBT with Online Tests” by Pamela J. Sharpe Ph.D.
Key Features: Known for its thorough content coverage, this guide includes practice tests with answer explanations, skill-building exercises for all sections, and online resources for additional practice. It provides effective strategies for improving reading, listening, speaking, and writing skills.
4. “Cambridge Preparation for the TOEFL Test” by Jolene Gear and Robert Gear
Key Features : This study guide offers a systematic approach to TOEFL preparation with skill-building exercises, practice tests, and audio material for improving listening and speaking skills. It includes effective strategies for answering TOEFL questions and maximizing scores.
5. “Cracking the TOEFL iBT” by Princeton Review
Key Features : This guide provides comprehensive content review, practice drills, and full-length practice tests with detailed explanations. It offers effective strategies for approaching each section of the TOEFL exam and includes tips for improving language skills.
6. “The Complete Guide to the TOEFL Test: iBT Edition” by Bruce Rogers
Key Features : This comprehensive guide offers in-depth coverage of all TOEFL sections, including practice tests, skill-building exercises, and online resources. It provides effective strategies for improving test-taking skills and maximizing scores.
7. “TOEFL iBT Interactive” by Kaplan Test Prep
Key Features : This interactive study guide combines a comprehensive review of TOEFL content with interactive online resources. It includes practice tests, video lessons, and personalized study plans to tailor your preparation to your specific needs.
8. “Delta’s Key to the TOEFL iBT: Advanced Skill Practice” by Nancy Gallagher
Key Features : Geared towards advanced learners, this guide focuses on refining TOEFL skills through targeted practice exercises and authentic test materials. It offers effective strategies for mastering challenging question types and optimizing performance.
9. “McGraw-Hill Education: Essential TOEFL Vocabulary” by Diane Engelhardt
Key Features : This vocabulary guide focuses on building essential TOEFL vocabulary through word lists, exercises, and contextual examples. It offers strategies for memorization and application of vocabulary in various TOEFL question types.
10. “TOEFL Strategies and Tips: Winning Strategies for the TOEFL iBT” by Bruce Stirling
Key Features : This guide provides winning strategies and tips for every section of the TOEFL iBT exam. It includes practical advice, sample responses, and effective techniques for maximizing scores.
Following are the top 8 TOEFL Prep Books for 2024:
- “The Official Guide to the TOEFL Test” by ETS
- “TOEFL iBT Prep Plus 2024” by Kaplan Test Prep
- “Barron’s TOEFL iBT with Online Tests” by Pamela J. Sharpe Ph.D.
- “Cambridge Preparation for the TOEFL Test” by Jolene Gear and Robert Gear
- “The Complete Guide to the TOEFL Test: iBT Edition” by Bruce Rogers
- “TOEFL iBT Interactive” by Kaplan Test Prep
- “Delta’s Key to the TOEFL iBT: Advanced Skill Practice” by Nancy Gallagher
- “McGraw-Hill Education: Essential TOEFL Vocabulary” by Diane Engelhardt.
This guide is considered by many TOEFL experts as a rock-solid introduction to all sections of the test. Some even claim it is better than the official guide in some aspects. It includes four practice tests, along with online and mobile resources. It always makes it to the list of highly recommended books for TOEFL.
The Official TOEFL iBT Tests collection comprises authentic practice tests developed by ETS, the creators of the TOEFL exam. Each test mirrors the format, content, and difficulty level of the actual TOEFL exam, providing invaluable preparation for test-takers.
- Authentic Practice : Experience the real TOEFL exam with practice tests that closely resemble the actual test environment.
- Comprehensive Coverage : Covering all sections of the TOEFL exam – Reading, Listening, Speaking, and Writing – these tests offer a thorough assessment of your skills.
- Detailed Explanations : Access detailed explanations for each question, allowing you to understand why certain answers are correct and others are not.
- Online Resources : Some editions of the Official TOEFL iBT Tests collection include access to online resources, such as audio files for Listening and Speaking sections, to enhance your preparation.
- Effective Preparation : By practicing with authentic TOEFL exam materials, you can familiarize yourself with the test format, improve your test-taking strategies, and boost your confidence.
- Accurate Assessment : The Official TOEFL iBT Tests collection provides an accurate gauge of your current skill level, helping you identify areas for improvement and track your progress over time.
Realistic exam simulation is essential for effective TOEFL preparation as it familiarizes test-takers with the exam format, timing, and question types. Practice test books offer invaluable opportunities to experience the actual TOEFL exam environment, allowing test-takers to assess their skills, identify areas for improvement, and build confidence.
a. “The Official Guide to the TOEFL Test” by ETS
- Key Features : Includes authentic practice tests developed by ETS, providing a reliable simulation of the actual TOEFL exam.
- Benefits : Offers test-takers the opportunity to experience the format, content, and difficulty level of the real exam, helping in effective preparation.
b. “TOEFL iBT Prep Plus 2024” by Kaplan Test Prep
- Key Features : Contains full-length practice tests with detailed answer explanations, offering comprehensive exam simulation.
- Benefits : Allows test-takers to practice under timed conditions and assess their performance across all sections of the TOEFL exam.
c. “Barron’s TOEFL iBT with Online Tests” by Pamela J. Sharpe Ph.D.
- Key Features : Provides practice tests with answer explanations, along with online resources for additional practice and interactive learning.
- Benefits: Offers a comprehensive approach to TOEFL preparation, including realistic exam simulation and skill-building exercises.
Vocabulary plays an important role in TOEFL success, influencing performance across all sections of the exam, including Reading, Listening, Speaking, and Writing. Vocabulary building resources offer targeted practice and exposure to English words commonly encountered in academic and everyday contexts, enhancing language proficiency and comprehension skills.
a. “Vocabulary for TOEFL iBT” by Ingrid Wisniewska
- Key Features : Presents essential TOEFL vocabulary through organized word lists, exercises, and contextual usage examples.
- Benefits : Offers a systematic approach to vocabulary acquisition, allowing test-takers to strengthen their word knowledge and improve their performance on the TOEFL exam.
b. “McGraw-Hill Education: Essential TOEFL Vocabulary” by Diane Engelhardt
- Key Features : Provides comprehensive coverage of essential TOEFL vocabulary with clear definitions, example sentences, and practice exercises.
- Benefits : Focuses on high-frequency words and phrases commonly tested on the TOEFL exam, helping test-takers build a strong foundation in English vocabulary.
c. “TOEFL Vocabulary Flashcards” by Kaplan Test Prep
- Key Features : Offers a portable and interactive way to study TOEFL vocabulary with flashcards featuring key words, definitions, and example sentences.
- Benefits : Allows for convenient review and reinforcement of vocabulary concepts, ideal for on-the-go study and quick revision.
Preparing for the TOEFL exam involves two main approaches: self-study and attending coaching classes. Both methods offer unique advantages, and neither is inherently superior to the other. If cost is a primary concern, self-study using TOEFL books and resources may be the preferred option. Conversely, if you seek professional guidance to gain an edge in TOEFL exam preparation, enrolling in a TOEFL coaching center could be more beneficial.
The TOEFL test assesses candidates’ English language proficiency at the university level through four sections: reading, writing, listening, and speaking. When preparing for the test, candidates should first familiarize themselves with its format, scoring system, and result interpretation. ETS, the organization conducting the TOEFL exam, offers both free and paid study materials, which are readily accessible.
TOEFL Practice Test: As an assessment of English language proficiency, TOEFL demands candidates to possess a strong grasp of the English language. Like other standardized tests for studying abroad, TOEFL is segmented into sections, each holding its significance and requiring tailored preparation. With a total of four sections, the exam mandates dedicated effort to comprehensively cover and excel in each segment.
Here are some top tips for your TOEFL exam day to ensure optimal performance. On test day, the only essential item you need to bring to the test center is a valid and acceptable ID, which for Indian test-takers is a valid passport—no exceptions are made to this rule. It’s important to note that your passport must meet the following criteria:
- It must be an original document; photocopies are not permitted.
- It must be currently valid; expired documents will not be accepted.
- Your full name on the passport must match the name provided during registration.
- The passport must contain a recent photo of you that matches your appearance on test day.
- Your signature must be present on the passport.
Additionally, remember that no other personal items are allowed inside the test center besides your ID, as they may be subject to inspection or confiscation.
Selecting the best books for TOEFL exam preparation is a critical step towards achieving success on test day. Whether you’re aiming to improve your language skills, familiarize yourself with the exam format, or refine your test-taking strategies, the right resources can make all the difference. The recommended books offer comprehensive coverage of TOEFL content, authentic practice materials, and effective strategies required to meet the needs of test-takers. By incorporating these top-rated books into your study regimen, you can enhance your preparation, build confidence, and maximize your chances of achieving your target score on the TOEFL exam. With dedication, perseverance, and the guidance of these trusted resources, you’re well-equipped to excel on your TOEFL journey and pursue your academic and professional aspirations with confidence.
Also Check:
- List of Entrance Exams to Study Abroad in 2024
- TOEFL Full Form – Eligibility, Exam Pattern, Benefits
Best Books for TOEFL Exam Preparation- FAQs
What are the best books for toefl exam preparation.
The best books include “The Official Guide to the TOEFL Test,” “TOEFL iBT Prep Plus 2024,” and “Barron’s TOEFL iBT with Online Tests.”
What makes these books the top choices?
They offer authentic practice tests, comprehensive content coverage, and effective strategies for TOEFL success.
Can these books help improve my language skills?
Yes, they provide opportunities to enhance English language proficiency through targeted practice and exposure to academic vocabulary.
Are these books suitable for self-study?
Absolutely, these books are designed for self-directed learners and offer structured study plans and resources for independent preparation.
Do these books include online resources?
Some editions may include access to online platforms with additional practice tests, interactive exercises, and video lessons.
Are these books suitable for all proficiency levels?
Yes, they cater to a wide range of proficiency levels, from beginner to advanced, with varying levels of difficulty in practice materials.
Can these books guarantee a high score on the TOEFL exam?
While no book can guarantee a specific score, these top-rated resources provide invaluable support and guidance to help you maximize your potential and achieve your target score.
Please Login to comment...
Similar reads.
- Study Abroad
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Download Interview guide PDF
Spring boot interview questions, download pdf.
Spring boot is the hottest topic of discussion in interviews if it comes to Java Application development. Because of its fast, low configuration, inbuilt server, and monitoring features, it helps to build a stand-alone Java application from scratch with very robust and maintainable.
The article will walk you through the Spring Boot interview questions for basic to advanced levels.
What is Spring boot?
Sprint boot is a Java-based spring framework used for Rapid Application Development (to build stand-alone microservices). It has extra support of auto-configuration and embedded application servers like tomcat, jetty, etc.
Features of Spring Boot that make it different?
- Creates stand-alone spring application with minimal configuration needed.
- It has embedded tomcat, jetty which makes it just code and run the application.
- Provide production-ready features such as metrics, health checks, and externalized configuration.
- Absolutely no requirement for XML configuration.
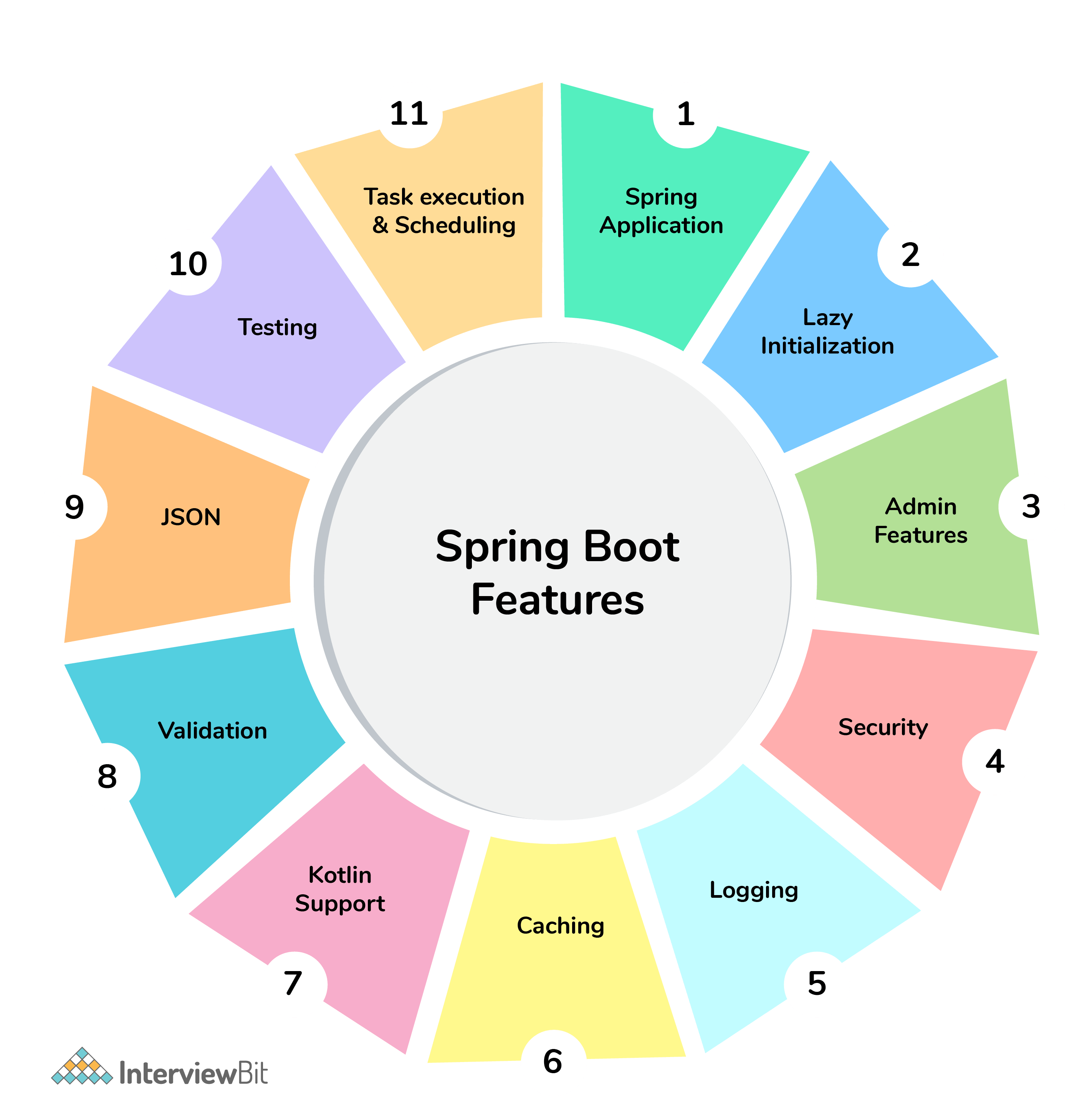
Spring Boot Interview Questions For Freshers
1. what are the advantages of using spring boot.
The advantages of Spring Boot are listed below:
- Easy to understand and develop spring applications.
- Spring Boot is nothing but an existing framework with the addition of an embedded HTTP server and annotation configuration which makes it easier to understand and faster the process of development.
- Increases productivity and reduces development time.
- Minimum configuration.
- We don’t need to write any XML configuration, only a few annotations are required to do the configuration.
2. What are the Spring Boot key components?
Below are the four key components of spring-boot:
- Spring Boot auto-configuration.
- Spring Boot CLI.
- Spring Boot starter POMs.
- Spring Boot Actuators.
3. Why Spring Boot over Spring?
Below are some key points which spring boot offers but spring doesn’t:
- Starter POM.
- Version Management.
- Auto Configuration.
- Component Scanning.
- Embedded server.
- InMemory DB.
Spring Boot simplifies the spring feature for the user:
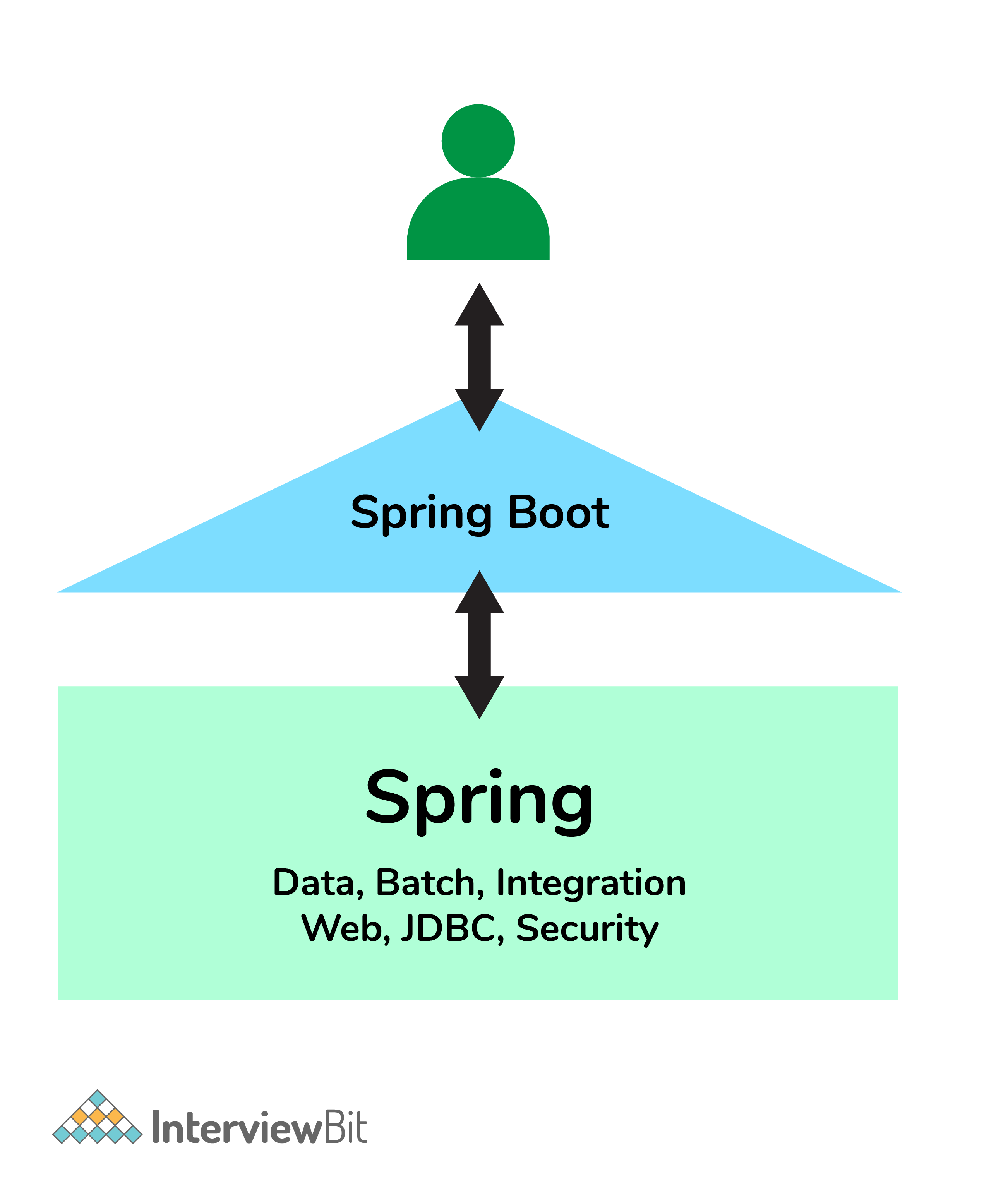
4. What is the starter dependency of the Spring boot module?
Spring boot provides numbers of starter dependency, here are the most commonly used -
- Data JPA starter.
- Test Starter.
- Security starter.
- Web starter.
- Mail starter.
- Thymeleaf starter.
5. How does Spring Boot works?
Spring Boot automatically configures your application based on the dependencies you have added to the project by using annotation. The entry point of the spring boot application is the class that contains @SpringBootApplication annotation and the main method.
Spring Boot automatically scans all the components included in the project by using @ComponentScan annotation.
Learn via our Video Courses
6. what does the @springbootapplication annotation do internally.
The @SpringBootApplication annotation is equivalent to using @Configuration, @EnableAutoConfiguration, and @ComponentScan with their default attributes. Spring Boot enables the developer to use a single annotation instead of using multiple. But, as we know, Spring provided loosely coupled features that we can use for each annotation as per our project needs.
7. What is the purpose of using @ComponentScan in the class files?
Spring Boot application scans all the beans and package declarations when the application initializes. You need to add the @ComponentScan annotation for your class file to scan your components added to your project.
8. How does a spring boot application get started?
Just like any other Java program, a Spring Boot application must have a main method. This method serves as an entry point, which invokes the SpringApplication#run method to bootstrap the application.
9. What are starter dependencies?
Spring boot starter is a maven template that contains a collection of all the relevant transitive dependencies that are needed to start a particular functionality. Like we need to import spring-boot-starter-web dependency for creating a web application.
10. What is Spring Initializer?
Spring Initializer is a web application that helps you to create an initial spring boot project structure and provides a maven or gradle file to build your code. It solves the problem of setting up a framework when you are starting a project from scratch.
11. What is Spring Boot CLI and what are its benefits?
Spring Boot CLI is a command-line interface that allows you to create a spring-based java application using Groovy.
Example: You don’t need to create getter and setter method or access modifier, return statement. If you use the JDBC template, it automatically loads for you.
12. What are the most common Spring Boot CLI commands?
-run, -test, -grap, -jar, -war, -install, -uninstall, --init, -shell, -help.
To check the description, run spring --help from the terminal.
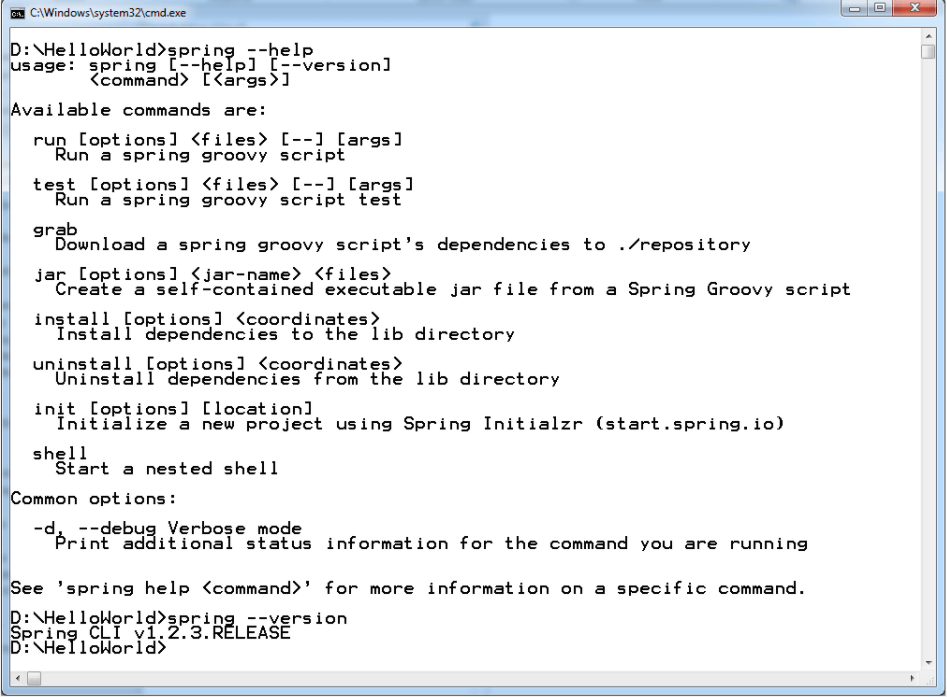
Advanced Spring Boot Questions
1. what are the basic annotations that spring boot offers.
The primary annotations that Spring Boot offers reside in its org.springframework.boot.autoconfigure and its sub-packages. Here are a couple of basic ones:
@EnableAutoConfiguration – to make Spring Boot look for auto-configuration beans on its classpath and automatically apply them.
@SpringBootApplication – used to denote the main class of a Boot Application. This annotation combines @Configuration, @EnableAutoConfiguration, and @ComponentScan annotations with their default attributes.
2. What is Spring Boot dependency management?
Spring Boot dependency management is used to manage dependencies and configuration automatically without you specifying the version for any of that dependencies.
3. Can we create a non-web application in Spring Boot?
Yes, we can create a non-web application by removing the web dependencies from the classpath along with changing the way Spring Boot creates the application context.
4. Is it possible to change the port of the embedded Tomcat server in Spring Boot?
Yes, it is possible. By using the server.port in the application.properties .
5. What is the default port of tomcat in spring boot?
The default port of the tomcat server-id 8080. It can be changed by adding sever.port properties in the application.property file.
6. Can we override or replace the Embedded tomcat server in Spring Boot?
Yes, we can replace the Embedded Tomcat server with any server by using the Starter dependency in the pom.xml file. Like you can use spring-boot-starter-jetty as a dependency for using a jetty server in your project.
7. Can we disable the default web server in the Spring boot application?
Yes, we can use application.properties to configure the web application type i.e spring.main.web-application-type=none.
8. How to disable a specific auto-configuration class?
You can use exclude attribute of @EnableAutoConfiguration if you want auto-configuration not to apply to any specific class.
9. Explain @RestController annotation in Spring boot?
It is a combination of @Controller and @ResponseBody, used for creating a restful controller. It converts the response to JSON or XML. It ensures that data returned by each method will be written straight into the response body instead of returning a template.
10. What is the difference between @RestController and @Controller in Spring Boot?
@Controller Map of the model object to view or template and make it human readable but @RestController simply returns the object and object data is directly written in HTTP response as JSON or XML.
11. Describe the flow of HTTPS requests through the Spring Boot application?
On a high-level spring boot application follow the MVC pattern which is depicted in the below flow diagram.
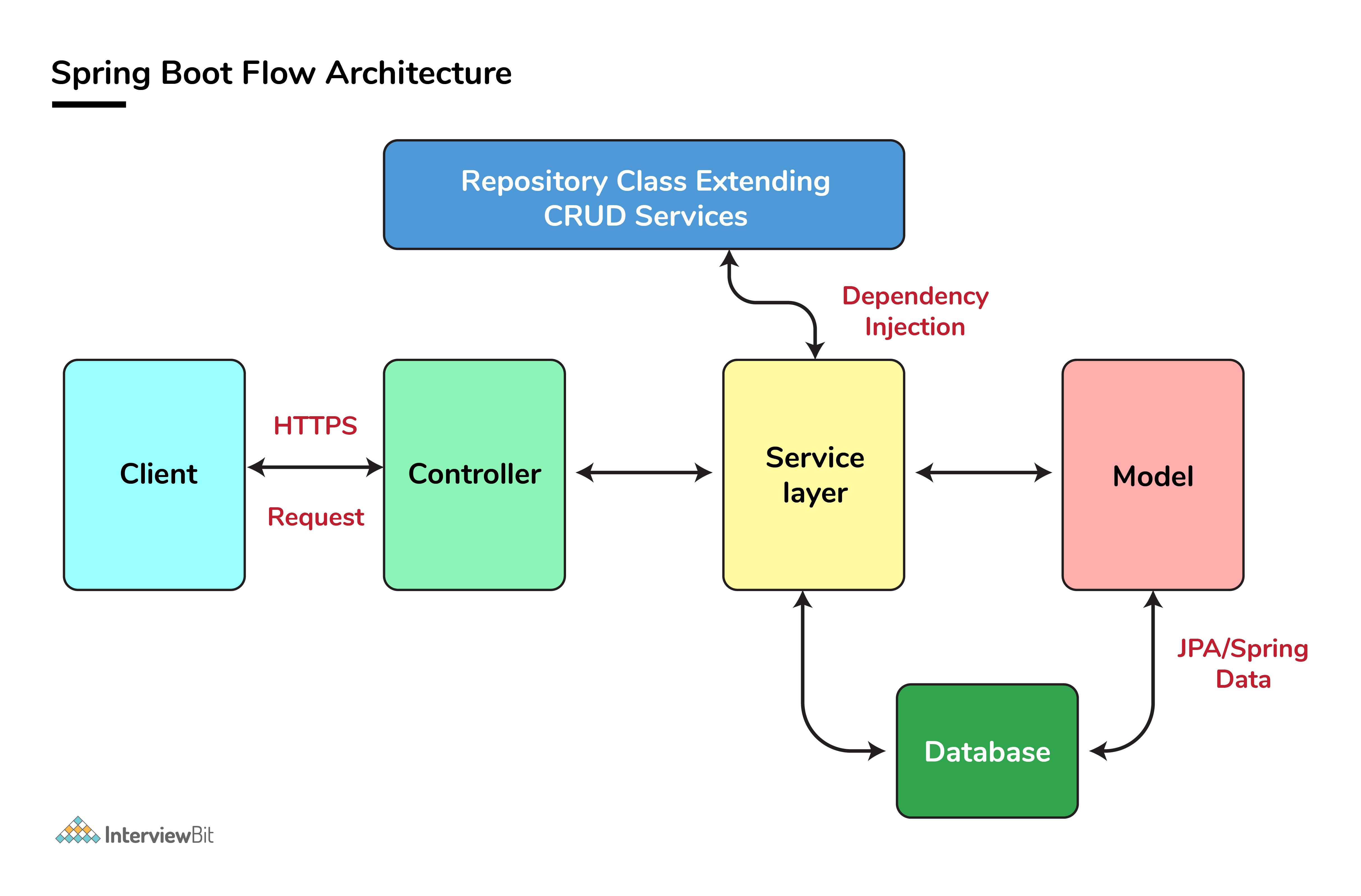
12. What is the difference between RequestMapping and GetMapping?
RequestMapping can be used with GET, POST, PUT, and many other request methods using the method attribute on the annotation. Whereas getMapping is only an extension of RequestMapping which helps you to improve on clarity on request.
13. What is the use of Profiles in spring boot?
While developing the application we deal with multiple environments such as dev, QA, Prod, and each environment requires a different configuration. For eg., we might be using an embedded H2 database for dev but for prod, we might have proprietary Oracle or DB2. Even if DBMS is the same across the environment, the URLs will be different.
To make this easy and clean, Spring has the provision of Profiles to keep the separate configuration of environments.
14. What is Spring Actuator? What are its advantages?
An actuator is an additional feature of Spring that helps you to monitor and manage your application when you push it to production. These actuators include auditing, health, CPU usage, HTTP hits, and metric gathering, and many more that are automatically applied to your application.
15. How to enable Actuator in Spring boot application?
To enable the spring actuator feature, we need to add the dependency of “spring-boot-starter-actuator” in pom.xml.
16. What are the actuator-provided endpoints used for monitoring the Spring boot application?
Actuators provide below pre-defined endpoints to monitor our application -
- Configprops
17. How to get the list of all the beans in your Spring boot application?
Spring Boot actuator “/Beans” is used to get the list of all the spring beans in your application.
18. How to check the environment properties in your Spring boot application?
Spring Boot actuator “/env” returns the list of all the environment properties of running the spring boot application.
19. How to enable debugging log in the spring boot application?
Debugging logs can be enabled in three ways -
- We can start the application with --debug switch.
- We can set the logging.level.root=debug property in application.property file.
- We can set the logging level of the root logger to debug in the supplied logging configuration file.
20. Where do we define properties in the Spring Boot application?
You can define both application and Spring boot-related properties into a file called application.properties. You can create this file manually or use Spring Initializer to create this file. You don’t need to do any special configuration to instruct Spring Boot to load this file, If it exists in classpath then spring boot automatically loads it and configure itself and the application code accordingly.
21. What is dependency Injection?
The process of injecting dependent bean objects into target bean objects is called dependency injection.
- Setter Injection: The IOC container will inject the dependent bean object into the target bean object by calling the setter method.
- Constructor Injection: The IOC container will inject the dependent bean object into the target bean object by calling the target bean constructor.
- Field Injection: The IOC container will inject the dependent bean object into the target bean object by Reflection API.
22. What is an IOC container?
IoC Container is a framework for implementing automatic dependency injection. It manages object creation and its life-time and also injects dependencies into the class.
Important Resources
- Java Spring Boot Free Course with Certificate
- Spring vs Spring Boot
- Difference Between Spring MVC and Spring Boot
- Spring Interview Questions
- Spring Security Interview Questions
Spring Boot MCQ Questions
Spring is used for?
Default HTML template engine in Spring Boot?
Annotation used for handling GET requests?
Annotation used for Rest Controller?
Which annotation is not Spring Boot Annotation?
Minimum Java version needed for Spring Boot?
Which of the following is used by Maven?
Is Dependency needed to create a Spring Boot web application?
Starting points of Spring Boot Application?
Database object must be annotated with?
- Privacy Policy
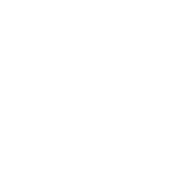
- Practice Questions
- Programming
- System Design
- Fast Track Courses
- Online Interviewbit Compilers
- Online C Compiler
- Online C++ Compiler
- Online Java Compiler
- Online Javascript Compiler
- Online Python Compiler
- Interview Preparation
- Java Interview Questions
- Sql Interview Questions
- Python Interview Questions
- Javascript Interview Questions
- Angular Interview Questions
- Networking Interview Questions
- Selenium Interview Questions
- Data Structure Interview Questions
- Data Science Interview Questions
- System Design Interview Questions
- Hr Interview Questions
- Html Interview Questions
- C Interview Questions
- Amazon Interview Questions
- Facebook Interview Questions
- Google Interview Questions
- Tcs Interview Questions
- Accenture Interview Questions
- Infosys Interview Questions
- Capgemini Interview Questions
- Wipro Interview Questions
- Cognizant Interview Questions
- Deloitte Interview Questions
- Zoho Interview Questions
- Hcl Interview Questions
- Highest Paying Jobs In India
- Exciting C Projects Ideas With Source Code
- Top Java 8 Features
- Angular Vs React
- 10 Best Data Structures And Algorithms Books
- Best Full Stack Developer Courses
- Best Data Science Courses
- Python Commands List
- Data Scientist Salary
- Maximum Subarray Sum Kadane’s Algorithm
- Python Cheat Sheet
- C++ Cheat Sheet
- Javascript Cheat Sheet
- Git Cheat Sheet
- Java Cheat Sheet
- Data Structure Mcq
- C Programming Mcq
- Javascript Mcq
1 Million +

IMAGES
VIDEO
COMMENTS
2. Grokking the Java Interview [My Book]. The Grokking the Java Interview books contain frequently asked Java questions from important topics like: 1. Object-Oriented Programming 2. Java ...
System Scalability is a important section of an interview. The book covers the theory well in its last chapter, even if it's Java-agnostic, something other books fail to address. Also contains Java 8+ functional programming exercises. Cons: It's worth emphasizing the word "coding" in the title. The book focuses on Java coding questions.
Java is well-known for its robustness in Object-Oriented Programming (OOP), and it provides a comprehensive foundation essential for developers at every level. This handbook offers a detailed pathway to help you excel in Java interviews. It focuses on delivering insights and techniques relevant to roles in esteemed big tech companies, ensuring ...
2. Grokking the Java Interview (My Book). This is my first book after 10 years of blogging and it contains 150 pages of interview questions, ideal if you have Java interviews in a couple of days ...
Product information. Title: The Complete Coding Interview Guide in Java. Author (s): Anghel Leonard. Release date: August 2020. Publisher (s): Packt Publishing. ISBN: 9781839212062. Explore a wide variety of popular interview questions and learn various techniques for breaking down tricky bits of code and algorithms into manageable chunks Key ...
This is a deeply technical book and focuses on the software engineering skills to ace your interview. The book is over 500 pages and includes 150 programming ... 301 pages, 7.4 x 9, $18, 150+ problems (C, C++, C#, Java) All four of these fine prep texts cover the usual suspects in Algorithms and Data structures, including a focus on "scalable ...
The Well-Grounded Java Developer: Vital techniques of Java 7 and polyglot programming. 7. Java Concurrency in Practice. 8. Effective Java. If you want to read just one book, read this one. 9. Java ...
Java Interview Questions and Answers. The pressure to perform well in an interview can feel overwhelming. Despite your knowledge and experience, nervousness could get in the way of putting your best foot forward. But, if you know the questions to expect and how to answer them, you can relax and focus on showcasing what you know and what you can do.
In my opinion this is one of the best books that I've ever read for Java interview preparation books. It is summarised, concrete and focused on the really most asked and essential Java interview questions. I think this book is a treasure for every developer in Java that wants to prepare efficiently for the ongoing Java interviews.
The Complete Coding Interview Guide in Java Paperback - 28 August 2020. The Complete Coding Interview Guide in Java. Paperback - 28 August 2020. by Anghel Leonard (Author, Contributor) 4.6 56 ratings. See all formats and editions. EMI starts at ₹115. No Cost EMI available EMI options. Save Extra with 3 offers.
Chapter 1: Where to Start and How to Prepare for the Interview. This chapter is a comprehensive guide that tackles the preparation process for a Java interview from the very start, to getting hired. More precisely, we want to highlight the main checkpoints that can ensure a smooth and successful career road ahead.
This book covers the following exciting features: Solve the most popular Java coding problems efficiently Tackle challenging algorithms that will help you develop robust and fast logic Practice answering commonly asked non-technical interview questions that can make the difference between a pass and a fail Get an overall picture of prospective ...
This guide having 200+ questions will help you revise most asked Java interview questions from beginner level to experienced and asked in small startups to big corporates. This guide starts with basic core java questions and progresses towards more advanced topics. I have tried to add answers to most questions and links to resources to read ...
Here's a quick list of the best coding interview prep sites/resources. Best technical interview prep course: Interview Cake. Best text-based code interview course: Grokking the Coding Interview. Best program for mock interviews/ career support: Interview Kickstart.
You Guys can find books on all Java Developer topics mentioned below, Core Java, Spring-Boot, Microservice, SQL. This will be complete preparation material for you guys. I am clubbing all the books down below. Here are my Books. I have written two Java interview books to help developer succeed in there interview.
Ace the interview and get the Java programming job you deserve. Java Programming Interviews Exposed is written to specifically prepare you for the questions you'll face when interviewing for highly sought-after jobs in Java. Expert interviewer and Java developer Noel Markham has teamed with Interview Zen to provide challenging Java-specific questions and answers compiled from over 20,000 ...
If you're trying to be a competitive candidate across big tech, then this book might be worth checking out. However, it's probably more important to study coding interview-specific topics like data structures and algorithms if you're pressed for time. 8. Clean Code: A Handbook of Agile Software Craftsmanship.
Java is the most used language in top companies such as Uber, Airbnb, Google, Netflix, Instagram, Spotify, Amazon, and many more because of its features and performance. In this article, we will provide 200+ Core Java Interview Questions tailored for both freshers and experienced professionals with 3, 5, and 8 years of experience.
Find my book Guide To Clear Java Developer Interview here Gumroad (PDF Format) and Amazon (Kindle eBook). Guide To Clear Spring-Boot Microservice Interview here Gumroa d ( PDF Format ) and Amazon ...
GRAB YOUR COPY OF CODING INTERVIEWS: QUESTIONS, ANALYSIS & SOLUTIONS. 5. Elements of Programming Interviews in C++. Elements of Programming Interviews in C++ by Adnan Aziz, Tsung-Hsien Lee and Amit Prakash is one of the best coding interview books out there.
In short: EPI is a highly technical book that is well-suited for anyone looking to prepare for technical coding questions that revolve around data structures and algorithms. The things that I particularly enjoyed: comes in 3 different versions: Java, Python and C++.
Write a Java Program to check if any number is a magic number or not. A number is said to be a magic number if after doing sum of digits in each step and inturn doing sum of digits of that sum, the ultimate result (when there is only one digit left) is 1. Example, consider the number: Step 1: 163 => 1+6+3 = 10.
2. System Design Interview Part 1 and 2 By Alex Xu. System design interview is an integral part of coding interviews and this book help you to prepare how to answer System design questions like ...
Following are the top 8 TOEFL Prep Books for 2024: "The Official Guide to the TOEFL Test" by ETS. "TOEFL iBT Prep Plus 2024" by Kaplan Test Prep. "Barron's TOEFL iBT with Online Tests" by Pamela J. Sharpe Ph.D. "Cambridge Preparation for the TOEFL Test" by Jolene Gear and Robert Gear.
Interview Guides All Problems Fast Track Courses Community Blog Interview Preparation Kit Video Courses. Contests ... Spring boot is the hottest topic of discussion in interviews if it comes to Java Application development. Because of its fast, low configuration, inbuilt server, and monitoring features, it helps to build a stand-alone Java ...